mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
x509_crt.h
00001 /** 00002 * \file x509_crt.h 00003 * 00004 * \brief X.509 certificate parsing and writing 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_X509_CRT_H 00025 #define POLARSSL_X509_CRT_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include "x509.h" 00034 #include "x509_crl.h" 00035 00036 /** 00037 * \addtogroup x509_module 00038 * \{ 00039 */ 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /** 00046 * \name Structures and functions for parsing and writing X.509 certificates 00047 * \{ 00048 */ 00049 00050 /** 00051 * Container for an X.509 certificate. The certificate may be chained. 00052 */ 00053 typedef struct _x509_crt 00054 { 00055 x509_buf raw; /**< The raw certificate data (DER). */ 00056 x509_buf tbs; /**< The raw certificate body (DER). The part that is To Be Signed. */ 00057 00058 int version; /**< The X.509 version. (1=v1, 2=v2, 3=v3) */ 00059 x509_buf serial; /**< Unique id for certificate issued by a specific CA. */ 00060 x509_buf sig_oid1; /**< Signature algorithm, e.g. sha1RSA */ 00061 00062 x509_buf issuer_raw; /**< The raw issuer data (DER). Used for quick comparison. */ 00063 x509_buf subject_raw; /**< The raw subject data (DER). Used for quick comparison. */ 00064 00065 x509_name issuer; /**< The parsed issuer data (named information object). */ 00066 x509_name subject; /**< The parsed subject data (named information object). */ 00067 00068 x509_time valid_from; /**< Start time of certificate validity. */ 00069 x509_time valid_to; /**< End time of certificate validity. */ 00070 00071 pk_context pk; /**< Container for the public key context. */ 00072 00073 x509_buf issuer_id; /**< Optional X.509 v2/v3 issuer unique identifier. */ 00074 x509_buf subject_id; /**< Optional X.509 v2/v3 subject unique identifier. */ 00075 x509_buf v3_ext; /**< Optional X.509 v3 extensions. */ 00076 x509_sequence subject_alt_names; /**< Optional list of Subject Alternative Names (Only dNSName supported). */ 00077 00078 int ext_types; /**< Bit string containing detected and parsed extensions */ 00079 int ca_istrue; /**< Optional Basic Constraint extension value: 1 if this certificate belongs to a CA, 0 otherwise. */ 00080 int max_pathlen; /**< Optional Basic Constraint extension value: The maximum path length to the root certificate. Path length is 1 higher than RFC 5280 'meaning', so 1+ */ 00081 00082 unsigned char key_usage; /**< Optional key usage extension value: See the values in x509.h */ 00083 00084 x509_sequence ext_key_usage; /**< Optional list of extended key usage OIDs. */ 00085 00086 unsigned char ns_cert_type; /**< Optional Netscape certificate type extension value: See the values in x509.h */ 00087 00088 x509_buf sig_oid2; /**< Signature algorithm. Must match sig_oid1. */ 00089 x509_buf sig; /**< Signature: hash of the tbs part signed with the private key. */ 00090 md_type_t sig_md; /**< Internal representation of the MD algorithm of the signature algorithm, e.g. POLARSSL_MD_SHA256 */ 00091 pk_type_t sig_pk; /**< Internal representation of the Public Key algorithm of the signature algorithm, e.g. POLARSSL_PK_RSA */ 00092 void *sig_opts; /**< Signature options to be passed to pk_verify_ext(), e.g. for RSASSA-PSS */ 00093 00094 struct _x509_crt *next; /**< Next certificate in the CA-chain. */ 00095 } 00096 x509_crt; 00097 00098 #define X509_CRT_VERSION_1 0 00099 #define X509_CRT_VERSION_2 1 00100 #define X509_CRT_VERSION_3 2 00101 00102 #define X509_RFC5280_MAX_SERIAL_LEN 32 00103 #define X509_RFC5280_UTC_TIME_LEN 15 00104 00105 /** 00106 * Container for writing a certificate (CRT) 00107 */ 00108 typedef struct _x509write_cert 00109 { 00110 int version; 00111 mpi serial; 00112 pk_context *subject_key; 00113 pk_context *issuer_key; 00114 asn1_named_data *subject; 00115 asn1_named_data *issuer; 00116 md_type_t md_alg; 00117 char not_before[X509_RFC5280_UTC_TIME_LEN + 1]; 00118 char not_after[X509_RFC5280_UTC_TIME_LEN + 1]; 00119 asn1_named_data *extensions; 00120 } 00121 x509write_cert; 00122 00123 #if defined(POLARSSL_X509_CRT_PARSE_C) 00124 /** 00125 * \brief Parse a single DER formatted certificate and add it 00126 * to the chained list. 00127 * 00128 * \param chain points to the start of the chain 00129 * \param buf buffer holding the certificate DER data 00130 * \param buflen size of the buffer 00131 * 00132 * \return 0 if successful, or a specific X509 or PEM error code 00133 */ 00134 int x509_crt_parse_der( x509_crt *chain, const unsigned char *buf, 00135 size_t buflen ); 00136 00137 /** 00138 * \brief Parse one or more certificates and add them 00139 * to the chained list. Parses permissively. If some 00140 * certificates can be parsed, the result is the number 00141 * of failed certificates it encountered. If none complete 00142 * correctly, the first error is returned. 00143 * 00144 * \param chain points to the start of the chain 00145 * \param buf buffer holding the certificate data 00146 * \param buflen size of the buffer 00147 * 00148 * \return 0 if all certificates parsed successfully, a positive number 00149 * if partly successful or a specific X509 or PEM error code 00150 */ 00151 int x509_crt_parse( x509_crt *chain, const unsigned char *buf, size_t buflen ); 00152 00153 #if defined(POLARSSL_FS_IO) 00154 /** 00155 * \brief Load one or more certificates and add them 00156 * to the chained list. Parses permissively. If some 00157 * certificates can be parsed, the result is the number 00158 * of failed certificates it encountered. If none complete 00159 * correctly, the first error is returned. 00160 * 00161 * \param chain points to the start of the chain 00162 * \param path filename to read the certificates from 00163 * 00164 * \return 0 if all certificates parsed successfully, a positive number 00165 * if partly successful or a specific X509 or PEM error code 00166 */ 00167 int x509_crt_parse_file( x509_crt *chain, const char *path ); 00168 00169 /** 00170 * \brief Load one or more certificate files from a path and add them 00171 * to the chained list. Parses permissively. If some 00172 * certificates can be parsed, the result is the number 00173 * of failed certificates it encountered. If none complete 00174 * correctly, the first error is returned. 00175 * 00176 * \warning This function is NOT thread-safe unless 00177 * POLARSSL_THREADING_PTHREADS is defined. If you're using an 00178 * alternative threading implementation, you should either use 00179 * this function only in the main thread, or mutex it. 00180 * 00181 * \param chain points to the start of the chain 00182 * \param path directory / folder to read the certificate files from 00183 * 00184 * \return 0 if all certificates parsed successfully, a positive number 00185 * if partly successful or a specific X509 or PEM error code 00186 */ 00187 int x509_crt_parse_path( x509_crt *chain, const char *path ); 00188 #endif /* POLARSSL_FS_IO */ 00189 00190 /** 00191 * \brief Returns an informational string about the 00192 * certificate. 00193 * 00194 * \param buf Buffer to write to 00195 * \param size Maximum size of buffer 00196 * \param prefix A line prefix 00197 * \param crt The X509 certificate to represent 00198 * 00199 * \return The amount of data written to the buffer, or -1 in 00200 * case of an error. 00201 */ 00202 int x509_crt_info( char *buf, size_t size, const char *prefix, 00203 const x509_crt *crt ); 00204 00205 /** 00206 * \brief Returns an informational string about the 00207 * verification status of a certificate. 00208 * 00209 * \param buf Buffer to write to 00210 * \param size Maximum size of buffer 00211 * \param prefix A line prefix 00212 * \param flags Verification flags created by x509_crt_verify() 00213 * 00214 * \return The amount of data written to the buffer, or -1 in 00215 * case of an error. 00216 */ 00217 int x509_crt_verify_info( char *buf, size_t size, const char *prefix, 00218 int flags ); 00219 00220 /** 00221 * \brief Verify the certificate signature 00222 * 00223 * The verify callback is a user-supplied callback that 00224 * can clear / modify / add flags for a certificate. If set, 00225 * the verification callback is called for each 00226 * certificate in the chain (from the trust-ca down to the 00227 * presented crt). The parameters for the callback are: 00228 * (void *parameter, x509_crt *crt, int certificate_depth, 00229 * int *flags). With the flags representing current flags for 00230 * that specific certificate and the certificate depth from 00231 * the bottom (Peer cert depth = 0). 