for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_WifiInterface.cpp
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD-SNIC UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "SNIC_WifiInterface.h" 00020 #include "SNIC_UartMsgUtil.h" 00021 00022 #define UART_CONNECT_BUF_SIZE 512 00023 unsigned char gCONNECT_BUF[UART_CONNECT_BUF_SIZE]; 00024 00025 C_SNIC_WifiInterface::C_SNIC_WifiInterface( PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, PinName alarm, int baud) 00026 { 00027 mUART_tx = tx; 00028 mUART_rx = rx; 00029 mUART_cts = cts; 00030 mUART_rts = rts;; 00031 mUART_baud = baud; 00032 mModuleReset = reset; 00033 } 00034 00035 C_SNIC_WifiInterface::~C_SNIC_WifiInterface() 00036 { 00037 } 00038 00039 int C_SNIC_WifiInterface::init() 00040 { 00041 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00042 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00043 00044 /* Initialize UART */ 00045 snic_core_p->initUart( mUART_tx, mUART_rx, mUART_baud ); 00046 00047 /* Module reset */ 00048 snic_core_p->resetModule( mModuleReset ); 00049 00050 wait(1); 00051 /* Initialize SNIC API */ 00052 // Get buffer for response payload from MemoryPool 00053 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00054 if( payload_buf_p == NULL ) 00055 { 00056 DEBUG_PRINT("snic_init payload_buf_p NULL\r\n"); 00057 return -1; 00058 } 00059 00060 C_SNIC_Core::tagSNIC_INIT_REQ_T req; 00061 // Make request 00062 req.cmd_sid = UART_CMD_SID_SNIC_INIT_REQ; 00063 req.seq = mUartRequestSeq++; 00064 req.buf_size[0] = 0x08; 00065 req.buf_size[1] = 0x00; 00066 00067 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00068 unsigned int command_len; 00069 // Preparation of command 00070 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00071 , sizeof(C_SNIC_Core::tagSNIC_INIT_REQ_T), payload_buf_p->buf, command_array_p ); 00072 00073 // Send uart command request 00074 snic_core_p->sendUart( command_len, command_array_p ); 00075 00076 int ret; 00077 // Wait UART response 00078 ret = uartCmdMgr_p->wait(); 00079 if( ret != 0 ) 00080 { 00081 DEBUG_PRINT( "snic_init failed\r\n" ); 00082 snic_core_p->freeCmdBuf( payload_buf_p ); 00083 return -1; 00084 } 00085 00086 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00087 { 00088 DEBUG_PRINT("snic_init status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00089 snic_core_p->freeCmdBuf( payload_buf_p ); 00090 return -1; 00091 } 00092 snic_core_p->freeCmdBuf( payload_buf_p ); 00093 00094 return ret; 00095 } 00096 00097 int C_SNIC_WifiInterface::getFWVersion( unsigned char *version_p ) 00098 { 00099 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00100 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00101 00102 // Get buffer for response payload from MemoryPool 00103 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00104 if( payload_buf_p == NULL ) 00105 { 00106 DEBUG_PRINT("getFWVersion payload_buf_p NULL\r\n"); 00107 return -1; 00108 } 00109 00110 C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T req; 00111 // Make request 00112 req.cmd_sid = UART_CMD_SID_GEN_FW_VER_GET_REQ; 00113 req.seq = mUartRequestSeq++; 00114 00115 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00116 unsigned int command_len; 00117 // Preparation of command 00118 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_GEN, req.cmd_sid, (unsigned char *)&req 00119 , sizeof(C_SNIC_Core::tagGEN_FW_VER_GET_REQ_T), payload_buf_p->buf, command_array_p ); 00120 00121 int ret; 00122 00123 // Send uart command request 00124 snic_core_p->sendUart( command_len, command_array_p ); 00125 00126 // Wait UART response 00127 ret = uartCmdMgr_p->wait(); 00128 if( ret != 0 ) 00129 { 00130 DEBUG_PRINT( "getFWversion failed\r\n" ); 00131 snic_core_p->freeCmdBuf( payload_buf_p ); 00132 return -1; 00133 } 00134 00135 if( uartCmdMgr_p->getCommandStatus() == 0 ) 00136 { 00137 unsigned char version_len = payload_buf_p->buf[3]; 00138 memcpy( version_p, &payload_buf_p->buf[4], version_len ); 00139 } 00140 snic_core_p->freeCmdBuf( payload_buf_p ); 00141 return 0; 00142 } 00143 00144 