mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
sha256.c
00001 /* 00002 * FIPS-180-2 compliant SHA-256 implementation 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 /* 00023 * The SHA-256 Secure Hash Standard was published by NIST in 2002. 00024 * 00025 * http://csrc.nist.gov/publications/fips/fips180-2/fips180-2.pdf 00026 */ 00027 00028 #if !defined(POLARSSL_CONFIG_FILE) 00029 #include "polarssl/config.h" 00030 #else 00031 #include POLARSSL_CONFIG_FILE 00032 #endif 00033 00034 #if defined(POLARSSL_SHA256_C) 00035 00036 #include "polarssl/sha256.h" 00037 00038 #include <string.h> 00039 00040 #if defined(POLARSSL_FS_IO) 00041 #include <stdio.h> 00042 #endif 00043 00044 #if defined(POLARSSL_SELF_TEST) 00045 #if defined(POLARSSL_PLATFORM_C) 00046 #include "polarssl/platform.h" 00047 #else 00048 #include <stdio.h> 00049 #define polarssl_printf printf 00050 #endif /* POLARSSL_PLATFORM_C */ 00051 #endif /* POLARSSL_SELF_TEST */ 00052 00053 /* Implementation that should never be optimized out by the compiler */ 00054 static void polarssl_zeroize( void *v, size_t n ) { 00055 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00056 } 00057 00058 #if !defined(POLARSSL_SHA256_ALT) 00059 00060 /* 00061 * 32-bit integer manipulation macros (big endian) 00062 */ 00063 #ifndef GET_UINT32_BE 00064 #define GET_UINT32_BE(n,b,i) \ 00065 { \ 00066 (n) = ( (uint32_t) (b)[(i) ] << 24 ) \ 00067 | ( (uint32_t) (b)[(i) + 1] << 16 ) \ 00068 | ( (uint32_t) (b)[(i) + 2] << 8 ) \ 00069 | ( (uint32_t) (b)[(i) + 3] ); \ 00070 } 00071 #endif 00072 00073 #ifndef PUT_UINT32_BE 00074 #define PUT_UINT32_BE(n,b,i) \ 00075 { \ 00076 (b)[(i) ] = (unsigned char) ( (n) >> 24 ); \ 00077 (b)[(i) + 1] = (unsigned char) ( (n) >> 16 ); \ 00078 (b)[(i) + 2] = (unsigned char) ( (n) >> 8 ); \ 00079 (b)[(i) + 3] = (unsigned char) ( (n) ); \ 00080 } 00081 #endif 00082 00083 void sha256_init( sha256_context *ctx ) 00084 { 00085 memset( ctx, 0, sizeof( sha256_context ) ); 00086 } 00087 00088 void sha256_free( sha256_context *ctx ) 00089 { 00090 if( ctx == NULL ) 00091 return; 00092 00093 polarssl_zeroize( ctx, sizeof( sha256_context ) ); 00094 } 00095 00096 /* 00097 * SHA-256 context setup 00098 */ 00099 void sha256_starts( sha256_context *ctx, int is224 ) 00100 { 00101 ctx->total [0] = 0; 00102 ctx->total [1] = 0; 00103 00104 if( is224 == 0 ) 00105 { 00106 /* SHA-256 */ 00107 ctx->state [0] = 0x6A09E667; 00108 ctx->state [1] = 0xBB67AE85; 00109 ctx->state [2] = 0x3C6EF372; 00110 ctx->state [3] = 0xA54FF53A; 00111 ctx->state [4] = 0x510E527F; 00112 ctx->state [5] = 0x9B05688C; 00113 ctx->state [6] = 0x1F83D9AB; 00114 ctx->state [7] = 0x5BE0CD19; 00115 } 00116 else 00117 { 00118 /* SHA-224 */ 00119 ctx->state [0] = 0xC1059ED8; 00120 ctx->state [1] = 0x367CD507; 00121 ctx->state [2] = 0x3070DD17; 00122 ctx->state [3] = 0xF70E5939; 00123 ctx->state [4] = 0xFFC00B31; 00124 ctx->state [5] = 