for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_WifiInterface.h
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD-SNIC UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #ifndef _SNIC_WIFIINTERFACE_H_ 00020 #define _SNIC_WIFIINTERFACE_H_ 00021 00022 #include "SNIC_Core.h" 00023 #include "MurataObject.h" 00024 00025 /** Wi-Fi status used by getWifiStatus(). */ 00026 typedef struct 00027 { 00028 /** status */ 00029 E_WIFI_STATUS status; 00030 /** Mac address */ 00031 char mac_address[BSSID_MAC_LENTH]; 00032 /** SSID */ 00033 char ssid[SSID_MAX_LENGTH+1]; 00034 }tagWIFI_STATUS_T; 00035 00036 /** Interface class for using SNIC UART. 00037 */ 00038 class C_SNIC_WifiInterface : public C_MurataObject { 00039 00040 public: 00041 /** Constructor 00042 @param tx mbed pin to use for tx line of Serial interface 00043 @param rx mbed pin to use for rx line of Serial interface 00044 @param cts mbed pin to use for cts line of Serial interface 00045 @param rts mbed pin to use for rts line of Serial interface 00046 @param reset reset pin of the wifi module 00047 @param alarm alarm pin of the wifi module (default: NC) 00048 @param baud baud rate of Serial interface (default: 115200) 00049 */ 00050 C_SNIC_WifiInterface(PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName alarm = NC, int baud = 115200); 00051 virtual ~C_SNIC_WifiInterface(); 00052 00053 /** Initialize the interface. 00054 @return 0 on success, a negative number on failure 00055 */ 00056 int init(); 00057 00058 /** Get Firmware version string. 00059 @param version_p Pointer of FW version string.(null terminated)[output] 00060 @return 0:success/other:fail 00061 @note This function is blocked until a returns. 00062 When you use it by UI thread, be careful. 00063 */ 00064 int getFWVersion( unsigned char *version_p ); 00065 00066 /** Connect to AP 00067 @param ssid_p Wi-Fi SSID(null terminated) 00068 @param ssid_len Wi-Fi SSID length 00069 @param sec_type Wi-Fi security type. 00070 @param sec_key_len Wi-Fi passphrase or security key length 00071 @param sec_key_p Wi-Fi passphrase or security key 00072 @return 0 on success, a negative number on failure 00073 @note This function is blocked until a returns. 00074 When you use it by UI thread, be careful. 00075 */ 00076 int connect(const char *ssid_p, unsigned char ssid_len, E_SECURITY sec_type, const char *sec_key_p, unsigned char sec_key_len); 00077 00078 /** Disconnect from AP 00079 @return 0 on success, a negative number on failure 00080 @note This function is blocked until a returns. 00081 When you use it by UI thread, be careful. 00082 */ 00083 int disconnect(); 00084 00085 /** Scan AP 00086 @param ssid_p Wi-Fi SSID(null terminated) 00087 If do not specify SSID, set to NULL. 00088 @param bssid_p Wi-Fi BSSID(null terminated) 00089 If do not specify SSID, set to NULL. 00090 @param result_handler_p Pointer of scan result callback function. 00091 @return 0 on success, a negative number on failure 00092 @note This function is blocked until a returns. 00093 When you use it by UI thread, be careful. 00094 Scan results will be notified by asynchronous callback function. 00095 If there is no continuity data, scan_result will be set NULL.. 00096 */ 00097 int scan( const char *ssid_p, unsigned char *bssid_p 00098 ,void (*result_handler_p)(tagSCAN_RESULT_T *scan_result) ); 00099 00100 /** Wi-Fi Turn on 00101 @param country_p Pointer of country code. 00102 @return 0 on success, a negative number on failure 00103 @note This function is blocked until a returns. 00104 When you use it by UI thread, be careful. 00105 */ 00106 int wifi_on( const char *country_p ); 00107 00108 /** Wi-Fi Turn off 00109 @return 0 on success, a negative number on failure 00110 @note This function is blocked until a returns. 00111 When you use it by UI thread, be careful. 00112 */ 00113 int wifi_off(); 00114 00115 /** Get Wi-Fi RSSI 00116 @param rssi_p Pointer of RSSI.[output] 00117 @return 0 on success, a negative number on failure 00118 @note This function is blocked until a returns. 00119 When you use it by UI thread, be careful. 00120 */ 00121 int getRssi( signed char *rssi_p ); 00122 00123 /** Get Wi-Fi status 00124 @param status_p Pointer of status structure.[output] 00125 @return 0 on success, a negative number on failure 00126 @note This function is blocked until a returns. 00127 When you use it by UI thread, be careful. 00128 */ 00129 int getWifiStatus( tagWIFI_STATUS_T *status_p); 00130 00131 /** Set IP configuration 00132 @param is_DHCP true:DHCP false:static IP. 00133 @param ip_p Pointer of strings of IP address.(null terminate). 00134 @param mask_p Pointer of strings of Netmask.(null terminate). 00135 @param gateway_p Pointer of strings of gateway address.(null terminate). 00136 @return 0 on success, a negative number on failure 00137 @note This function is blocked until a returns. 00138 When you use it by UI thread, be careful. 00139 */ 00140 int setIPConfig( bool is_DHCP, const char *ip_p=NULL, const char *mask_p=NULL, const char *gateway_p=NULL ); 00141 00142 private: 00143 PinName mUART_tx; 00144 PinName mUART_rx; 00145 PinName mUART_cts; 00146 PinName mUART_rts; 00147 int mUART_baud; 00148 PinName mModuleReset; 00149 }; 00150 #endif /* _YD_WIFIINTERFACE_H_ */
Generated on Tue Jul 12 2022 18:43:50 by
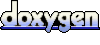