int C_SNIC_WifiInterface::connect(const char *ssid_p, unsigned char ssid_len, E_SECURITY sec_type 00145 , const char *sec_key_p, unsigned char sec_key_len) 00146 { 00147 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00148 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00149 00150 // Parameter check(SSID) 00151 if( (ssid_p == NULL) || (ssid_len == 0) ) 00152 { 00153 DEBUG_PRINT( "connect failed [ parameter NG:SSID ]\r\n" ); 00154 return -1; 00155 } 00156 00157 // Parameter check(Security key) 00158 if( (sec_type != e_SEC_OPEN) && ( (sec_key_len == 0) || (sec_key_p == NULL) ) ) 00159 { 00160 DEBUG_PRINT( "connect failed [ parameter NG:Security key ]\r\n" ); 00161 return -1; 00162 } 00163 00164 // Get buffer for response payload from MemoryPool 00165 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00166 if( payload_buf_p == NULL ) 00167 { 00168 DEBUG_PRINT("connect payload_buf_p NULL\r\n"); 00169 return -1; 00170 } 00171 00172 unsigned char *buf = &gCONNECT_BUF[0]; 00173 unsigned int buf_len = 0; 00174 unsigned int command_len; 00175 00176 memset( buf, 0, UART_CONNECT_BUF_SIZE ); 00177 // Make request 00178 buf[0] = UART_CMD_SID_WIFI_JOIN_REQ; 00179 buf_len++; 00180 buf[1] = mUartRequestSeq++; 00181 buf_len++; 00182 // SSID 00183 memcpy( &buf[2], ssid_p, ssid_len ); 00184 buf_len += ssid_len; 00185 buf_len++; 00186 00187 // Security mode 00188 buf[ buf_len ] = (unsigned char)sec_type; 00189 buf_len++; 00190 00191 // Security key 00192 if( sec_type != e_SEC_OPEN ) 00193 { 00194 buf[ buf_len ] = sec_key_len; 00195 buf_len++; 00196 if( sec_key_len > 0 ) 00197 { 00198 memcpy( &buf[buf_len], sec_key_p, sec_key_len ); 00199 buf_len += sec_key_len; 00200 } 00201 } 00202 00203 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00204 // Preparation of command 00205 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, UART_CMD_SID_WIFI_JOIN_REQ, buf 00206 , buf_len, payload_buf_p->buf, command_array_p ); 00207 00208 // Send uart command request 00209 snic_core_p->sendUart( command_len, command_array_p ); 00210 00211 int ret; 00212 // Wait UART response 00213 ret = uartCmdMgr_p->wait(); 00214 if(uartCmdMgr_p->getCommandStatus() != UART_CMD_RES_WIFI_ERR_ALREADY_JOINED) 00215 { 00216 DEBUG_PRINT( "Already connected\r\n" ); 00217 } 00218 else 00219 { 00220 if( ret != 0 ) 00221 { 00222 DEBUG_PRINT( "join failed\r\n" ); 00223 snic_core_p->freeCmdBuf( payload_buf_p ); 00224 return -1; 00225 } 00226 } 00227 00228 if(uartCmdMgr_p->getCommandStatus() != 0) 00229 { 00230 DEBUG_PRINT("join status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00231 snic_core_p->freeCmdBuf( payload_buf_p ); 00232 return -1; 00233 } 00234 snic_core_p->freeCmdBuf( payload_buf_p ); 00235 00236 return ret; 00237 } 00238 00239 int C_SNIC_WifiInterface::disconnect() 00240 { 00241 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00242 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00243 00244 // Get buffer for response payload from MemoryPool 00245 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00246 if( payload_buf_p == NULL ) 00247 { 00248 DEBUG_PRINT("disconnect payload_buf_p NULL\r\n"); 00249 return -1; 00250 } 00251 00252 C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T req; 00253 // Make request 00254 req.cmd_sid = UART_CMD_SID_WIFI_DISCONNECT_REQ; 00255 req.seq = mUartRequestSeq++; 00256 00257 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00258 unsigned int command_len; 00259 // Preparation of command 00260 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00261 , sizeof(C_SNIC_Core::tagWIFI_DISCONNECT_REQ_T), payload_buf_p->buf, command_array_p ); 00262 00263 // Send uart command request 00264 snic_core_p->sendUart( command_len, command_array_p ); 00265 00266 int ret; 00267 // Wait UART response 00268 ret = uartCmdMgr_p->wait(); 00269 if( ret != 0 ) 00270 { 00271 DEBUG_PRINT( "disconnect failed\r\n" ); 00272 snic_core_p->freeCmdBuf( payload_buf_p ); 00273 return -1; 00274 } 00275 00276 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00277 { 00278 DEBUG_PRINT("disconnect