mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pem.h
00001 /** 00002 * \file pem.h 00003 * 00004 * \brief Privacy Enhanced Mail (PEM) decoding 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_PEM_H 00025 #define POLARSSL_PEM_H 00026 00027 #include <stddef.h> 00028 00029 /** 00030 * \name PEM Error codes 00031 * These error codes are returned in case of errors reading the 00032 * PEM data. 00033 * \{ 00034 */ 00035 #define POLARSSL_ERR_PEM_NO_HEADER_FOOTER_PRESENT -0x1080 /**< No PEM header or footer found. */ 00036 #define POLARSSL_ERR_PEM_INVALID_DATA -0x1100 /**< PEM string is not as expected. */ 00037 #define POLARSSL_ERR_PEM_MALLOC_FAILED -0x1180 /**< Failed to allocate memory. */ 00038 #define POLARSSL_ERR_PEM_INVALID_ENC_IV -0x1200 /**< RSA IV is not in hex-format. */ 00039 #define POLARSSL_ERR_PEM_UNKNOWN_ENC_ALG -0x1280 /**< Unsupported key encryption algorithm. */ 00040 #define POLARSSL_ERR_PEM_PASSWORD_REQUIRED -0x1300 /**< Private key password can't be empty. */ 00041 #define POLARSSL_ERR_PEM_PASSWORD_MISMATCH -0x1380 /**< Given private key password does not allow for correct decryption. */ 00042 #define POLARSSL_ERR_PEM_FEATURE_UNAVAILABLE -0x1400 /**< Unavailable feature, e.g. hashing/encryption combination. */ 00043 #define POLARSSL_ERR_PEM_BAD_INPUT_DATA -0x1480 /**< Bad input parameters to function. */ 00044 /* \} name */ 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 #if defined(POLARSSL_PEM_PARSE_C) 00051 /** 00052 * \brief PEM context structure 00053 */ 00054 typedef struct 00055 { 00056 unsigned char *buf ; /*!< buffer for decoded data */ 00057 size_t buflen ; /*!< length of the buffer */ 00058 unsigned char *info ; /*!< buffer for extra header information */ 00059 } 00060 pem_context; 00061 00062 /** 00063 * \brief PEM context setup 00064 * 00065 * \param ctx context to be initialized 00066 */ 00067 void pem_init( pem_context *ctx ); 00068 00069 /** 00070 * \brief Read a buffer for PEM information and store the resulting 00071 * data into the specified context buffers. 00072 * 00073 * \param ctx context to use 00074 * \param header header string to seek and expect 00075 * \param footer footer string to seek and expect 00076 * \param data source data to look in 00077 * \param pwd password for decryption (can be NULL) 00078 * \param pwdlen length of password 00079 * \param use_len destination for total length used (set after header is 00080 * correctly read, so unless you get 00081 * POLARSSL_ERR_PEM_BAD_INPUT_DATA or 00082 * POLARSSL_ERR_PEM_NO_HEADER_FOOTER_PRESENT, use_len is 00083 * the length to skip) 00084 * 00085 * \note Attempts to check password correctness by verifying if 00086 * the decrypted text starts with an ASN.1 sequence of 00087 * appropriate length 00088 * 00089 * \return 0 on success, or a specific PEM error code 00090 */ 00091 int pem_read_buffer( pem_context *ctx, const char *header, const char *footer, 00092 const unsigned char *data, 00093 const unsigned char *pwd, 00094 size_t pwdlen, size_t *use_len ); 00095 00096 /** 00097 * \brief PEM context memory freeing 00098 * 00099 * \param ctx context to be freed 00100 */ 00101 void pem_free( pem_context *ctx ); 00102 #endif /* POLARSSL_PEM_PARSE_C */ 00103 00104 #if defined(POLARSSL_PEM_WRITE_C) 00105 /** 00106 * \brief Write a buffer of PEM information from a DER encoded 00107 * buffer. 00108 * 00109 * \param header header string to write 00110 * \param footer footer string to write 00111 * \param der_data DER data to write 00112 * \param der_len length of the DER data 00113 * \param buf buffer to write to 00114 * \param buf_len length of output buffer 00115 * \param olen total length written / required (if buf_len is not enough) 00116 * 00117 * \return 0 on success, or a specific PEM or BASE64 error code. On 00118 * POLARSSL_ERR_BASE64_BUFFER_TOO_SMALL olen is the required 00119 * size. 00120 */ 00121 int pem_write_buffer( const char *header, const char *footer, 00122 const unsigned char *der_data, size_t der_len, 00123 unsigned char *buf, size_t buf_len, size_t *olen ); 00124 #endif /* POLARSSL_PEM_WRITE_C */ 00125 00126 #ifdef __cplusplus 00127 } 00128 #endif 00129 00130 #endif /* pem.h */ 00131
Generated on Tue Jul 12 2022 13:50:37 by
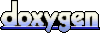