mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
memory_buffer_alloc.c
00001 /* 00002 * Buffer-based memory allocator 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 #if !defined(POLARSSL_CONFIG_FILE) 00024 #include "polarssl/config.h" 00025 #else 00026 #include POLARSSL_CONFIG_FILE 00027 #endif 00028 00029 #if defined(POLARSSL_MEMORY_BUFFER_ALLOC_C) 00030 #include "polarssl/memory_buffer_alloc.h" 00031 00032 /* No need for the header guard as POLARSSL_MEMORY_BUFFER_ALLOC_C 00033 is dependent upon POLARSSL_PLATFORM_C */ 00034 #include "polarssl/platform.h" 00035 00036 #include <string.h> 00037 00038 #if defined(POLARSSL_MEMORY_BACKTRACE) 00039 #include <execinfo.h> 00040 #endif 00041 00042 #if defined(POLARSSL_THREADING_C) 00043 #include "polarssl/threading.h" 00044 #endif 00045 00046 /* Implementation that should never be optimized out by the compiler */ 00047 static void polarssl_zeroize( void *v, size_t n ) { 00048 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00049 } 00050 00051 #define MAGIC1 0xFF00AA55 00052 #define MAGIC2 0xEE119966 00053 #define MAX_BT 20 00054 00055 typedef struct _memory_header memory_header; 00056 struct _memory_header 00057 { 00058 size_t magic1; 00059 size_t size; 00060 size_t alloc; 00061 memory_header *prev; 00062 memory_header *next; 00063 memory_header *prev_free; 00064 memory_header *next_free; 00065 #if defined(POLARSSL_MEMORY_BACKTRACE) 00066 char **trace; 00067 size_t trace_count; 00068 #endif 00069 size_t magic2; 00070 }; 00071 00072 typedef struct 00073 { 00074 unsigned char *buf; 00075 size_t len; 00076 memory_header *first; 00077 memory_header *first_free; 00078 int verify; 00079 #if defined(POLARSSL_MEMORY_DEBUG) 00080 size_t malloc_count; 00081 size_t free_count; 00082 size_t total_used; 00083 size_t maximum_used; 00084 size_t header_count; 00085 size_t maximum_header_count; 00086 #endif 00087 #if defined(POLARSSL_THREADING_C) 00088 threading_mutex_t mutex; 00089 #endif 00090 } 00091 buffer_alloc_ctx; 00092 00093 static buffer_alloc_ctx heap; 00094 00095 #if defined(POLARSSL_MEMORY_DEBUG) 00096 static void debug_header( memory_header *hdr ) 00097 { 00098 #if defined(POLARSSL_MEMORY_BACKTRACE) 00099 size_t i; 00100 #endif 00101 00102 polarssl_fprintf( stderr, "HDR: PTR(%10zu), PREV(%10zu), NEXT(%10zu), " 00103 "ALLOC(%zu), SIZE(%10zu)\n", 00104 (size_t) hdr, (size_t) hdr->prev, (size_t) hdr->next, 00105 hdr->alloc, hdr->size ); 00106 polarssl_fprintf( stderr, " FPREV(%10zu), FNEXT(%10zu)\n", 00107 (size_t) hdr->prev_free, (size_t) hdr->next_free ); 00108 00109 #if defined(POLARSSL_MEMORY_BACKTRACE) 00110 polarssl_fprintf( stderr, "TRACE: \n" ); 00111 for( i = 0; i < hdr->trace_count; i++ ) 00112 polarssl_fprintf( stderr, "%s\n", hdr->trace[i] ); 00113 polarssl_fprintf( stderr, "\n" ); 00114 #endif 00115 } 00116 00117 static void debug_chain() 00118 { 00119 memory_header *cur = heap.first; 00120 00121 polarssl_fprintf( stderr, "\nBlock list\n" ); 00122 while( cur != NULL ) 00123 { 00124 debug_header( cur ); 00125 cur = cur->next; 00126 } 00127 00128 polarssl_fprintf( stderr, "Free list\n" ); 00129 cur = heap.first_free; 00130 00131 while( cur != NULL ) 00132 { 00133 debug_header( cur ); 00134 cur = cur->next_free; 00135 } 00136 } 00137 #endif /* POLARSSL_MEMORY_DEBUG */ 00138 00139 static int verify_header( memory_header *hdr ) 00140 { 00141 if( hdr->magic1 != MAGIC1 ) 00142 { 00143 #if defined(POLARSSL_MEMORY_DEBUG) 00144 polarssl_fprintf( stderr, "FATAL: MAGIC1 mismatch\n" ); 00145 #endif 00146 return( 1 ); 00147 } 00148 00149 if( hdr->magic2 != MAGIC2 ) 00150 { 00151 #if defined(POLARSSL_MEMORY_DEBUG) 00152 polarssl_fprintf( stderr, "FATAL: MAGIC2 mismatch\n" ); 00153 #endif 00154 return( 1 ); 00155 } 00156 00157 if( hdr->alloc > 1 ) 00158 { 00159 #if defined(POLARSSL_MEMORY_DEBUG) 00160 polarssl_fprintf( stderr, "FATAL: alloc has illegal value\n" ); 00161 #endif 00162 return( 1 ); 00163 } 00164 00165 if( hdr->prev != NULL && hdr->prev == hdr->next ) 00166 { 00167 #if defined(POLARSSL_MEMORY_DEBUG) 00168 polarssl_fprintf( stderr, "FATAL: prev == next\n" ); 00169 #endif 00170 return( 1 ); 00171 } 00172 00173 if( hdr->prev_free != NULL && hdr->prev_free == hdr->next_free ) 00174 { 00175 #if defined(POLARSSL_MEMORY_DEBUG) 00176 polarssl_fprintf( stderr, "FATAL: prev_free == next_free\n" ); 00177 #endif 00178 return( 1 ); 00179 } 00180 00181 return( 0 ); 00182 } 00183 00184 static int verify_chain() 00185 { 00186 memory_header *prv = heap.first, *cur = heap.first->next; 00187 00188 if( verify_header( heap.first ) != 0 ) 00189 { 00190 #if defined(POLARSSL_MEMORY_DEBUG) 00191 polarssl_fprintf( stderr, "FATAL: verification of first header " 00192 "failed\n" ); 00193 #endif 00194 return( 1 ); 00195 } 00196 00197 if( heap.first->prev != NULL ) 00198 { 00199 #if defined(POLARSSL_MEMORY_DEBUG) 00200 polarssl_fprintf( stderr, "FATAL: verification failed: " 00201 "first->prev != NULL\n" ); 00202 #endif 00203 return( 1 ); 00204 } 00205 00206 while( cur != NULL ) 00207 { 00208 if( verify_header( cur ) != 0 ) 00209 { 00210 #if defined(POLARSSL_MEMORY_DEBUG) 00211 polarssl_fprintf( stderr, "FATAL: verification of header " 00212 "failed\n" ); 00213 #endif 00214 return( 1 ); 00215 } 00216 00217 if( cur->prev != prv ) 00218 { 00219 #if defined(POLARSSL_MEMORY_DEBUG) 00220 polarssl_fprintf( stderr, "FATAL: verification failed: " 00221 "cur->prev != prv\n" ); 00222 #endif 00223 return( 1 ); 00224 } 00225 00226 prv = cur; 00227 cur = cur->next; 00228 } 00229 00230 return( 0 ); 00231 } 00232 00233 static void *buffer_alloc_malloc( size_t len ) 00234 { 00235 memory_header *new, *cur = heap.first_free; 00236 unsigned char *p; 00237 #if defined(POLARSSL_MEMORY_BACKTRACE) 00238 void *trace_buffer[MAX_BT]; 00239 size_t trace_cnt; 00240 #endif 00241 00242 if( heap.buf == NULL || heap.first == NULL ) 00243 return( NULL ); 00244 00245 if( len % POLARSSL_MEMORY_ALIGN_MULTIPLE ) 00246 { 00247 len -= len % POLARSSL_MEMORY_ALIGN_MULTIPLE; 00248 len += POLARSSL_MEMORY_ALIGN_MULTIPLE; 00249 } 00250 00251 // Find block that fits 00252 // 00253 while( cur != NULL ) 00254 { 00255 if( cur->size >= len ) 00256 break; 00257 00258 cur = cur->next_free; 00259 } 00260 00261 if( cur == NULL ) 00262 return( NULL ); 00263 00264 if( cur->alloc != 0 ) 00265 { 00266 #if defined(POLARSSL_MEMORY_DEBUG) 00267 polarssl_fprintf( stderr, "FATAL: block in free_list but allocated " 00268 "data\n" ); 00269 #endif 00270 polarssl_exit( 1 ); 00271 } 00272 00273 #if defined(POLARSSL_MEMORY_DEBUG) 00274 heap.malloc_count++; 00275 #endif 00276 00277 // Found location, split block if > memory_header + 4 room left 00278 // 00279 if( cur->size - len < sizeof(memory_header) + 00280 POLARSSL_MEMORY_ALIGN_MULTIPLE ) 00281 { 00282 cur->alloc = 1; 00283 00284 // Remove from free_list 00285 // 00286 if( cur->prev_free != NULL ) 00287 cur->prev_free->next_free = cur->next_free; 00288 else 00289 heap.first_free = cur->next_free; 00290 00291 if( cur->next_free != NULL ) 00292 cur->next_free->prev_free = cur->prev_free; 00293 00294 cur->prev_free = NULL; 00295 cur->next_free = NULL; 00296 00297 #if defined(POLARSSL_MEMORY_DEBUG) 00298 heap.total_used += cur->size; 00299 if( heap.total_used > heap.maximum_used ) 00300 heap.maximum_used = heap.total_used; 00301 #endif 00302 #if defined(POLARSSL_MEMORY_BACKTRACE) 00303 trace_cnt = backtrace( trace_buffer, MAX_BT ); 00304 cur->trace = backtrace_symbols( trace_buffer, trace_cnt ); 00305 cur->trace_count = trace_cnt; 00306 #endif 00307 00308 if( ( heap.verify & MEMORY_VERIFY_ALLOC ) && verify_chain() != 0 ) 00309 polarssl_exit( 1 ); 00310 00311 return( ( (unsigned char *) cur ) + sizeof(memory_header) ); 00312 } 00313 00314 p = ( (unsigned char *) cur ) + sizeof(memory_header) + len; 00315 new = (memory_header *) p; 00316 00317 new->size = cur->size - len - sizeof(memory_header); 00318 new->alloc = 0; 00319 new->prev = cur; 00320 new->next = cur->next; 00321 #if defined(POLARSSL_MEMORY_BACKTRACE) 00322 new->trace = NULL; 00323 new->trace_count = 0; 00324 #endif 00325 new->magic1 = MAGIC1; 00326 new->magic2 = MAGIC2; 00327 00328 if( new->next != NULL ) 00329 new->next->prev = new; 00330 00331 // Replace cur with new in free_list 00332 // 00333 new->prev_free = cur->prev_free; 00334 new->next_free = cur->next_free; 00335 if( new->prev_free != NULL ) 00336 new->prev_free->next_free = new; 00337 else 00338 heap.first_free = new; 00339 00340 if( new->next_free != NULL ) 00341 new->next_free->prev_free = new; 00342 00343 cur->alloc = 1; 00344 cur->size = len; 00345 cur->next = new; 00346 cur->prev_free = NULL; 00347 cur->next_free = NULL; 00348 00349 #if defined(POLARSSL_MEMORY_DEBUG) 00350 heap.header_count++; 00351 if( heap.header_count > heap.maximum_header_count ) 00352 heap.maximum_header_count = heap.header_count; 00353 heap.total_used += cur->size; 00354 if( heap.total_used > heap.maximum_used ) 00355 heap.maximum_used = heap.total_used; 00356 #endif 00357 #if defined(POLARSSL_MEMORY_BACKTRACE) 00358 trace_cnt = backtrace( trace_buffer, MAX_BT ); 00359 cur->trace = backtrace_symbols( trace_buffer, trace_cnt ); 00360 cur->trace_count = trace_cnt; 00361 #endif 00362 00363 if( ( heap.verify & MEMORY_VERIFY_ALLOC ) && verify_chain() != 0 ) 00364 polarssl_exit( 1 ); 00365 00366 return( ( (unsigned char *) cur ) + sizeof(memory_header) ); 00367 } 00368 00369 static void buffer_alloc_free( void *ptr ) 00370 { 00371 memory_header *hdr, *old = NULL; 00372 unsigned char *p = (unsigned char *) ptr; 00373 00374 if( ptr == NULL || heap.buf == NULL || heap.first == NULL ) 00375 return; 00376 00377 if( p < heap.buf || p > heap.buf + heap.len ) 