for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_UartMsgUtil.h
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #ifndef _SNIC_WIFI_UART_MSG_UTIL_H_ 00020 #define _SNIC_WIFI_UART_MSG_UTILH_ 00021 #include "MurataObject.h" 00022 #include "mbed.h" 00023 #include "rtos.h" 00024 #include "RawSerial.h" 00025 00026 #define UART_CMD_SOM 0x02 00027 #define UART_CMD_EOM 0x04 00028 #define UART_CMD_ESC 0x10 00029 00030 /* SNIC UART Command ID */ 00031 #define UART_CMD_ID_GEN 0x01 //General command 00032 #define UART_CMD_ID_SNIC 0x70 //SNIC command 00033 #define UART_CMD_ID_WIFI 0x50 //Wi-Fi command 00034 00035 /* SNIC UART Subcommand ID */ 00036 #define UART_CMD_SID_GEN_PWR_UP_IND 0x00 //Power up indication 00037 #define UART_CMD_SID_GEN_FW_VER_GET_REQ 0x08 //Get firmware version string 00038 00039 #define UART_CMD_SID_SNIC_INIT_REQ 0x00 // SNIC API initialization 00040 #define UART_CMD_SID_SNIC_CLEANUP_REQ 0x01 // SNIC API cleanup 00041 #define UART_CMD_SID_SNIC_SEND_FROM_SOCKET_REQ 0x02 // Send from socket 00042 #define UART_CMD_SID_SNIC_CLOSE_SOCKET_REQ 0x03 // Close socket 00043 #define UART_CMD_SID_SNIC_ SOCKET _PARTIAL_CLOSE_ REQ 0x04 // Socket partial close 00044 #define UART_CMD_SID_SNIC_GETSOCKOPT_REQ 0x05 // Get socket option 00045 #define UART_CMD_SID_SNIC_SETSOCKOPT_REQ 0x06 // Set socket option 00046 #define UART_CMD_SID_SNIC_SOCKET_GETNAME_REQ 0x07 // Get name or peer name 00047 #define UART_CMD_SID_SNIC_SEND_ARP_REQ 0x08 // Send ARP request 00048 #define UART_CMD_SID_SNIC_GET_DHCP_INFO_REQ 0x09 // Get DHCP info 00049 #define UART_CMD_SID_SNIC_RESOLVE_NAME_REQ 0x0A // Resolve a host name to IP address 00050 #define UART_CMD_SID_SNIC_IP_CONFIG_REQ 0x0B // Configure DHCP or static IP 00051 #define UART_CMD_SID_SNIC_DATA_IND_ACK_CONFIG_REQ 0x0C // ACK configuration for data indications 00052 #define UART_CMD_SID_SNIC_TCP_CREATE_SOCKET_REQ 0x10 // Create TCP socket 00053 #define UART_CMD_SID_SNIC_TCP_CREATE_CONNECTION_REQ 0x11 // Create TCP connection server 00054 #define UART_CMD_SID_SNIC_TCP_CONNECT_TO_SERVER_REQ 0x12 // Connect to TCP server 00055 #define UART_CMD_SID_SNIC_UDP_CREATE_SOCKET_REQ 0x13 // Create UDP socket 00056 #define UART_CMD_SID_SNIC_UDP_START_RECV_REQ 0x14 // Start UDP receive on socket 00057 #define UART_CMD_SID_SNIC_UDP_SIMPLE_SEND_REQ 0x15 // Send UDP packet 00058 #define UART_CMD_SID_SNIC_UDP_SEND_FROM_SOCKET_REQ 0x16 // Send UDP packet from socket 00059 #define UART_CMD_SID_SNIC_HTTP_REQ 0x17 // Send HTTP request 00060 #define UART_CMD_SID_SNIC_HTTP_MORE_REQ 0x18 // Send HTTP more data request 00061 #define UART_CMD_SID_SNIC_HTTPS_REQ 0x19 // Send HTTPS request 00062 #define UART_CMD_SID_SNIC_TCP_CREATE_ADV_TLS_SOCKET_REQ 0x1A // Create advanced TLS TCP socket 00063 #define UART_CMD_SID_SNIC_TCP_CREAET_SIMPLE_TLS_SOCKET_REQ 0x1B // Create simple TLS TCP socket 00064 #define