mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
oid.h
00001 /** 00002 * \file oid.h 00003 * 00004 * \brief Object Identifier (OID) database 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_OID_H 00025 #define POLARSSL_OID_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include "asn1.h" 00034 #include "pk.h" 00035 00036 #include <stddef.h> 00037 00038 #if defined(POLARSSL_CIPHER_C) 00039 #include "cipher.h" 00040 #endif 00041 00042 #if defined(POLARSSL_MD_C) 00043 #include "md.h" 00044 #endif 00045 00046 #if defined(POLARSSL_X509_USE_C) || defined(POLARSSL_X509_CREATE_C) 00047 #include "x509.h" 00048 #endif 00049 00050 #define POLARSSL_ERR_OID_NOT_FOUND -0x002E /**< OID is not found. */ 00051 #define POLARSSL_ERR_OID_BUF_TOO_SMALL -0x000B /**< output buffer is too small */ 00052 00053 /* 00054 * Top level OID tuples 00055 */ 00056 #define OID_ISO_MEMBER_BODIES "\x2a" /* {iso(1) member-body(2)} */ 00057 #define OID_ISO_IDENTIFIED_ORG "\x2b" /* {iso(1) identified-organization(3)} */ 00058 #define OID_ISO_CCITT_DS "\x55" /* {joint-iso-ccitt(2) ds(5)} */ 00059 #define OID_ISO_ITU_COUNTRY "\x60" /* {joint-iso-itu-t(2) country(16)} */ 00060 00061 /* 00062 * ISO Member bodies OID parts 00063 */ 00064 #define OID_COUNTRY_US "\x86\x48" /* {us(840)} */ 00065 #define OID_ORG_RSA_DATA_SECURITY "\x86\xf7\x0d" /* {rsadsi(113549)} */ 00066 #define OID_RSA_COMPANY OID_ISO_MEMBER_BODIES OID_COUNTRY_US \ 00067 OID_ORG_RSA_DATA_SECURITY /* {iso(1) member-body(2) us(840) rsadsi(113549)} */ 00068 #define OID_ORG_ANSI_X9_62 "\xce\x3d" /* ansi-X9-62(10045) */ 00069 #define OID_ANSI_X9_62 OID_ISO_MEMBER_BODIES OID_COUNTRY_US \ 00070 OID_ORG_ANSI_X9_62 00071 00072 /* 00073 * ISO Identified organization OID parts 00074 */ 00075 #define OID_ORG_DOD "\x06" /* {dod(6)} */ 00076 #define OID_ORG_OIW "\x0e" 00077 #define OID_OIW_SECSIG OID_ORG_OIW "\x03" 00078 #define OID_OIW_SECSIG_ALG OID_OIW_SECSIG "\x02" 00079 #define OID_OIW_SECSIG_SHA1 OID_OIW_SECSIG_ALG "\x1a" 00080 #define OID_ORG_CERTICOM "\x81\x04" /* certicom(132) */ 00081 #define OID_CERTICOM OID_ISO_IDENTIFIED_ORG OID_ORG_CERTICOM 00082 #define OID_ORG_TELETRUST "\x24" /* teletrust(36) */ 00083 #define OID_TELETRUST OID_ISO_IDENTIFIED_ORG OID_ORG_TELETRUST 00084 00085 /* 00086 * ISO ITU OID parts 00087 */ 00088 #define OID_ORGANIZATION "\x01" /* {organization(1)} */ 00089 #define OID_ISO_ITU_US_ORG OID_ISO_ITU_COUNTRY OID_COUNTRY_US OID_ORGANIZATION /* {joint-iso-itu-t(2) country(16) us(840) organization(1)} */ 00090 00091 #define OID_ORG_GOV "\x65" /* {gov(101)} */ 00092 #define OID_GOV OID_ISO_ITU_US_ORG OID_ORG_GOV /* {joint-iso-itu-t(2) country(16) us(840) organization(1) gov(101)} */ 00093 00094 #define OID_ORG_NETSCAPE "\x86\xF8\x42" /* {netscape(113730)} */ 00095 #define OID_NETSCAPE OID_ISO_ITU_US_ORG OID_ORG_NETSCAPE /* Netscape OID {joint-iso-itu-t(2) country(16) us(840) organization(1) netscape(113730)} */ 00096 00097 /* ISO arc for standard certificate and CRL extensions */ 00098 #define OID_ID_CE OID_ISO_CCITT_DS "\x1D" /**< id-ce OBJECT IDENTIFIER ::= {joint-iso-ccitt(2) ds(5) 29} */ 00099 00100 /** 00101 * Private Internet Extensions 00102 * { iso(1) identified-organization(3) dod(6) internet(1) 00103 * security(5) mechanisms(5) pkix(7) } 00104 */ 00105 #define OID_PKIX OID_ISO_IDENTIFIED_ORG