
ok
Fork of rtos_basic by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "cmsis_os.h" 00003 #include "rtos.h" 00004 00005 Serial pc(USBTX, USBRX); 00006 00007 00008 /* 00009 typedef enum { 00010 osPriorityIdle = -3, ///< priority: idle (lowest) 00011 osPriorityLow = -2, ///< priority: low 00012 osPriorityBelowNormal = -1, ///< priority: below normal 00013 osPriorityNormal = 0, ///< priority: normal (default) 00014 osPriorityAboveNormal = +1, ///< priority: above normal 00015 osPriorityHigh = +2, ///< priority: high 00016 osPriorityRealtime = +3, ///< priority: realtime (highest) 00017 osPriorityError = 0x84 ///< system cannot determine priority or thread has illegal priority 00018 } osPriority; 00019 */ 00020 00021 00022 DigitalOut led1(LED1); 00023 DigitalOut led2(LED2); 00024 00025 /* 00026 * This thread just flips the led2 00027 */ 00028 00029 00030 void led2_thread(void const *args) { 00031 while (true) { 00032 led2 = !led2; 00033 Thread::wait(1000); 00034 } 00035 } 00036 00037 00038 /* 00039 * This thread does non blocking read 00040 */ 00041 00042 void nbread_thread(void const *args) { 00043 while(1) { 00044 if(pc.readable()) { 00045 pc.printf("got a character: %c\r\n", pc.getc()); 00046 } 00047 00048 Thread::wait(10); 00049 } 00050 } 00051 00052 /* 00053 * the main thread sets upthread priorities and flips led's 00054 */ 00055 00056 int main() { 00057 00058 osPriority pri; 00059 Thread thread(led2_thread); 00060 Thread thread1(nbread_thread); 00061 00062 pc.printf("testing thread features \r\n"); 00063 printf("led2_thread priority: %d\r\n", thread.get_priority()); 00064 pri = osPriorityHigh; 00065 thread.set_priority(pri); 00066 printf("led2_thread priority: %d\r\n", thread.get_priority()); 00067 while (true) { 00068 led1 = !led1; 00069 Thread::wait(500); 00070 } 00071 }
Generated on Tue Jul 12 2022 23:26:46 by
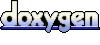