UART_CMD_SID_SNIC_TCP_CONNECTION_STATUS_IND 0x20 // Connection status indication 00065 #define UART_CMD_SID_SNIC_TCP_CLIENT_SOCKET_IND 0x21 // TCP client socket indication 00066 #define UART_CMD_SID_SNIC_CONNECTION_RECV_IND 0x22 // TCP or connected UDP packet received indication 00067 #define UART_CMD_SID_SNIC_UDP_RECV_IND 0x23 // UCP packet received indication 00068 #define UART_CMD_SID_SNIC_ARP_REPLY_IND 0x24 // ARP reply indication 00069 #define UART_CMD_SID_SNIC_HTTP_RSP_IND 0x25 // HTTP response indication 00070 00071 #define UART_CMD_SID_WIFI_ON_REQ 0x00 // Turn on Wifi 00072 #define UART_CMD_SID_WIFI_OFF_REQ 0x01 // Turn off Wifi 00073 #define UART_CMD_SID_WIFI_JOIN_REQ 0x02 // Associate to a network 00074 #define UART_CMD_SID_WIFI_DISCONNECT_REQ 0x03 // Disconnect from a network 00075 #define UART_CMD_SID_WIFI_GET_STATUS_REQ 0x04 // Get WiFi status 00076 #define UART_CMD_SID_WIFI_SCAN_REQ 0x05 // Scan WiFi networks 00077 #define UART_CMD_SID_WIFI_GET_STA_RSSI_REQ 0x06 // Get STA signal strength (RSSI) 00078 #define UART_CMD_SID_WIFI_AP_CTRL_REQ 0x07 // Soft AP on-off control 00079 #define UART_CMD_SID_WIFI_WPS_REQ 0x08 // Start WPS process 00080 #define UART_CMD_SID_WIFI_AP_GET_CLIENT_REQ 0x0A // Get clients that are associated to the soft AP. 00081 #define UART_CMD_SID_WIFI_NETWORK_STATUS_IND 0x10 // Network status indication 00082 #define UART_CMD_SID_WIFI_SCAN_RESULT_IND 0x11 // Scan result indication 00083 00084 /* SNIC UART Command response status code */ 00085 #define UART_CMD_RES_SNIC_SUCCESS 0x00 00086 #define UART_CMD_RES_SNIC_FAIL 0x01 00087 #define UART_CMD_RES_SNIC_INIT_FAIL 0x02 00088 #define UART_CMD_RES_SNIC_CLEANUP_FAIL 0x03 00089 #define UART_CMD_RES_SNIC_GETADDRINFO_FAIL 0x04 00090 #define UART_CMD_RES_SNIC_CREATE_SOCKET_FAIL 0x05 00091 #define UART_CMD_RES_SNIC_BIND_SOCKET_FAIL 0x06 00092 #define UART_CMD_RES_SNIC_LISTEN_SOCKET_FAIL 0x07 00093 #define UART_CMD_RES_SNIC_ACCEPT_SOCKET_FAIL 0x08 00094 #define UART_CMD_RES_SNIC_PARTIAL_CLOSE_FAIL 0x09 00095 #define UART_CMD_RES_SNIC_SOCKET_PARTIALLY_CLOSED 0x0A 00096 #define UART_CMD_RES_SNIC_SOCKET_CLOSED 0x0B 00097 #define UART_CMD_RES_SNIC_CLOSE_SOCKET_FAIL 0x0C 00098 #define UART_CMD_RES_SNIC_PACKET_TOO_LARGE 0x0D 00099 #define UART_CMD_RES_SNIC_SEND_FAIL 0x0E 00100 #define UART_CMD_RES_SNIC_CONNECT_TO_SERVER_FAIL 0x0F 00101 #define UART_CMD_RES_SNIC_NOT_ENOUGH_MEMORY 0x10 00102 #define UART_CMD_RES_SNIC_TIMEOUT 0x11 00103 #define UART_CMD_RES_SNIC_CONNECTION_UP 0x12 00104 #define UART_CMD_RES_SNIC_GETSOCKOPT_FAIL 0x13 00105 #define UART_CMD_RES_SNIC_SETSOCKOPT_FAIL 0x14 00106 #define UART_CMD_RES_SNIC_INVALID_ARGUMENT 0x15 00107 #define UART_CMD_RES_SNIC_SEND_ARP_FAIL 0x16 00108 #define UART_CMD_RES_SNIC_INVALID_SOCKET 0x17 00109 #define UART_CMD_RES_SNIC_COMMAND_PENDING 0x18 00110 #define UART_CMD_RES_SNIC_SOCKET_NOT_BOUND 0x19 00111 #define UART_CMD_RES_SNIC_SOCKET_NOT_CONNECTED 