Asynchronous (Non-blocking) Serial Communication library with variable length software ring buffer (FIFO). You can use primary method of the RawSerial Library. Operability confirmed on mbed 2.0. (Rev.146)
Dependents: InvertedPendulum2017 SerialConnect mbed_2018 mbed_2019_rx3 ... more
AsyncSerial Class Reference
Asynchronous Serial Communication with FIFO. More...
#include <AsyncSerial.hpp>
Public Member Functions | |
AsyncSerial (PinName txpin, PinName rxpin, uint32_t baudrate=9600, uint32_t buffer_size=256) | |
Create a new AsyncSerial Port. | |
virtual | ~AsyncSerial (void) |
Disable the AsyncSerial Port. | |
virtual int | readable (void) |
Get how many bytes are in the rx buffer. | |
virtual int | writeable (void) |
Check writeable or not. | |
virtual int | getc (void) |
Get 1byte from the AsyncSerial port. | |
virtual int | peekc (void) |
Peek 1byte from the AsyncSerial port. | |
virtual void | putc (int c) |
Put 1byte to the AsyncSerial port. | |
virtual void | puts (const char *str) |
Write a string with new line. | |
virtual int | printf (const char *format,...) |
Write a formatted string to the AsyncSerial port. | |
virtual int | write (const uint8_t *buffer, int length) |
Write byte array to the AsyncSerial port. | |
virtual void | abort_read (void) |
Abort the on-going read transfer. | |
virtual void | abort_write (void) |
Abort the on-going write transfer. | |
virtual void | wait (void) |
Wait until finish all sending. | |
virtual void | format (int bits=8, RawSerial::Parity parity=RawSerial::None, int stop_bits=1) |
Set bits, parity and stop bits. | |
virtual void | baud (int baudrate) |
Set baud rate. |
Detailed Description
Asynchronous Serial Communication with FIFO.
Definition at line 35 of file AsyncSerial.hpp.
Constructor & Destructor Documentation
AsyncSerial | ( | PinName | txpin, |
PinName | rxpin, | ||
uint32_t | baudrate = 9600 , |
||
uint32_t | buffer_size = 256 |
||
) |
Create a new AsyncSerial Port.
- Parameters:
-
txpin Tx pin name (Defined in PinName.h) rxpin Rx pin name (Defined in PinName.h) baudrate Baudrate (ex: 115200). Default value is 9600. buffer_size Buffer size. Default value is 256. (byte)
Definition at line 22 of file AsyncSerial.cpp.
~AsyncSerial | ( | void | ) | [virtual] |
Disable the AsyncSerial Port.
- Parameters:
-
No parameters.
Definition at line 42 of file AsyncSerial.cpp.
Member Function Documentation
void abort_read | ( | void | ) | [virtual] |
Abort the on-going read transfer.
- Parameters:
-
No parameters.
Definition at line 160 of file AsyncSerial.cpp.
void abort_write | ( | void | ) | [virtual] |
Abort the on-going write transfer.
- Parameters:
-
No parameters.
Definition at line 165 of file AsyncSerial.cpp.
void baud | ( | int | baudrate ) | [virtual] |
void format | ( | int | bits = 8 , |
RawSerial::Parity | parity = RawSerial::None , |
||
int | stop_bits = 1 |
||
) | [virtual] |
Set bits, parity and stop bits.
- Parameters:
-
bits Bits (5 ~ 8) parity Parity stop_bits Stop bits (1 or 2)
Definition at line 175 of file AsyncSerial.cpp.
int getc | ( | void | ) | [virtual] |
Get 1byte from the AsyncSerial port.
- Parameters:
-
No parameters.
- Return values:
-
All Got Data 0 Error.
Definition at line 76 of file AsyncSerial.cpp.
int peekc | ( | void | ) | [virtual] |
Peek 1byte from the AsyncSerial port.
- Parameters:
-
No parameters.
- Return values:
-
ALL Got Data 0 Error.
Definition at line 80 of file AsyncSerial.cpp.
int printf | ( | const char * | format, |
... | |||
) | [virtual] |
Write a formatted string to the AsyncSerial port.
- Parameters:
-
*format A Formatted string for write.
- Return values:
-
0 Error. 1+ Wrote string size (byte).
Definition at line 112 of file AsyncSerial.cpp.
void putc | ( | int | c ) | [virtual] |
Put 1byte to the AsyncSerial port.
- Parameters:
-
data A Data for put
- Returns:
- Nothing.
Definition at line 84 of file AsyncSerial.cpp.
void puts | ( | const char * | str ) | [virtual] |
Write a string with new line.
The string must be NULL terminated.
- Parameters:
-
*str A String for write (Must be NULL terminated).
- Returns:
- Nothing.
Definition at line 94 of file AsyncSerial.cpp.
int readable | ( | void | ) | [virtual] |
Get how many bytes are in the rx buffer.
- Parameters:
-
No parameters.
- Returns:
- Size of readable data. (byte)
Definition at line 68 of file AsyncSerial.cpp.
void wait | ( | void | ) | [virtual] |
Wait until finish all sending.
- Parameters:
-
No parameters.
Definition at line 170 of file AsyncSerial.cpp.
int write | ( | const uint8_t * | buffer, |
int | length | ||
) | [virtual] |
Write byte array to the AsyncSerial port.
- Parameters:
-
*s A pointer to the array for write. length Write size (byte).
- Return values:
-
0 Error. 1 Success.
Definition at line 140 of file AsyncSerial.cpp.
int writeable | ( | void | ) | [virtual] |
Check writeable or not.
- Parameters:
-
No Parameters.
- Return values:
-
1 Always return 1 because this library provides asynchronous serial.
Definition at line 72 of file AsyncSerial.cpp.
Generated on Tue Jul 12 2022 20:12:48 by
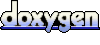