
uIP 1.0 based webserver for LPC1114 + ENC28J60
Functions used by an application running of top of uIP. More...
Functions | |
void | uip_listen (uint16_t port) |
Start listening to the specified port. | |
void | uip_unlisten (uint16_t port) |
Stop listening to the specified port. | |
struct uip_conn * | uip_connect (uip_ipaddr_t *ripaddr, uint16_t port) |
Connect to a remote host using TCP. | |
CCIF void | uip_send (const void *data, int len) |
Send data on the current connection. | |
struct uip_udp_conn * | uip_udp_new (const uip_ipaddr_t *ripaddr, uint16_t rport) |
Set up a new UDP connection. |
Detailed Description
Functions used by an application running of top of uIP.
Function Documentation
struct uip_conn* uip_connect | ( | uip_ipaddr_t * | ripaddr, |
uint16_t | port | ||
) | [read] |
Connect to a remote host using TCP.
This function is used to start a new connection to the specified port on the specified host. It allocates a new connection identifier, sets the connection to the SYN_SENT state and sets the retransmission timer to 0. This will cause a TCP SYN segment to be sent out the next time this connection is periodically processed, which usually is done within 0.5 seconds after the call to uip_connect().
- Note:
- This function is available only if support for active open has been configured by defining UIP_ACTIVE_OPEN to 1 in uipopt.h.
- Since this function requires the port number to be in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_ipaddr_t ipaddr; uip_ipaddr(&ipaddr, 192,168,1,2); uip_connect(&ipaddr, UIP_HTONS(80));
- Parameters:
-
ripaddr The IP address of the remote host. port A 16-bit port number in network byte order.
- Returns:
- A pointer to the uIP connection identifier for the new connection, or NULL if no connection could be allocated.
void uip_listen | ( | uint16_t | port ) |
Start listening to the specified port.
- Note:
- Since this function expects the port number in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_listen(UIP_HTONS(80));
- Parameters:
-
port A 16-bit port number in network byte order.
CCIF void uip_send | ( | const void * | data, |
int | len | ||
) |
Send data on the current connection.
This function is used to send out a single segment of TCP data. Only applications that have been invoked by uIP for event processing can send data.
The amount of data that actually is sent out after a call to this function is determined by the maximum amount of data TCP allows. uIP will automatically crop the data so that only the appropriate amount of data is sent. The function uip_mss() can be used to query uIP for the amount of data that actually will be sent.
- Note:
- This function does not guarantee that the sent data will arrive at the destination. If the data is lost in the network, the application will be invoked with the uip_rexmit() event being set. The application will then have to resend the data using this function.
- Parameters:
-
data A pointer to the data which is to be sent. len The maximum amount of data bytes to be sent.
struct uip_udp_conn* uip_udp_new | ( | const uip_ipaddr_t * | ripaddr, |
uint16_t | rport | ||
) | [read] |
Set up a new UDP connection.
This function sets up a new UDP connection. The function will automatically allocate an unused local port for the new connection. However, another port can be chosen by using the uip_udp_bind() call, after the uip_udp_new() function has been called.
Example:
uip_ipaddr_t addr; struct uip_udp_conn *c; uip_ipaddr(&addr, 192,168,2,1); c = uip_udp_new(&addr, UIP_HTONS(12345)); if(c != NULL) { uip_udp_bind(c, UIP_HTONS(12344)); }
- Parameters:
-
ripaddr The IP address of the remote host. rport The remote port number in network byte order.
- Returns:
- The uip_udp_conn structure for the new connection, or NULL if no connection could be allocated.
void uip_unlisten | ( | uint16_t | port ) |
Stop listening to the specified port.
- Note:
- Since this function expects the port number in network byte order, a conversion using UIP_HTONS() or uip_htons() is necessary.
uip_unlisten(UIP_HTONS(80));
- Parameters:
-
port A 16-bit port number in network byte order.
Generated on Tue Jul 12 2022 12:52:12 by
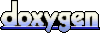