mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
xtea.h
00001 /** 00002 * \file xtea.h 00003 * 00004 * \brief XTEA block cipher (32-bit) 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_XTEA_H 00025 #define POLARSSL_XTEA_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 #define XTEA_ENCRYPT 1 00043 #define XTEA_DECRYPT 0 00044 00045 #define POLARSSL_ERR_XTEA_INVALID_INPUT_LENGTH -0x0028 /**< The data input has an invalid length. */ 00046 00047 #if !defined(POLARSSL_XTEA_ALT) 00048 // Regular implementation 00049 // 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /** 00056 * \brief XTEA context structure 00057 */ 00058 typedef struct 00059 { 00060 uint32_t k[4]; /*!< key */ 00061 } 00062 xtea_context; 00063 00064 /** 00065 * \brief Initialize XTEA context 00066 * 00067 * \param ctx XTEA context to be initialized 00068 */ 00069 void xtea_init( xtea_context *ctx ); 00070 00071 /** 00072 * \brief Clear XTEA context 00073 * 00074 * \param ctx XTEA context to be cleared 00075 */ 00076 void xtea_free( xtea_context *ctx ); 00077 00078 /** 00079 * \brief XTEA key schedule 00080 * 00081 * \param ctx XTEA context to be initialized 00082 * \param key the secret key 00083 */ 00084 void xtea_setup( xtea_context *ctx, const unsigned char key[16] ); 00085 00086 /** 00087 * \brief XTEA cipher function 00088 * 00089 * \param ctx XTEA context 00090 * \param mode XTEA_ENCRYPT or XTEA_DECRYPT 00091 * \param input 8-byte input block 00092 * \param output 8-byte output block 00093 * 00094 * \return 0 if successful 00095 */ 00096 int xtea_crypt_ecb( xtea_context *ctx, 00097 int mode, 00098 const unsigned char input[8], 00099 unsigned char output[8] ); 00100 00101 #if defined(POLARSSL_CIPHER_MODE_CBC) 00102 /** 00103 * \brief XTEA CBC cipher function 00104 * 00105 * \param ctx XTEA context 00106 * \param mode XTEA_ENCRYPT or XTEA_DECRYPT 00107 * \param length the length of input, multiple of 8 00108 * \param iv initialization vector for CBC mode 00109 * \param input input block 00110 * \param output output block 00111 * 00112 * \return 0 if successful, 00113 * POLARSSL_ERR_XTEA_INVALID_INPUT_LENGTH if the length % 8 != 0 00114 */ 00115 int xtea_crypt_cbc( xtea_context *ctx, 00116 int mode, 00117 size_t length, 00118 unsigned char iv[8], 00119 const unsigned char *input, 00120 unsigned char *output); 00121 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00122 00123 #ifdef __cplusplus 00124 } 00125 #endif 00126 00127 #else /* POLARSSL_XTEA_ALT */ 00128 #include "xtea_alt.h" 00129 #endif /* POLARSSL_XTEA_ALT */ 00130 00131 #ifdef __cplusplus 00132 extern "C" { 00133 #endif 00134 00135 /** 00136 * \brief Checkup routine 00137 * 00138 * \return 0 if successful, or 1 if the test failed 00139 */ 00140 int xtea_self_test( int verbose ); 00141 00142 #ifdef __cplusplus 00143 } 00144 #endif 00145 00146 #endif /* xtea.h */ 00147
Generated on Tue Jul 12 2022 13:50:39 by
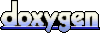