status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00279 ret = -1; 00280 } 00281 snic_core_p->freeCmdBuf( payload_buf_p ); 00282 return ret; 00283 } 00284 00285 int C_SNIC_WifiInterface::scan( const char *ssid_p, unsigned char *bssid_p 00286 , void (*result_handler_p)(tagSCAN_RESULT_T *scan_result) ) 00287 { 00288 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00289 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00290 00291 // Get buffer for response payload from MemoryPool 00292 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00293 if( payload_buf_p == NULL ) 00294 { 00295 DEBUG_PRINT("scan payload_buf_p NULL\r\n"); 00296 return -1; 00297 } 00298 00299 C_SNIC_Core::tagWIFI_SCAN_REQ_T req; 00300 unsigned int buf_len = 0; 00301 00302 memset( &req, 0, sizeof(C_SNIC_Core::tagWIFI_SCAN_REQ_T) ); 00303 // Make request 00304 req.cmd_sid = UART_CMD_SID_WIFI_SCAN_REQ; 00305 buf_len++; 00306 req.seq = mUartRequestSeq++; 00307 buf_len++; 00308 00309 // Set scan type(Active scan) 00310 req.scan_type = 0; 00311 buf_len++; 00312 // Set bss type(any) 00313 req.bss_type = 2; 00314 buf_len++; 00315 // Set BSSID 00316 if( bssid_p != NULL ) 00317 { 00318 memcpy( req.bssid, bssid_p, BSSID_MAC_LENTH ); 00319 } 00320 buf_len += BSSID_MAC_LENTH; 00321 // Set channel list(0) 00322 req.chan_list = 0; 00323 buf_len++; 00324 //Set SSID 00325 if( ssid_p != NULL ) 00326 { 00327 strcpy( (char *)req.ssid, ssid_p ); 00328 buf_len += strlen(ssid_p); 00329 } 00330 buf_len++; 00331 00332 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00333 unsigned int command_len; 00334 // Preparation of command 00335 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00336 , buf_len, payload_buf_p->buf, command_array_p ); 00337 00338 // Set scan result callback 00339 uartCmdMgr_p->setScanResultHandler( result_handler_p ); 00340 00341 // Send uart command request 00342 snic_core_p->sendUart( command_len, command_array_p ); 00343 00344 int ret; 00345 // Wait UART response 00346 ret = uartCmdMgr_p->wait(); 00347 DEBUG_PRINT( "scan wait:%d\r\n", ret ); 00348 if( ret != 0 ) 00349 { 00350 DEBUG_PRINT( "scan failed\r\n" ); 00351 snic_core_p->freeCmdBuf( payload_buf_p ); 00352 return -1; 00353 } 00354 00355 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00356 { 00357 DEBUG_PRINT("scan status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00358 snic_core_p->freeCmdBuf( payload_buf_p ); 00359 return -1; 00360 } 00361 00362 snic_core_p->freeCmdBuf( payload_buf_p ); 00363 00364 return ret; 00365 } 00366 00367 int C_SNIC_WifiInterface::wifi_on( const char *country_p ) 00368 { 00369 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00370 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00371 00372 // Parameter check 00373 if( country_p == NULL ) 00374 { 00375 DEBUG_PRINT("wifi_on parameter error\r\n"); 00376 return -1; 00377 } 00378 00379 // Get buffer for response payload from MemoryPool 00380 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00381 if( payload_buf_p == NULL ) 00382 { 00383 DEBUG_PRINT("wifi_on payload_buf_p NULL\r\n"); 00384 return -1; 00385 } 00386 00387 C_SNIC_Core::tagWIFI_ON_REQ_T req; 00388 // Make request 00389 req.cmd_sid = UART_CMD_SID_WIFI_ON_REQ; 00390 req.seq = mUartRequestSeq++; 00391 memcpy( req.country, country_p, COUNTRYC_CODE_LENTH ); 00392 00393 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00394 unsigned int command_len; 00395 // Preparation of command 00396 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00397 , sizeof(C_SNIC_Core::tagWIFI_ON_REQ_T), payload_buf_p->buf, command_array_p ); 00398 00399 // Send uart command request 00400 snic_core_p->sendUart( command_len, command_array_p ); 00401 00402 int ret; 00403 // Wait UART response 00404 ret = uartCmdMgr_p->wait(); 00405 if( ret != 0 ) 00406 { 00407 DEBUG_PRINT( "wifi_on failed\r\n" ); 00408 snic_core_p->freeCmdBuf( payload_buf_p ); 00409 return -1; 00410 } 00411 00412 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00413 { 00414 DEBUG_PRINT("wifi_on status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00415 