
OK View: http://sockets.mbed.org/demo/viewer
Dependencies: MMA7660 WebSocketClient WiflyInterface mbed
Fork of Websocket_Wifly_HelloWorld by
main.cpp
00001 #define OLD 00002 #ifdef OLD 00003 #include "mbed.h" 00004 #include "WiflyInterface.h" 00005 #include "Websocket.h" 00006 00007 00008 /* wifly interface: 00009 * - p9 and p10 are for the serial communication 00010 * - p19 is for the reset pin 00011 * - p26 is for the connection status 00012 * - "mbed" is the ssid of the network 00013 * - "password" is the password 00014 * - WPA is the security 00015 */ 00016 //apps board 00017 //WiflyInterface wifly(p9, p10, p30, p29, "iotlab", "42F67YxLX4AawRdcj", WPA); 00018 00019 //pololu 00020 WiflyInterface wifly(p28, p27, p26, NC, "iotlab", "42F67YxLX4AawRdcj", WPA); 00021 00022 int main() { 00023 00024 char recv[128]; 00025 00026 wifly.init(); //Use DHCP 00027 while (!wifly.connect()); 00028 printf("IP Address is %s\n\r", wifly.getIPAddress()); 00029 00030 Websocket ws("ws://echo.websocket.org/"); 00031 Websocket ws1("ws://sockets.mbed.org:443/ws/demo/wo"); 00032 while (!ws.connect()); 00033 00034 while (1) { 00035 ws.send("WebSocket Hello World over Wifly AA"); 00036 wait(1.0); 00037 printf("send OK\n\r"); 00038 if (ws.read(recv)) 00039 printf("read: %s\r\n", recv); 00040 } 00041 } 00042 00043 #endif 00044 00045 #ifdef MMA 00046 //Uses the measured z-acceleration to drive leds 2 and 3 of the mbed 00047 00048 #include "mbed.h" 00049 #include "MMA7660.h" 00050 00051 MMA7660 MMA(p28, p27); 00052 00053 DigitalOut connectionLed(LED4); 00054 PwmOut Xaxis_p(LED1); 00055 PwmOut Yaxis_p(LED2); 00056 PwmOut Zaxis_p(LED3); 00057 00058 int main() { 00059 if (MMA.testConnection()) 00060 connectionLed = 1; 00061 00062 while(1) { 00063 Xaxis_p = MMA.x(); 00064 //Zaxis_n = -MMA.z(); 00065 Yaxis_p = MMA.y(); 00066 //Zaxis_n = -MMA.y(); 00067 Zaxis_p = MMA.z(); 00068 //Zaxis_n = -MMA.z(); 00069 00070 } 00071 00072 00073 } 00074 #endif 00075 00076 #ifdef NEW 00077 #include "mbed.h" 00078 //#include "Wifly.h" 00079 #include "WiflyInterface.h" 00080 #include "Websocket.h" 00081 //#include "ADXL345.h" 00082 00083 //ADXL345 accelerometer(p5, p6, p7, p8); 00084 DigitalIn tcp(p20); 00085 00086 DigitalOut Gled(p29); 00087 DigitalOut Rled(p27); 00088 DigitalOut Yled(p28); 00089 00090 //Wifly wifly(p9, p10, p22, "mbed", "password", true); 00091 WiflyInterface wifly(p9, p10, p25, p26, "bubbles", "", NONE); 00092 //Websocket ws("ws://sockets.mbed.org/ws/sensors/wo",&wifly); 00093 Websocket ws("ws://sockets.mbed.org/ws/sensors/wo"); 00094 00095 #include "MMA7660.h" 00096 00097 MMA7660 MMA(p28, p27); 00098 00099 DigitalOut connectionLed(LED4); 00100 PwmOut Xaxis_p(LED1); 00101 PwmOut Yaxis_p(LED2); 00102 PwmOut Zaxis_p(LED3); 00103 00104 int main() { 00105 char json_str[100]; 00106 00107 int readings[3] = {0, 0, 0}; 00108 00109 //Go into standby mode to configure the device. 00110 //accelerometer.setPowerControl(0x00); 00111 //accelerometer.setDataFormatControl(0x0B); 00112 //accelerometer.setDataRate(ADXL345_3200HZ); 00113 //accelerometer.setPowerControl(0x08); 00114 00115 #ifdef DOTHIS 00116 while (!wifly.cmdMode()) { 00117 wifly.send("a\r\n"); 00118 } 00119 //wifly.send("set sys iofunc 0x40\r\n", "AOK"); 00120 #endif 00121 wifly.init(); // new code 00122 printf("here\n"); 00123 while (1) { 00124 Rled = 1; 00125 Yled = 0; 00126 Gled = 0; 00127 00128 #ifdef DOTHIS 00129 while (!wifly.join()) { 00130 wifly.reset(); 00131 } 00132 #endif 00133 00134 Rled = 0; 00135 Yled = 1; 00136 Gled = 0; 00137 00138 while (!ws.connect()); 00139 00140 Rled = 0; 00141 Yled = 0; 00142 Gled = 1; 00143 00144 while (1) { 00145 00146 wait(0.1); 00147 00148 Xaxis_p = MMA.x(); 00149 Yaxis_p = MMA.y(); 00150 Zaxis_p = MMA.z(); 00151 00152 //we read accelerometers values 00153 //accelerometer.getOutput(readings); 00154 00155 //sprintf(json_str, "{\"id\":\"wifly_acc\",\"ax\":\"%d\",\"ay\":\"%d\",\"az\":\"%d\"}", (int16_t)readings[0], (int16_t)readings[1], (int16_t)readings[2]); 00156 sprintf(json_str, "{\"id\":\"wifly_acc\",\"ax\":\"%d\",\"ay\":\"%d\",\"az\":\"%d\"}", (int16_t)Xaxis_p, (int16_t)Yaxis_p, (int16_t)Zaxis_p); 00157 ws.send(json_str); 00158 00159 if (tcp.read() != 1) { 00160 wifly.reset(); 00161 break; 00162 } 00163 } 00164 } 00165 } 00166 #endif
Generated on Tue Jul 12 2022 20:41:26 by
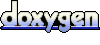