LSM6DS3 Class Reference
Class representing a LSM6DS3 sensor component. More...
#include <lsm6ds3_class.h>
Inherits GyroSensor, and MotionSensor.
Public Member Functions | |
LSM6DS3 (DevI2C &i2c, PinName irq_pin) | |
Constructor. | |
virtual | ~LSM6DS3 () |
Destructor. | |
virtual int | Init (void *init_struct) |
Initialization of sensor. | |
virtual int | ReadID (uint8_t *xg_id) |
Get ID of sensor. | |
virtual int | Get_X_Axes (int32_t *pData) |
Get current accelerometer linear acceleration X/Y/Z-axes values in standard data units [mg]. | |
virtual int | Get_X_AxesRaw (int16_t *pData) |
Get current accelerometer raw data X/Y/Z-axes values in device sepcific LSB units. | |
virtual int | Get_G_Axes (int32_t *pData) |
Get current gyroscope angular rate X/Y/Z-axes values in standard data units [mdps]. | |
virtual int | Get_G_AxesRaw (int16_t *pData) |
Get current gyroscope raw data X/Y/Z-axes values in device sepcific LSB units. | |
virtual int | Get_X_ODR (float *odr) |
Get accelerometer's current output data rate [Hz]. | |
virtual int | Set_X_ODR (float odr) |
Set accelerometer's output data rate. | |
virtual int | Get_X_Sensitivity (float *pfData) |
Get accelerometer's current sensitivity [mg/LSB]. | |
virtual int | Get_X_FS (float *fullScale) |
Get accelerometer's full scale value i.e. min/max measurable value [g]. | |
virtual int | Set_X_FS (float fullScale) |
Set accelerometer's full scale value i.e. min/max measurable value. | |
virtual int | Get_G_ODR (float *odr) |
Get gyroscope's current output data rate [Hz]. | |
virtual int | Set_G_ODR (float odr) |
Set gyroscope's output data rate. | |
virtual int | Get_G_Sensitivity (float *pfData) |
Get gyroscope's current sensitivity [mdps/LSB]. | |
virtual int | Get_G_FS (float *fullScale) |
Get gyroscope's full scale value i.e. min/max measurable value [dps]. | |
virtual int | Set_G_FS (float fullScale) |
Set gyroscope's full scale value i.e. min/max measurable value. | |
IMU_6AXES_StatusTypeDef | Enable_Free_Fall_Detection (void) |
Enable free fall detection. | |
IMU_6AXES_StatusTypeDef | Disable_Free_Fall_Detection (void) |
Disable free fall detection. | |
IMU_6AXES_StatusTypeDef | Get_Status_Free_Fall_Detection (uint8_t *status) |
Get status of free fall detection. | |
void | Attach_Free_Fall_Detection_IRQ (void(*fptr)(void)) |
Attach a function to call when a free fall is detected. | |
void | Enable_Free_Fall_Detection_IRQ (void) |
Enable Free Fall IRQ. | |
void | Disable_Free_Fall_Detection_IRQ (void) |
Disable free Fall IRQ. | |
Protected Member Functions | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Init (IMU_6AXES_InitTypeDef *LSM6DS3_Init) |
Set LSM6DS3 Initialization. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Read_XG_ID (uint8_t *xg_id) |
Read ID of LSM6DS3 Accelerometer and Gyroscope. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_GetAxes (int32_t *pData) |
Read data from LSM6DS3 Accelerometer and calculate linear acceleration in mg. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_GetAxesRaw (int16_t *pData) |
Read raw data from LSM6DS3 Accelerometer output register. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_GetAxes (int32_t *pData) |
Read data from LSM6DS3 Gyroscope and calculate angular rate in mdps. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_GetAxesRaw (int16_t *pData) |
Read raw data from LSM6DS3 Gyroscope output register. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_Get_ODR (float *odr) |
Read Accelero Output Data Rate. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_Set_ODR (float odr) |
Write Accelero Output Data Rate. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_GetSensitivity (float *pfData) |
Read Accelero Sensitivity. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_Get_FS (float *fullScale) |
Read Accelero Full Scale. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_Set_FS (float fullScale) |
Write Accelero Full Scale. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_Get_ODR (float *odr) |
Read Gyro Output Data Rate. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_Set_ODR (float odr) |
Write Gyro Output Data Rate. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_GetSensitivity (float *pfData) |
Read Gyro Sensitivity. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_Get_FS (float *fullScale) |
Read Gyro Full Scale. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_Set_FS (float fullScale) |
Write Gyro Full Scale. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Enable_Free_Fall_Detection (void) |
Enable free fall detection. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Disable_Free_Fall_Detection (void) |
Disable free fall detection. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Get_Status_Free_Fall_Detection (uint8_t *status) |
Get status of free fall detection. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_Common_Sensor_Enable (void) |
Set LSM6DS3 common initialization. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_X_Set_Axes_Status (uint8_t enableX, uint8_t enableY, uint8_t enableZ) |
Set the status of the axes for accelerometer. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_G_Set_Axes_Status (uint8_t enableX, uint8_t enableY, uint8_t enableZ) |
Set the status of the axes for gyroscope. | |
void | LSM6DS3_IO_ITConfig (void) |
Configures LSM6DS3 interrupt lines for NUCLEO boards. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_IO_Init (void) |
Configures LSM6DS3 I2C interface. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_IO_Read (uint8_t *pBuffer, uint8_t RegisterAddr, uint16_t NumByteToRead) |
Utility function to read data from LSM6DS3. | |
IMU_6AXES_StatusTypeDef | LSM6DS3_IO_Write (uint8_t *pBuffer, uint8_t RegisterAddr, uint16_t NumByteToWrite) |
Utility function to write data to LSM6DS3. |
Detailed Description
Class representing a LSM6DS3 sensor component.
