mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ssl_cache.h
00001 /** 00002 * \file ssl_cache.h 00003 * 00004 * \brief SSL session cache implementation 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_SSL_CACHE_H 00025 #define POLARSSL_SSL_CACHE_H 00026 00027 #include "ssl.h" 00028 00029 #if defined(POLARSSL_THREADING_C) 00030 #include "threading.h" 00031 #endif 00032 00033 /** 00034 * \name SECTION: Module settings 00035 * 00036 * The configuration options you can set for this module are in this section. 00037 * Either change them in config.h or define them on the compiler command line. 00038 * \{ 00039 */ 00040 00041 #if !defined(SSL_CACHE_DEFAULT_TIMEOUT) 00042 #define SSL_CACHE_DEFAULT_TIMEOUT 86400 /*!< 1 day */ 00043 #endif 00044 00045 #if !defined(SSL_CACHE_DEFAULT_MAX_ENTRIES) 00046 #define SSL_CACHE_DEFAULT_MAX_ENTRIES 50 /*!< Maximum entries in cache */ 00047 #endif 00048 00049 /* \} name SECTION: Module settings */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 typedef struct _ssl_cache_context ssl_cache_context; 00056 typedef struct _ssl_cache_entry ssl_cache_entry; 00057 00058 /** 00059 * \brief This structure is used for storing cache entries 00060 */ 00061 struct _ssl_cache_entry 00062 { 00063 #if defined(POLARSSL_HAVE_TIME) 00064 time_t timestamp ; /*!< entry timestamp */ 00065 #endif 00066 ssl_session session ; /*!< entry session */ 00067 #if defined(POLARSSL_X509_CRT_PARSE_C) 00068 x509_buf peer_cert ; /*!< entry peer_cert */ 00069 #endif 00070 ssl_cache_entry *next ; /*!< chain pointer */ 00071 }; 00072 00073 /** 00074 * \brief Cache context 00075 */ 00076 struct _ssl_cache_context 00077 { 00078 ssl_cache_entry *chain ; /*!< start of the chain */ 00079 int timeout ; /*!< cache entry timeout */ 00080 int max_entries ; /*!< maximum entries */ 00081 #if defined(POLARSSL_THREADING_C) 00082 threading_mutex_t mutex ; /*!< mutex */ 00083 #endif 00084 }; 00085 00086 /** 00087 * \brief Initialize an SSL cache context 00088 * 00089 * \param cache SSL cache context 00090 */ 00091 void ssl_cache_init( ssl_cache_context *cache ); 00092 00093 /** 00094 * \brief Cache get callback implementation 00095 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00096 * 00097 * \param data SSL cache context 00098 * \param session session to retrieve entry for 00099 */ 00100 int ssl_cache_get( void *data, ssl_session *session ); 00101 00102 /** 00103 * \brief Cache set callback implementation 00104 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00105 * 00106 * \param data SSL cache context 00107 * \param session session to store entry for 00108 */ 00109 int ssl_cache_set( void *data, const ssl_session *session ); 00110 00111 #if defined(POLARSSL_HAVE_TIME) 00112 /** 00113 * \brief Set the cache timeout 00114 * (Default: SSL_CACHE_DEFAULT_TIMEOUT (1 day)) 00115 * 00116 * A timeout of 0 indicates no timeout. 00117 * 00118 * \param cache SSL cache context 00119 * \param timeout cache entry timeout in seconds 00120 */ 00121 void ssl_cache_set_timeout( ssl_cache_context *cache, int timeout ); 00122 #endif /* POLARSSL_HAVE_TIME */ 00123 00124 /** 00125 * \brief Set the cache timeout 00126 * (Default: SSL_CACHE_DEFAULT_MAX_ENTRIES (50)) 00127 * 00128 * \param cache SSL cache context 00129 * \param max cache entry maximum 00130 */ 00131 void ssl_cache_set_max_entries( ssl_cache_context *cache, int max ); 00132 00133 /** 00134 * \brief Free referenced items in a cache context and clear memory 00135 * 00136 * \param cache SSL cache context 00137 */ 00138 void ssl_cache_free( ssl_cache_context *cache ); 00139 00140 #ifdef __cplusplus 00141 } 00142 #endif 00143 00144 #endif /* ssl_cache.h */ 00145
Generated on Tue Jul 12 2022 13:50:38 by
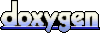