mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
md2.h
00001 /** 00002 * \file md2.h 00003 * 00004 * \brief MD2 message digest algorithm (hash function) 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_MD2_H 00025 #define POLARSSL_MD2_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #define POLARSSL_ERR_MD2_FILE_IO_ERROR -0x0070 /**< Read/write error in file. */ 00036 00037 #if !defined(POLARSSL_MD2_ALT) 00038 // Regular implementation 00039 // 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /** 00046 * \brief MD2 context structure 00047 */ 00048 typedef struct 00049 { 00050 unsigned char cksum[16]; /*!< checksum of the data block */ 00051 unsigned char state[48]; /*!< intermediate digest state */ 00052 unsigned char buffer[16]; /*!< data block being processed */ 00053 00054 unsigned char ipad[16]; /*!< HMAC: inner padding */ 00055 unsigned char opad[16]; /*!< HMAC: outer padding */ 00056 size_t left ; /*!< amount of data in buffer */ 00057 } 00058 md2_context; 00059 00060 /** 00061 * \brief Initialize MD2 context 00062 * 00063 * \param ctx MD2 context to be initialized 00064 */ 00065 void md2_init( md2_context *ctx ); 00066 00067 /** 00068 * \brief Clear MD2 context 00069 * 00070 * \param ctx MD2 context to be cleared 00071 */ 00072 void md2_free( md2_context *ctx ); 00073 00074 /** 00075 * \brief MD2 context setup 00076 * 00077 * \param ctx context to be initialized 00078 */ 00079 void md2_starts( md2_context *ctx ); 00080 00081 /** 00082 * \brief MD2 process buffer 00083 * 00084 * \param ctx MD2 context 00085 * \param input buffer holding the data 00086 * \param ilen length of the input data 00087 */ 00088 void md2_update( md2_context *ctx, const unsigned char *input, size_t ilen ); 00089 00090 /** 00091 * \brief MD2 final digest 00092 * 00093 * \param ctx MD2 context 00094 * \param output MD2 checksum result 00095 */ 00096 void md2_finish( md2_context *ctx, unsigned char output[16] ); 00097 00098 #ifdef __cplusplus 00099 } 00100 #endif 00101 00102 #else /* POLARSSL_MD2_ALT */ 00103 #include "md2_alt.h" 00104 #endif /* POLARSSL_MD2_ALT */ 00105 00106 #ifdef __cplusplus 00107 extern "C" { 00108 #endif 00109 00110 /** 00111 * \brief Output = MD2( input buffer ) 00112 * 00113 * \param input buffer holding the data 00114 * \param ilen length of the input data 00115 * \param output MD2 checksum result 00116 */ 00117 void md2( const unsigned char *input, size_t ilen, unsigned char output[16] ); 00118 00119 /** 00120 * \brief Output = MD2( file contents ) 00121 * 00122 * \param path input file name 00123 * \param output MD2 checksum result 00124 * 00125 * \return 0 if successful, or POLARSSL_ERR_MD2_FILE_IO_ERROR 00126 */ 00127 int md2_file( const char *path, unsigned char output[16] ); 00128 00129 /** 00130 * \brief MD2 HMAC context setup 00131 * 00132 * \param ctx HMAC context to be initialized 00133 * \param key HMAC secret key 00134 * \param keylen length of the HMAC key 00135 */ 00136 void md2_hmac_starts( md2_context *ctx, const unsigned char *key, 00137 size_t keylen ); 00138 00139 /** 00140 * \brief MD2 HMAC process buffer 00141 * 00142 * \param ctx HMAC context 00143 * \param input buffer holding the data 00144 * \param ilen length of the input data 00145 */ 00146 void md2_hmac_update( md2_context *ctx, const unsigned char *input, 00147 size_t ilen ); 00148 00149 /** 00150 * \brief MD2 HMAC final digest 00151 * 00152 * \param ctx HMAC context 00153 * \param output MD2 HMAC checksum result 00154 */ 00155 void md2_hmac_finish( md2_context *ctx, unsigned char output[16] ); 00156 00157 /** 00158 * \brief MD2 HMAC context reset 00159 * 00160 * \param ctx HMAC context to be reset 00161 */ 00162 void md2_hmac_reset( md2_context *ctx ); 00163 00164 /** 00165 * \brief Output = HMAC-MD2( hmac key, input buffer ) 00166 * 00167 * \param key HMAC secret key 00168 * \param keylen length of the HMAC key 00169 * \param input buffer holding the data 00170 * \param ilen length of the input data 00171 * \param output HMAC-MD2 result 00172 */ 00173 void md2_hmac( const unsigned char *key, size_t keylen, 00174 const unsigned char *input, size_t ilen, 00175 unsigned char output[16] ); 00176 00177 /** 00178 * \brief Checkup routine 00179 * 00180 * \return 0 if successful, or 1 if the test failed 00181 */ 00182 int md2_self_test( int verbose ); 00183 00184 /* Internal use */ 00185 void md2_process( md2_context *ctx ); 00186 00187 #ifdef __cplusplus 00188 } 00189 #endif 00190 00191 #endif /* md2.h */ 00192
Generated on Tue Jul 12 2022 13:50:37 by
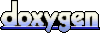