Definition at line 51 of file lsm6ds3_class.h.
Constructor & Destructor Documentation
LSM6DS3 | ( | DevI2C & | i2c, |
PinName | irq_pin | ||
) |
Constructor.
- Parameters:
-
[in] i2c device I2C to be used for communication [in] irq_pin pin name for free fall detection interrupt
Definition at line 57 of file lsm6ds3_class.h.
virtual ~LSM6DS3 | ( | ) | [virtual] |
Destructor.
Definition at line 63 of file lsm6ds3_class.h.
Member Function Documentation
void Attach_Free_Fall_Detection_IRQ | ( | void(*)(void) | fptr ) |
Attach a function to call when a free fall is detected.
- Parameters:
-
[in] fptr A pointer to a void function, or 0 to set as none
Definition at line 161 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef Disable_Free_Fall_Detection | ( | void | ) |
Disable free fall detection.
- Returns:
- IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 143 of file lsm6ds3_class.h.
void Disable_Free_Fall_Detection_IRQ | ( | void | ) |
Disable free Fall IRQ.
Definition at line 173 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef Enable_Free_Fall_Detection | ( | void | ) |
Enable free fall detection.
- Returns:
- IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 135 of file lsm6ds3_class.h.
void Enable_Free_Fall_Detection_IRQ | ( | void | ) |
Enable Free Fall IRQ.
Definition at line 167 of file lsm6ds3_class.h.
virtual int Get_G_Axes | ( | int32_t * | pData ) | [virtual] |
Get current gyroscope angular rate X/Y/Z-axes values in standard data units [mdps].
- Parameters:
-
[out] pData Pointer to where to store angular rates to. pData must point to an array of (at least) three elements, where: pData[0] corresponds to X-axis, pData[1] corresponds to Y-axis, and pData[2] corresponds to Z-axis.
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 82 of file lsm6ds3_class.h.
virtual int Get_G_AxesRaw | ( | int16_t * | pData ) | [virtual] |
Get current gyroscope raw data X/Y/Z-axes values in device sepcific LSB units.
- Parameters:
-
[out] pData Pointer to where to store gyroscope raw data to. pData must point to an array of (at least) three elements, where: pData[0] corresponds to X-axis, pData[1] corresponds to Y-axis, and pData[2] corresponds to Z-axis.
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 86 of file lsm6ds3_class.h.
virtual int Get_G_FS | ( | float * | pfData ) | [virtual] |
Get gyroscope's full scale value i.e. min/max measurable value [dps].
- Parameters:
-
[out] pfData Pointer to where the gyroscope full scale value is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 122 of file lsm6ds3_class.h.
virtual int Get_G_ODR | ( | float * | pfData ) | [virtual] |
Get gyroscope's current output data rate [Hz].
- Parameters:
-
[out] pfData Pointer to where the gyroscope output data rate is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 110 of file lsm6ds3_class.h.
virtual int Get_G_Sensitivity | ( | float * | pfData ) | [virtual] |
Get gyroscope's current sensitivity [mdps/LSB].
- Parameters:
-
[out] pfData Pointer to where the gyroscope's sensitivity is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 118 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef Get_Status_Free_Fall_Detection | ( | uint8_t * | status ) |
Get status of free fall detection.
- Parameters:
-
[out] status the pointer where the status of free fall detection is stored; 0 means no detection, 1 means detection happened
- Returns:
- IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 153 of file lsm6ds3_class.h.
virtual int Get_X_Axes | ( | int32_t * | pData ) | [virtual] |
Get current accelerometer linear acceleration X/Y/Z-axes values in standard data units [mg].