00232 * 00233 * All flags left after returning from the callback 00234 * are also returned to the application. The function should 00235 * return 0 for anything but a fatal error. 00236 * 00237 * \note In case verification failed, the results can be displayed 00238 * using \c x509_crt_verify_info() 00239 * 00240 * \param crt a certificate to be verified 00241 * \param trust_ca the trusted CA chain 00242 * \param ca_crl the CRL chain for trusted CA's 00243 * \param cn expected Common Name (can be set to 00244 * NULL if the CN must not be verified) 00245 * \param flags result of the verification 00246 * \param f_vrfy verification function 00247 * \param p_vrfy verification parameter 00248 * 00249 * \return 0 if successful or POLARSSL_ERR_X509_SIG_VERIFY_FAILED, 00250 * in which case *flags will have one or more BADCERT_XXX or 00251 * BADCRL_XXX flags set, 00252 * or another error in case of a fatal error encountered 00253 * during the verification process. 00254 */ 00255 int x509_crt_verify( x509_crt *crt, 00256 x509_crt *trust_ca, 00257 x509_crl *ca_crl, 00258 const char *cn, int *flags, 00259 int (*f_vrfy)(void *, x509_crt *, int, int *), 00260 void *p_vrfy ); 00261 00262 #if defined(POLARSSL_X509_CHECK_KEY_USAGE) 00263 /** 00264 * \brief Check usage of certificate against keyUsage extension. 00265 * 00266 * \param crt Leaf certificate used. 00267 * \param usage Intended usage(s) (eg KU_KEY_ENCIPHERMENT before using the 00268 * certificate to perform an RSA key exchange). 00269 * 00270 * \return 0 is these uses of the certificate are allowed, 00271 * POLARSSL_ERR_X509_BAD_INPUT_DATA if the keyUsage extension 00272 * is present but does not contain all the bits set in the 00273 * usage argument. 00274 * 00275 * \note You should only call this function on leaf certificates, on 00276 * (intermediate) CAs the keyUsage extension is automatically 00277 * checked by \c x509_crt_verify(). 00278 */ 00279 int x509_crt_check_key_usage( const x509_crt *crt, int usage ); 00280 #endif /* POLARSSL_X509_CHECK_KEY_USAGE) */ 00281 00282 #if defined(POLARSSL_X509_CHECK_EXTENDED_KEY_USAGE) 00283 /** 00284 * \brief Check usage of certificate against extentedJeyUsage. 00285 * 00286 * \param crt Leaf certificate used. 00287 * \param usage_oid Intended usage (eg OID_SERVER_AUTH or OID_CLIENT_AUTH). 00288 * \param usage_len Length of usage_oid (eg given by OID_SIZE()). 00289 * 00290 * \return 0 is this use of the certificate is allowed, 00291 * POLARSSL_ERR_X509_BAD_INPUT_DATA if not. 00292 * 00293 * \note Usually only makes sense on leaf certificates. 00294 */ 00295 int x509_crt_check_extended_key_usage( const x509_crt *crt, 00296 const char *usage_oid, 00297 size_t usage_len ); 00298 #endif /* POLARSSL_X509_CHECK_EXTENDED_KEY_USAGE) */ 00299 00300 #if defined(POLARSSL_X509_CRL_PARSE_C) 00301 /** 00302 * \brief Verify the certificate revocation status 00303 * 00304 * \param crt a certificate to be verified 00305 * \param crl the CRL to verify against 00306 * 00307 * \return 1 if the certificate is revoked, 0 otherwise 00308 * 00309 */ 00310 int x509_crt_revoked( const x509_crt *crt, const x509_crl *crl ); 00311 #endif /* POLARSSL_X509_CRL_PARSE_C */ 00312 00313 /** 00314 * \brief Initialize a certificate (chain) 00315 * 00316 * \param crt Certificate chain to initialize 00317 */ 00318 void x509_crt_init( x509_crt *crt ); 00319 00320 /** 00321 * \brief Unallocate all certificate data 00322 * 00323 * \param crt Certificate chain to free 00324 */ 00325 void x509_crt_free( x509_crt *crt ); 00326 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00327 00328 /* \} name */ 00329 /* \} addtogroup x509_module */ 00330 00331 #if defined(POLARSSL_X509_CRT_WRITE_C) 00332 /** 00333 * \brief Initialize a CRT writing context 00334 * 00335 * \param ctx CRT context to initialize 00336 */ 00337 void x509write_crt_init( x509write_cert *ctx ); 00338 00339 /** 00340 * \brief Set the verion for a Certificate 00341 * Default: X509_CRT_VERSION_3 00342 * 00343 * \param ctx CRT context to use 00344 * \param version version to set (X509_CRT_VERSION_1, X509_CRT_VERSION_2 or 00345 * X509_CRT_VERSION_3) 00346 */ 00347 void x509write_crt_set_version( x509write_cert *ctx, int version ); 00348 00349 /** 00350 * \brief Set the serial number for a Certificate. 