mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
x509.h
00001 /** 00002 * \file x509.h 00003 * 00004 * \brief X.509 generic defines and structures 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_X509_H 00025 #define POLARSSL_X509_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include "asn1.h" 00034 #include "pk.h" 00035 00036 #if defined(POLARSSL_RSA_C) 00037 #include "rsa.h" 00038 #endif 00039 00040 /** 00041 * \addtogroup x509_module 00042 * \{ 00043 */ 00044 00045 #if !defined(POLARSSL_X509_MAX_INTERMEDIATE_CA) 00046 /** 00047 * Maximum number of intermediate CAs in a verification chain. 00048 * That is, maximum length of the chain, excluding the end-entity certificate 00049 * and the trusted root certificate. 00050 * 00051 * Set this to a low value to prevent an adversary from making you waste 00052 * resources verifying an overlong certificate chain. 00053 */ 00054 #define POLARSSL_X509_MAX_INTERMEDIATE_CA 8 00055 #endif 00056 00057 /** 00058 * \name X509 Error codes 00059 * \{ 00060 */ 00061 #define POLARSSL_ERR_X509_FEATURE_UNAVAILABLE -0x2080 /**< Unavailable feature, e.g. RSA hashing/encryption combination. */ 00062 #define POLARSSL_ERR_X509_UNKNOWN_OID -0x2100 /**< Requested OID is unknown. */ 00063 #define POLARSSL_ERR_X509_INVALID_FORMAT -0x2180 /**< The CRT/CRL/CSR format is invalid, e.g. different type expected. */ 00064 #define POLARSSL_ERR_X509_INVALID_VERSION -0x2200 /**< The CRT/CRL/CSR version element is invalid. */ 00065 #define POLARSSL_ERR_X509_INVALID_SERIAL -0x2280 /**< The serial tag or value is invalid. */ 00066 #define POLARSSL_ERR_X509_INVALID_ALG -0x2300 /**< The algorithm tag or value is invalid. */ 00067 #define POLARSSL_ERR_X509_INVALID_NAME -0x2380 /**< The name tag or value is invalid. */ 00068 #define POLARSSL_ERR_X509_INVALID_DATE -0x2400 /**< The date tag or value is invalid. */ 00069 #define POLARSSL_ERR_X509_INVALID_SIGNATURE -0x2480 /**< The signature tag or value invalid. */ 00070 #define POLARSSL_ERR_X509_INVALID_EXTENSIONS -0x2500 /**< The extension tag or value is invalid. */ 00071 #define POLARSSL_ERR_X509_UNKNOWN_VERSION -0x2580 /**< CRT/CRL/CSR has an unsupported version number. */ 00072 #define POLARSSL_ERR_X509_UNKNOWN_SIG_ALG -0x2600 /**< Signature algorithm (oid) is unsupported. */ 00073 #define POLARSSL_ERR_X509_SIG_MISMATCH -0x2680 /**< Signature algorithms do not match. (see \c ::x509_crt sig_oid) */ 00074 #define POLARSSL_ERR_X509_CERT_VERIFY_FAILED -0x2700 /**< Certificate verification failed, e.g. CRL, CA or signature check failed. */ 00075 #define POLARSSL_ERR_X509_CERT_UNKNOWN_FORMAT -0x2780 /**< Format not recognized as DER or PEM. */ 00076 #define POLARSSL_ERR_X509_BAD_INPUT_DATA -0x2800 /**< Input invalid. */ 00077 #define POLARSSL_ERR_X509_MALLOC_FAILED -0x2880 /**< Allocation of memory failed. */ 00078 #define POLARSSL_ERR_X509_FILE_IO_ERROR -0x2900 /**< Read/write of file failed. */ 00079 /* \} name */ 00080 00081 /** 00082 * \name X509 Verify codes 00083 * \{ 00084 */ 00085 /* Reminder: update x509_crt_verify_strings[] in library/x509_crt.c */ 00086 #define BADCERT_EXPIRED 0x01 /**< The certificate validity has expired. */ 00087 #define BADCERT_REVOKED 0x02 /**< The certificate has been