mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
platform.c
00001 /* 00002 * Platform abstraction layer 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 #if !defined(POLARSSL_CONFIG_FILE) 00024 #include "polarssl/config.h" 00025 #else 00026 #include POLARSSL_CONFIG_FILE 00027 #endif 00028 00029 #if defined(POLARSSL_PLATFORM_C) 00030 00031 #include "polarssl/platform.h" 00032 00033 #if defined(POLARSSL_PLATFORM_MEMORY) 00034 #if !defined(POLARSSL_PLATFORM_STD_MALLOC) 00035 static void *platform_malloc_uninit( size_t len ) 00036 { 00037 ((void) len); 00038 return( NULL ); 00039 } 00040 00041 #define POLARSSL_PLATFORM_STD_MALLOC platform_malloc_uninit 00042 #endif /* !POLARSSL_PLATFORM_STD_MALLOC */ 00043 00044 #if !defined(POLARSSL_PLATFORM_STD_FREE) 00045 static void platform_free_uninit( void *ptr ) 00046 { 00047 ((void) ptr); 00048 } 00049 00050 #define POLARSSL_PLATFORM_STD_FREE platform_free_uninit 00051 #endif /* !POLARSSL_PLATFORM_STD_FREE */ 00052 00053 void * (*polarssl_malloc)( size_t ) = POLARSSL_PLATFORM_STD_MALLOC; 00054 void (*polarssl_free)( void * ) = POLARSSL_PLATFORM_STD_FREE; 00055 00056 int platform_set_malloc_free( void * (*malloc_func)( size_t ), 00057 void (*free_func)( void * ) ) 00058 { 00059 polarssl_malloc = malloc_func; 00060 polarssl_free = free_func; 00061 return( 0 ); 00062 } 00063 #endif /* POLARSSL_PLATFORM_MEMORY */ 00064 00065 #if defined(POLARSSL_PLATFORM_SNPRINTF_ALT) 00066 #if !defined(POLARSSL_PLATFORM_STD_SNPRINTF) 00067 /* 00068 * Make dummy function to prevent NULL pointer dereferences 00069 */ 00070 static int platform_snprintf_uninit( char * s, size_t n, 00071 const char * format, ... ) 00072 { 00073 ((void) s); 00074 ((void) n); 00075 ((void) format); 00076 return( 0 ); 00077 } 00078 00079 #define POLARSSL_PLATFORM_STD_SNPRINTF platform_snprintf_uninit 00080 #endif /* !POLARSSL_PLATFORM_STD_SNPRINTF */ 00081 00082 int (*polarssl_snprintf)( char * s, size_t n, 00083 const char * format, 00084 ... ) = POLARSSL_PLATFORM_STD_SNPRINTF; 00085 00086 int platform_set_snprintf( int (*snprintf_func)( char * s, size_t n, 00087 const char * format, 00088 ... ) ) 00089 { 00090 polarssl_snprintf = snprintf_func; 00091 return( 0 ); 00092 } 00093 #endif /* POLARSSL_PLATFORM_SNPRINTF_ALT */ 00094 00095 #if defined(POLARSSL_PLATFORM_PRINTF_ALT) 00096 #if !defined(POLARSSL_PLATFORM_STD_PRINTF) 00097 /* 00098 * Make dummy function to prevent NULL pointer dereferences 00099 */ 00100 static int platform_printf_uninit( const char *format, ... ) 00101 { 00102 ((void) format); 00103 return( 0 ); 00104 } 00105 00106 #define POLARSSL_PLATFORM_STD_PRINTF platform_printf_uninit 00107 #endif /* !POLARSSL_PLATFORM_STD_PRINTF */ 00108 00109 int (*polarssl_printf)( const char *, ... ) = POLARSSL_PLATFORM_STD_PRINTF; 00110 00111 int platform_set_printf( int (*printf_func)( const char *, ... ) ) 00112 { 00113 polarssl_printf = printf_func; 00114 return( 0 ); 00115 } 00116 #endif /* POLARSSL_PLATFORM_PRINTF_ALT */ 00117 00118 #if defined(POLARSSL_PLATFORM_FPRINTF_ALT) 00119 #if !defined(POLARSSL_PLATFORM_STD_FPRINTF) 00120 /* 00121 * Make dummy function to prevent NULL pointer dereferences 00122 */ 00123 static int platform_fprintf_uninit( FILE *stream, const char *format, ... ) 00124 { 00125 ((void) stream); 00126 ((void) format); 00127 return( 0 ); 00128 } 00129 00130 #define POLARSSL_PLATFORM_STD_FPRINTF platform_fprintf_uninit 00131 #endif /* !POLARSSL_PLATFORM_STD_FPRINTF */ 00132 00133 int (*polarssl_fprintf)( FILE *, const char *, ... ) = 00134 POLARSSL_PLATFORM_STD_FPRINTF; 00135 00136 int platform_set_fprintf( int (*fprintf_func)( FILE *, const char *, ... ) ) 00137 { 00138 polarssl_fprintf = fprintf_func; 00139 return( 0 ); 00140 } 00141 #endif /* POLARSSL_PLATFORM_FPRINTF_ALT */ 00142 00143 #if defined(POLARSSL_PLATFORM_EXIT_ALT) 00144 #if !defined(POLARSSL_PLATFORM_STD_EXIT) 00145 /* 00146 * Make dummy function to prevent NULL pointer dereferences 00147 */ 00148 static void platform_exit_uninit( int status ) 00149 { 00150 ((void) status); 00151 } 00152 00153 #define POLARSSL_PLATFORM_STD_EXIT platform_exit_uninit 00154 #endif /* !POLARSSL_PLATFORM_STD_EXIT */ 00155 00156 void (*polarssl_exit)( int status ) = POLARSSL_PLATFORM_STD_EXIT; 00157 00158 int platform_set_exit( void (*exit_func)( int status ) ) 00159 { 00160 polarssl_exit = exit_func; 00161 return( 0 ); 00162 } 00163 #endif /* POLARSSL_PLATFORM_EXIT_ALT */ 00164 00165 #endif /* POLARSSL_PLATFORM_C */ 00166
Generated on Tue Jul 12 2022 13:50:38 by
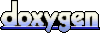