for EthernetInterface library compatibility.\\ ** Unoffical fix. may be a problem. **
Dependents: SNIC-httpclient-example SNIC-ntpclient-example
Fork of SNICInterface by
SNIC_Core.h
00001 /* Copyright (C) 2014 Murata Manufacturing Co.,Ltd., MIT License 00002 * muRata, SWITCH SCIENCE Wi-FI module TypeYD SNIC-UART. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #ifndef _SNIC_CORE_H_ 00020 #define _SNIC_CORE_H_ 00021 00022 #include "MurataObject.h" 00023 #include "mbed.h" 00024 #include "rtos.h" 00025 #include "RawSerial.h" 00026 #include "CBuffer.h" 00027 00028 #include "SNIC_UartCommandManager.h" 00029 00030 #define UART_REQUEST_PAYLOAD_MAX 2048 00031 00032 #define MEMPOOL_BLOCK_SIZE 2048 00033 #define MEMPOOL_PAYLOAD_NUM 1 00034 #define MAX_SOCKET_ID 5 00035 00036 #define MEMPOOL_UART_RECV_NUM 6 00037 #define SNIC_UART_RECVBUF_SIZE 2048 00038 00039 /** Wi-Fi security 00040 */ 00041 typedef enum SECURITY { 00042 /** Securiry Open */ 00043 e_SEC_OPEN = 0x00, 00044 /** Securiry WEP */ 00045 e_SEC_WEP = 0x01, 00046 /** Securiry WPA-PSK(TKIP) */ 00047 e_SEC_WPA_TKIP = 0x02, 00048 /** Securiry WPA2-PSK(AES) */ 00049 e_SEC_WPA2_AES = 0x04, 00050 /** Securiry WPA2-PSK(TKIP/AES) */ 00051 e_SEC_WPA2_MIXED = 0x06, 00052 /** Securiry WPA-PSK(AES) */ 00053 e_SEC_WPA_AES = 0x07 00054 }E_SECURITY; 00055 00056 /** Wi-Fi status 00057 */ 00058 typedef enum WIFI_STATUS { 00059 /** Wi-Fi OFF */ 00060 e_STATUS_OFF = 0, 00061 /** No network */ 00062 e_NO_NETWORK, 00063 /** Connected to AP (STA mode) */ 00064 e_STA_JOINED, 00065 /** Started on AP mode */ 00066 e_AP_STARTED 00067 }E_WIFI_STATUS; 00068 00069 /** Memorypool 00070 */ 00071 typedef struct 00072 { 00073 unsigned int size; 00074 unsigned int demand_size; 00075 unsigned char buf[MEMPOOL_BLOCK_SIZE]; 00076 }tagMEMPOOL_BLOCK_T; 00077 00078 /** Internal class used by any other classes. This class is singleton. 00079 */ 00080 class C_SNIC_Core: public C_MurataObject 00081 { 00082 friend class C_SNIC_UartCommandManager; 00083 friend class C_SNIC_WifiInterface; 00084 friend class TCPSocketConnection; 00085 friend class TCPSocketServer; 00086 friend class UDPSocket; 00087 friend class Socket; 00088 00089 private: 00090 /** Wi-Fi Network type 00091 */ 00092 typedef enum NETWORK_TYPE { 00093 /** Infrastructure */ 00094 e_INFRA = 0, 00095 /** Adhoc */ 00096 e_ADHOC = 1 00097 }E_NETWORK_TYPE; 00098 00099 /** Connection information 00100 */ 00101 typedef struct { 00102 CircBuffer<char> *recvbuf_p; 00103 bool is_connected; 00104 bool is_received; 00105 bool is_receive_complete; 00106 int parent_socket; 00107 int from_ip; 00108 short from_port; 00109 bool is_accept; 00110 Mutex mutex; 00111 }tagCONNECT_INFO_T; 00112 00113 /** UDP Recv information 00114 */ 00115 typedef struct { 00116 CircBuffer<char> *recvbuf_p; 00117 int from_ip; 00118 short from_port; 00119 int parent_socket; 00120 bool