revoked (is on a CRL). */ 00088 #define BADCERT_CN_MISMATCH 0x04 /**< The certificate Common Name (CN) does not match with the expected CN. */ 00089 #define BADCERT_NOT_TRUSTED 0x08 /**< The certificate is not correctly signed by the trusted CA. */ 00090 #define BADCRL_NOT_TRUSTED 0x10 /**< The CRL is not correctly signed by the trusted CA. */ 00091 #define BADCRL_EXPIRED 0x20 /**< The CRL is expired. */ 00092 #define BADCERT_MISSING 0x40 /**< Certificate was missing. */ 00093 #define BADCERT_SKIP_VERIFY 0x80 /**< Certificate verification was skipped. */ 00094 #define BADCERT_OTHER 0x0100 /**< Other reason (can be used by verify callback) */ 00095 #define BADCERT_FUTURE 0x0200 /**< The certificate validity starts in the future. */ 00096 #define BADCRL_FUTURE 0x0400 /**< The CRL is from the future */ 00097 #define BADCERT_KEY_USAGE 0x0800 /**< Usage does not match the keyUsage extension. */ 00098 #define BADCERT_EXT_KEY_USAGE 0x1000 /**< Usage does not match the extendedKeyUsage extension. */ 00099 #define BADCERT_NS_CERT_TYPE 0x2000 /**< Usage does not match the nsCertType extension. */ 00100 /* \} name */ 00101 /* \} addtogroup x509_module */ 00102 00103 /* 00104 * X.509 v3 Key Usage Extension flags 00105 */ 00106 #define KU_DIGITAL_SIGNATURE (0x80) /* bit 0 */ 00107 #define KU_NON_REPUDIATION (0x40) /* bit 1 */ 00108 #define KU_KEY_ENCIPHERMENT (0x20) /* bit 2 */ 00109 #define KU_DATA_ENCIPHERMENT (0x10) /* bit 3 */ 00110 #define KU_KEY_AGREEMENT (0x08) /* bit 4 */ 00111 #define KU_KEY_CERT_SIGN (0x04) /* bit 5 */ 00112 #define KU_CRL_SIGN (0x02) /* bit 6 */ 00113 00114 /* 00115 * Netscape certificate types 00116 * (http://www.mozilla.org/projects/security/pki/nss/tech-notes/tn3.html) 00117 */ 00118 00119 #define NS_CERT_TYPE_SSL_CLIENT (0x80) /* bit 0 */ 00120 #define NS_CERT_TYPE_SSL_SERVER (0x40) /* bit 1 */ 00121 #define NS_CERT_TYPE_EMAIL (0x20) /* bit 2 */ 00122 #define NS_CERT_TYPE_OBJECT_SIGNING (0x10) /* bit 3 */ 00123 #define NS_CERT_TYPE_RESERVED (0x08) /* bit 4 */ 00124 #define NS_CERT_TYPE_SSL_CA (0x04) /* bit 5 */ 00125 #define NS_CERT_TYPE_EMAIL_CA (0x02) /* bit 6 */ 00126 #define NS_CERT_TYPE_OBJECT_SIGNING_CA (0x01) /* bit 7 */ 00127 00128 /* 00129 * X.509 extension types 00130 * 00131 * Comments refer to the status for using certificates. Status can be 00132 * different for writing certificates or reading CRLs or CSRs. 00133 */ 00134 #define EXT_AUTHORITY_KEY_IDENTIFIER (1 << 0) 00135 #define EXT_SUBJECT_KEY_IDENTIFIER (1 << 1) 00136 #define EXT_KEY_USAGE (1 << 2) /* Parsed but not used */ 00137 #define EXT_CERTIFICATE_POLICIES (1 << 3) 00138 #define EXT_POLICY_MAPPINGS (1 << 4) 00139 #define EXT_SUBJECT_ALT_NAME (1 << 5) /* Supported (DNS) */ 00140 #define EXT_ISSUER_ALT_NAME (1 << 6) 00141 #define EXT_SUBJECT_DIRECTORY_ATTRS (1 << 7) 00142 #define EXT_BASIC_CONSTRAINTS (1 << 8) /* Supported */ 00143 #define EXT_NAME_CONSTRAINTS (1 << 9) 00144 #define EXT_POLICY_CONSTRAINTS (1 << 10) 00145 #define EXT_EXTENDED_KEY_USAGE (1 << 11) /* Parsed but not used */ 00146 #define EXT_CRL_DISTRIBUTION_POINTS (1 << 12) 00147 #define EXT_INIHIBIT_ANYPOLICY (1 << 13) 00148 #define EXT_FRESHEST_CRL (1 << 14) 00149 00150 #define EXT_NS_CERT_TYPE (1 << 16) /* Parsed (and then ?) */ 00151 00152 /* 00153 * Storage format identifiers 00154 * Recognized formats: PEM and DER 00155 */ 00156 #define X509_FORMAT_DER 1 00157 #define X509_FORMAT_PEM 2 00158 00159 #define X509_MAX_DN_NAME_SIZE 256 /**< Maximum value size of a DN entry */ 00160 00161 #ifdef __cplusplus 00162 extern "C" { 00163 #endif 00164 00165 /** 00166 * \addtogroup x509_module 00167 * \{ */ 00168 00169 /** 00170 * \name Structures for parsing X.509 certificates, CRLs and CSRs 00171 * \{ 00172 */ 00173 00174 /** 00175 * Type-length-value structure that allows for ASN1 using DER. 00176 */ 00177 typedef asn1_buf x509_buf; 00178 00179 /** 00180 * Container for ASN1 bit strings. 00181 */ 00182 typedef asn1_bitstring x509_bitstring; 00183 00184 /** 00185 * Container for ASN1 named information objects. 00186 * It allows for Relative Distinguished Names (e.g. cn=polarssl,ou=code,etc.). 00187 */ 00188 typedef asn1_named_data x509_name; 00189 00190 /** 00191 * Container for a sequence of ASN.1 items 00192 */ 00193 typedef asn1_sequence x509_sequence; 00194 00195 /** Container for date and time (precision in seconds). */ 00196 typedef struct _x509_time 00197 { 00198 int year, mon, day; /**< Date. */ 00199 int hour, min, sec; /**< Time. */ 00200 } 00201 x509_time; 00202 00203 /** \} name Structures for parsing X.509 certificates, CRLs and CSRs */ 00204 /** \} addtogroup x509_module */ 00205 00206 /** 00207 * \brief Store the certificate DN in printable form into buf; 00208 * no more than size characters will be written. 00209 * 00210 * \param buf Buffer to write to 00211 * \param size Maximum size of buffer 00212 * \param dn The X509 name to represent 00213 * 00214 * \return The amount of data written to the buffer, or -1 in 00215 * case of an error. 00216 */ 00217 int x509_dn_gets( char *buf, size_t size, const x509_name *dn ); 00218 00219 /** 00220 * \brief Store the certificate serial in printable form into buf; 00221 * no more than size characters will be written. 00222 * 00223 * \param buf Buffer to write to 00224 * \param size Maximum size of buffer 00225 * \param serial The X509 serial to represent 00226 * 00227 * \return The amount of data written to the buffer, or -1 in 00228 * case of an error. 00229 */ 00230 int x509_serial_gets( char *buf, size_t size, const x509_buf *serial ); 00231 00232 #if ! defined(POLARSSL_DEPRECATED_REMOVED) 00233 #if defined(POLARSSL_DEPRECATED_WARNING) 00234 #define DEPRECATED __attribute__((deprecated)) 00235 #else 00236 #define DEPRECATED 00237 #endif 00238 /** 00239 * \brief Give an known OID, return its descriptive string. 00240 * 00241 * \deprecated Use oid_get_extended_key_usage() instead. 00242 * 00243 * \warning Only works for extended_key_usage OIDs! 00244 * 00245 * \param oid buffer containing the oid 00246 * 00247 * \return Return a string if the OID is known, 00248 * or NULL otherwise. 00249 */ 00250 const char *x509_oid_get_description( x509_buf *oid ) DEPRECATED; 00251 00252 /** 00253 * \brief Give an OID, return a string version of its OID number. 00254 * 00255 * \deprecated Use oid_get_numeric_string() instead. 