OID_ORG_DOD "\x01\x05\x05\x07" 00106 00107 /* 00108 * Arc for standard naming attributes 00109 */ 00110 #define OID_AT OID_ISO_CCITT_DS "\x04" /**< id-at OBJECT IDENTIFIER ::= {joint-iso-ccitt(2) ds(5) 4} */ 00111 #define OID_AT_CN OID_AT "\x03" /**< id-at-commonName AttributeType:= {id-at 3} */ 00112 #define OID_AT_SUR_NAME OID_AT "\x04" /**< id-at-surName AttributeType:= {id-at 4} */ 00113 #define OID_AT_SERIAL_NUMBER OID_AT "\x05" /**< id-at-serialNumber AttributeType:= {id-at 5} */ 00114 #define OID_AT_COUNTRY OID_AT "\x06" /**< id-at-countryName AttributeType:= {id-at 6} */ 00115 #define OID_AT_LOCALITY OID_AT "\x07" /**< id-at-locality AttributeType:= {id-at 7} */ 00116 #define OID_AT_STATE OID_AT "\x08" /**< id-at-state AttributeType:= {id-at 8} */ 00117 #define OID_AT_ORGANIZATION OID_AT "\x0A" /**< id-at-organizationName AttributeType:= {id-at 10} */ 00118 #define OID_AT_ORG_UNIT OID_AT "\x0B" /**< id-at-organizationalUnitName AttributeType:= {id-at 11} */ 00119 #define OID_AT_TITLE OID_AT "\x0C" /**< id-at-title AttributeType:= {id-at 12} */ 00120 #define OID_AT_POSTAL_ADDRESS OID_AT "\x10" /**< id-at-postalAddress AttributeType:= {id-at 16} */ 00121 #define OID_AT_POSTAL_CODE OID_AT "\x11" /**< id-at-postalCode AttributeType:= {id-at 17} */ 00122 #define OID_AT_GIVEN_NAME OID_AT "\x2A" /**< id-at-givenName AttributeType:= {id-at 42} */ 00123 #define OID_AT_INITIALS OID_AT "\x2B" /**< id-at-initials AttributeType:= {id-at 43} */ 00124 #define OID_AT_GENERATION_QUALIFIER OID_AT "\x2C" /**< id-at-generationQualifier AttributeType:= {id-at 44} */ 00125 #define OID_AT_UNIQUE_IDENTIFIER OID_AT "\x2D" /**< id-at-uniqueIdentifier AttributType:= {id-at 45} */ 00126 #define OID_AT_DN_QUALIFIER OID_AT "\x2E" /**< id-at-dnQualifier AttributeType:= {id-at 46} */ 00127 #define OID_AT_PSEUDONYM OID_AT "\x41" /**< id-at-pseudonym AttributeType:= {id-at 65} */ 00128 00129 #define OID_DOMAIN_COMPONENT "\x09\x92\x26\x89\x93\xF2\x2C\x64\x01\x19" /** id-domainComponent AttributeType:= {itu-t(0) data(9) pss(2342) ucl(19200300) pilot(100) pilotAttributeType(1) domainComponent(25)} */ 00130 00131 /* 00132 * OIDs for standard certificate extensions 00133 */ 00134 #define OID_AUTHORITY_KEY_IDENTIFIER OID_ID_CE "\x23" /**< id-ce-authorityKeyIdentifier OBJECT IDENTIFIER ::= { id-ce 35 } */ 00135 #define OID_SUBJECT_KEY_IDENTIFIER OID_ID_CE "\x0E" /**< id-ce-subjectKeyIdentifier OBJECT IDENTIFIER ::= { id-ce 14 } */ 00136 #define OID_KEY_USAGE OID_ID_CE "\x0F" /**< id-ce-keyUsage OBJECT IDENTIFIER ::= { id-ce 15 } */ 00137 #define OID_CERTIFICATE_POLICIES OID_ID_CE "\x20" /**< id-ce-certificatePolicies OBJECT IDENTIFIER ::= { id-ce 32 } */ 00138 #define OID_POLICY_MAPPINGS OID_ID_CE "\x21" /**< id-ce-policyMappings OBJECT IDENTIFIER ::= { id-ce 33 } */ 00139 #define OID_SUBJECT_ALT_NAME OID_ID_CE "\x11" /**< id-ce-subjectAltName OBJECT IDENTIFIER ::= { id-ce 17 } */ 00140 #define OID_ISSUER_ALT_NAME OID_ID_CE "\x12" /**< id-ce-issuerAltName OBJECT IDENTIFIER ::= { id-ce 18 } */ 00141 #define OID_SUBJECT_DIRECTORY_ATTRS OID_ID_CE "\x09" /**< id-ce-subjectDirectoryAttributes OBJECT IDENTIFIER ::= { id-ce 9 } */ 00142 #define OID_BASIC_CONSTRAINTS OID_ID_CE "\x13" /**< id-ce-basicConstraints OBJECT IDENTIFIER ::= { id-ce 19 } */ 00143 #define OID_NAME_CONSTRAINTS OID_ID_CE "\x1E" /**< id-ce-nameConstraints OBJECT IDENTIFIER ::= { id-ce 30 } */ 00144 #define OID_POLICY_CONSTRAINTS OID_ID_CE "\x24" /**< id-ce-policyConstraints OBJECT IDENTIFIER ::= { id-ce 36 } */ 00145 #define OID_EXTENDED_KEY_USAGE OID_ID_CE "\x25" /**< id-ce-extKeyUsage OBJECT IDENTIFIER ::= { id-ce 37 } */ 00146 #define OID_CRL_DISTRIBUTION_POINTS OID_ID_CE "\x1F" /**< id-ce-cRLDistributionPoints OBJECT IDENTIFIER ::= { id-ce 31 } */ 00147 #define OID_INIHIBIT_ANYPOLICY OID_ID_CE "\x36" /**< id-ce-inhibitAnyPolicy OBJECT IDENTIFIER ::= { id-ce 54 } */ 00148 #define OID_FRESHEST_CRL OID_ID_CE "\x2E" /**< id-ce-freshestCRL OBJECT IDENTIFIER ::= { id-ce 46 } */ 00149 00150 /* 00151 * Netscape certificate extensions 00152 */ 00153 #define OID_NS_CERT OID_NETSCAPE "\x01" 00154 #define OID_NS_CERT_TYPE OID_NS_CERT "\x01" 00155 #define OID_NS_BASE_URL OID_NS_CERT "\x02" 00156 #define OID_NS_REVOCATION_URL OID_NS_CERT "\x03" 00157 #define OID_NS_CA_REVOCATION_URL OID_NS_CERT "\x04" 00158 #define OID_NS_RENEWAL_URL OID_NS_CERT "\x07" 00159 #define OID_NS_CA_POLICY_URL OID_NS_CERT "\x08" 00160 #define OID_NS_SSL_SERVER_NAME OID_NS_CERT "\x0C" 00161 #define OID_NS_COMMENT OID_NS_CERT "\x0D" 00162 #define OID_NS_DATA_TYPE OID_NETSCAPE "\x02" 00163 #define OID_NS_CERT_SEQUENCE OID_NS_DATA_TYPE "\x05" 00164 00165 /* 00166 * OIDs for CRL extensions 00167 */ 00168 #define OID_PRIVATE_KEY_USAGE_PERIOD OID_ID_CE "\x10" 00169 #define OID_CRL_NUMBER OID_ID_CE "\x14" /**< id-ce-cRLNumber OBJECT IDENTIFIER ::= { id-ce 20 } */ 00170 00171 /* 00172 * X.509 v3 Extended key usage OIDs 00173 */ 00174 #define OID_ANY_EXTENDED_KEY_USAGE OID_EXTENDED_KEY_USAGE "\x00" /**< anyExtendedKeyUsage OBJECT IDENTIFIER ::= { id-ce-extKeyUsage 0 } */ 00175 00176 #define OID_KP OID_PKIX "\x03" /**< id-kp OBJECT IDENTIFIER ::= { id-pkix 3 } */ 00177 #define OID_SERVER_AUTH OID_KP "\x01" /**< id-kp-serverAuth OBJECT IDENTIFIER ::= { id-kp 1 } */ 00178 #define OID_CLIENT_AUTH OID_KP "\x02" /**< id-kp-clientAuth OBJECT IDENTIFIER ::= { id-kp 2 } */ 00179 #define OID_CODE_SIGNING OID_KP "\x03" /**< id-kp-codeSigning OBJECT IDENTIFIER ::= { id-kp 3 } */ 00180 #define OID_EMAIL_PROTECTION OID_KP "\x04" /**< id-kp-emailProtection OBJECT IDENTIFIER ::= { id-kp 4 } */ 00181 #define OID_TIME_STAMPING OID_KP "\x08" /**< id-kp-timeStamping OBJECT IDENTIFIER ::= { id-kp 8 } */ 00182 #define OID_OCSP_SIGNING OID_KP "\x09" /**< id-kp-OCSPSigning OBJECT IDENTIFIER ::= { id-kp 9 } */ 00183 00184 /* 00185 * PKCS definition OIDs 00186 */ 00187 00188 #define OID_PKCS OID_RSA_COMPANY "\x01" /**< pkcs OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) 1 } */ 00189 #define OID_PKCS1 OID_PKCS "\x01" /**< pkcs-1 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) pkcs(1) 1 } */ 00190 #define OID_PKCS5 OID_PKCS "\x05" /**< pkcs-5 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) pkcs(1) 5 } */ 00191 #define OID_PKCS9 OID_PKCS "\x09" /**< pkcs-9 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) pkcs(1) 9 } */ 00192 #define OID_PKCS12 OID_PKCS "\x0c" /**< pkcs-12 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) pkcs(1) 12 } */ 00193 00194 /* 00195 * PKCS#1 OIDs 00196 */ 00197 #define OID_PKCS1_RSA OID_PKCS1 "\x01" /**< rsaEncryption OBJECT IDENTIFIER ::= { pkcs-1 1 } */ 00198 #define OID_PKCS1_MD2 OID_PKCS1 "\x02" /**< md2WithRSAEncryption ::= { pkcs-1 2 } */ 00199 #define OID_PKCS1_MD4 OID_PKCS1 "\x03" /**< md4WithRSAEncryption ::= { pkcs-1 3 } */ 00200 #define OID_PKCS1_MD5 OID_PKCS1 "\x04" /**< md5WithRSAEncryption ::= { pkcs-1 4 } */ 00201 #define OID_PKCS1_SHA1 OID_PKCS1 "\x05" /**< sha1WithRSAEncryption ::= { pkcs-1 5 } */ 00202 #define OID_PKCS1_SHA224 OID_PKCS1 "\x0e" /**< sha224WithRSAEncryption ::= { pkcs-1 14 } */ 00203 #define OID_PKCS1_SHA256 OID_PKCS1 "\x0b" /**< sha256WithRSAEncryption ::= { pkcs-1 11 } */ 00204 #define OID_PKCS1_SHA384 OID_PKCS1 "\x0c" /**< sha384WithRSAEncryption ::= { pkcs-1 12 } */ 00205 #define OID_PKCS1_SHA512 OID_PKCS1 "\x0d" /**< sha512WithRSAEncryption ::= { pkcs-1 13 } */ 00206 00207 #define OID_RSA_SHA_OBS "\x2B\x0E\x03\x02\x1D" 00208 00209 #define OID_PKCS9_EMAIL OID_PKCS9 "\x01" /**< emailAddress AttributeType ::= { pkcs-9 1 } */ 00210 00211 /* RFC 4055 */ 00212 #define OID_RSASSA_PSS OID_PKCS1 "\x0a" /**< id-RSASSA-PSS ::= { pkcs-1 10 } */ 00213 #define OID_MGF1 OID_PKCS1 "\x08" /**< id-mgf1 ::= { pkcs-1 8 } */ 00214 00215 /* 00216 * Digest algorithms 00217 */ 00218 #define OID_DIGEST_ALG_MD2 OID_RSA_COMPANY "\x02\x02" /**< id-md2 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) digestAlgorithm(2) 2 } */ 00219 #define OID_DIGEST_ALG_MD4 OID_RSA_COMPANY "\x02\x04" /**< id-md4 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) digestAlgorithm(2) 4 } */ 00220 #define OID_DIGEST_ALG_MD5 OID_RSA_COMPANY "\x02\x05" /**< id-md5 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) digestAlgorithm(2) 5 } */ 00221 #define OID_DIGEST_ALG_SHA1 OID_ISO_IDENTIFIED_ORG OID_OIW_SECSIG_SHA1 /**< id-sha1 OBJECT IDENTIFIER ::= { iso(1) identified-organization(3) oiw(14) secsig(3) algorithms(2) 26 } */ 00222 #define OID_DIGEST_ALG_SHA224 OID_GOV "\x03\x04\x02\x04" /**< id-sha224 OBJECT IDENTIFIER ::= { joint-iso-itu-t(2) country(16) us(840) organization(1) gov(101) csor(3) nistalgorithm(4) hashalgs(2) 4 } */ 00223 #define OID_DIGEST_ALG_SHA256 OID_GOV "\x03\x04\x02\x01" /**< id-sha256 OBJECT IDENTIFIER ::= { joint-iso-itu-t(2) country(16) us(840) organization(1) gov(101) csor(3) nistalgorithm(4) hashalgs(2) 1 } */ 00224 00225 #define OID_DIGEST_ALG_SHA384 OID_GOV "\x03\x04\x02\x02" /**< id-sha384 OBJECT IDENTIFIER ::= { joint-iso-itu-t(2) country(16) us(840) organization(1) gov(101) csor(3) nistalgorithm(4) hashalgs(2) 2 } */ 00226 00227 #define OID_DIGEST_ALG_SHA512 OID_GOV "\x03\x04\x02\x03" /**< id-sha512 OBJECT IDENTIFIER ::= { joint-iso-itu-t(2) country(16) us(840) organization(1) gov(101) csor(3) nistalgorithm(4) hashalgs(2) 3 } */ 00228 00229 #define OID_HMAC_SHA1 OID_RSA_COMPANY "\x02\x07" /**< id-hmacWithSHA1 OBJECT IDENTIFIER ::= { iso(1) member-body(2) us(840) rsadsi(113549) digestAlgorithm(2) 7 } */ 00230 00231 /* 00232 * Encryption algorithms 00233 */ 00234 #define OID_DES_CBC OID_ISO_IDENTIFIED_ORG OID_OIW_SECSIG_ALG "\x07" /**< desCBC OBJECT IDENTIFIER ::= { iso(1) identified-organization(3) oiw(14) secsig(3) algorithms(2) 7 } */ 00235 #define OID_DES_EDE3_CBC OID_RSA_COMPANY "\x03\x07" /**< des-ede3-cbc OBJECT IDENTIFIER ::= { iso(1) member-body(2) -- us(840) rsadsi(113549) encryptionAlgorithm(3) 7 } */ 00236 00237 /* 00238 * PKCS#5 OIDs 00239 */ 00240 #define OID_PKCS5_PBKDF2 OID_PKCS5 "\x0c" /**< id-PBKDF2 