mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pkcs5.h
00001 /** 00002 * \file pkcs5.h 00003 * 00004 * \brief PKCS#5 functions 00005 * 00006 * \author Mathias Olsson <mathias@kompetensum.com> 00007 * 00008 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 #ifndef POLARSSL_PKCS5_H 00027 #define POLARSSL_PKCS5_H 00028 00029 #include "asn1.h" 00030 #include "md.h" 00031 00032 #include <stddef.h> 00033 00034 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00035 #include <basetsd.h> 00036 typedef UINT32 uint32_t; 00037 #else 00038 #include <inttypes.h> 00039 #endif 00040 00041 #define POLARSSL_ERR_PKCS5_BAD_INPUT_DATA -0x3f80 /**< Bad input parameters to function. */ 00042 #define POLARSSL_ERR_PKCS5_INVALID_FORMAT -0x3f00 /**< Unexpected ASN.1 data. */ 00043 #define POLARSSL_ERR_PKCS5_FEATURE_UNAVAILABLE -0x3e80 /**< Requested encryption or digest alg not available. */ 00044 #define POLARSSL_ERR_PKCS5_PASSWORD_MISMATCH -0x3e00 /**< Given private key password does not allow for correct decryption. */ 00045 00046 #define PKCS5_DECRYPT 0 00047 #define PKCS5_ENCRYPT 1 00048 00049 #ifdef __cplusplus 00050 extern "C" { 00051 #endif 00052 00053 /** 00054 * \brief PKCS#5 PBES2 function 00055 * 00056 * \param pbe_params the ASN.1 algorithm parameters 00057 * \param mode either PKCS5_DECRYPT or PKCS5_ENCRYPT 00058 * \param pwd password to use when generating key 00059 * \param pwdlen length of password 00060 * \param data data to process 00061 * \param datalen length of data 00062 * \param output output buffer 00063 * 00064 * \returns 0 on success, or a POLARSSL_ERR_xxx code if verification fails. 00065 */ 00066 int pkcs5_pbes2( asn1_buf *pbe_params, int mode, 00067 const unsigned char *pwd, size_t pwdlen, 00068 const unsigned char *data, size_t datalen, 00069 unsigned char *output ); 00070 00071 /** 00072 * \brief PKCS#5 PBKDF2 using HMAC 00073 * 00074 * \param ctx Generic HMAC context 00075 * \param password Password to use when generating key 00076 * \param plen Length of password 00077 * \param salt Salt to use when generating key 00078 * \param slen Length of salt 00079 * \param iteration_count Iteration count 00080 * \param key_length Length of generated key 00081 * \param output Generated key. Must be at least as big as key_length 00082 * 00083 * \returns 0 on success, or a POLARSSL_ERR_xxx code if verification fails. 00084 */ 00085 int pkcs5_pbkdf2_hmac( md_context_t *ctx, const unsigned char *password, 00086 size_t plen, const unsigned char *salt, size_t slen, 00087 unsigned int iteration_count, 00088 uint32_t key_length, unsigned char *output ); 00089 00090 /** 00091 * \brief Checkup routine 00092 * 00093 * \return 0 if successful, or 1 if the test failed 00094 */ 00095 int pkcs5_self_test( int verbose ); 00096 00097 #ifdef __cplusplus 00098 } 00099 #endif 00100 00101 #endif /* pkcs5.h */ 00102
Generated on Tue Jul 12 2022 13:50:37 by
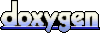