0x68581511; 00125 ctx->state [6] = 0x64F98FA7; 00126 ctx->state [7] = 0xBEFA4FA4; 00127 } 00128 00129 ctx->is224 = is224; 00130 } 00131 00132 void sha256_process( sha256_context *ctx, const unsigned char data[64] ) 00133 { 00134 uint32_t temp1, temp2, W[64]; 00135 uint32_t A, B, C, D, E, F, G, H; 00136 00137 GET_UINT32_BE( W[ 0], data, 0 ); 00138 GET_UINT32_BE( W[ 1], data, 4 ); 00139 GET_UINT32_BE( W[ 2], data, 8 ); 00140 GET_UINT32_BE( W[ 3], data, 12 ); 00141 GET_UINT32_BE( W[ 4], data, 16 ); 00142 GET_UINT32_BE( W[ 5], data, 20 ); 00143 GET_UINT32_BE( W[ 6], data, 24 ); 00144 GET_UINT32_BE( W[ 7], data, 28 ); 00145 GET_UINT32_BE( W[ 8], data, 32 ); 00146 GET_UINT32_BE( W[ 9], data, 36 ); 00147 GET_UINT32_BE( W[10], data, 40 ); 00148 GET_UINT32_BE( W[11], data, 44 ); 00149 GET_UINT32_BE( W[12], data, 48 ); 00150 GET_UINT32_BE( W[13], data, 52 ); 00151 GET_UINT32_BE( W[14], data, 56 ); 00152 GET_UINT32_BE( W[15], data, 60 ); 00153 00154 #define SHR(x,n) ((x & 0xFFFFFFFF) >> n) 00155 #define ROTR(x,n) (SHR(x,n) | (x << (32 - n))) 00156 00157 #define S0(x) (ROTR(x, 7) ^ ROTR(x,18) ^ SHR(x, 3)) 00158 #define S1(x) (ROTR(x,17) ^ ROTR(x,19) ^ SHR(x,10)) 00159 00160 #define S2(x) (ROTR(x, 2) ^ ROTR(x,13) ^ ROTR(x,22)) 00161 #define S3(x) (ROTR(x, 6) ^ ROTR(x,11) ^ ROTR(x,25)) 00162 00163 #define F0(x,y,z) ((x & y) | (z & (x | y))) 00164 #define F1(x,y,z) (z ^ (x & (y ^ z))) 00165 00166 #define R(t) \ 00167 ( \ 00168 W[t] = S1(W[t - 2]) + W[t - 7] + \ 00169 S0(W[t - 15]) + W[t - 16] \ 00170 ) 00171 00172 #define P(a,b,c,d,e,f,g,h,x,K) \ 00173 { \ 00174 temp1 = h + S3(e) + F1(e,f,g) + K + x; \ 00175 temp2 = S2(a) + F0(a,b,c); \ 00176 d += temp1; h = temp1 + temp2; \ 00177 } 00178 00179 A = ctx->state [0]; 00180 B = ctx->state [1]; 00181 C = ctx->state [2]; 00182 D = ctx->state [3]; 00183 E = ctx->state [4]; 00184 F = ctx->state [5]; 00185 G = ctx->state [6]; 00186 H = ctx->state [7]; 00187 00188 P( A, B, C, D, E, F, G, H, W[ 0], 0x428A2F98 ); 00189 P( H, A, B, C, D, E, F, G, W[ 1], 0x71374491 ); 00190 P( G, H, A, B, C, D, E, F, W[ 2], 0xB5C0FBCF ); 00191 P( F, G, H, A, B, C, D, E, W[ 3], 0xE9B5DBA5 ); 00192 P( E, F, G, H, A, B, C, D, W[ 4], 0x3956C25B ); 00193 P( D, E, F, G, H, A, B, C, W[ 5], 0x59F111F1 ); 00194 P( C, D, E, F, G, H, A, B, W[ 6], 0x923F82A4 ); 00195 P( B, C, D, E, F, G, H, A, W[ 7], 0xAB1C5ED5 ); 00196 P( A, B, C, D, E, F, G, H, W[ 8], 0xD807AA98 ); 00197 P( H, A, B, C, D, E, F, G, W[ 9], 0x12835B01 ); 00198 P( G, H, A, B, C, D, E, F, W[10], 0x243185BE ); 00199 P( F, G, H, A, B, C, D, E, W[11], 0x550C7DC3 ); 00200 P( E, F, G, H, A, B, C, D, W[12], 0x72BE5D74 ); 00201 P( D, E, F, G, H, A, B, C, W[13], 0x80DEB1FE ); 00202 P( C, D, E, F, G, H, A, B, W[14], 0x9BDC06A7 ); 00203 P( B, C, D, E, F, G, H, A, W[15], 0xC19BF174 ); 00204 P( A, B, C, D, E, F, G, H, R(16), 0xE49B69C1 ); 00205 P( H, A, B, C, D, E, F, G, R(17), 0xEFBE4786 ); 00206 