for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_UartMsgUtil.cpp
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #include "SNIC_UartMsgUtil.h" 00020 00021 C_SNIC_UartMsgUtil::C_SNIC_UartMsgUtil() 00022 { 00023 } 00024 00025 C_SNIC_UartMsgUtil::~C_SNIC_UartMsgUtil() 00026 { 00027 } 00028 00029 unsigned int C_SNIC_UartMsgUtil::makeRequest( unsigned char cmd_id,unsigned char *payload_p 00030 , unsigned short payload_len, unsigned char *uart_command_p ) 00031 { 00032 unsigned char check_sum = 0; // Check Sum 00033 unsigned int uart_cmd_len = 0; 00034 int i; 00035 00036 // set SOM 00037 *uart_command_p = UART_CMD_SOM; 00038 uart_command_p++; 00039 uart_cmd_len++; 00040 00041 // set payload length L0 00042 *uart_command_p = (0x80 | (payload_len & 0x007f)); 00043 check_sum += *uart_command_p; 00044 uart_command_p++; 00045 uart_cmd_len++; 00046 00047 // set payload length L1 00048 *uart_command_p = (0x80 | ( (payload_len >> 7) & 0x003f)); 00049 check_sum += *uart_command_p; 00050 uart_command_p++; 00051 uart_cmd_len++; 00052 00053 // set Command ID 00054 *uart_command_p = (0x80 | cmd_id); 00055 check_sum += *uart_command_p; 00056 uart_command_p++; 00057 uart_cmd_len++; 00058 00059 // set Payload 00060 for( i = 0; i < payload_len; i++, uart_command_p++, uart_cmd_len++ ) 00061 { 00062 *uart_command_p = payload_p[i]; 00063 } 00064 00065 // set Check sum 00066 *uart_command_p = (0x80 | check_sum); 00067 uart_command_p++; 00068 uart_cmd_len++; 00069 00070 // set EOM 00071 *uart_command_p = UART_CMD_EOM; 00072 uart_cmd_len++; 00073 00074 return uart_cmd_len; 00075 } 00076 00077 unsigned int C_SNIC_UartMsgUtil::getResponsePayload( unsigned int recvdata_len, unsigned char *recvdata_p 00078 , unsigned char *command_id_p, unsigned char *payload_p ) 00079 { 00080 unsigned short payload_len = 0; 00081 unsigned char *buf_p = NULL; 00082 int i; 00083 00084 // get payload length 00085 payload_len = ( ( (recvdata_p[1] & ~0x80) & 0xff) | ( ( (recvdata_p[2] & ~0xC0) << 7) & 0xff80) ); 00086 00087 // get Command ID 00088 *command_id_p = (recvdata_p[3] & ~0x80); 00089 00090 buf_p = &recvdata_p[4]; 00091 00092 // get payload data 00093 for( i = 0; i < payload_len; i++, buf_p++ ) 00094 { 00095 *payload_p = *buf_p; 00096 payload_p++; 00097 } 00098 00099 return payload_len; 00100 } 00101 00102 int C_SNIC_UartMsgUtil::addrToInteger( const char *addr_p ) 00103 { 00104 if( addr_p == NULL ) 00105 { 00106 DEBUG_PRINT("addrToInteger parameter error\r\n"); 00107 return 0; 00108 } 00109 00110 unsigned char ipadr[4]; 00111 unsigned char temp= 0; 00112 int i,j,k; 00113 00114 /* convert to char[4] */ 00115 k=0; 00116 for(i=0; i<4; i ++) 00117 { 00118 for(j=0; j<4; j ++) 00119 { 00120 if((addr_p[k] > 0x2F)&&(addr_p[k] < 0x3A)) 00121 { 00122 temp = (temp * 10) + addr_p[k]-0x30; 00123 } 00124 else if((addr_p[k] == 0x20)&&(temp == 0)) 00125 { 00126 } 00127 else 00128 { 00129 ipadr[i]=temp; 00130 temp = 0; 00131 k++; 00132 break; 00133 } 00134 k++; 00135 } 00136 } 00137 00138 int addr = ( (ipadr[0]<<24) | (ipadr[1]<<16) | (ipadr[2]<<8) | ipadr[3] ); 00139 00140 return addr; 00141 } 00142 00143 void C_SNIC_UartMsgUtil::convertIntToByteAdday( int addr, char *addr_array_p ) 00144 { 00145 addr_array_p[0] = ((addr & 0xFF000000) >> 24 ); 00146 addr_array_p[1] = ((addr & 0xFF0000) >> 16 ); 00147 addr_array_p[2] = ((addr & 0xFF00) >> 8 ); 00148 addr_array_p[3] = ( addr & 0xFF); 00149 }
Generated on Tue Jul 12 2022 18:43:50 by
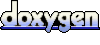