is_received; 00121 Mutex mutex; 00122 }tagUDP_RECVINFO_T; 00123 00124 /** GEN_FW_VER_GET_REQ Command */ 00125 typedef struct 00126 { 00127 unsigned char cmd_sid; 00128 unsigned char seq; 00129 }tagGEN_FW_VER_GET_REQ_T; 00130 00131 /** SNIC_INIT_REQ */ 00132 typedef struct 00133 { 00134 unsigned char cmd_sid; 00135 unsigned char seq; 00136 unsigned char buf_size[2]; 00137 }tagSNIC_INIT_REQ_T; 00138 00139 /** SNIC_IP_CONFIG_REQ */ 00140 typedef struct 00141 { 00142 unsigned char cmd_sid; 00143 unsigned char seq; 00144 unsigned char interface; 00145 unsigned char dhcp; 00146 }tagSNIC_IP_CONFIG_REQ_DHCP_T; 00147 00148 /** SNIC_IP_CONFIG_REQ */ 00149 typedef struct 00150 { 00151 unsigned char cmd_sid; 00152 unsigned char seq; 00153 unsigned char interface; 00154 unsigned char dhcp; 00155 unsigned char ip_addr[4]; 00156 unsigned char netmask[4]; 00157 unsigned char gateway[4]; 00158 }tagSNIC_IP_CONFIG_REQ_STATIC_T; 00159 00160 /** SNIC_TCP_CREATE_SOCKET_REQ */ 00161 typedef struct 00162 { 00163 unsigned char cmd_sid; 00164 unsigned char seq; 00165 unsigned char bind; 00166 unsigned char local_addr[4]; 00167 unsigned char local_port[2]; 00168 }tagSNIC_TCP_CREATE_SOCKET_REQ_T; 00169 00170 /** SNIC_CLOSE_SOCKET_REQ */ 00171 typedef struct 00172 { 00173 unsigned char cmd_sid; 00174 unsigned char seq; 00175 unsigned char socket_id; 00176 }tagSNIC_CLOSE_SOCKET_REQ_T; 00177 00178 /** SNIC_TCP_SEND_FROM_SOCKET_REQ */ 00179 typedef struct 00180 { 00181 unsigned char cmd_sid; 00182 unsigned char seq; 00183 unsigned char socket_id; 00184 unsigned char option; 00185 unsigned char payload_len[2]; 00186 }tagSNIC_TCP_SEND_FROM_SOCKET_REQ_T; 00187 00188 /** SNIC_TCP_CREATE_CONNECTION_REQ */ 00189 typedef struct 00190 { 00191 unsigned char cmd_sid; 00192 unsigned char seq; 00193 unsigned char socket_id; 00194 unsigned char recv_bufsize[2]; 00195 unsigned char max_client; 00196 }tagSNIC_TCP_CREATE_CONNECTION_REQ_T; 00197 00198 /** SNIC_TCP_CONNECT_TO_SERVER_REQ */ 00199 typedef struct 00200 { 00201 unsigned char cmd_sid; 00202 unsigned char seq; 00203 unsigned char socket_id; 00204 unsigned char remote_addr[4]; 00205 unsigned char remote_port[2]; 00206 unsigned char recv_bufsize[2]; 00207 unsigned char timeout; 00208 }tagSNIC_TCP_CONNECT_TO_SERVER_REQ_T; 00209 00210 /** SNIC_UDP_SIMPLE_SEND_REQ */ 00211 typedef struct 00212 { 00213 unsigned char cmd_sid; 00214 unsigned char seq; 00215 unsigned char remote_ip[4]; 00216 unsigned char remote_port[2]; 00217 unsigned char payload_len[2]; 00218 }tagSNIC_UDP_SIMPLE_SEND_REQ_T; 00219 00220 /** SNIC_UDP_CREATE_SOCKET_REQ */ 00221 typedef struct 00222 { 00223 unsigned char cmd_sid; 00224 unsigned char seq; 00225 unsigned char bind; 00226 unsigned char local_addr[4]; 00227 unsigned char local_port[2]; 00228 }tagSNIC_UDP_CREATE_SOCKET_REQ_T; 00229 00230 /** SNIC_UDP_CREATE_SOCKET_REQ */ 00231 typedef struct 