00256 * 00257 * \param buf Buffer to write to 00258 * \param size Maximum size of buffer 00259 * \param oid Buffer containing the OID 00260 * 00261 * \return Length of the string written (excluding final NULL) or 00262 * POLARSSL_ERR_OID_BUF_TO_SMALL in case of error 00263 */ 00264 int x509_oid_get_numeric_string( char *buf, size_t size, x509_buf *oid ) DEPRECATED; 00265 #undef DEPRECATED 00266 #endif /* POLARSSL_DEPRECATED_REMOVED */ 00267 00268 /** 00269 * \brief Check a given x509_time against the system time and check 00270 * if it is not expired. 00271 * 00272 * \param time x509_time to check 00273 * 00274 * \return 0 if the x509_time is still valid, 00275 * 1 otherwise. 00276 */ 00277 int x509_time_expired( const x509_time *time ); 00278 00279 /** 00280 * \brief Check a given x509_time against the system time and check 00281 * if it is not from the future. 00282 * 00283 * \param time x509_time to check 00284 * 00285 * \return 0 if the x509_time is already valid, 00286 * 1 otherwise. 00287 */ 00288 int x509_time_future( const x509_time *time ); 00289 00290 /** 00291 * \brief Checkup routine 00292 * 00293 * \return 0 if successful, or 1 if the test failed 00294 */ 00295 int x509_self_test( int verbose ); 00296 00297 /* 00298 * Internal module functions. You probably do not want to use these unless you 00299 * know you do. 00300 */ 00301 int x509_get_name( unsigned char **p, const unsigned char *end, 00302 x509_name *cur ); 00303 int x509_get_alg_null( unsigned char **p, const unsigned char *end, 00304 x509_buf *alg ); 00305 int x509_get_alg( unsigned char **p, const unsigned char *end, 00306 x509_buf *alg, x509_buf *params ); 00307 #if defined(POLARSSL_X509_RSASSA_PSS_SUPPORT) 00308 int x509_get_rsassa_pss_params( const x509_buf *params, 00309 md_type_t *md_alg, md_type_t *mgf_md, 00310 int *salt_len ); 00311 #endif 00312 int x509_get_sig( unsigned char **p, const unsigned char *end, x509_buf *sig ); 00313 int x509_get_sig_alg( const x509_buf *sig_oid, const x509_buf *sig_params, 00314 md_type_t *md_alg, pk_type_t *pk_alg, 00315 void **sig_opts ); 00316 int x509_get_time( unsigned char **p, const unsigned char *end, 00317 x509_time *time ); 00318 int x509_get_serial( unsigned char **p, const unsigned char *end, 00319 x509_buf *serial ); 00320 int x509_get_ext( unsigned char **p, const unsigned char *end, 00321 x509_buf *ext, int tag ); 00322 int x509_sig_alg_gets( char *buf, size_t size, const x509_buf *sig_oid, 00323 pk_type_t pk_alg, md_type_t md_alg, 00324 const void *sig_opts ); 00325 int x509_key_size_helper( char *buf, size_t size, const char *name ); 00326 int x509_string_to_names( asn1_named_data **head, const char *name ); 00327 int x509_set_extension( asn1_named_data **head, const char *oid, size_t oid_len, 00328 int critical, const unsigned char *val, 00329 size_t val_len ); 00330 int x509_write_extensions( unsigned char **p, unsigned char *start, 00331 asn1_named_data *first ); 00332 int x509_write_names( unsigned char **p, unsigned char *start, 00333 asn1_named_data *first ); 00334 int x509_write_sig( unsigned char **p, unsigned char *start, 00335 const char *oid, size_t oid_len, 00336 unsigned char *sig, size_t size ); 00337 00338 #ifdef __cplusplus 00339 } 00340 #endif 00341 00342 #endif /* x509.h */ 00343
Generated on Tue Jul 12 2022 13:50:39 by
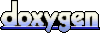