OBJECT IDENTIFIER ::= {pkcs-5 12} */ 00241 #define OID_PKCS5_PBES2 OID_PKCS5 "\x0d" /**< id-PBES2 OBJECT IDENTIFIER ::= {pkcs-5 13} */ 00242 #define OID_PKCS5_PBMAC1 OID_PKCS5 "\x0e" /**< id-PBMAC1 OBJECT IDENTIFIER ::= {pkcs-5 14} */ 00243 00244 /* 00245 * PKCS#5 PBES1 algorithms 00246 */ 00247 #define OID_PKCS5_PBE_MD2_DES_CBC OID_PKCS5 "\x01" /**< pbeWithMD2AndDES-CBC OBJECT IDENTIFIER ::= {pkcs-5 1} */ 00248 #define OID_PKCS5_PBE_MD2_RC2_CBC OID_PKCS5 "\x04" /**< pbeWithMD2AndRC2-CBC OBJECT IDENTIFIER ::= {pkcs-5 4} */ 00249 #define OID_PKCS5_PBE_MD5_DES_CBC OID_PKCS5 "\x03" /**< pbeWithMD5AndDES-CBC OBJECT IDENTIFIER ::= {pkcs-5 3} */ 00250 #define OID_PKCS5_PBE_MD5_RC2_CBC OID_PKCS5 "\x06" /**< pbeWithMD5AndRC2-CBC OBJECT IDENTIFIER ::= {pkcs-5 6} */ 00251 #define OID_PKCS5_PBE_SHA1_DES_CBC OID_PKCS5 "\x0a" /**< pbeWithSHA1AndDES-CBC OBJECT IDENTIFIER ::= {pkcs-5 10} */ 00252 #define OID_PKCS5_PBE_SHA1_RC2_CBC OID_PKCS5 "\x0b" /**< pbeWithSHA1AndRC2-CBC OBJECT IDENTIFIER ::= {pkcs-5 11} */ 00253 00254 /* 00255 * PKCS#8 OIDs 00256 */ 00257 #define OID_PKCS9_CSR_EXT_REQ OID_PKCS9 "\x0e" /**< extensionRequest OBJECT IDENTIFIER ::= {pkcs-9 14} */ 00258 00259 /* 00260 * PKCS#12 PBE OIDs 00261 */ 00262 #define OID_PKCS12_PBE OID_PKCS12 "\x01" /**< pkcs-12PbeIds OBJECT IDENTIFIER ::= {pkcs-12 1} */ 00263 00264 #define OID_PKCS12_PBE_SHA1_RC4_128 OID_PKCS12_PBE "\x01" /**< pbeWithSHAAnd128BitRC4 OBJECT IDENTIFIER ::= {pkcs-12PbeIds 1} */ 00265 #define OID_PKCS12_PBE_SHA1_RC4_40 OID_PKCS12_PBE "\x02" /**< pbeWithSHAAnd40BitRC4 OBJECT IDENTIFIER ::= {pkcs-12PbeIds 2} */ 00266 #define OID_PKCS12_PBE_SHA1_DES3_EDE_CBC OID_PKCS12_PBE "\x03" /**< pbeWithSHAAnd3-KeyTripleDES-CBC OBJECT IDENTIFIER ::= {pkcs-12PbeIds 3} */ 00267 #define OID_PKCS12_PBE_SHA1_DES2_EDE_CBC OID_PKCS12_PBE "\x04" /**< pbeWithSHAAnd2-KeyTripleDES-CBC OBJECT IDENTIFIER ::= {pkcs-12PbeIds 4} */ 00268 #define OID_PKCS12_PBE_SHA1_RC2_128_CBC OID_PKCS12_PBE "\x05" /**< pbeWithSHAAnd128BitRC2-CBC OBJECT IDENTIFIER ::= {pkcs-12PbeIds 5} */ 00269 #define OID_PKCS12_PBE_SHA1_RC2_40_CBC OID_PKCS12_PBE "\x06" /**< pbeWithSHAAnd40BitRC2-CBC OBJECT IDENTIFIER ::= {pkcs-12PbeIds 6} */ 00270 00271 /* 00272 * EC key algorithms from RFC 5480 00273 */ 00274 00275 /* id-ecPublicKey OBJECT IDENTIFIER ::= { 00276 * iso(1) member-body(2) us(840) ansi-X9-62(10045) keyType(2) 1 } */ 00277 #define OID_EC_ALG_UNRESTRICTED OID_ANSI_X9_62 "\x02\01" 00278 00279 /* id-ecDH OBJECT IDENTIFIER ::= { 00280 * iso(1) identified-organization(3) certicom(132) 00281 * schemes(1) ecdh(12) } */ 00282 #define OID_EC_ALG_ECDH OID_CERTICOM "\x01\x0c" 00283 00284 /* 00285 * ECParameters namedCurve identifiers, from RFC 5480, RFC 5639, and SEC2 00286 */ 00287 00288 /* secp192r1 OBJECT IDENTIFIER ::= { 00289 * iso(1) member-body(2) us(840) ansi-X9-62(10045) curves(3) prime(1) 1 } */ 00290 #define OID_EC_GRP_SECP192R1 OID_ANSI_X9_62 "\x03\x01\x01" 00291 00292 /* secp224r1 OBJECT IDENTIFIER ::= { 00293 * iso(1) identified-organization(3) certicom(132) curve(0) 33 } */ 00294 #define OID_EC_GRP_SECP224R1 OID_CERTICOM "\x00\x21" 00295 00296 /* secp256r1 OBJECT IDENTIFIER ::= { 00297 * iso(1) member-body(2) us(840) ansi-X9-62(10045) curves(3) prime(1) 7 } */ 00298 #define OID_EC_GRP_SECP256R1 OID_ANSI_X9_62 "\x03\x01\x07" 00299 00300 /* secp384r1 