P( G, H, A, B, C, D, E, F, R(18), 0x0FC19DC6 ); 00207 P( F, G, H, A, B, C, D, E, R(19), 0x240CA1CC ); 00208 P( E, F, G, H, A, B, C, D, R(20), 0x2DE92C6F ); 00209 P( D, E, F, G, H, A, B, C, R(21), 0x4A7484AA ); 00210 P( C, D, E, F, G, H, A, B, R(22), 0x5CB0A9DC ); 00211 P( B, C, D, E, F, G, H, A, R(23), 0x76F988DA ); 00212 P( A, B, C, D, E, F, G, H, R(24), 0x983E5152 ); 00213 P( H, A, B, C, D, E, F, G, R(25), 0xA831C66D ); 00214 P( G, H, A, B, C, D, E, F, R(26), 0xB00327C8 ); 00215 P( F, G, H, A, B, C, D, E, R(27), 0xBF597FC7 ); 00216 P( E, F, G, H, A, B, C, D, R(28), 0xC6E00BF3 ); 00217 P( D, E, F, G, H, A, B, C, R(29), 0xD5A79147 ); 00218 P( C, D, E, F, G, H, A, B, R(30), 0x06CA6351 ); 00219 P( B, C, D, E, F, G, H, A, R(31), 0x14292967 ); 00220 P( A, B, C, D, E, F, G, H, R(32), 0x27B70A85 ); 00221 P( H, A, B, C, D, E, F, G, R(33), 0x2E1B2138 ); 00222 P( G, H, A, B, C, D, E, F, R(34), 0x4D2C6DFC ); 00223 P( F, G, H, A, B, C, D, E, R(35), 0x53380D13 ); 00224 P( E, F, G, H, A, B, C, D, R(36), 0x650A7354 ); 00225 P( D, E, F, G, H, A, B, C, R(37), 0x766A0ABB ); 00226 P( C, D, E, F, G, H, A, B, R(38), 0x81C2C92E ); 00227 P( B, C, D, E, F, G, H, A, R(39), 0x92722C85 ); 00228 P( A, B, C, D, E, F, G, H, R(40), 0xA2BFE8A1 ); 00229 P( H, A, B, C, D, E, F, G, R(41), 0xA81A664B ); 00230 P( G, H, A, B, C, D, E, F, R(42), 0xC24B8B70 ); 00231 P( F, G, H, A, B, C, D, E, R(43), 0xC76C51A3 ); 00232 P( E, F, G, H, A, B, C, D, R(44), 0xD192E819 ); 00233 P( D, E, F, G, H, A, B, C, R(45), 0xD6990624 ); 00234 P( C, D, E, F, G, H, A, B, R(46), 0xF40E3585 ); 00235 P( B, C, D, E, F, G, H, A, R(47), 0x106AA070 ); 00236 P( A, B, C, D, E, F, G, H, R(48), 0x19A4C116 ); 00237 P( H, A, B, C, D, E, F, G, R(49), 0x1E376C08 ); 00238 P( G, H, A, B, C, D, E, F, R(50), 0x2748774C ); 00239 P( F, G, H, A, B, C, D, E, R(51), 0x34B0BCB5 ); 00240 P( E, F, G, H, A, B, C, D, R(52), 0x391C0CB3 ); 00241 P( D, E, F, G, H, A, B, C, R(53), 0x4ED8AA4A ); 00242 P( C, D, E, F, G, H, A, B, R(54), 0x5B9CCA4F ); 00243 P( B, C, D, E, F, G, H, A, R(55), 0x682E6FF3 ); 00244 P( A, B, C, D, E, F, G, H, R(56), 0x748F82EE ); 00245 P( H, A, B, C, D, E, F, G, R(57), 0x78A5636F ); 00246 P( G, H, A, B, C, D, E, F, R(58), 0x84C87814 ); 00247 P( F, G, H, A, B, C, D, E, R(59), 0x8CC70208 ); 00248 P( E, F, G, H, A, B, C, D, R(60), 0x90BEFFFA ); 00249 P( D, E, F, G, H, A, B, C, R(61), 0xA4506CEB ); 00250 P( C, D, E, F, G, H, A, B, R(62), 0xBEF9A3F7 ); 00251 P( B, C, D, E, F, G, H, A, R(63), 0xC67178F2 ); 00252 00253 ctx->state [0] += A; 00254 ctx->state [1] += B; 00255 ctx->state [2] += C; 00256 ctx->state [3] += D; 00257 ctx->state [4] += E; 00258 ctx->state [5] += F; 00259 ctx->state [6] += G; 00260 ctx->state [7] += H; 00261 } 00262 00263 /* 00264 * SHA-256 process buffer 00265 */ 00266 void sha256_update( sha256_context *ctx, const unsigned char *input, 00267 size_t ilen ) 00268 { 00269 size_t fill; 00270 uint32_t left; 00271 