00351 * 00352 * \param ctx CRT context to use 00353 * \param serial serial number to set 00354 * 00355 * \return 0 if successful 00356 */ 00357 int x509write_crt_set_serial( x509write_cert *ctx, const mpi *serial ); 00358 00359 /** 00360 * \brief Set the validity period for a Certificate 00361 * Timestamps should be in string format for UTC timezone 00362 * i.e. "YYYYMMDDhhmmss" 00363 * e.g. "20131231235959" for December 31st 2013 00364 * at 23:59:59 00365 * 00366 * \param ctx CRT context to use 00367 * \param not_before not_before timestamp 00368 * \param not_after not_after timestamp 00369 * 00370 * \return 0 if timestamp was parsed successfully, or 00371 * a specific error code 00372 */ 00373 int x509write_crt_set_validity( x509write_cert *ctx, const char *not_before, 00374 const char *not_after ); 00375 00376 /** 00377 * \brief Set the issuer name for a Certificate 00378 * Issuer names should contain a comma-separated list 00379 * of OID types and values: 00380 * e.g. "C=UK,O=ARM,CN=mbed TLS CA" 00381 * 00382 * \param ctx CRT context to use 00383 * \param issuer_name issuer name to set 00384 * 00385 * \return 0 if issuer name was parsed successfully, or 00386 * a specific error code 00387 */ 00388 int x509write_crt_set_issuer_name( x509write_cert *ctx, 00389 const char *issuer_name ); 00390 00391 /** 00392 * \brief Set the subject name for a Certificate 00393 * Subject names should contain a comma-separated list 00394 * of OID types and values: 00395 * e.g. "C=UK,O=ARM,CN=mbed TLS Server 1" 00396 * 00397 * \param ctx CRT context to use 00398 * \param subject_name subject name to set 00399 * 00400 * \return 0 if subject name was parsed successfully, or 00401 * a specific error code 00402 */ 00403 int x509write_crt_set_subject_name( x509write_cert *ctx, 00404 const char *subject_name ); 00405 00406 /** 00407 * \brief Set the subject public key for the certificate 00408 * 00409 * \param ctx CRT context to use 00410 * \param key public key to include 00411 */ 00412 void x509write_crt_set_subject_key( x509write_cert *ctx, pk_context *key ); 00413 00414 /** 00415 * \brief Set the issuer key used for signing the certificate 00416 * 00417 * \param ctx CRT context to use 00418 * \param key private key to sign with 00419 */ 00420 void x509write_crt_set_issuer_key( x509write_cert *ctx, pk_context *key ); 00421 00422 /** 00423 * \brief Set the MD algorithm to use for the signature 00424 * (e.g. POLARSSL_MD_SHA1) 00425 * 00426 * \param ctx CRT context to use 00427 * \param md_alg MD algorithm to use 00428 */ 00429 void x509write_crt_set_md_alg( x509write_cert *ctx, md_type_t md_alg ); 00430 00431 /** 00432 * \brief Generic function to add to or replace an extension in the 00433 * CRT 00434 * 00435 * \param ctx CRT context to use 00436 * \param oid OID of the extension 00437 * \param oid_len length of the OID 00438 * \param critical if the extension is critical (per the RFC's definition) 00439 * \param val value of the extension OCTET STRING 00440 * \param val_len length of the value data 00441 * 00442 * \return 0 if successful, or a POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00443 */ 00444 int x509write_crt_set_extension( x509write_cert *ctx, 00445 const char *oid, size_t oid_len, 00446 int critical, 00447 const unsigned char *val, size_t val_len ); 00448 00449 /** 00450 * \brief Set the basicConstraints extension for a CRT 00451 * 00452 * \param ctx CRT context to use 00453 * \param is_ca is this a CA certificate 00454 * \param max_pathlen maximum length of certificate chains below this 00455 * certificate (only for CA certificates, -1 is 00456 * inlimited) 00457 * 00458 * \return 