Fixed compatibility for HTTPClient Library. (HTTPClient by Donatien Garnier)
Dependents: FlashAir_Twitter CyaSSL-Twitter-OAuth4Tw TweetTest NetworkThermometer ... more
Fork of OAuth4Tw by
OAuth4Tw.cpp
00001 #include "OAuth4Tw.h" 00002 #include "mbed.h" 00003 #include "twicpps/oauth.h" 00004 00005 #include <HTTPClient.h> 00006 #include "HTTPPostText.h" 00007 00008 OAuth4Tw::OAuth4Tw(const char *c_key, const char *c_secret, 00009 const char *t_key, const char *t_secret) 00010 :consumer_key(c_key), 00011 consumer_secret(c_secret), 00012 token_key(t_key), 00013 token_secret(t_secret) { } 00014 00015 std::string OAuth4Tw::url_escape(const char *str) 00016 { 00017 return oauth_url_escape(str); 00018 } 00019 00020 HTTPResult OAuth4Tw::get(const char *url, IHTTPDataIn *response, int timeout /*= HTTP_CLIENT_DEFAULT_TIMEOUT*/) 00021 { 00022 std::string req_url; 00023 00024 req_url = oauth_sign_url2(url, NULL, OA_HMAC, 0, 00025 consumer_key, consumer_secret, 00026 token_key, token_secret); 00027 00028 HTTPClient http; 00029 HTTPResult r = http.get(req_url.c_str(), response); 00030 00031 return r; 00032 } 00033 00034 HTTPResult OAuth4Tw::post(const char *url, IHTTPDataIn *response, int timeout /*= HTTP_CLIENT_DEFAULT_TIMEOUT*/) 00035 { 00036 std::string req_url; 00037 std::string postargs; 00038 00039 req_url = oauth_sign_url2(url, &postargs, OA_HMAC, 0, 00040 consumer_key, consumer_secret, 00041 token_key, token_secret); 00042 00043 const char *poststr = postargs.c_str(); 00044 00045 HTTPClient http; 00046 HTTPPostText request((char *)poststr, strlen(poststr) + 1); 00047 HTTPResult r = http.post(req_url.c_str(), request, response); 00048 00049 return r; 00050 } 00051 00052 HTTPResult OAuth4Tw::post(const char *url, std::vector<std::string> *postdata, IHTTPDataIn *response, int timeout /*= HTTP_CLIENT_DEFAULT_TIMEOUT*/) 00053 { 00054 std::string req_url; 00055 std::string postargs; 00056 00057 { 00058 std::vector<std::string> argv; 00059 argv.reserve(postdata->size()+8); 00060 00061 oauth_split_post_paramters(url, &argv, 0); 00062 for (int i=0; i<postdata->size(); i++) { 00063 argv.push_back(postdata->at(i)); 00064 } 00065 00066 req_url = oauth_sign_array2(&argv, &postargs, OA_HMAC, 0, 00067 consumer_key, consumer_secret, 00068 token_key, token_secret); 00069 00070 #if 0 00071 for (int i=0; i<argv.size(); i++) { 00072 printf("param[%d]: %s\n", i, argv.at(i).c_str()); 00073 } 00074 #endif 00075 } 00076 00077 const char *poststr = postargs.c_str(); 00078 00079 HTTPClient http; 00080 HTTPPostText request((char *)poststr, strlen(poststr) + 1); 00081 HTTPResult r = http.post(req_url.c_str(), request, response); 00082 00083 return r; 00084 }
Generated on Thu Jul 14 2022 04:27:06 by
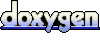