00378 { 00379 #if defined(POLARSSL_MEMORY_DEBUG) 00380 polarssl_fprintf( stderr, "FATAL: polarssl_free() outside of managed " 00381 "space\n" ); 00382 #endif 00383 polarssl_exit( 1 ); 00384 } 00385 00386 p -= sizeof(memory_header); 00387 hdr = (memory_header *) p; 00388 00389 if( verify_header( hdr ) != 0 ) 00390 polarssl_exit( 1 ); 00391 00392 if( hdr->alloc != 1 ) 00393 { 00394 #if defined(POLARSSL_MEMORY_DEBUG) 00395 polarssl_fprintf( stderr, "FATAL: polarssl_free() on unallocated " 00396 "data\n" ); 00397 #endif 00398 polarssl_exit( 1 ); 00399 } 00400 00401 hdr->alloc = 0; 00402 00403 #if defined(POLARSSL_MEMORY_DEBUG) 00404 heap.free_count++; 00405 heap.total_used -= hdr->size; 00406 #endif 00407 00408 // Regroup with block before 00409 // 00410 if( hdr->prev != NULL && hdr->prev->alloc == 0 ) 00411 { 00412 #if defined(POLARSSL_MEMORY_DEBUG) 00413 heap.header_count--; 00414 #endif 00415 hdr->prev->size += sizeof(memory_header) + hdr->size; 00416 hdr->prev->next = hdr->next; 00417 old = hdr; 00418 hdr = hdr->prev; 00419 00420 if( hdr->next != NULL ) 00421 hdr->next->prev = hdr; 00422 00423 #if defined(POLARSSL_MEMORY_BACKTRACE) 00424 free( old->trace ); 00425 #endif 00426 memset( old, 0, sizeof(memory_header) ); 00427 } 00428 00429 // Regroup with block after 00430 // 00431 if( hdr->next != NULL && hdr->next->alloc == 0 ) 00432 { 00433 #if defined(POLARSSL_MEMORY_DEBUG) 00434 heap.header_count--; 00435 #endif 00436 hdr->size += sizeof(memory_header) + hdr->next->size; 00437 old = hdr->next; 00438 hdr->next = hdr->next->next; 00439 00440 if( hdr->prev_free != NULL || hdr->next_free != NULL ) 00441 { 00442 if( hdr->prev_free != NULL ) 00443 hdr->prev_free->next_free = hdr->next_free; 00444 else 00445 heap.first_free = hdr->next_free; 00446 00447 if( hdr->next_free != NULL ) 00448 hdr->next_free->prev_free = hdr->prev_free; 00449 } 00450 00451 hdr->prev_free = old->prev_free; 00452 hdr->next_free = old->next_free; 00453 00454 if( hdr->prev_free != NULL ) 00455 hdr->prev_free->next_free = hdr; 00456 else 00457 heap.first_free = hdr; 00458 00459 if( hdr->next_free != NULL ) 00460 hdr->next_free->prev_free = hdr; 00461 00462 if( hdr->next != NULL ) 00463 hdr->next->prev = hdr; 00464 00465 #if defined(POLARSSL_MEMORY_BACKTRACE) 00466 free( old->trace ); 00467 #endif 00468 memset( old, 0, sizeof(memory_header) ); 00469 } 00470 00471 // Prepend to free_list if we have not merged 00472 // (Does not have to stay in same order as prev / next list) 00473 // 00474 if( old == NULL ) 00475 { 00476 hdr->next_free = heap.first_free; 00477 if( heap.first_free != NULL ) 00478 heap.first_free->prev_free = hdr; 00479 heap.first_free = hdr; 00480 } 00481 00482 #if defined(POLARSSL_MEMORY_BACKTRACE) 00483 hdr->trace = NULL; 00484 hdr->trace_count = 0; 00485 #endif 00486 00487 if( ( heap.verify & MEMORY_VERIFY_FREE ) && verify_chain() != 0 ) 00488 polarssl_exit( 1 ); 00489 } 00490 00491 void memory_buffer_set_verify( int verify ) 00492 { 00493 heap.verify = verify; 00494 } 00495 00496 int memory_buffer_alloc_verify() 00497 { 00498 return verify_chain(); 00499 } 00500 00501 #if defined(POLARSSL_MEMORY_DEBUG) 00502 void memory_buffer_alloc_status() 00503 { 00504 polarssl_fprintf( stderr, 