mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pkcs12.h
00001 /** 00002 * \file pkcs12.h 00003 * 00004 * \brief PKCS#12 Personal Information Exchange Syntax 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_PKCS12_H 00025 #define POLARSSL_PKCS12_H 00026 00027 #include "md.h" 00028 #include "cipher.h" 00029 #include "asn1.h" 00030 00031 #include <stddef.h> 00032 00033 #define POLARSSL_ERR_PKCS12_BAD_INPUT_DATA -0x1F80 /**< Bad input parameters to function. */ 00034 #define POLARSSL_ERR_PKCS12_FEATURE_UNAVAILABLE -0x1F00 /**< Feature not available, e.g. unsupported encryption scheme. */ 00035 #define POLARSSL_ERR_PKCS12_PBE_INVALID_FORMAT -0x1E80 /**< PBE ASN.1 data not as expected. */ 00036 #define POLARSSL_ERR_PKCS12_PASSWORD_MISMATCH -0x1E00 /**< Given private key password does not allow for correct decryption. */ 00037 00038 #define PKCS12_DERIVE_KEY 1 /**< encryption/decryption key */ 00039 #define PKCS12_DERIVE_IV 2 /**< initialization vector */ 00040 #define PKCS12_DERIVE_MAC_KEY 3 /**< integrity / MAC key */ 00041 00042 #define PKCS12_PBE_DECRYPT 0 00043 #define PKCS12_PBE_ENCRYPT 1 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** 00050 * \brief PKCS12 Password Based function (encryption / decryption) 00051 * for pbeWithSHAAnd128BitRC4 00052 * 00053 * \param pbe_params an ASN1 buffer containing the pkcs-12PbeParams structure 00054 * \param mode either PKCS12_PBE_ENCRYPT or PKCS12_PBE_DECRYPT 00055 * \param pwd the password used (may be NULL if no password is used) 00056 * \param pwdlen length of the password (may be 0) 00057 * \param input the input data 00058 * \param len data length 00059 * \param output the output buffer 00060 * 00061 * \return 0 if successful, or a POLARSSL_ERR_xxx code 00062 */ 00063 int pkcs12_pbe_sha1_rc4_128( asn1_buf *pbe_params, int mode, 00064 const unsigned char *pwd, size_t pwdlen, 00065 const unsigned char *input, size_t len, 00066 unsigned char *output ); 00067 00068 /** 00069 * \brief PKCS12 Password Based function (encryption / decryption) 00070 * for cipher-based and md-based PBE's 00071 * 00072 * \param pbe_params an ASN1 buffer containing the pkcs-12PbeParams structure 00073 * \param mode either PKCS12_PBE_ENCRYPT or PKCS12_PBE_DECRYPT 00074 * \param cipher_type the cipher used 00075 * \param md_type the md used 00076 * \param pwd the password used (may be NULL if no password is used) 00077 * \param pwdlen length of the password (may be 0) 00078 * \param input the input data 00079 * \param len data length 00080 * \param output the output buffer 00081 * 00082 * \return 0 if successful, or a POLARSSL_ERR_xxx code 00083 */ 00084 int pkcs12_pbe( asn1_buf *pbe_params, int mode, 00085 cipher_type_t cipher_type, md_type_t md_type, 00086 const unsigned char *pwd, size_t pwdlen, 00087 const unsigned char *input, size_t len, 00088 unsigned char *output ); 00089 00090 /** 00091 * \brief The PKCS#12 derivation function uses a password and a salt 00092 * to produce pseudo-random bits for a particular "purpose". 00093 * 00094 * Depending on the given id, this function can produce an 00095 * encryption/decryption key, an nitialization vector or an 00096 * integrity key. 00097 * 00098 * \param data buffer to store the derived data in 00099 * \param datalen length to fill 00100 * \param pwd password to use (may be NULL if no password is used) 00101 * \param pwdlen length of the password (may be 0) 00102 * \param salt salt buffer to use 00103 * \param saltlen length of the salt 00104 * \param md md type to use during the derivation 00105 * \param id id that describes the purpose (can be PKCS12_DERIVE_KEY, 00106 * PKCS12_DERIVE_IV or PKCS12_DERIVE_MAC_KEY) 00107 * \param iterations number of iterations 00108 * 00109 * \return 0 if successful, or a MD, BIGNUM type error. 00110 */ 00111 int pkcs12_derivation( unsigned char *data, size_t datalen, 00112 const unsigned char *pwd, size_t pwdlen, 00113 const unsigned char *salt, size_t saltlen, 00114 md_type_t md, int id, int iterations ); 00115 00116 #ifdef __cplusplus 00117 } 00118 #endif 00119 00120 #endif /* pkcs12.h */ 00121
Generated on Tue Jul 12 2022 13:50:37 by
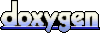