mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pkcs11.h
00001 /** 00002 * \file pkcs11.h 00003 * 00004 * \brief Wrapper for PKCS#11 library libpkcs11-helper 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 #ifndef POLARSSL_PKCS11_H 00027 #define POLARSSL_PKCS11_H 00028 00029 #if !defined(POLARSSL_CONFIG_FILE) 00030 #include "config.h" 00031 #else 00032 #include POLARSSL_CONFIG_FILE 00033 #endif 00034 00035 #if defined(POLARSSL_PKCS11_C) 00036 00037 #include "x509_crt.h" 00038 00039 #include <pkcs11-helper-1.0/pkcs11h-certificate.h> 00040 00041 #if defined(_MSC_VER) && !defined(inline) 00042 #define inline _inline 00043 #else 00044 #if defined(__ARMCC_VERSION) && !defined(inline) 00045 #define inline __inline 00046 #endif /* __ARMCC_VERSION */ 00047 #endif /*_MSC_VER */ 00048 00049 #ifdef __cplusplus 00050 extern "C" { 00051 #endif 00052 00053 /** 00054 * Context for PKCS #11 private keys. 00055 */ 00056 typedef struct { 00057 pkcs11h_certificate_t pkcs11h_cert; 00058 int len; 00059 } pkcs11_context; 00060 00061 /** 00062 * Fill in a mbed TLS certificate, based on the given PKCS11 helper certificate. 00063 * 00064 * \param cert X.509 certificate to fill 00065 * \param pkcs11h_cert PKCS #11 helper certificate 00066 * 00067 * \return 0 on success. 00068 */ 00069 int pkcs11_x509_cert_init( x509_crt *cert, pkcs11h_certificate_t pkcs11h_cert ); 00070 00071 /** 00072 * Initialise a pkcs11_context, storing the given certificate. Note that the 00073 * pkcs11_context will take over control of the certificate, freeing it when 00074 * done. 00075 * 00076 * \param priv_key Private key structure to fill. 00077 * \param pkcs11_cert PKCS #11 helper certificate 00078 * 00079 * \return 0 on success 00080 */ 00081 int pkcs11_priv_key_init( pkcs11_context *priv_key, 00082 pkcs11h_certificate_t pkcs11_cert ); 00083 00084 /** 00085 * Free the contents of the given private key context. Note that the structure 00086 * itself is not freed. 00087 * 00088 * \param priv_key Private key structure to cleanup 00089 */ 00090 void pkcs11_priv_key_free( pkcs11_context *priv_key ); 00091 00092 /** 00093 * \brief Do an RSA private key decrypt, then remove the message 00094 * padding 00095 * 00096 * \param ctx PKCS #11 context 00097 * \param mode must be RSA_PRIVATE, for compatibility with rsa.c's signature 00098 * \param input buffer holding the encrypted data 00099 * \param output buffer that will hold the plaintext 00100 * \param olen will contain the plaintext length 00101 * \param output_max_len maximum length of the output buffer 00102 * 00103 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00104 * 00105 * \note The output buffer must be as large as the size 00106 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00107 * an error is thrown. 00108 */ 00109 int pkcs11_decrypt( pkcs11_context *ctx, 00110 int mode, size_t *olen, 00111 const unsigned char *input, 00112 unsigned char *output, 00113 size_t output_max_len ); 00114 00115 /** 00116 * \brief Do a private RSA to sign a message digest 00117 * 00118 * \param ctx PKCS #11 context 00119 * \param mode must be RSA_PRIVATE, for compatibility with rsa.c's signature 00120 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00121 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00122 * \param hash buffer holding the message digest 00123 * \param sig buffer that will hold the ciphertext 00124 * 00125 * \return 0 if the signing operation was successful, 00126 * or an POLARSSL_ERR_RSA_XXX error code 00127 * 00128 * \note The "sig" buffer must be as large as the size 00129 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00130 */ 00131 int pkcs11_sign( pkcs11_context *ctx, 00132 int mode, 00133 md_type_t md_alg, 00134 unsigned int hashlen, 00135 const unsigned char *hash, 00136 unsigned char *sig ); 00137 00138 /** 00139 * SSL/TLS wrappers for PKCS#11 functions 00140 */ 00141 static inline int ssl_pkcs11_decrypt( void *ctx, int mode, size_t *olen, 00142 const unsigned char *input, unsigned char *output, 00143 size_t output_max_len ) 00144 { 00145 return pkcs11_decrypt( (pkcs11_context *) ctx, mode, olen, input, output, 00146 output_max_len ); 00147 } 00148 00149 static inline int ssl_pkcs11_sign( void *ctx, 00150 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00151 int mode, md_type_t md_alg, unsigned int hashlen, 00152 const unsigned char *hash, unsigned char *sig ) 00153 { 00154 ((void) f_rng); 00155 ((void) p_rng); 00156 return pkcs11_sign( (pkcs11_context *) ctx, mode, md_alg, 00157 hashlen, hash, sig ); 00158 } 00159 00160 static inline size_t ssl_pkcs11_key_len( void *ctx ) 00161 { 00162 return ( (pkcs11_context *) ctx )->len; 00163 } 00164 00165 #ifdef __cplusplus 00166 } 00167 #endif 00168 00169 #endif /* POLARSSL_PKCS11_C */ 00170 00171 #endif /* POLARSSL_PKCS11_H */ 00172
Generated on Tue Jul 12 2022 13:50:37 by
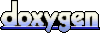