for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
TCPSocketServer.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00019 * port to the muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00020 */ 00021 #include "TCPSocketServer.h" 00022 #include "SNIC_Core.h" 00023 00024 #include <cstring> 00025 00026 TCPSocketServer::TCPSocketServer() 00027 { 00028 } 00029 00030 TCPSocketServer::~TCPSocketServer() 00031 { 00032 } 00033 00034 int TCPSocketServer::bind(unsigned short port) 00035 { 00036 int ret; 00037 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00038 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00039 00040 snic_core_p->lockAPI(); 00041 // Get local ip address. 00042 // Get buffer for response payload from MemoryPool 00043 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00044 if( payload_buf_p == NULL ) 00045 { 00046 DEBUG_PRINT("bind payload_buf_p NULL\r\n"); 00047 snic_core_p->unlockAPI(); 00048 return -1; 00049 } 00050 00051 C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T req; 00052 // Make request 00053 req.cmd_sid = UART_CMD_SID_SNIC_GET_DHCP_INFO_REQ; 00054 req.seq = mUartRequestSeq++; 00055 req.interface = 0; 00056 00057 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00058 unsigned int command_len; 00059 // Preparation of command 00060 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00061 , sizeof(C_SNIC_Core::tagSNIC_GET_DHCP_INFO_REQ_T), payload_buf_p->buf, command_array_p ); 00062 // Send uart command request 00063 snic_core_p->sendUart( command_len, command_array_p ); 00064 // Wait UART response 00065 ret = uartCmdMgr_p->wait(); 00066 if( ret != 0 ) 00067 { 00068 DEBUG_PRINT( "bind failed\r\n" ); 00069 snic_core_p->freeCmdBuf( payload_buf_p ); 00070 snic_core_p->unlockAPI(); 00071 return -1; 00072 } 00073 00074 if( uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_SNIC_SUCCESS ) 00075 { 00076 DEBUG_PRINT("bind status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00077 snic_core_p->freeCmdBuf( payload_buf_p ); 00078 snic_core_p->unlockAPI(); 00079 return -1; 00080 } 00081 00082 snic_core_p->freeCmdBuf( payload_buf_p ); 00083 snic_core_p->unlockAPI(); 00084 00085 unsigned int local_addr = (payload_buf_p->buf[9] << 24) 00086 | (payload_buf_p->buf[10] << 16) 00087 | (payload_buf_p->buf[11] << 8) 00088 | (payload_buf_p->buf[12]); 00089 00090 // Socket create 00091 ret = createSocket( 1, local_addr, port ); 00092 if( ret != 0 ) 00093 { 00094 DEBUG_PRINT("bind error : %d\r\n", ret); 00095 return -1; 00096 } 00097 00098 return 0; 00099 } 00100 00101 int TCPSocketServer::listen(int max) 00102 { 00103 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00104 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00105 00106 snic_core_p->lockAPI(); 00107 // Get buffer for response payload from MemoryPool 00108 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00109 if( payload_buf_p == NULL ) 00110 { 00111 DEBUG_PRINT("listen payload_buf_p NULL\r\n"); 00112 snic_core_p->unlockAPI(); 00113 return -1; 00114 } 00115 00116 C_SNIC_Core::tagSNIC_TCP_CREATE_CONNECTION_REQ_T req; 00117 // Make request 00118 req.cmd_sid = UART_CMD_SID_SNIC_TCP_CREATE_CONNECTION_REQ; 00119 req.seq = mUartRequestSeq++; 00120 req.socket_id = mSocketID; 00121 req.recv_bufsize[0] = ( (SNIC_UART_RECVBUF_SIZE & 0xFF00) >> 8 ); 00122 req.recv_bufsize[1] = (SNIC_UART_RECVBUF_SIZE & 0xFF); 00123 req.max_client = max; 00124 00125 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00126 unsigned int command_len; 00127 // Preparation of command 00128 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00129 , sizeof(C_SNIC_Core::tagSNIC_TCP_CREATE_CONNECTION_REQ_T), payload_buf_p->buf, command_array_p ); 00130 00131 int ret; 00132 00133 // Send uart command request 00134 snic_core_p->sendUart( command_len, command_array_p ); 00135 00136 // Wait UART response 00137 ret = uartCmdMgr_p->wait(); 00138 if( ret != 0 ) 00139 { 00140 DEBUG_PRINT( "listen failed\r\n" ); 00141 snic_core_p->freeCmdBuf( payload_buf_p ); 00142 snic_core_p->unlockAPI(); 00143 return -1; 00144 } 00145 00146 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00147 { 00148 DEBUG_PRINT("listen status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00149 snic_core_p->freeCmdBuf( payload_buf_p ); 00150 snic_core_p->unlockAPI(); 00151 return -1; 00152 } 00153 00154 snic_core_p->freeCmdBuf( payload_buf_p ); 00155 snic_core_p->unlockAPI(); 00156 return 0; 00157 } 00158 00159 int TCPSocketServer::accept(TCPSocketConnection *connection) 00160 { 00161 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00162 int i; 00163 int ret = -1; 00164 00165 C_SNIC_Core::tagCONNECT_INFO_T *con_info_p; 00166 for( i = 0; i < MAX_SOCKET_ID+1; i++ ) 00167 { 00168 // Get connection information 00169 con_info_p = snic_core_p->getConnectInfo( i ); 00170 if( (con_info_p->is_connected == true) 00171 && (con_info_p->is_accept == true) 00172 && (con_info_p->parent_socket == mSocketID) ) 00173 { 00174 // Set socket id 00175 connection->setAcceptSocket( i ); 00176 ret = 0; 00177 } 00178 } 00179 00180 return ret; 00181 }
Generated on Tue Jul 12 2022 18:43:50 by
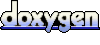