A simple I2C library for ESCs using the Blue Robotics version of SimonK's TGY firmware (https://github.com/bluerobotics/tgy). This library supports the BlueESC, but also the Afro Nfet 30A. I2C is an alternative interface for micro-controllers, rather than using PWM (as a servo) -- this provides some additional features like temperature level, RPM, etc.
Dependents: SimonK_I2C_ESC_Example
SimonK_I2C_ESC.h
00001 //! SimonK I2C ESC Control Library 00002 /*! 00003 Title: Mbed I2C ESC Library 00004 Description: This library provide methods to control I2C capable ESCs 00005 using the Blue Robotics fork of the tgy firmware. It's designed for the 00006 "BlueESC" but will work with an tgy compatible ESC (this code was tested on the Afro nfet 30A). 00007 The code is designed for the Mbed boards. 00008 00009 Inspired by the Arduino I2C library provided by the blue robotics team (https://github.com/bluerobotics/Arduino_I2C_ESC). 00010 ------------------------------- 00011 The MIT License (MIT) 00012 Copyright (c) 2016 Daniel Knox. 00013 Permission is hereby granted, free of charge, to any person obtaining a copy 00014 of this software and associated documentation files (the "Software"), to deal 00015 in the Software without restriction, including without limitation the rights 00016 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00017 copies of the Software, and to permit persons to whom the Software is 00018 furnished to do so, subject to the following conditions: 00019 The above copyright notice and this permission notice shall be included in 00020 all copies or substantial portions of the Software. 00021 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00022 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00023 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00024 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00025 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00026 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00027 THE SOFTWARE. 00028 -------------------------------*/ 00029 00030 #ifndef SimonK_I2C_ESC_H 00031 #define SimonK_I2C_ESC_H 00032 00033 #include "SimonK_I2C_ESC.h" 00034 00035 #define MAX_ESCS 16 00036 00037 // THERMISTOR SPECIFICATIONS 00038 // resistance at 25 degrees C 00039 #define THERMISTORNOMINAL 10000 00040 // temp. for nominal resistance (almost always 25 C) 00041 #define TEMPERATURENOMINAL 25 00042 // The beta coefficient of the thermistor (usually 3000-4000) 00043 #define BCOEFFICIENT 3900 00044 // the value of the 'other' resistor 00045 #define SERIESRESISTOR 3300 00046 00047 #include "mbed.h" 00048 00049 class SimonK_I2C_ESC { 00050 public: 00051 /** Constructor for ESC instance. Requires input of I2C address (ESC 0,1...16 is address 00052 * 0x29,0x2A...0x39). Optionally accepts pole count for RPM measurements. T100 is 6 and 00053 * T200 is 7. */ 00054 SimonK_I2C_ESC(I2C &_i2c, char address, char poleCount = 6); 00055 00056 /** Sends updated throttle setting for the motor. Throttle input range is 16 bit 00057 * (-32767 to +32767). */ 00058 void set(short throttle); 00059 00060 /** Alternate function to set throttle using standard servo pulse range (1100-1900) */ 00061 void setPWM(short pwm); 00062 00063 /** The update function reads new data from the ESC. */ 00064 void update(); 00065 00066 /** Returns true if the ESC is connected */ 00067 bool isAlive(); 00068 00069 /** Returns voltage measured by the ESC in volts */ 00070 float voltage(); 00071 00072 /** Returns current measured by the ESC in amps */ 00073 float current(); 00074 00075 /** Returns temperature measured by the ESC in degree Celsius */ 00076 float temperature(); 00077 00078 /** Returns RPM of the motor. Note that this measurement will be 00079 * more accurate if the I2C data is read slowly. */ 00080 short rpm(); 00081 00082 private: 00083 I2C _i2c; 00084 char _address; 00085 unsigned short _voltage_raw, _current_raw, _temp_raw; 00086 short _rpm; 00087 int _rpmTimer; 00088 Timer mbed_rpm_timer; 00089 char _identifier; 00090 char _poleCount; 00091 00092 void readBuffer(char buffer[]); 00093 00094 void readSensors(char address, unsigned short *rpm, unsigned short *vbat, unsigned short *temp, unsigned short *curr); 00095 }; 00096 00097 #endif
Generated on Tue Jul 12 2022 20:03:33 by
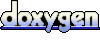