00505 "Current use: %zu blocks / %zu bytes, max: %zu blocks / " 00506 "%zu bytes (total %zu bytes), malloc / free: %zu / %zu\n", 00507 heap.header_count, heap.total_used, 00508 heap.maximum_header_count, heap.maximum_used, 00509 heap.maximum_header_count * sizeof( memory_header ) 00510 + heap.maximum_used, 00511 heap.malloc_count, heap.free_count ); 00512 00513 if( heap.first->next == NULL ) 00514 polarssl_fprintf( stderr, "All memory de-allocated in stack buffer\n" ); 00515 else 00516 { 00517 polarssl_fprintf( stderr, "Memory currently allocated:\n" ); 00518 debug_chain(); 00519 } 00520 } 00521 00522 void memory_buffer_alloc_max_get( size_t *max_used, size_t *max_blocks ) 00523 { 00524 *max_used = heap.maximum_used; 00525 *max_blocks = heap.maximum_header_count; 00526 } 00527 00528 void memory_buffer_alloc_max_reset( void ) 00529 { 00530 heap.maximum_used = 0; 00531 heap.maximum_header_count = 0; 00532 } 00533 00534 void memory_buffer_alloc_cur_get( size_t *cur_used, size_t *cur_blocks ) 00535 { 00536 *cur_used = heap.total_used; 00537 *cur_blocks = heap.header_count; 00538 } 00539 #endif /* POLARSSL_MEMORY_DEBUG */ 00540 00541 #if defined(POLARSSL_THREADING_C) 00542 static void *buffer_alloc_malloc_mutexed( size_t len ) 00543 { 00544 void *buf; 00545 polarssl_mutex_lock( &heap.mutex ); 00546 buf = buffer_alloc_malloc( len ); 00547 polarssl_mutex_unlock( &heap.mutex ); 00548 return( buf ); 00549 } 00550 00551 static void buffer_alloc_free_mutexed( void *ptr ) 00552 { 00553 polarssl_mutex_lock( &heap.mutex ); 00554 buffer_alloc_free( ptr ); 00555 polarssl_mutex_unlock( &heap.mutex ); 00556 } 00557 #endif /* POLARSSL_THREADING_C */ 00558 00559 int memory_buffer_alloc_init( unsigned char *buf, size_t len ) 00560 { 00561 memset( &heap, 0, sizeof(buffer_alloc_ctx) ); 00562 memset( buf, 0, len ); 00563 00564 #if defined(POLARSSL_THREADING_C) 00565 polarssl_mutex_init( &heap.mutex ); 00566 platform_set_malloc_free( buffer_alloc_malloc_mutexed, 00567 buffer_alloc_free_mutexed ); 00568 #else 00569 platform_set_malloc_free( buffer_alloc_malloc, buffer_alloc_free ); 00570 #endif 00571 00572 if( (size_t) buf % POLARSSL_MEMORY_ALIGN_MULTIPLE ) 00573 { 00574 /* Adjust len first since buf is used in the computation */ 00575 len -= POLARSSL_MEMORY_ALIGN_MULTIPLE 00576 - (size_t) buf % POLARSSL_MEMORY_ALIGN_MULTIPLE; 00577 buf += POLARSSL_MEMORY_ALIGN_MULTIPLE 00578 - (size_t) buf % POLARSSL_MEMORY_ALIGN_MULTIPLE; 00579 } 00580 00581 heap.buf = buf; 00582 heap.len = len; 00583 00584 heap.first = (memory_header *) buf; 00585 heap.first->size = len - sizeof(memory_header); 00586 heap.first->magic1 = MAGIC1; 00587 heap.first->magic2 = MAGIC2; 00588 heap.first_free = heap.first; 00589 return( 0 ); 00590 } 00591 00592 void memory_buffer_alloc_free() 00593 { 00594 #if defined(POLARSSL_THREADING_C) 00595 polarssl_mutex_free( &heap.mutex ); 00596 #endif 00597 polarssl_zeroize( &heap, sizeof(buffer_alloc_ctx) ); 00598 } 00599 00600 #if defined(POLARSSL_SELF_TEST) 00601 static int check_pointer( void *p ) 00602 { 00603 if( p == NULL ) 00604 return( -1 ); 00605 00606 if( (size_t) p % POLARSSL_MEMORY_ALIGN_MULTIPLE != 0 ) 00607 return( -1 ); 00608 00609 return( 0 ); 00610 } 00611 