0 if successful, or a POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00459 */ 00460 int x509write_crt_set_basic_constraints( x509write_cert *ctx, 00461 int is_ca, int max_pathlen ); 00462 00463 #if defined(POLARSSL_SHA1_C) 00464 /** 00465 * \brief Set the subjectKeyIdentifier extension for a CRT 00466 * Requires that x509write_crt_set_subject_key() has been 00467 * called before 00468 * 00469 * \param ctx CRT context to use 00470 * 00471 * \return 0 if successful, or a POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00472 */ 00473 int x509write_crt_set_subject_key_identifier( x509write_cert *ctx ); 00474 00475 /** 00476 * \brief Set the authorityKeyIdentifier extension for a CRT 00477 * Requires that x509write_crt_set_issuer_key() has been 00478 * called before 00479 * 00480 * \param ctx CRT context to use 00481 * 00482 * \return 0 if successful, or a POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00483 */ 00484 int x509write_crt_set_authority_key_identifier( x509write_cert *ctx ); 00485 #endif /* POLARSSL_SHA1_C */ 00486 00487 /** 00488 * \brief Set the Key Usage Extension flags 00489 * (e.g. KU_DIGITAL_SIGNATURE | KU_KEY_CERT_SIGN) 00490 * 00491 * \param ctx CRT context to use 00492 * \param key_usage key usage flags to set 00493 * 00494 * \return 0 if successful, or POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00495 */ 00496 int x509write_crt_set_key_usage( x509write_cert *ctx, unsigned char key_usage ); 00497 00498 /** 00499 * \brief Set the Netscape Cert Type flags 00500 * (e.g. NS_CERT_TYPE_SSL_CLIENT | NS_CERT_TYPE_EMAIL) 00501 * 00502 * \param ctx CRT context to use 00503 * \param ns_cert_type Netscape Cert Type flags to set 00504 * 00505 * \return 0 if successful, or POLARSSL_ERR_X509WRITE_MALLOC_FAILED 00506 */ 00507 int x509write_crt_set_ns_cert_type( x509write_cert *ctx, 00508 unsigned char ns_cert_type ); 00509 00510 /** 00511 * \brief Free the contents of a CRT write context 00512 * 00513 * \param ctx CRT context to free 00514 */ 00515 void x509write_crt_free( x509write_cert *ctx ); 00516 00517 /** 00518 * \brief Write a built up certificate to a X509 DER structure 00519 * Note: data is written at the end of the buffer! Use the 00520 * return value to determine where you should start 00521 * using the buffer 00522 * 00523 * \param ctx certificate to write away 00524 * \param buf buffer to write to 00525 * \param size size of the buffer 00526 * \param f_rng RNG function (for signature, see note) 00527 * \param p_rng RNG parameter 00528 * 00529 * \return length of data written if successful, or a specific 00530 * error code 00531 * 00532 * \note f_rng may be NULL if RSA is used for signature and the 00533 * signature is made offline (otherwise f_rng is desirable 00534 * for countermeasures against timing attacks). 00535 * ECDSA signatures always require a non-NULL f_rng. 00536 */ 00537 int x509write_crt_der( x509write_cert *ctx, unsigned char *buf, size_t size, 00538 int (*f_rng)(void *, unsigned char *, size_t), 00539 void *p_rng ); 00540 00541 #if defined(POLARSSL_PEM_WRITE_C) 00542 /** 00543 * \brief Write a built up certificate to a X509 PEM string 00544 * 00545 * \param ctx certificate to write away 00546 * \param buf buffer to write to 00547 * \param size size of the buffer 00548 * \param f_rng RNG function (for signature, see note) 00549 * \param p_rng RNG parameter 00550 * 00551 * \return 0 successful, or a specific error code 00552 * 00553 * \note f_rng may be NULL if RSA is used for signature and the 00554 * signature is made offline (otherwise f_rng is desirable 00555 * for countermeasures against timing attacks). 00556 * ECDSA signatures always require a non-NULL f_rng. 00557 */ 00558 int x509write_crt_pem( x509write_cert *ctx, unsigned char *buf, size_t size, 00559 int (*f_rng)(void *, unsigned char *, size_t), 00560 void *p_rng ); 00561 #endif /* POLARSSL_PEM_WRITE_C */ 00562 #endif /* POLARSSL_X509_CRT_WRITE_C */ 00563 00564 #ifdef __cplusplus 00565 } 00566 #endif 00567 00568 #endif /* x509_crt.h */ 00569
Generated on Tue Jul 12 2022 13:50:39 by
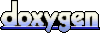