snic_core_p->freeCmdBuf( payload_buf_p ); 00416 return -1; 00417 } 00418 snic_core_p->freeCmdBuf( payload_buf_p ); 00419 00420 return ret; 00421 } 00422 00423 int C_SNIC_WifiInterface::wifi_off() 00424 { 00425 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00426 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00427 00428 // Get buffer for response payload from MemoryPool 00429 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00430 if( payload_buf_p == NULL ) 00431 { 00432 DEBUG_PRINT("wifi_off payload_buf_p NULL\r\n"); 00433 return -1; 00434 } 00435 00436 C_SNIC_Core::tagWIFI_OFF_REQ_T req; 00437 // Make request 00438 req.cmd_sid = UART_CMD_SID_WIFI_OFF_REQ; 00439 req.seq = mUartRequestSeq++; 00440 00441 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00442 unsigned int command_len; 00443 // Preparation of command 00444 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00445 , sizeof(C_SNIC_Core::tagWIFI_OFF_REQ_T), payload_buf_p->buf, command_array_p ); 00446 00447 // Send uart command request 00448 snic_core_p->sendUart( command_len, command_array_p ); 00449 00450 int ret; 00451 // Wait UART response 00452 ret = uartCmdMgr_p->wait(); 00453 if( ret != 0 ) 00454 { 00455 DEBUG_PRINT( "wifi_off failed\r\n" ); 00456 snic_core_p->freeCmdBuf( payload_buf_p ); 00457 return -1; 00458 } 00459 00460 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00461 { 00462 DEBUG_PRINT("wifi_off status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00463 snic_core_p->freeCmdBuf( payload_buf_p ); 00464 return -1; 00465 } 00466 snic_core_p->freeCmdBuf( payload_buf_p ); 00467 00468 return ret; 00469 } 00470 00471 int C_SNIC_WifiInterface::getRssi( signed char *rssi_p ) 00472 { 00473 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00474 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00475 if( rssi_p == NULL ) 00476 { 00477 DEBUG_PRINT("getRssi parameter error\r\n"); 00478 return -1; 00479 } 00480 00481 // Get buffer for response payload from MemoryPool 00482 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00483 if( payload_buf_p == NULL ) 00484 { 00485 DEBUG_PRINT("getRssi payload_buf_p NULL\r\n"); 00486 return -1; 00487 } 00488 00489 C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T req; 00490 00491 // Make request 00492 req.cmd_sid = UART_CMD_SID_WIFI_GET_STA_RSSI_REQ; 00493 req.seq = mUartRequestSeq++; 00494 00495 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00496 unsigned int command_len; 00497 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00498 , sizeof(C_SNIC_Core::tagWIFI_GET_STA_RSSI_REQ_T), payload_buf_p->buf, command_array_p ); 00499 00500 int ret; 00501 // Send uart command request 00502 snic_core_p->sendUart( command_len, command_array_p ); 00503 00504 // Wait UART response 00505 ret = uartCmdMgr_p->wait(); 00506 if( ret != 0 ) 00507 { 00508 DEBUG_PRINT( "getRssi failed\r\n" ); 00509 snic_core_p->freeCmdBuf( payload_buf_p ); 00510 return -1; 00511 } 00512 00513 *rssi_p = (signed char)payload_buf_p->buf[2]; 00514 00515 snic_core_p->freeCmdBuf( payload_buf_p ); 00516 return 0; 00517 } 00518 00519 int C_SNIC_WifiInterface::getWifiStatus( tagWIFI_STATUS_T *status_p) 00520 { 00521 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00522 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00523 00524 if( status_p == NULL ) 00525 { 00526 DEBUG_PRINT("getWifiStatus parameter error\r\n"); 00527 return -1; 00528 } 00529 00530 // Get buffer for response payload from MemoryPool 00531 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00532 if( payload_buf_p == NULL ) 00533 { 00534 DEBUG_PRINT("getWifiStatus payload_buf_p NULL\r\n"); 00535 return -1; 00536 } 00537 00538 C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T req; 00539 // Make request 00540 req.cmd_sid = UART_CMD_SID_WIFI_GET_STATUS_REQ; 00541 req.seq = mUartRequestSeq++; 00542 req.interface = 0; 00543 00544 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00545 unsigned int command_len; 00546 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_WIFI, req.cmd_sid, (unsigned