OBJECT IDENTIFIER ::= { 00301 * iso(1) identified-organization(3) certicom(132) curve(0) 34 } */ 00302 #define OID_EC_GRP_SECP384R1 OID_CERTICOM "\x00\x22" 00303 00304 /* secp521r1 OBJECT IDENTIFIER ::= { 00305 * iso(1) identified-organization(3) certicom(132) curve(0) 35 } */ 00306 #define OID_EC_GRP_SECP521R1 OID_CERTICOM "\x00\x23" 00307 00308 /* secp192k1 OBJECT IDENTIFIER ::= { 00309 * iso(1) identified-organization(3) certicom(132) curve(0) 31 } */ 00310 #define OID_EC_GRP_SECP192K1 OID_CERTICOM "\x00\x1f" 00311 00312 /* secp224k1 OBJECT IDENTIFIER ::= { 00313 * iso(1) identified-organization(3) certicom(132) curve(0) 32 } */ 00314 #define OID_EC_GRP_SECP224K1 OID_CERTICOM "\x00\x20" 00315 00316 /* secp256k1 OBJECT IDENTIFIER ::= { 00317 * iso(1) identified-organization(3) certicom(132) curve(0) 10 } */ 00318 #define OID_EC_GRP_SECP256K1 OID_CERTICOM "\x00\x0a" 00319 00320 /* RFC 5639 4.1 00321 * ecStdCurvesAndGeneration OBJECT IDENTIFIER::= {iso(1) 00322 * identified-organization(3) teletrust(36) algorithm(3) signature- 00323 * algorithm(3) ecSign(2) 8} 00324 * ellipticCurve OBJECT IDENTIFIER ::= {ecStdCurvesAndGeneration 1} 00325 * versionOne OBJECT IDENTIFIER ::= {ellipticCurve 1} */ 00326 #define OID_EC_BRAINPOOL_V1 OID_TELETRUST "\x03\x03\x02\x08\x01\x01" 00327 00328 /* brainpoolP256r1 OBJECT IDENTIFIER ::= {versionOne 7} */ 00329 #define OID_EC_GRP_BP256R1 OID_EC_BRAINPOOL_V1 "\x07" 00330 00331 /* brainpoolP384r1 OBJECT IDENTIFIER ::= {versionOne 11} */ 00332 #define OID_EC_GRP_BP384R1 OID_EC_BRAINPOOL_V1 "\x0B" 00333 00334 /* brainpoolP512r1 OBJECT IDENTIFIER ::= {versionOne 13} */ 00335 #define OID_EC_GRP_BP512R1 OID_EC_BRAINPOOL_V1 "\x0D" 00336 00337 /* 00338 * SEC1 C.1 00339 * 00340 * prime-field OBJECT IDENTIFIER ::= { id-fieldType 1 } 00341 * id-fieldType OBJECT IDENTIFIER ::= { ansi-X9-62 fieldType(1)} 00342 */ 00343 #define OID_ANSI_X9_62_FIELD_TYPE OID_ANSI_X9_62 "\x01" 00344 #define OID_ANSI_X9_62_PRIME_FIELD OID_ANSI_X9_62_FIELD_TYPE "\x01" 00345 00346 /* 00347 * ECDSA signature identifiers, from RFC 5480 00348 */ 00349 #define OID_ANSI_X9_62_SIG OID_ANSI_X9_62 "\x04" /* signatures(4) */ 00350 #define OID_ANSI_X9_62_SIG_SHA2 OID_ANSI_X9_62_SIG "\x03" /* ecdsa-with-SHA2(3) */ 00351 00352 /* ecdsa-with-SHA1 OBJECT IDENTIFIER ::= { 00353 * iso(1) member-body(2) us(840) ansi-X9-62(10045) signatures(4) 1 } */ 00354 #define OID_ECDSA_SHA1 OID_ANSI_X9_62_SIG "\x01" 00355 00356 /* ecdsa-with-SHA224 OBJECT IDENTIFIER ::= { 00357 * iso(1) member-body(2) us(840) ansi-X9-62(10045) signatures(4) 00358 * ecdsa-with-SHA2(3) 1 } */ 00359 #define OID_ECDSA_SHA224 OID_ANSI_X9_62_SIG_SHA2 "\x01" 00360 00361 /* ecdsa-with-SHA256 OBJECT IDENTIFIER ::= { 00362 * iso(1) member-body(2) us(840) ansi-X9-62(10045) signatures(4) 00363 * ecdsa-with-SHA2(3) 2 } */ 00364 #define OID_ECDSA_SHA256 OID_ANSI_X9_62_SIG_SHA2 "\x02" 00365 00366 /* ecdsa-with-SHA384 OBJECT IDENTIFIER ::= { 00367 * iso(1) member-body(2) us(840) ansi-X9-62(10045) signatures(4) 00368 * ecdsa-with-SHA2(3) 3 } */ 00369 #define OID_ECDSA_SHA384 OID_ANSI_X9_62_SIG_SHA2 "\x03" 00370 00371 /* ecdsa-with-SHA512 OBJECT IDENTIFIER ::= { 00372 * iso(1) member-body(2) us(840) ansi-X9-62(10045) signatures(4) 00373 * ecdsa-with-SHA2(3) 4 } */ 00374 #define OID_ECDSA_SHA512 OID_ANSI_X9_62_SIG_SHA2 "\x04" 00375 00376 #ifdef __cplusplus 00377 extern "C" { 00378 #endif 00379 00380 /** 00381 * \brief Base OID descriptor structure 00382 */ 00383 typedef struct { 00384 const char *asn1 ; /*!