
ok
Dependencies: WiflyInterface m3pi mbed
Fork of m3pi_HelloWorld by
main.cpp
00001 //#define BASIC 00002 #ifdef BASIC 00003 #include "mbed.h" 00004 #include "m3pi.h" 00005 00006 m3pi m3pi; 00007 00008 int main() { 00009 00010 m3pi.locate(0,1); 00011 m3pi.printf("LO World"); 00012 00013 wait (2.0); 00014 00015 m3pi.forward(0.5); // Forward half speed 00016 wait (0.5); // wait half a second 00017 m3pi.left(0.5); // Turn left at half speed 00018 wait (0.5); // wait half a second 00019 m3pi.backward(0.5);// Backward at half speed 00020 wait (0.5); // wait half a second 00021 m3pi.right(0.5); // Turn right at half speed 00022 wait (0.5); // wait half a second 00023 00024 m3pi.stop(); 00025 } 00026 00027 #else 00028 #include "mbed.h" 00029 #include "m3pi.h" 00030 #include "WiflyInterface.h" 00031 00032 m3pi m3pi; 00033 int echo(); 00034 00035 00036 int main() { 00037 00038 m3pi.locate(0,1); 00039 m3pi.printf("LO World"); 00040 00041 wait (2.0); 00042 00043 m3pi.forward(0.5); // Forward half speed 00044 wait (0.5); // wait half a second 00045 m3pi.left(0.5); // Turn left at half speed 00046 wait (0.5); // wait half a second 00047 m3pi.backward(0.5);// Backward at half speed 00048 wait (0.5); // wait half a second 00049 m3pi.right(0.5); // Turn right at half speed 00050 wait (0.5); // wait half a second 00051 m3pi.stop(); 00052 00053 m3pi.stop(); 00054 //echo(); 00055 } 00056 00057 00058 00059 #define ECHO_SERVER_PORT 7 00060 00061 /* wifly object where: 00062 * - p9 and p10 are for the serial communication 00063 * - p25 is for the reset pin 00064 * - p26 is for the connection status 00065 * - "mbed" is the ssid of the network 00066 * - "password" is the password 00067 * - WPA is the security 00068 */ 00069 00070 WiflyInterface wifly(p9, p10, p25, p26, "bubbles", "", NONE); 00071 00072 int echo (void) 00073 { 00074 wifly.init(); // use DHCP 00075 while (!wifly.connect()); // join the network 00076 m3pi.printf("IP Address:port %s:%d\n\r", wifly.getIPAddress(), 7); 00077 00078 TCPSocketServer server; 00079 00080 server.bind(ECHO_SERVER_PORT); 00081 server.listen(); 00082 00083 m3pi.printf("\nWait for new connection...\n"); 00084 TCPSocketConnection client; 00085 server.accept(client); 00086 00087 char buffer[256]; 00088 while (true) { 00089 int n = client.receive(buffer, sizeof(buffer)); 00090 if (n <= 0) continue; 00091 buffer[n] = 0; 00092 00093 client.send_all(buffer, n); 00094 } 00095 } 00096 #endif 00097
Generated on Sat Jul 23 2022 09:50:39 by
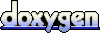