00272 if( ilen == 0 ) 00273 return; 00274 00275 left = ctx->total [0] & 0x3F; 00276 fill = 64 - left; 00277 00278 ctx->total [0] += (uint32_t) ilen; 00279 ctx->total [0] &= 0xFFFFFFFF; 00280 00281 if( ctx->total [0] < (uint32_t) ilen ) 00282 ctx->total [1]++; 00283 00284 if( left && ilen >= fill ) 00285 { 00286 memcpy( (void *) (ctx->buffer + left), input, fill ); 00287 sha256_process( ctx, ctx->buffer ); 00288 input += fill; 00289 ilen -= fill; 00290 left = 0; 00291 } 00292 00293 while( ilen >= 64 ) 00294 { 00295 sha256_process( ctx, input ); 00296 input += 64; 00297 ilen -= 64; 00298 } 00299 00300 if( ilen > 0 ) 00301 memcpy( (void *) (ctx->buffer + left), input, ilen ); 00302 } 00303 00304 static const unsigned char sha256_padding[64] = 00305 { 00306 0x80, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00307 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00308 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 00309 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 00310 }; 00311 00312 /* 00313 * SHA-256 final digest 00314 */ 00315 void sha256_finish( sha256_context *ctx, unsigned char output[32] ) 00316 { 00317 uint32_t last, padn; 00318 uint32_t high, low; 00319 unsigned char msglen[8]; 00320 00321 high = ( ctx->total [0] >> 29 ) 00322 | ( ctx->total [1] << 3 ); 00323 low = ( ctx->total [0] << 3 ); 00324 00325 PUT_UINT32_BE( high, msglen, 0 ); 00326 PUT_UINT32_BE( low, msglen, 4 ); 00327 00328 last = ctx->total [0] & 0x3F; 00329 padn = ( last < 56 ) ? ( 56 - last ) : ( 120 - last ); 00330 00331 sha256_update( ctx, sha256_padding, padn ); 00332 sha256_update( ctx, msglen, 8 ); 00333 00334 PUT_UINT32_BE( ctx->state [0], output, 0 ); 00335 PUT_UINT32_BE( ctx->state [1], output, 4 ); 00336 PUT_UINT32_BE( ctx->state [2], output, 8 ); 00337 PUT_UINT32_BE( ctx->state [3], output, 12 ); 00338 PUT_UINT32_BE( ctx->state [4], output, 16 ); 00339 PUT_UINT32_BE( ctx->state [5], output, 20 ); 00340 PUT_UINT32_BE( ctx->state [6], output, 24 ); 00341 00342 if( ctx->is224 == 0 ) 00343 PUT_UINT32_BE( ctx->state [7], output, 28 ); 00344 } 00345 00346 #endif /* !POLARSSL_SHA256_ALT */ 00347 00348 /* 00349 * output = SHA-256( input buffer ) 00350 */ 00351 void sha256( const unsigned char *input, size_t ilen, 00352 unsigned char output[32], int is224 ) 00353 { 00354 sha256_context ctx; 00355 00356 sha256_init( &ctx ); 00357 sha256_starts( &ctx, is224 ); 00358 sha256_update( &ctx, input, ilen ); 00359 sha256_finish( &ctx, output ); 00360 sha256_free( &ctx ); 00361 } 00362 00363 #if defined(POLARSSL_FS_IO) 00364 /* 00365 * output = SHA-256( file contents ) 00366 */ 00367 int sha256_file( const char *path, unsigned char output[32], int is224 ) 00368 { 00369 FILE *f; 00370 size_t n; 00371 sha256_context ctx; 00372 unsigned char buf[1024]; 00373 00374 if( ( f = fopen( path, "rb" ) ) == NULL ) 00375 return( POLARSSL_ERR_SHA256_FILE_IO_ERROR ); 00376 00377 