0x1A 00112 #define UART_CMD_RES_SNIC_NO_NETWORK 0x20 00113 #define UART_CMD_RES_SNIC_INIT_NOT_DONE 0x21 00114 #define UART_CMD_RES_SNIC_NET_IF_FAIL 0x22 00115 #define UART_CMD_RES_SNIC_NET_IF_NOT_UP 0x23 00116 #define UART_CMD_RES_SNIC_DHCP_START_FAIL 0x24 00117 00118 #define UART_CMD_RES_WIFI_SUCCESS 0x00 00119 #define UART_CMD_RES_WIFI_ERR_UNKNOWN_COUNTRY 0x01 00120 #define UART_CMD_RES_WIFI_ERR_INIT_FAIL 0x02 00121 #define UART_CMD_RES_WIFI_ERR_ALREADY_JOINED 0x03 00122 #define UART_CMD_RES_WIFI_ERR_AUTH_TYPE 0x04 00123 #define UART_CMD_RES_WIFI_ERR_JOIN_FAIL 0x05 00124 #define UART_CMD_RES_WIFI_ERR_NOT_JOINED 0x06 00125 #define UART_CMD_RES_WIFI_ERR_LEAVE_FAILED 0x07 00126 #define UART_CMD_RES_WIFI_COMMAND_PENDING 0x08 00127 #define UART_CMD_RES_WIFI_WPS_NO_CONFIG 0x09 00128 #define UART_CMD_RES_WIFI_NETWORK_UP 0x10 00129 #define UART_CMD_RES_WIFI_NETWORK_DOWN 0x11 00130 #define UART_CMD_RES_WIFI_FAIL 0xFF 00131 00132 /** UART Command sequence number 00133 */ 00134 static unsigned char mUartRequestSeq; 00135 00136 /** Internal utility class used by any other classes. 00137 */ 00138 class C_SNIC_UartMsgUtil: public C_MurataObject 00139 { 00140 friend class C_SNIC_Core; 00141 friend class C_SNIC_WifiInterface; 00142 friend class UDPSocket; 00143 friend class TCPSocketConnection; 00144 friend class Socket; 00145 00146 private: 00147 C_SNIC_UartMsgUtil(); 00148 virtual ~C_SNIC_UartMsgUtil(); 00149 00150 /** Make SNIC UART command. 00151 @param cmd_id Command ID 00152 @param payload_p Payload pointer 00153 @param uart_command_p UART Command pointer [output] 00154 @return UART Command length 00155 */ 00156 static unsigned int makeRequest( unsigned char cmd_id, unsigned char *payload_p, unsigned short payload_len, unsigned char *uart_command_p ); 00157 00158 00159 /** Get uart command from receive data. 00160 @param recvdata_len Receive data length 00161 @param recvdata_p Pointer of received data from UART 00162 @param command_id_p Pointer of command ID[output] 00163 @param payload_p Pointer of payload[output] 00164 @return Payload length 00165 */ 00166 static unsigned int getResponsePayload( unsigned int recvdata_len, unsigned char *recvdata_p 00167 , unsigned char *command_id_p, unsigned char *payload_p ); 00168 00169 /** Convert a string to number of ip address. 00170 @param recvdata_p Pointer of string of IP address 00171 @return Number of ip address 00172 */ 00173 static int addrToInteger( const char *addr_p ); 00174 00175 /** Convert a integer to byte array of ip address. 00176 @param recvdata_p Pointer of string of IP address 00177 @return Number of ip address 00178 */ 00179 static void convertIntToByteAdday( int addr, char *addr_array_p ); 00180 00181 protected: 00182 00183 }; 00184 00185 #endif /* _YD_WIFI_UART_MSG_H_ */
Generated on Tue Jul 12 2022 18:43:50 by
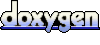