mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
memory_buffer_alloc.h
00001 /** 00002 * \file memory_buffer_alloc.h 00003 * 00004 * \brief Buffer-based memory allocator 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_MEMORY_BUFFER_ALLOC_H 00025 #define POLARSSL_MEMORY_BUFFER_ALLOC_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 /** 00036 * \name SECTION: Module settings 00037 * 00038 * The configuration options you can set for this module are in this section. 00039 * Either change them in config.h or define them on the compiler command line. 00040 * \{ 00041 */ 00042 00043 #if !defined(POLARSSL_MEMORY_ALIGN_MULTIPLE) 00044 #define POLARSSL_MEMORY_ALIGN_MULTIPLE 4 /**< Align on multiples of this value */ 00045 #endif 00046 00047 /* \} name SECTION: Module settings */ 00048 00049 #define MEMORY_VERIFY_NONE 0 00050 #define MEMORY_VERIFY_ALLOC (1 << 0) 00051 #define MEMORY_VERIFY_FREE (1 << 1) 00052 #define MEMORY_VERIFY_ALWAYS (MEMORY_VERIFY_ALLOC | MEMORY_VERIFY_FREE) 00053 00054 #ifdef __cplusplus 00055 extern "C" { 00056 #endif 00057 00058 /** 00059 * \brief Initialize use of stack-based memory allocator. 00060 * The stack-based allocator does memory management inside the 00061 * presented buffer and does not call malloc() and free(). 00062 * It sets the global polarssl_malloc() and polarssl_free() pointers 00063 * to its own functions. 00064 * (Provided polarssl_malloc() and polarssl_free() are thread-safe if 00065 * POLARSSL_THREADING_C is defined) 00066 * 00067 * \note This code is not optimized and provides a straight-forward 00068 * implementation of a stack-based memory allocator. 00069 * 00070 * \param buf buffer to use as heap 00071 * \param len size of the buffer 00072 * 00073 * \return 0 if successful 00074 */ 00075 int memory_buffer_alloc_init( unsigned char *buf, size_t len ); 00076 00077 /** 00078 * \brief Free the mutex for thread-safety and clear remaining memory 00079 */ 00080 void memory_buffer_alloc_free( void ); 00081 00082 /** 00083 * \brief Determine when the allocator should automatically verify the state 00084 * of the entire chain of headers / meta-data. 00085 * (Default: MEMORY_VERIFY_NONE) 00086 * 00087 * \param verify One of MEMORY_VERIFY_NONE, MEMORY_VERIFY_ALLOC, 00088 * MEMORY_VERIFY_FREE or MEMORY_VERIFY_ALWAYS 00089 */ 00090 void memory_buffer_set_verify( int verify ); 00091 00092 #if defined(POLARSSL_MEMORY_DEBUG) 00093 /** 00094 * \brief Print out the status of the allocated memory (primarily for use 00095 * after a program should have de-allocated all memory) 00096 * Prints out a list of 'still allocated' blocks and their stack 00097 * trace if POLARSSL_MEMORY_BACKTRACE is defined. 00098 */ 00099 void memory_buffer_alloc_status( void ); 00100 00101 /** 00102 * \brief Get the peak heap usage so far 00103 * 00104 * \param max_used Peak number of bytes reauested by the application 00105 * \param max_blocks Peak number of blocks reauested by the application 00106 */ 00107 void memory_buffer_alloc_max_get( size_t *max_used, size_t *max_blocks ); 00108 00109 /** 00110 * \brief Reset peak statistics 00111 */ 00112 void memory_buffer_alloc_max_reset( void ); 00113 00114 /** 00115 * \brief Get the current heap usage 00116 * 00117 * \param cur_used Number of bytes reauested by the application 00118 * \param cur_blocks Number of blocks reauested by the application 00119 */ 00120 void memory_buffer_alloc_cur_get( size_t *cur_used, size_t *cur_blocks ); 00121 #endif /* POLARSSL_MEMORY_DEBUG */ 00122 00123 /** 00124 * \brief Verifies that all headers in the memory buffer are correct 00125 * and contain sane values. Helps debug buffer-overflow errors. 00126 * 00127 * Prints out first failure if POLARSSL_MEMORY_DEBUG is defined. 00128 * Prints out full header information if POLARSSL_MEMORY_DEBUG_HEADERS 00129 * is defined. (Includes stack trace information for each block if 00130 * POLARSSL_MEMORY_BACKTRACE is defined as well). 00131 * 00132 * \returns 0 if verified, 1 otherwise 00133 */ 00134 int memory_buffer_alloc_verify( void ); 00135 00136 #if defined(POLARSSL_SELF_TEST) 00137 /** 00138 * \brief Checkup routine 00139 * 00140 * \return 0 if successful, or 1 if a test failed 00141 */ 00142 int memory_buffer_alloc_self_test( int verbose ); 00143 #endif 00144 00145 #ifdef __cplusplus 00146 } 00147 #endif 00148 00149 #endif /* memory_buffer_alloc.h */ 00150
Generated on Tue Jul 12 2022 13:50:37 by
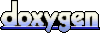