mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
padlock.c
00001 /* 00002 * VIA PadLock support functions 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 /* 00023 * This implementation is based on the VIA PadLock Programming Guide: 00024 * 00025 * http://www.via.com.tw/en/downloads/whitepapers/initiatives/padlock/ 00026 * programming_guide.pdf 00027 */ 00028 00029 #if !defined(POLARSSL_CONFIG_FILE) 00030 #include "polarssl/config.h" 00031 #else 00032 #include POLARSSL_CONFIG_FILE 00033 #endif 00034 00035 #if defined(POLARSSL_PADLOCK_C) 00036 00037 #include "polarssl/padlock.h" 00038 00039 #include <string.h> 00040 00041 #if defined(POLARSSL_HAVE_X86) 00042 00043 /* 00044 * PadLock detection routine 00045 */ 00046 int padlock_supports( int feature ) 00047 { 00048 static int flags = -1; 00049 int ebx = 0, edx = 0; 00050 00051 if( flags == -1 ) 00052 { 00053 asm( "movl %%ebx, %0 \n\t" 00054 "movl $0xC0000000, %%eax \n\t" 00055 "cpuid \n\t" 00056 "cmpl $0xC0000001, %%eax \n\t" 00057 "movl $0, %%edx \n\t" 00058 "jb unsupported \n\t" 00059 "movl $0xC0000001, %%eax \n\t" 00060 "cpuid \n\t" 00061 "unsupported: \n\t" 00062 "movl %%edx, %1 \n\t" 00063 "movl %2, %%ebx \n\t" 00064 : "=m" (ebx), "=m" (edx) 00065 : "m" (ebx) 00066 : "eax", "ecx", "edx" ); 00067 00068 flags = edx; 00069 } 00070 00071 return( flags & feature ); 00072 } 00073 00074 /* 00075 * PadLock AES-ECB block en(de)cryption 00076 */ 00077 int padlock_xcryptecb( aes_context *ctx, 00078 int mode, 00079 const unsigned char input[16], 00080 unsigned char output[16] ) 00081 { 00082 int ebx = 0; 00083 uint32_t *rk; 00084 uint32_t *blk; 00085 uint32_t *ctrl; 00086 unsigned char buf[256]; 00087 00088 rk = ctx->rk ; 00089 blk = PADLOCK_ALIGN16( buf ); 00090 memcpy( blk, input, 16 ); 00091 00092 ctrl = blk + 4; 00093 *ctrl = 0x80 | ctx->nr | ( ( ctx->nr + ( mode^1 ) - 10 ) << 9 ); 00094 00095 asm( "pushfl \n\t" 00096 "popfl \n\t" 00097 "movl %%ebx, %0 \n\t" 00098 "movl $1, %%ecx \n\t" 00099 "movl %2, %%edx \n\t" 00100 "movl %3, %%ebx \n\t" 00101 "movl %4, %%esi \n\t" 00102 "movl %4, %%edi \n\t" 00103 ".byte 0xf3,0x0f,0xa7,0xc8 \n\t" 00104 "movl %1, %%ebx \n\t" 00105 : "=m" (ebx) 00106 : "m" (ebx), "m" (ctrl), "m" (rk), "m" (blk) 00107 : "memory", "ecx", "edx", "esi", "edi" ); 00108 00109 memcpy( output, blk, 16 ); 00110 00111 return( 0 ); 00112 } 00113 00114 /* 00115 * PadLock AES-CBC buffer en(de)cryption 00116 */ 00117 int padlock_xcryptcbc( aes_context *ctx, 00118 int mode, 00119 size_t length, 00120 unsigned char iv[16], 00121 const unsigned char *input, 00122 unsigned char *output ) 00123 { 00124 int ebx = 0; 00125 size_t count; 00126 uint32_t *rk; 00127 uint32_t *iw; 00128 uint32_t *ctrl; 00129 unsigned char buf[256]; 00130 00131 if( ( (long) input & 15 ) != 0 || 00132 ( (long) output & 15 ) != 0 ) 00133 return( POLARSSL_ERR_PADLOCK_DATA_MISALIGNED ); 00134 00135 rk = ctx->rk ; 00136 iw = PADLOCK_ALIGN16( buf ); 00137 memcpy( iw, iv, 16 ); 00138 00139 ctrl = iw + 4; 00140 *ctrl = 0x80 | ctx->nr | ( ( ctx->nr + ( mode ^ 1 ) - 10 ) << 9 ); 00141 00142 count = ( length + 15 ) >> 4; 00143 00144 asm( "pushfl \n\t" 00145 "popfl \n\t" 00146 "movl %%ebx, %0 \n\t" 00147 "movl %2, %%ecx \n\t" 00148 "movl %3, %%edx \n\t" 00149 "movl %4, %%ebx \n\t" 00150 "movl %5, %%esi \n\t" 00151 "movl %6, %%edi \n\t" 00152 "movl %7, %%eax \n\t" 00153 ".byte 0xf3,0x0f,0xa7,0xd0 \n\t" 00154 "movl %1, %%ebx \n\t" 00155 : "=m" (ebx) 00156 : "m" (ebx), "m" (count), "m" (ctrl), 00157 "m" (rk), "m" (input), "m" (output), "m" (iw) 00158 : "memory", "eax", "ecx", "edx", "esi", "edi" ); 00159 00160 memcpy( iv, iw, 16 ); 00161 00162 return( 0 ); 00163 } 00164 00165 #endif /* POLARSSL_HAVE_X86 */ 00166 00167 #endif /* POLARSSL_PADLOCK_C */ 00168
Generated on Tue Jul 12 2022 13:50:37 by
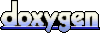