sha256_init( &ctx ); 00378 sha256_starts( &ctx, is224 ); 00379 00380 while( ( n = fread( buf, 1, sizeof( buf ), f ) ) > 0 ) 00381 sha256_update( &ctx, buf, n ); 00382 00383 sha256_finish( &ctx, output ); 00384 sha256_free( &ctx ); 00385 00386 if( ferror( f ) != 0 ) 00387 { 00388 fclose( f ); 00389 return( POLARSSL_ERR_SHA256_FILE_IO_ERROR ); 00390 } 00391 00392 fclose( f ); 00393 return( 0 ); 00394 } 00395 #endif /* POLARSSL_FS_IO */ 00396 00397 /* 00398 * SHA-256 HMAC context setup 00399 */ 00400 void sha256_hmac_starts( sha256_context *ctx, const unsigned char *key, 00401 size_t keylen, int is224 ) 00402 { 00403 size_t i; 00404 unsigned char sum[32]; 00405 00406 if( keylen > 64 ) 00407 { 00408 sha256( key, keylen, sum, is224 ); 00409 keylen = ( is224 ) ? 28 : 32; 00410 key = sum; 00411 } 00412 00413 memset( ctx->ipad , 0x36, 64 ); 00414 memset( ctx->opad , 0x5C, 64 ); 00415 00416 for( i = 0; i < keylen; i++ ) 00417 { 00418 ctx->ipad [i] = (unsigned char)( ctx->ipad [i] ^ key[i] ); 00419 ctx->opad [i] = (unsigned char)( ctx->opad [i] ^ key[i] ); 00420 } 00421 00422 sha256_starts( ctx, is224 ); 00423 sha256_update( ctx, ctx->ipad , 64 ); 00424 00425 polarssl_zeroize( sum, sizeof( sum ) ); 00426 } 00427 00428 /* 00429 * SHA-256 HMAC process buffer 00430 */ 00431 void sha256_hmac_update( sha256_context *ctx, const unsigned char *input, 00432 size_t ilen ) 00433 { 00434 sha256_update( ctx, input, ilen ); 00435 } 00436 00437 /* 00438 * SHA-256 HMAC final digest 00439 */ 00440 void sha256_hmac_finish( sha256_context *ctx, unsigned char output[32] ) 00441 { 00442 int is224, hlen; 00443 unsigned char tmpbuf[32]; 00444 00445 is224 = ctx->is224 ; 00446 hlen = ( is224 == 0 ) ? 32 : 28; 00447 00448 sha256_finish( ctx, tmpbuf ); 00449 sha256_starts( ctx, is224 ); 00450 sha256_update( ctx, ctx->opad , 64 ); 00451 sha256_update( ctx, tmpbuf, hlen ); 00452 sha256_finish( ctx, output ); 00453 00454 polarssl_zeroize( tmpbuf, sizeof( tmpbuf ) ); 00455 } 00456 00457 /* 00458 * SHA-256 HMAC context reset 00459 */ 00460 void sha256_hmac_reset( sha256_context *ctx ) 00461 { 00462 sha256_starts( ctx, ctx->is224 ); 00463 sha256_update( ctx, ctx->ipad , 64 ); 00464 } 00465 00466 /* 00467 * output = HMAC-SHA-256( hmac key, input buffer ) 00468 */ 00469 void sha256_hmac( const unsigned char *key, size_t keylen, 00470 const unsigned char *input, size_t ilen, 00471 unsigned char output[32], int is224 ) 00472 { 00473 sha256_context ctx; 00474 00475 sha256_init( &ctx ); 00476 sha256_hmac_starts( &ctx, key, keylen, is224 ); 00477 sha256_hmac_update( &ctx, input, ilen ); 00478 sha256_hmac_finish( &ctx, output ); 00479 sha256_free( &ctx ); 00480 } 00481 00482 #if defined(POLARSSL_SELF_TEST) 00483 /* 00484 * FIPS-180-2 test vectors 00485 */ 00486 static const unsigned char sha256_test_buf[3][57] = 00487 { 00488 { "abc" }, 00489 { "abcdbcdecdefdefgefghfghighijhijkijkljklmklmnlmnomnopnopq" }, 