- Parameters:
-
[out] pData Pointer to where to store linear accelerations to. pData must point to an array of (at least) three elements, where: pData[0] corresponds to X-axis, pData[1] corresponds to Y-axis, and pData[2] corresponds to Z-axis.
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 74 of file lsm6ds3_class.h.
virtual int Get_X_AxesRaw | ( | int16_t * | pData ) | [virtual] |
Get current accelerometer raw data X/Y/Z-axes values in device sepcific LSB units.
- Parameters:
-
[out] pData Pointer to where to store accelerometer raw data to. pData must point to an array of (at least) three elements, where: pData[0] corresponds to X-axis, pData[1] corresponds to Y-axis, and pData[2] corresponds to Z-axis.
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 78 of file lsm6ds3_class.h.
virtual int Get_X_FS | ( | float * | pfData ) | [virtual] |
Get accelerometer's full scale value i.e. min/max measurable value [g].
- Parameters:
-
[out] pfData Pointer to where the accelerometer full scale value is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 102 of file lsm6ds3_class.h.
virtual int Get_X_ODR | ( | float * | pfData ) | [virtual] |
Get accelerometer's current output data rate [Hz].
- Parameters:
-
[out] pfData Pointer to where the accelerometer output data rate is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 90 of file lsm6ds3_class.h.
virtual int Get_X_Sensitivity | ( | float * | pfData ) | [virtual] |
Get accelerometer's current sensitivity [mg/LSB].
- Parameters:
-
[out] pfData Pointer to where the accelerometer's sensitivity is stored to
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 98 of file lsm6ds3_class.h.
virtual int Init | ( | void * | ptr ) | [virtual] |
Initialization of sensor.
- Parameters:
-
[out] ptr Pointer to device specific initalization structure
- Returns:
- 0 in case of success, an error code otherwise
Implements GenericSensor.
Definition at line 66 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef LSM6DS3_Common_Sensor_Enable | ( | void | ) | [protected] |
Set LSM6DS3 common initialization.
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 132 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_Disable_Free_Fall_Detection | ( | void | ) | [protected] |
Disable free fall detection.
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 950 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_Enable_Free_Fall_Detection | ( | void | ) | [protected] |
Enable free fall detection.
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 863 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_Get_FS | ( | float * | fullScale ) | [protected] |
Read Gyro Full Scale.
- Parameters:
-
fullScale the pointer where the gyroscope full scale is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 746 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_Get_ODR | ( | float * | odr ) | [protected] |
Read Gyro Output Data Rate.
- Parameters:
-
odr the pointer where the gyroscope output data rate is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 604 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_GetAxes | ( | int32_t * | pData ) | [protected] |
Read data from LSM6DS3 Gyroscope and calculate angular rate in mdps.
- Parameters:
-
pData the pointer where the gyroscope data are stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 368 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_GetAxesRaw | ( | int16_t * | pData ) | [protected] |
Read raw data from LSM6DS3 Gyroscope output register.
- Parameters:
-
pData the pointer where the gyroscope raw data are stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 246 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_GetSensitivity | ( | float * | pfData ) | [protected] |
Read Gyro Sensitivity.
- Parameters:
-
pfData the pointer where the gyroscope sensitivity is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 693 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_Set_Axes_Status | ( | uint8_t | enableX, |
uint8_t | enableY, | ||
uint8_t | enableZ | ||
) | [protected] |
Set the status of the axes for gyroscope.
- Parameters:
-
enableX the status of the x axis to be set enableY the status of the y axis to be set enableZ the status of the z axis to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 327 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_Set_FS | ( | float | fullScale ) | [protected] |
Write Gyro Full Scale.
- Parameters:
-
fullScale the gyroscope full scale to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 799 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_G_Set_ODR | ( | float | odr ) | [protected] |
Write Gyro Output Data Rate.
- Parameters:
-
odr the gyroscope output data rate to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 657 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_Get_Status_Free_Fall_Detection | ( | uint8_t * | status ) | [protected] |
Get status of free fall detection.
- Parameters:
-
status the pointer where the status of free fall detection is stored; 0 means no detection, 1 means detection happened
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 994 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_Init | ( | IMU_6AXES_InitTypeDef * | LSM6DS3_Init ) | [protected] |
Set LSM6DS3 Initialization.
- Parameters:
-
LSM6DS3_Init the configuration setting for the LSM6DS3
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 53 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_IO_Init | ( | void | ) | [protected] |
Configures LSM6DS3 I2C interface.
- Returns:
- IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 215 of file lsm6ds3_class.h.
void LSM6DS3_IO_ITConfig | ( | void | ) | [protected] |
Configures LSM6DS3 interrupt lines for NUCLEO boards.
Definition at line 206 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef LSM6DS3_IO_Read | ( | uint8_t * | pBuffer, |
uint8_t | RegisterAddr, | ||
uint16_t | NumByteToRead | ||
) | [protected] |
Utility function to read data from LSM6DS3.
