
WIZnet WIZ820io (W5200) support
Dependencies: HTTPClient WIZ820ioInterface mbed
Fork of HTTPClient_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 //#include "EthernetInterface.h" 00003 #include "WIZ820ioInterface.h" 00004 #include "HTTPClient.h" 00005 00006 //EthernetInterface eth; 00007 #if defined(TARGET_LPC1114) 00008 SPI spi(dp2, dp1, dp6); // mosi, miso, sclk 00009 WIZ820ioInterface eth(&spi, dp25, dp26); // spi, cs, reset 00010 00011 #elif defined(TARGET_LPC1768) 00012 SPI spi(p11, p12, p13); // mosi, miso, sclk 00013 WIZ820ioInterface eth(&spi, p14, p15); // spi, cs, reset 00014 00015 #endif 00016 00017 HTTPClient http; 00018 char str[512]; 00019 00020 int main() 00021 { 00022 int ret = eth.init(); //Use DHCP 00023 if (!ret) 00024 { 00025 printf("Initialized, MAC: %s\n", eth.getMACAddress()); 00026 } 00027 else 00028 { 00029 printf("Error eth.init() - ret = %d\n", ret); 00030 return -1; 00031 } 00032 00033 ret = eth.connect(); 00034 if (!ret) 00035 { 00036 printf("Connected, IP: %s, MASK: %s, GW: %s\n", 00037 eth.getIPAddress(), eth.getNetworkMask(), eth.getGateway()); 00038 } 00039 else 00040 { 00041 printf("Error eth.connect() - ret = %d\n", ret); 00042 return -1; 00043 } 00044 00045 00046 //GET data 00047 printf("\nTrying to fetch page...\n"); 00048 ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00049 if (!ret) 00050 { 00051 printf("Page fetched successfully - read %d characters\n", strlen(str)); 00052 printf("Result: %s\n", str); 00053 } 00054 else 00055 { 00056 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00057 } 00058 00059 //POST data 00060 HTTPMap map; 00061 HTTPText inText(str, 512); 00062 map.put("Hello", "World"); 00063 map.put("test", "1234"); 00064 printf("\nTrying to post data...\n"); 00065 ret = http.post("http://httpbin.org/post", map, &inText); 00066 if (!ret) 00067 { 00068 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00069 printf("Result: %s\n", str); 00070 } 00071 else 00072 { 00073 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00074 } 00075 00076 //PUT data 00077 strcpy(str, "This is a PUT test!"); 00078 HTTPText outText(str); 00079 //HTTPText inText(str, 512); 00080 printf("\nTrying to put resource...\n"); 00081 ret = http.put("http://httpbin.org/put", outText, &inText); 00082 if (!ret) 00083 { 00084 printf("Executed PUT successfully - read %d characters\n", strlen(str)); 00085 printf("Result: %s\n", str); 00086 } 00087 else 00088 { 00089 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00090 } 00091 00092 //DELETE data 00093 //HTTPText inText(str, 512); 00094 printf("\nTrying to delete resource...\n"); 00095 ret = http.del("http://httpbin.org/delete", &inText); 00096 if (!ret) 00097 { 00098 printf("Executed DELETE successfully - read %d characters\n", strlen(str)); 00099 printf("Result: %s\n", str); 00100 } 00101 else 00102 { 00103 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00104 } 00105 00106 00107 printf("\n"); 00108 ret = eth.disconnect(); 00109 if (!ret) 00110 { 00111 printf("Disconnected\n"); 00112 } 00113 else 00114 { 00115 printf("Error eth.disconnect() - ret = %d\n", ret); 00116 } 00117 00118 00119 while(1) { 00120 } 00121 }
Generated on Wed Jul 13 2022 05:30:18 by
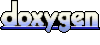