00232 { 00233 unsigned char cmd_sid; 00234 unsigned char seq; 00235 unsigned char bind; 00236 }tagSNIC_UDP_CREATE_SOCKET_REQ_CLIENT_T; 00237 00238 /** SNIC_UDP_SEND_FROM_SOCKET_REQ */ 00239 typedef struct 00240 { 00241 unsigned char cmd_sid; 00242 unsigned char seq; 00243 unsigned char remote_ip[4]; 00244 unsigned char remote_port[2]; 00245 unsigned char socket_id; 00246 unsigned char connection_mode; 00247 unsigned char payload_len[2]; 00248 }tagSNIC_UDP_SEND_FROM_SOCKET_REQ_T; 00249 00250 /** SNIC_UDP_START_RECV_REQ */ 00251 typedef struct 00252 { 00253 unsigned char cmd_sid; 00254 unsigned char seq; 00255 unsigned char socket_id; 00256 unsigned char recv_bufsize[2]; 00257 }tagSNIC_UDP_START_RECV_REQ_T; 00258 00259 /** SNIC_GET_DHCP_INFO_REQ */ 00260 typedef struct 00261 { 00262 unsigned char cmd_sid; 00263 unsigned char seq; 00264 unsigned char interface; 00265 }tagSNIC_GET_DHCP_INFO_REQ_T; 00266 00267 /** WIFI_ON_REQ Command */ 00268 typedef struct 00269 { 00270 unsigned char cmd_sid; 00271 unsigned char seq; 00272 char country[COUNTRYC_CODE_LENTH]; 00273 }tagWIFI_ON_REQ_T; 00274 00275 /** WIFI_OFF_REQ Command */ 00276 typedef struct 00277 { 00278 unsigned char cmd_sid; 00279 unsigned char seq; 00280 }tagWIFI_OFF_REQ_T; 00281 00282 /** WIFI_DISCONNECT_REQ Command */ 00283 typedef struct 00284 { 00285 unsigned char cmd_sid; 00286 unsigned char seq; 00287 }tagWIFI_DISCONNECT_REQ_T; 00288 00289 /** WIFI_GET_STA_RSSI_REQ Command */ 00290 typedef struct 00291 { 00292 unsigned char cmd_sid; 00293 unsigned char seq; 00294 }tagWIFI_GET_STA_RSSI_REQ_T; 00295 00296 /** WIFI_SCAN_REQ Command */ 00297 typedef struct 00298 { 00299 unsigned char cmd_sid; 00300 unsigned char seq; 00301 unsigned char scan_type; 00302 unsigned char bss_type; 00303 unsigned char bssid[BSSID_MAC_LENTH]; 00304 unsigned char chan_list; 00305 unsigned char ssid[SSID_MAX_LENGTH+1]; 00306 }tagWIFI_SCAN_REQ_T; 00307 00308 /** WIFI_GET_STATUS_REQ Command */ 00309 typedef struct 00310 { 00311 unsigned char cmd_sid; 00312 unsigned char seq; 00313 unsigned char interface; 00314 }tagWIFI_GET_STATUS_REQ_T; 00315 00316 /** Get buffer for command from memory pool. 00317 @return Pointer of buffer 00318 */ 00319 tagMEMPOOL_BLOCK_T *allocCmdBuf(); 00320 00321 /** Release buffer to memory pool. 00322 @param buf_p Pointer of buffer 00323 */ 00324 void freeCmdBuf( tagMEMPOOL_BLOCK_T *buf_p ); 00325 00326 /** Get buffer for command from memory pool. 00327 @return Pointer of buffer 00328 */ 00329 tagMEMPOOL_BLOCK_T *allocUartRcvBuf(); 00330 00331 /** Release buffer to memory pool. 00332 @param buf_p Pointer of buffer 00333 */ 00334 void freeUartRecvBuf( tagMEMPOOL_BLOCK_T *buf_p ); 00335 00336 /** Module Reset 00337 */ 00338 int resetModule( PinName reset ); 00339 00340 /** Initialize UART 00341 */ 00342 int initUart( PinName tx, PinName rx, int baud ); 00343 00344 /** Send