00490 { "" } 00491 }; 00492 00493 static const int sha256_test_buflen[3] = 00494 { 00495 3, 56, 1000 00496 }; 00497 00498 static const unsigned char sha256_test_sum[6][32] = 00499 { 00500 /* 00501 * SHA-224 test vectors 00502 */ 00503 { 0x23, 0x09, 0x7D, 0x22, 0x34, 0x05, 0xD8, 0x22, 00504 0x86, 0x42, 0xA4, 0x77, 0xBD, 0xA2, 0x55, 0xB3, 00505 0x2A, 0xAD, 0xBC, 0xE4, 0xBD, 0xA0, 0xB3, 0xF7, 00506 0xE3, 0x6C, 0x9D, 0xA7 }, 00507 { 0x75, 0x38, 0x8B, 0x16, 0x51, 0x27, 0x76, 0xCC, 00508 0x5D, 0xBA, 0x5D, 0xA1, 0xFD, 0x89, 0x01, 0x50, 00509 0xB0, 0xC6, 0x45, 0x5C, 0xB4, 0xF5, 0x8B, 0x19, 00510 0x52, 0x52, 0x25, 0x25 }, 00511 { 0x20, 0x79, 0x46, 0x55, 0x98, 0x0C, 0x91, 0xD8, 00512 0xBB, 0xB4, 0xC1, 0xEA, 0x97, 0x61, 0x8A, 0x4B, 00513 0xF0, 0x3F, 0x42, 0x58, 0x19, 0x48, 0xB2, 0xEE, 00514 0x4E, 0xE7, 0xAD, 0x67 }, 00515 00516 /* 00517 * SHA-256 test vectors 00518 */ 00519 { 0xBA, 0x78, 0x16, 0xBF, 0x8F, 0x01, 0xCF, 0xEA, 00520 0x41, 0x41, 0x40, 0xDE, 0x5D, 0xAE, 0x22, 0x23, 00521 0xB0, 0x03, 0x61, 0xA3, 0x96, 0x17, 0x7A, 0x9C, 00522 0xB4, 0x10, 0xFF, 0x61, 0xF2, 0x00, 0x15, 0xAD }, 00523 { 0x24, 0x8D, 0x6A, 0x61, 0xD2, 0x06, 0x38, 0xB8, 00524 0xE5, 0xC0, 0x26, 0x93, 0x0C, 0x3E, 0x60, 0x39, 00525 0xA3, 0x3C, 0xE4, 0x59, 0x64, 0xFF, 0x21, 0x67, 00526 0xF6, 0xEC, 0xED, 0xD4, 0x19, 0xDB, 0x06, 0xC1 }, 00527 { 0xCD, 0xC7, 0x6E, 0x5C, 0x99, 0x14, 0xFB, 0x92, 00528 0x81, 0xA1, 0xC7, 0xE2, 0x84, 0xD7, 0x3E, 0x67, 00529 0xF1, 0x80, 0x9A, 0x48, 0xA4, 0x97, 0x20, 0x0E, 00530 0x04, 0x6D, 0x39, 0xCC, 0xC7, 0x11, 0x2C, 0xD0 } 00531 }; 00532 00533 /* 00534 * RFC 4231 test vectors 00535 */ 00536 static const unsigned char sha256_hmac_test_key[7][26] = 00537 { 00538 { "\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B\x0B" 00539 "\x0B\x0B\x0B\x0B" }, 00540 { "Jefe" }, 00541 { "\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA\xAA" 00542 "\xAA\xAA\xAA\xAA" }, 00543 { "\x01\x02\x03\x04\x05\x06\x07\x08\x09\x0A\x0B\x0C\x0D\x0E\x0F\x10" 00544 "\x11\x12\x13\x14\x15\x16\x17\x18\x19" }, 00545 { "\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C\x0C" 00546 "\x0C\x0C\x0C\x0C" }, 00547 { "" }, /* 0xAA 131 times */ 00548 { "" } 00549 }; 00550 00551 static const int sha256_hmac_test_keylen[7] = 00552 { 00553 20, 4, 20, 25, 20, 131, 131 00554 }; 00555 00556 static const unsigned char sha256_hmac_test_buf[7][153] = 00557 { 00558 { "Hi There" }, 00559 { "what do ya want for nothing?" }, 00560 { "\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD" 00561 "\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD" 00562 "\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD" 00563 "\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD" 00564 "\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD\xDD" }, 00565 { "\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD" 00566 "\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD" 00567 "\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD" 00568 "\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD" 00569 "\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD\xCD" }, 00570 { "Test With Truncation" }, 00571 { "Test Using Larger Than Block-Size Key - Hash Key First" }, 00572 { "This is a test using a larger than block-size key " 00573 "and a larger than block-size data. The key needs to " 00574 "be hashed before being used by the HMAC algorithm." } 00575 }; 00576 00577 static const int sha256_hmac_test_buflen[7] = 00578 { 00579 8, 28, 50, 50, 20, 54, 152 00580 }; 00581 00582 static const unsigned char sha256_hmac_test_sum[14][32] = 00583 { 00584 /* 00585 * HMAC-SHA-224 test vectors 00586 */ 00587 { 0x89, 0x6F, 0xB1, 0x12, 0x8A, 0xBB, 0xDF, 0x19, 00588 0x68, 0x32, 0x10, 0x7C, 0xD4, 0x9D, 0xF3, 0x3F, 00589 0x47, 0xB4, 0xB1, 0x16, 0x99, 0x12, 0xBA, 0x4F, 00590 0x53, 0x68, 0x4B, 0x22 }, 00591 { 0xA3, 0x0E, 0x01, 0x09, 0x8B, 0xC6, 0xDB, 0xBF, 00592 0x45, 0x69, 0x0F, 0x3A, 0x7E, 0x9E, 0x6D, 0x0F, 00593 0x8B, 0xBE, 0xA2, 0xA3, 0x9E, 0x61, 0x48, 0x00, 00594 0x8F, 0xD0, 0x5E, 0x44 }, 00595 { 0x7F, 0xB3, 0xCB, 0x35, 0x88, 0xC6, 0xC1, 0xF6, 00596 0xFF, 0xA9, 0x69, 0x4D, 0x7D, 0x6A, 0xD2, 0x64, 00597 0x93, 0x65, 0xB0, 0xC1, 0xF6, 0x5D, 0x69, 0xD1, 00598 0xEC, 0x83, 0x33, 0xEA }, 00599 { 0x6C, 0x11, 0x50, 0x68, 0x74, 0x01, 0x3C, 0xAC, 00600 0x6A, 0x2A, 0xBC, 0x1B, 0xB3, 0x82, 0x62, 0x7C, 00601 0xEC, 0x6A, 0x90, 0xD8, 0x6E, 0xFC, 0x01, 0x2D, 00602 0xE7, 0xAF, 0xEC, 0x5A }, 00603 { 0x0E, 0x2A, 0xEA, 0x68, 0xA9, 0x0C, 0x8D, 0x37, 00604 0xC9, 0x88, 0xBC, 0xDB, 0x9F, 0xCA, 0x6F, 0xA8 }, 00605 { 0x95, 0xE9, 0xA0, 0xDB, 0x96, 0x20, 0x95, 0xAD, 00606 0xAE, 0xBE, 0x9B, 0x2D, 0x6F, 0x0D, 0xBC, 0xE2, 00607 0xD4, 0x99, 0xF1, 0x12, 0xF2, 0xD2, 0xB7, 0x27, 00608 0x3F, 0xA6, 0x87, 0x0E }, 00609 { 0x3A, 0x85, 0x41, 0x66, 0xAC, 0x5D, 0x9F, 0x02, 00610 0x3F, 0x54, 0xD5, 0x17, 0xD0, 0xB3, 0x9D, 0xBD, 00611 0x94, 0x67, 0x70, 0xDB, 0x9C, 0x2B, 0x95, 0xC9, 00612 0xF6, 0xF5, 0x65, 0xD1 }, 00613 00614 /* 00615 * HMAC-SHA-256 test vectors 00616 */ 00617 { 0xB0, 0x34, 0x4C, 0x61, 0xD8, 0xDB, 0x38, 0x53, 00618 0x5C, 0xA8, 0xAF, 0xCE, 0xAF, 0x0B, 0xF1, 0x2B, 00619 0x88, 0x1D, 0xC2, 0x00, 0xC9, 0x83, 0x3D, 0xA7, 00620 0x26, 0xE9, 0x37, 0x6C, 0x2E, 0x32, 0xCF, 0xF7 }, 00621 { 0x5B, 0xDC, 0xC1, 0x46, 0xBF, 0x60, 0x75, 0x4E, 00622 0x6A, 0x04, 0x24, 0x26, 0x08, 0x95, 0x75, 0xC7, 00623 0x5A, 0x00, 0x3F, 0x08, 0x9D, 0x27, 0x39, 0x83, 00624 0x9D, 0xEC, 0x58, 0xB9, 0x64, 0xEC, 0x38, 0x43 }, 00625 { 0x77, 0x3E, 0xA9, 0x1E, 0x36, 0x80, 0x0E, 0x46, 00626 0x85, 0x4D, 0xB8, 0xEB, 0xD0, 0x91, 0x81, 0xA7, 00627 0x29, 0x59, 0x09, 0x8B, 0x3E, 0xF8, 0xC1, 0x22, 00628 0xD9, 0x63, 0x55, 0x14, 0xCE, 0xD5, 0x65, 0xFE }, 00629 { 0x82, 0x55, 0x8A, 0x38, 0x9A, 0x44, 0x3C, 0x0E, 00630 0xA4, 0xCC, 0x81, 0x98, 0x99, 0xF2, 0x08, 0x3A, 00631 0x85, 0xF0, 0xFA, 0xA3, 0xE5, 0x78, 0xF8, 0x07, 00632 0x7A, 0x2E, 0x3F, 0xF4, 0x67, 