< OID ASN.1 representation */ 00385 size_t asn1_len ; /*!< length of asn1 */ 00386 const char *name ; /*!< official name (e.g. from RFC) */ 00387 const char *description ; /*!< human friendly description */ 00388 } oid_descriptor_t; 00389 00390 /** 00391 * \brief Translate an ASN.1 OID into its numeric representation 00392 * (e.g. "\x2A\x86\x48\x86\xF7\x0D" into "1.2.840.113549") 00393 * 00394 * \param buf buffer to put representation in 00395 * \param size size of the buffer 00396 * \param oid OID to translate 00397 * 00398 * \return Length of the string written (excluding final NULL) or 00399 * POLARSSL_ERR_OID_BUF_TOO_SMALL in case of error 00400 */ 00401 int oid_get_numeric_string( char *buf, size_t size, const asn1_buf *oid ); 00402 00403 #if defined(POLARSSL_X509_USE_C) || defined(POLARSSL_X509_CREATE_C) 00404 /** 00405 * \brief Translate an X.509 extension OID into local values 00406 * 00407 * \param oid OID to use 00408 * \param ext_type place to store the extension type 00409 * 00410 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00411 */ 00412 int oid_get_x509_ext_type( const asn1_buf *oid, int *ext_type ); 00413 #endif 00414 00415 /** 00416 * \brief Translate an X.509 attribute type OID into the short name 00417 * (e.g. the OID for an X520 Common Name into "CN") 00418 * 00419 * \param oid OID to use 00420 * \param short_name place to store the string pointer 00421 * 00422 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00423 */ 00424 int oid_get_attr_short_name( const asn1_buf *oid, const char **short_name ); 00425 00426 /** 00427 * \brief Translate PublicKeyAlgorithm OID into pk_type 00428 * 00429 * \param oid OID to use 00430 * \param pk_alg place to store public key algorithm 00431 * 00432 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00433 */ 00434 int oid_get_pk_alg( const asn1_buf *oid, pk_type_t *pk_alg ); 00435 00436 /** 00437 * \brief Translate pk_type into PublicKeyAlgorithm OID 00438 * 00439 * \param pk_alg Public key type to look for 00440 * \param oid place to store ASN.1 OID string pointer 00441 * \param olen length of the OID 00442 * 00443 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00444 */ 00445 int oid_get_oid_by_pk_alg( pk_type_t pk_alg, 00446 const char **oid, size_t *olen ); 00447 00448 #if defined(POLARSSL_ECP_C) 00449 /** 00450 * \brief Translate NamedCurve OID into an EC group identifier 00451 * 00452 * \param oid OID to use 00453 * \param grp_id place to store group id 00454 * 00455 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00456 */ 00457 int oid_get_ec_grp( const asn1_buf *oid, ecp_group_id *grp_id ); 00458 00459 /** 00460 * \brief Translate EC group identifier into NamedCurve OID 00461 * 00462 * \param grp_id EC group identifier 00463 * \param oid place to store ASN.1 OID string pointer 00464 * \param olen length of the OID 00465 * 00466 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00467 */ 00468 int oid_get_oid_by_ec_grp( ecp_group_id grp_id, 00469 const char **oid, size_t *olen ); 00470 #endif /* POLARSSL_ECP_C */ 00471 00472 #if defined(POLARSSL_MD_C) 00473 /** 00474 * \brief Translate