00612 static int check_all_free( ) 00613 { 00614 if( 00615 #if defined(POLARSSL_MEMORY_DEBUG) 00616 heap.total_used != 0 || 00617 #endif 00618 heap.first != heap.first_free || 00619 (void *) heap.first != (void *) heap.buf ) 00620 { 00621 return( -1 ); 00622 } 00623 00624 return( 0 ); 00625 } 00626 00627 #define TEST_ASSERT( condition ) \ 00628 if( ! (condition) ) \ 00629 { \ 00630 if( verbose != 0 ) \ 00631 polarssl_printf( "failed\n" ); \ 00632 \ 00633 ret = 1; \ 00634 goto cleanup; \ 00635 } 00636 00637 int memory_buffer_alloc_self_test( int verbose ) 00638 { 00639 unsigned char buf[1024]; 00640 unsigned char *p, *q, *r, *end; 00641 int ret = 0; 00642 00643 if( verbose != 0 ) 00644 polarssl_printf( " MBA test #1 (basic alloc-free cycle): " ); 00645 00646 memory_buffer_alloc_init( buf, sizeof( buf ) ); 00647 00648 p = polarssl_malloc( 1 ); 00649 q = polarssl_malloc( 128 ); 00650 r = polarssl_malloc( 16 ); 00651 00652 TEST_ASSERT( check_pointer( p ) == 0 && 00653 check_pointer( q ) == 0 && 00654 check_pointer( r ) == 0 ); 00655 00656 polarssl_free( r ); 00657 polarssl_free( q ); 00658 polarssl_free( p ); 00659 00660 TEST_ASSERT( check_all_free( ) == 0 ); 00661 00662 /* Memorize end to compare with the next test */ 00663 end = heap.buf + heap.len; 00664 00665 memory_buffer_alloc_free( ); 00666 00667 if( verbose != 0 ) 00668 polarssl_printf( "passed\n" ); 00669 00670 if( verbose != 0 ) 00671 polarssl_printf( " MBA test #2 (buf not aligned): " ); 00672 00673 memory_buffer_alloc_init( buf + 1, sizeof( buf ) - 1 ); 00674 00675 TEST_ASSERT( heap.buf + heap.len == end ); 00676 00677 p = polarssl_malloc( 1 ); 00678 q = polarssl_malloc( 128 ); 00679 r = polarssl_malloc( 16 ); 00680 00681 TEST_ASSERT( check_pointer( p ) == 0 && 00682 check_pointer( q ) == 0 && 00683 check_pointer( r ) == 0 ); 00684 00685 polarssl_free( r ); 00686 polarssl_free( q ); 00687 polarssl_free( p ); 00688 00689 TEST_ASSERT( check_all_free( ) == 0 ); 00690 00691 memory_buffer_alloc_free( ); 00692 00693 if( verbose != 0 ) 00694 polarssl_printf( "passed\n" ); 00695 00696 if( verbose != 0 ) 00697 polarssl_printf( " MBA test #3 (full): " ); 00698 00699 memory_buffer_alloc_init( buf, sizeof( buf ) ); 00700 00701 p = polarssl_malloc( sizeof( buf ) - sizeof( memory_header ) ); 00702 00703 TEST_ASSERT( check_pointer( p ) == 0 ); 00704 TEST_ASSERT( polarssl_malloc( 1 ) == NULL ); 00705 00706 polarssl_free( p ); 00707 00708 p = polarssl_malloc( sizeof( buf ) - 2 * sizeof( memory_header ) - 16 ); 00709 q = polarssl_malloc( 16 ); 00710 00711 TEST_ASSERT( check_pointer( p ) == 0 && check_pointer( q ) == 0 ); 00712 TEST_ASSERT( polarssl_malloc( 1 ) == NULL ); 00713 00714 polarssl_free( q ); 00715 00716 TEST_ASSERT( polarssl_malloc( 17 ) == NULL ); 00717 00718 polarssl_free( p ); 00719 00720 TEST_ASSERT( check_all_free( ) == 0 ); 00721 00722 memory_buffer_alloc_free( ); 00723 00724 if( verbose != 0 ) 00725 polarssl_printf( "passed\n" ); 00726 00727 cleanup: 00728 memory_buffer_alloc_free( ); 00729 00730 return( ret ); 00731 } 00732 #endif /* POLARSSL_SELF_TEST */ 00733 00734 #endif /* POLARSSL_MEMORY_BUFFER_ALLOC_C */ 00735
Generated on Tue Jul 12 2022 13:50:37 by
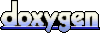