RTOS Support.
Dependents: SerialRPC_rtos_example
Fork of RPCInterface by
SerialRPCInterface.cpp
00001 /** 00002 * @section LICENSE 00003 *Copyright (c) 2010 ARM Ltd. 00004 * 00005 *Permission is hereby granted, free of charge, to any person obtaining a copy 00006 *of this software and associated documentation files (the "Software"), to deal 00007 *in the Software without restriction, including without limitation the rights 00008 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 *copies of the Software, and to permit persons to whom the Software is 00010 *furnished to do so, subject to the following conditions: 00011 * 00012 *The above copyright notice and this permission notice shall be included in 00013 *all copies or substantial portions of the Software. 00014 * 00015 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 *THE SOFTWARE. 00022 * 00023 * 00024 * @section DESCRIPTION 00025 * 00026 *This class sets up RPC communication. This allows objects on mbed to be controlled. Objects can be created or existing objects can be used 00027 */ 00028 #include "SerialRPCInterface.h" 00029 00030 using namespace mbed; 00031 00032 //Requires multiple contstructors for each type, serial to set different pin numbers, TCP for port. 00033 SerialRPCInterface::SerialRPCInterface(PinName tx, PinName rx, int baud):pc(tx, rx), _thread(SerialRPCInterface::_MsgProcessStarter, this, osPriorityNormal, 1024) { 00034 _RegClasses(); 00035 _enabled = true; 00036 _command_ptr = _command; 00037 pc.attach(this, &SerialRPCInterface::_RPCSerial, Serial::RxIrq); 00038 if(baud != 9600)pc.baud(baud); 00039 } 00040 00041 void SerialRPCInterface::_RegClasses(void){ 00042 //Register classes with base 00043 //RPC::add_rpc_class<RpcAnalogIn>(); 00044 RPC::add_rpc_class<RpcDigitalIn>(); 00045 RPC::add_rpc_class<RpcDigitalOut>(); 00046 RPC::add_rpc_class<RpcDigitalInOut>(); 00047 RPC::add_rpc_class<RpcPwmOut>(); 00048 RPC::add_rpc_class<RpcTimer>(); 00049 //RPC::add_rpc_class<RpcBusOut>(); 00050 //RPC::add_rpc_class<RpcBusIn>(); 00051 //RPC::add_rpc_class<RpcBusInOut>(); 00052 RPC::add_rpc_class<RpcSerial>(); 00053 RPC::add_rpc_class<RpcSPI>(); 00054 //RPC::add_rpc_class<RpcI2C>(); 00055 00056 //AnalogOut not avaliable on mbed LPC11U24 so only compile for other devices 00057 #if !defined(TARGET_LPC11U24) 00058 //RPC::add_rpc_class<RpcAnalogOut>(); 00059 #endif 00060 } 00061 00062 void SerialRPCInterface::Disable(void){ 00063 _enabled = false; 00064 } 00065 void SerialRPCInterface::Enable(void){ 00066 _enabled = true; 00067 } 00068 void SerialRPCInterface::_MsgProcessStarter(const void *args){ 00069 SerialRPCInterface *instance = (SerialRPCInterface*)args; 00070 while(1){ 00071 instance->_MsgProcess(); 00072 } 00073 } 00074 void SerialRPCInterface::_MsgProcess(void) { 00075 osEvent evt = _commandMail.get(); 00076 if(evt.status == osEventMail){ 00077 mail_t *mail = (mail_t*)evt.value.p; 00078 if(_enabled == true){ 00079 RPC::call(_command, _response); 00080 pc.printf("%s\n", _response); 00081 } 00082 _commandMail.free(mail); 00083 } 00084 } 00085 00086 void SerialRPCInterface::_RPCSerial() { 00087 _RPCflag = true; 00088 if(_enabled == true){ 00089 char c = pc.getc(); 00090 if(c == 13){ 00091 *_command_ptr = '\0'; 00092 mail_t *mail = _commandMail.alloc(); 00093 strcpy(mail->command, _command); 00094 _commandMail.put(mail); 00095 _command_ptr = _command; 00096 }else if(_command_ptr - _command < 256){ 00097 *_command_ptr = c; 00098 _command_ptr++; 00099 } 00100 } 00101 _RPCflag = false; 00102 }
Generated on Fri Jul 22 2022 03:41:42 by
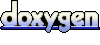