SignatureAlgorithm OID into md_type and pk_type 00475 * 00476 * \param oid OID to use 00477 * \param md_alg place to store message digest algorithm 00478 * \param pk_alg place to store public key algorithm 00479 * 00480 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00481 */ 00482 int oid_get_sig_alg( const asn1_buf *oid, 00483 md_type_t *md_alg, pk_type_t *pk_alg ); 00484 00485 /** 00486 * \brief Translate SignatureAlgorithm OID into description 00487 * 00488 * \param oid OID to use 00489 * \param desc place to store string pointer 00490 * 00491 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00492 */ 00493 int oid_get_sig_alg_desc( const asn1_buf *oid, const char **desc ); 00494 00495 /** 00496 * \brief Translate md_type and pk_type into SignatureAlgorithm OID 00497 * 00498 * \param md_alg message digest algorithm 00499 * \param pk_alg public key algorithm 00500 * \param oid place to store ASN.1 OID string pointer 00501 * \param olen length of the OID 00502 * 00503 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00504 */ 00505 int oid_get_oid_by_sig_alg( pk_type_t pk_alg, md_type_t md_alg, 00506 const char **oid, size_t *olen ); 00507 00508 /** 00509 * \brief Translate hash algorithm OID into md_type 00510 * 00511 * \param oid OID to use 00512 * \param md_alg place to store message digest algorithm 00513 * 00514 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00515 */ 00516 int oid_get_md_alg( const asn1_buf *oid, md_type_t *md_alg ); 00517 #endif /* POLARSSL_MD_C */ 00518 00519 /** 00520 * \brief Translate Extended Key Usage OID into description 00521 * 00522 * \param oid OID to use 00523 * \param desc place to store string pointer 00524 * 00525 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00526 */ 00527 int oid_get_extended_key_usage( const asn1_buf *oid, const char **desc ); 00528 00529 /** 00530 * \brief Translate md_type into hash algorithm OID 00531 * 00532 * \param md_alg message digest algorithm 00533 * \param oid place to store ASN.1 OID string pointer 00534 * \param olen length of the OID 00535 * 00536 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00537 */ 00538 int oid_get_oid_by_md( md_type_t md_alg, const char **oid, size_t *olen ); 00539 00540 #if defined(POLARSSL_CIPHER_C) 00541 /** 00542 * \brief Translate encryption algorithm OID into cipher_type 00543 * 00544 * \param oid OID to use 00545 * \param cipher_alg place to store cipher algorithm 00546 * 00547 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00548 */ 00549 int oid_get_cipher_alg( const asn1_buf *oid, cipher_type_t *cipher_alg ); 00550 #endif /* POLARSSL_CIPHER_C */ 00551 00552 #if defined(POLARSSL_PKCS12_C) 00553 /** 00554 * \brief Translate PKCS#12 PBE algorithm OID into md_type and 00555 * cipher_type 00556 * 00557 * \param oid OID to use 00558 * \param md_alg place to store message digest algorithm 00559 * \param cipher_alg place to store cipher algorithm 00560 * 00561 * \return 0 if successful, or POLARSSL_ERR_OID_NOT_FOUND 00562 */ 00563 int oid_get_pkcs12_pbe_alg( const asn1_buf *oid, md_type_t *md_alg, 00564 cipher_type_t *cipher_alg ); 00565 #endif /* POLARSSL_PKCS12_C */ 00566 00567 #ifdef __cplusplus 00568 } 00569 #endif 00570 00571 #endif /* oid.h */ 00572
Generated on Tue Jul 12 2022 13:50:37 by
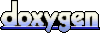