data to UART 00345 @param len Length of send data 00346 @param data Pointer of send data 00347 @return 0:success/other:fail 00348 */ 00349 int sendUart( unsigned int len, unsigned char *data ); 00350 00351 /** Preparation of the UART command 00352 @param cmd_id UART Command ID 00353 @param cmd_sid UART Command SubID 00354 @param req_buf_p Pointer of UART request buffer 00355 @param req_buf_len Length of UART request buffer 00356 @param response_buf_p Pointer of UART response buffer 00357 @param command_p Pointer of UART command[output] 00358 @return Length of UART command. 00359 */ 00360 unsigned int preparationSendCommand( unsigned char cmd_id, unsigned char cmd_sid 00361 , unsigned char *req_buf_p, unsigned int req_buf_len 00362 , unsigned char *response_buf_p, unsigned char *command_p ); 00363 00364 /** 00365 Get pointer of connection information. 00366 @param socket_id Socket ID 00367 @return The pointer of connection information 00368 */ 00369 C_SNIC_Core::tagCONNECT_INFO_T *getConnectInfo( int socket_id ); 00370 00371 /** 00372 Get pointer of UDP Recv information. 00373 @param socket_id Socket ID 00374 @return The pointer of UDP Recv information 00375 */ 00376 C_SNIC_Core::tagUDP_RECVINFO_T *getUdpRecvInfo( int socket_id ); 00377 00378 /** 00379 Get pointer of the instance of C_SNIC_UartCommandManager. 00380 @return The pointer of the instance of C_SNIC_UartCommandManager. 00381 */ 00382 C_SNIC_UartCommandManager *getUartCommand(); 00383 00384 unsigned char *getCommandBuf(); 00385 00386 /** Get an instance of the C_SNIC_Core class. 00387 @return Instance of the C_SNIC_Core class 00388 @note Please do not create an instance in the default constructor this class. 00389 Please use this method when you want to get an instance. 00390 */ 00391 static C_SNIC_Core *getInstance(); 00392 00393 /** Mutex lock of API calls 00394 */ 00395 void lockAPI( void ); 00396 00397 /** Mutex unlock of API calls 00398 */ 00399 void unlockAPI( void ); 00400 00401 private: 00402 static C_SNIC_Core *mInstance_p; 00403 Thread *mUartRecvThread_p; 00404 Thread *mUartRecvDispatchThread_p; 00405 RawSerial *mUart_p; 00406 Mutex mUartMutex; 00407 Mutex mAPIMutex; 00408 00409 // DigitalInOut mModuleReset; 00410 C_SNIC_UartCommandManager *mUartCommand_p; 00411 00412 CircBuffer<char> *mUartRecvBuf_p; // UART RecvBuffer 00413 00414 /** Socket buffer */ 00415 tagCONNECT_INFO_T mConnectInfo[MAX_SOCKET_ID+1]; 00416 00417 /** UDP Information */ 00418 tagUDP_RECVINFO_T mUdpRecvInfo[MAX_SOCKET_ID+1]; 00419 00420 /** Constructor 00421 */ 00422 C_SNIC_Core(); 00423 00424 virtual ~C_SNIC_Core(); 00425 00426 static void uartRecvCallback( void ); 00427 /** Receiving thread of UART 00428 */ 00429 static void uartRecvDispatchThread( void const *args_p ); 00430 }; 00431 00432 #endif
Generated on Tue Jul 12 2022 18:43:49 by
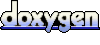