0x29, 0x66, 0x5B }, 00633 { 0xA3, 0xB6, 0x16, 0x74, 0x73, 0x10, 0x0E, 0xE0, 00634 0x6E, 0x0C, 0x79, 0x6C, 0x29, 0x55, 0x55, 0x2B }, 00635 { 0x60, 0xE4, 0x31, 0x59, 0x1E, 0xE0, 0xB6, 0x7F, 00636 0x0D, 0x8A, 0x26, 0xAA, 0xCB, 0xF5, 0xB7, 0x7F, 00637 0x8E, 0x0B, 0xC6, 0x21, 0x37, 0x28, 0xC5, 0x14, 00638 0x05, 0x46, 0x04, 0x0F, 0x0E, 0xE3, 0x7F, 0x54 }, 00639 { 0x9B, 0x09, 0xFF, 0xA7, 0x1B, 0x94, 0x2F, 0xCB, 00640 0x27, 0x63, 0x5F, 0xBC, 0xD5, 0xB0, 0xE9, 0x44, 00641 0xBF, 0xDC, 0x63, 0x64, 0x4F, 0x07, 0x13, 0x93, 00642 0x8A, 0x7F, 0x51, 0x53, 0x5C, 0x3A, 0x35, 0xE2 } 00643 }; 00644 00645 /* 00646 * Checkup routine 00647 */ 00648 int sha256_self_test( int verbose ) 00649 { 00650 int i, j, k, buflen, ret = 0; 00651 unsigned char buf[1024]; 00652 unsigned char sha256sum[32]; 00653 sha256_context ctx; 00654 00655 sha256_init( &ctx ); 00656 00657 for( i = 0; i < 6; i++ ) 00658 { 00659 j = i % 3; 00660 k = i < 3; 00661 00662 if( verbose != 0 ) 00663 polarssl_printf( " SHA-%d test #%d: ", 256 - k * 32, j + 1 ); 00664 00665 sha256_starts( &ctx, k ); 00666 00667 if( j == 2 ) 00668 { 00669 memset( buf, 'a', buflen = 1000 ); 00670 00671 for( j = 0; j < 1000; j++ ) 00672 sha256_update( &ctx, buf, buflen ); 00673 } 00674 else 00675 sha256_update( &ctx, sha256_test_buf[j], 00676 sha256_test_buflen[j] ); 00677 00678 sha256_finish( &ctx, sha256sum ); 00679 00680 if( memcmp( sha256sum, sha256_test_sum[i], 32 - k * 4 ) != 0 ) 00681 { 00682 if( verbose != 0 ) 00683 polarssl_printf( "failed\n" ); 00684 00685 ret = 1; 00686 goto exit; 00687 } 00688 00689 if( verbose != 0 ) 00690 polarssl_printf( "passed\n" ); 00691 } 00692 00693 if( verbose != 0 ) 00694 polarssl_printf( "\n" ); 00695 00696 for( i = 0; i < 14; i++ ) 00697 { 00698 j = i % 7; 00699 k = i < 7; 00700 00701 if( verbose != 0 ) 00702 polarssl_printf( " HMAC-SHA-%d test #%d: ", 256 - k * 32, j + 1 ); 00703 00704 if( j == 5 || j == 6 ) 00705 { 00706 memset( buf, 0xAA, buflen = 131 ); 00707 sha256_hmac_starts( &ctx, buf, buflen, k ); 00708 } 00709 else 00710 sha256_hmac_starts( &ctx, sha256_hmac_test_key[j], 00711 sha256_hmac_test_keylen[j], k ); 00712 00713 sha256_hmac_update( &ctx, sha256_hmac_test_buf[j], 00714 sha256_hmac_test_buflen[j] ); 00715 00716 sha256_hmac_finish( &ctx, sha256sum ); 00717 00718 buflen = ( j == 4 ) ? 16 : 32 - k * 4; 00719 00720 if( memcmp( sha256sum, sha256_hmac_test_sum[i], buflen ) != 0 ) 00721 { 00722 if( verbose != 0 ) 00723 polarssl_printf( "failed\n" ); 00724 00725 ret = 1; 00726 goto exit; 00727 } 00728 00729 if( verbose != 0 ) 00730 polarssl_printf( "passed\n" ); 00731 } 00732 00733 if( verbose != 0 ) 00734 polarssl_printf( "\n" ); 00735 00736 exit: 00737 sha256_free( &ctx ); 00738 00739 return( ret ); 00740 } 00741 00742 #endif /* POLARSSL_SELF_TEST */ 00743 00744 #endif /* POLARSSL_SHA256_C */ 00745
Generated on Tue Jul 12 2022 13:50:38 by
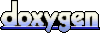