char *)&req 00547 , sizeof(C_SNIC_Core::tagWIFI_GET_STATUS_REQ_T), payload_buf_p->buf, command_array_p ); 00548 00549 // Send uart command request 00550 snic_core_p->sendUart( command_len, command_array_p ); 00551 00552 int ret; 00553 // Wait UART response 00554 ret = uartCmdMgr_p->wait(); 00555 if( ret != 0 ) 00556 { 00557 DEBUG_PRINT( "getWifiStatus failed\r\n" ); 00558 snic_core_p->freeCmdBuf( payload_buf_p ); 00559 return -1; 00560 } 00561 00562 // set status 00563 status_p->status = (E_WIFI_STATUS)payload_buf_p->buf[2]; 00564 00565 // set Mac address 00566 if( status_p->status != e_STATUS_OFF ) 00567 { 00568 memcpy( status_p->mac_address, &payload_buf_p->buf[3], BSSID_MAC_LENTH ); 00569 } 00570 00571 // set SSID 00572 if( ( status_p->status == e_STA_JOINED ) || ( status_p->status == e_AP_STARTED ) ) 00573 { 00574 memcpy( status_p->ssid, &payload_buf_p->buf[9], strlen( (char *)&payload_buf_p->buf[9]) ); 00575 } 00576 00577 snic_core_p->freeCmdBuf( payload_buf_p ); 00578 return 0; 00579 } 00580 00581 int C_SNIC_WifiInterface::setIPConfig( bool is_DHCP 00582 , const char *ip_p, const char *mask_p, const char *gateway_p ) 00583 { 00584 // Parameter check 00585 if( is_DHCP == false ) 00586 { 00587 if( (ip_p == NULL) || (mask_p == NULL) ||(gateway_p == NULL) ) 00588 { 00589 DEBUG_PRINT("setIPConfig parameter error\r\n"); 00590 return -1; 00591 } 00592 } 00593 00594 C_SNIC_Core *snic_core_p = C_SNIC_Core::getInstance(); 00595 C_SNIC_UartCommandManager *uartCmdMgr_p = snic_core_p->getUartCommand(); 00596 00597 // Get buffer for response payload from MemoryPool 00598 tagMEMPOOL_BLOCK_T *payload_buf_p = snic_core_p->allocCmdBuf(); 00599 if( payload_buf_p == NULL ) 00600 { 00601 DEBUG_PRINT("setIPConfig payload_buf_p NULL\r\n"); 00602 return -1; 00603 } 00604 00605 unsigned char *command_array_p = snic_core_p->getCommandBuf(); 00606 unsigned int command_len; 00607 if( is_DHCP == true ) 00608 { 00609 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T req; 00610 // Make request 00611 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00612 req.seq = mUartRequestSeq++; 00613 req.interface = 0; 00614 req.dhcp = 1; 00615 00616 // Preparation of command 00617 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00618 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_DHCP_T), payload_buf_p->buf, command_array_p ); 00619 } 00620 else 00621 { 00622 C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T req; 00623 // Make request 00624 req.cmd_sid = UART_CMD_SID_SNIC_IP_CONFIG_REQ; 00625 req.seq = mUartRequestSeq++; 00626 req.interface = 0; 00627 req.dhcp = 0; 00628 00629 // Set paramter of address 00630 int addr_temp; 00631 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( ip_p ); 00632 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.ip_addr ); 00633 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( mask_p ); 00634 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.netmask ); 00635 addr_temp = C_SNIC_UartMsgUtil::addrToInteger( gateway_p ); 00636 C_SNIC_UartMsgUtil::convertIntToByteAdday( addr_temp, (char *)req.gateway ); 00637 00638 // Preparation of command 00639 command_len = snic_core_p->preparationSendCommand( UART_CMD_ID_SNIC, req.cmd_sid, (unsigned char *)&req 00640 , sizeof(C_SNIC_Core::tagSNIC_IP_CONFIG_REQ_STATIC_T), payload_buf_p->buf, command_array_p ); 00641 } 00642 // Send uart command request 00643 snic_core_p->sendUart( command_len, command_array_p ); 00644 00645 int ret; 00646 // Wait UART response 00647 ret = uartCmdMgr_p->wait(); 00648 if( ret != 0 ) 00649 { 00650 DEBUG_PRINT( "setIPConfig failed\r\n" ); 00651 snic_core_p->freeCmdBuf( payload_buf_p ); 00652 return -1; 00653 } 00654 00655 if( uartCmdMgr_p->getCommandStatus() != 0 ) 00656 { 00657 DEBUG_PRINT("setIPConfig status:%02x\r\n", uartCmdMgr_p->getCommandStatus()); 00658 snic_core_p->freeCmdBuf( payload_buf_p ); 00659 return -1; 00660 } 00661 00662 snic_core_p->freeCmdBuf( payload_buf_p ); 00663 return ret; 00664 }
Generated on Tue Jul 12 2022 18:43:50 by
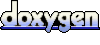