- Parameters:
-
[out] pBuffer pointer to the byte-array to read data in to [in] RegisterAddr specifies internal address register to read from. [in] NumByteToRead number of bytes to be read.
- Return values:
-
IMU_6AXES_OK if ok, IMU_6AXES_ERROR if an I2C error has occured
Definition at line 228 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef LSM6DS3_IO_Write | ( | uint8_t * | pBuffer, |
uint8_t | RegisterAddr, | ||
uint16_t | NumByteToWrite | ||
) | [protected] |
Utility function to write data to LSM6DS3.
- Parameters:
-
[in] pBuffer pointer to the byte-array data to send [in] RegisterAddr specifies internal address register to read from. [in] NumByteToWrite number of bytes to write.
- Return values:
-
IMU_6AXES_OK if ok, IMU_6AXES_ERROR if an I2C error has occured
Definition at line 249 of file lsm6ds3_class.h.
IMU_6AXES_StatusTypeDef LSM6DS3_Read_XG_ID | ( | uint8_t * | xg_id ) | [protected] |
Read ID of LSM6DS3 Accelerometer and Gyroscope.
- Parameters:
-
xg_id the pointer where the ID of the device is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 118 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_Get_FS | ( | float * | fullScale ) | [protected] |
Read Accelero Full Scale.
- Parameters:
-
fullScale the pointer where the accelerometer full scale is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 533 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_Get_ODR | ( | float * | odr ) | [protected] |
Read Accelero Output Data Rate.
- Parameters:
-
odr the pointer where the accelerometer output data rate is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 396 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_GetAxes | ( | int32_t * | pData ) | [protected] |
Read data from LSM6DS3 Accelerometer and calculate linear acceleration in mg.
- Parameters:
-
pData the pointer where the accelerometer data are stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 216 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_GetAxesRaw | ( | int16_t * | pData ) | [protected] |
Read raw data from LSM6DS3 Accelerometer output register.
- Parameters:
-
pData the pointer where the accelerometer raw data are stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 178 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_GetSensitivity | ( | float * | pfData ) | [protected] |
Read Accelero Sensitivity.
- Parameters:
-
pfData the pointer where the accelerometer sensitivity is stored
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 493 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_Set_Axes_Status | ( | uint8_t | enableX, |
uint8_t | enableY, | ||
uint8_t | enableZ | ||
) | [protected] |
Set the status of the axes for accelerometer.
- Parameters:
-
enableX the status of the x axis to be set enableY the status of the y axis to be set enableZ the status of the z axis to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 284 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_Set_FS | ( | float | fullScale ) | [protected] |
Write Accelero Full Scale.
- Parameters:
-
fullScale the accelerometer full scale to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 573 of file lsm6ds3_class.cpp.
IMU_6AXES_StatusTypeDef LSM6DS3_X_Set_ODR | ( | float | odr ) | [protected] |
Write Accelero Output Data Rate.
- Parameters:
-
odr the accelerometer output data rate to be set
- Return values:
-
IMU_6AXES_OK in case of success, an error code otherwise
Definition at line 455 of file lsm6ds3_class.cpp.
virtual int ReadID | ( | uint8_t * | id ) | [virtual] |
Get ID of sensor.
- Parameters:
-
[out] id Pointer to where to store the ID to
- Returns:
- 0 in case of success, an error code otherwise
Implements GenericSensor.
Definition at line 70 of file lsm6ds3_class.h.
virtual int Set_G_FS | ( | float | fs ) | [virtual] |
Set gyroscope's full scale value i.e. min/max measurable value.
- Parameters:
-
[in] fs New full scale value for gyroscope in [dps]
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 126 of file lsm6ds3_class.h.
virtual int Set_G_ODR | ( | float | odr ) | [virtual] |
Set gyroscope's output data rate.
- Parameters:
-
[in] odr New value for gyroscope's output data rate in [Hz]
- Returns:
- 0 in case of success, an error code otherwise
Implements GyroSensor.
Definition at line 114 of file lsm6ds3_class.h.
virtual int Set_X_FS | ( | float | fs ) | [virtual] |
Set accelerometer's full scale value i.e. min/max measurable value.
- Parameters:
-
[in] fs New full scale value for accelerometer in [g]
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 106 of file lsm6ds3_class.h.
virtual int Set_X_ODR | ( | float | odr ) | [virtual] |
Set accelerometer's output data rate.
- Parameters:
-
[in] odr New value for accelerometer's output data rate in [Hz]
- Returns:
- 0 in case of success, an error code otherwise
Implements MotionSensor.
Definition at line 94 of file lsm6ds3_class.h.
Generated on Tue Jul 12 2022 20:24:47 by
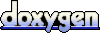