mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
gcm.h
00001 /** 00002 * \file gcm.h 00003 * 00004 * \brief Galois/Counter mode for 128-bit block ciphers 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_GCM_H 00025 #define POLARSSL_GCM_H 00026 00027 #include "cipher.h" 00028 00029 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00030 #include <basetsd.h> 00031 typedef UINT32 uint32_t; 00032 typedef UINT64 uint64_t; 00033 #else 00034 #include <stdint.h> 00035 #endif 00036 00037 #define GCM_ENCRYPT 1 00038 #define GCM_DECRYPT 0 00039 00040 #define POLARSSL_ERR_GCM_AUTH_FAILED -0x0012 /**< Authenticated decryption failed. */ 00041 #define POLARSSL_ERR_GCM_BAD_INPUT -0x0014 /**< Bad input parameters to function. */ 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /** 00048 * \brief GCM context structure 00049 */ 00050 typedef struct { 00051 cipher_context_t cipher_ctx ;/*!< cipher context used */ 00052 uint64_t HL[16]; /*!< Precalculated HTable */ 00053 uint64_t HH[16]; /*!< Precalculated HTable */ 00054 uint64_t len ; /*!< Total data length */ 00055 uint64_t add_len ; /*!< Total add length */ 00056 unsigned char base_ectr[16];/*!< First ECTR for tag */ 00057 unsigned char y[16]; /*!< Y working value */ 00058 unsigned char buf[16]; /*!< buf working value */ 00059 int mode ; /*!< Encrypt or Decrypt */ 00060 } 00061 gcm_context; 00062 00063 /** 00064 * \brief GCM initialization (encryption) 00065 * 00066 * \param ctx GCM context to be initialized 00067 * \param cipher cipher to use (a 128-bit block cipher) 00068 * \param key encryption key 00069 * \param keysize must be 128, 192 or 256 00070 * 00071 * \return 0 if successful, or a cipher specific error code 00072 */ 00073 int gcm_init( gcm_context *ctx, cipher_id_t cipher, const unsigned char *key, 00074 unsigned int keysize ); 00075 00076 /** 00077 * \brief GCM buffer encryption/decryption using a block cipher 00078 * 00079 * \note On encryption, the output buffer can be the same as the input buffer. 00080 * On decryption, the output buffer cannot be the same as input buffer. 00081 * If buffers overlap, the output buffer must trail at least 8 bytes 00082 * behind the input buffer. 00083 * 00084 * \param ctx GCM context 00085 * \param mode GCM_ENCRYPT or GCM_DECRYPT 00086 * \param length length of the input data 00087 * \param iv initialization vector 00088 * \param iv_len length of IV 00089 * \param add additional data 00090 * \param add_len length of additional data 00091 * \param input buffer holding the input data 00092 * \param output buffer for holding the output data 00093 * \param tag_len length of the tag to generate 00094 * \param tag buffer for holding the tag 00095 * 00096 * \return 0 if successful 00097 */ 00098 int gcm_crypt_and_tag( gcm_context *ctx, 00099 int mode, 00100 size_t length, 00101 const unsigned char *iv, 00102 size_t iv_len, 00103 const unsigned char *add, 00104 size_t add_len, 00105 const unsigned char *input, 00106 unsigned char *output, 00107 size_t tag_len, 00108 unsigned char *tag ); 00109 00110 /** 00111 * \brief GCM buffer authenticated decryption using a block cipher 00112 * 00113 * \note On decryption, the output buffer cannot be the same as input buffer. 00114 * If buffers overlap, the output buffer must trail at least 8 bytes 00115 * behind the input buffer. 00116 * 00117 * \param ctx GCM context 00118 * \param length length of the input data 00119 * \param iv initialization vector 00120 * \param iv_len length of IV 00121 * \param add additional data 00122 * \param add_len length of additional data 00123 * \param tag buffer holding the tag 00124 * \param tag_len length of the tag 00125 * \param input buffer holding the input data 00126 * \param output buffer for holding the output data 00127 * 00128 * \return 0 if successful and authenticated, 00129 * POLARSSL_ERR_GCM_AUTH_FAILED if tag does not match 00130 */ 00131 int gcm_auth_decrypt( gcm_context *ctx, 00132 size_t length, 00133 const unsigned char *iv, 00134 size_t iv_len, 00135 const unsigned char *add, 00136 size_t add_len, 00137 const unsigned char *tag, 00138 size_t tag_len, 00139 const unsigned char *input, 00140 unsigned char *output ); 00141 00142 /** 00143 * \brief Generic GCM stream start function 00144 * 00145 * \param ctx GCM context 00146 * \param mode GCM_ENCRYPT or GCM_DECRYPT 00147 * \param iv initialization vector 00148 * \param iv_len length of IV 00149 * \param add additional data (or NULL if length is 0) 00150 * \param add_len length of additional data 00151 * 00152 * \return 0 if successful 00153 */ 00154 int gcm_starts( gcm_context *ctx, 00155 int mode, 00156 const unsigned char *iv, 00157 size_t iv_len, 00158 const unsigned char *add, 00159 size_t add_len ); 00160 00161 /** 00162 * \brief Generic GCM update function. Encrypts/decrypts using the 00163 * given GCM context. Expects input to be a multiple of 16 00164 * bytes! Only the last call before gcm_finish() can be less 00165 * than 16 bytes! 00166 * 00167 * \note On decryption, the output buffer cannot be the same as input buffer. 00168 * If buffers overlap, the output buffer must trail at least 8 bytes 00169 * behind the input buffer. 00170 * 00171 * \param ctx GCM context 00172 * \param length length of the input data 00173 * \param input buffer holding the input data 00174 * \param output buffer for holding the output data 00175 * 00176 * \return 0 if successful or POLARSSL_ERR_GCM_BAD_INPUT 00177 */ 00178 int gcm_update( gcm_context *ctx, 00179 size_t length, 00180 const unsigned char *input, 00181 unsigned char *output ); 00182 00183 /** 00184 * \brief Generic GCM finalisation function. Wraps up the GCM stream 00185 * and generates the tag. The tag can have a maximum length of 00186 * 16 bytes. 00187 * 00188 * \param ctx GCM context 00189 * \param tag buffer for holding the tag (may be NULL if tag_len is 0) 00190 * \param tag_len length of the tag to generate 00191 * 00192 * \return 0 if successful or POLARSSL_ERR_GCM_BAD_INPUT 00193 */ 00194 int gcm_finish( gcm_context *ctx, 00195 unsigned char *tag, 00196 size_t tag_len ); 00197 00198 /** 00199 * \brief Free a GCM context and underlying cipher sub-context 00200 * 00201 * \param ctx GCM context to free 00202 */ 00203 void gcm_free( gcm_context *ctx ); 00204 00205 /** 00206 * \brief Checkup routine 00207 * 00208 * \return 0 if successful, or 1 if the test failed 00209 */ 00210 int gcm_self_test( int verbose ); 00211 00212 #ifdef __cplusplus 00213 } 00214 #endif 00215 00216 #endif /* gcm.h */ 00217
Generated on Tue Jul 12 2022 13:50:37 by
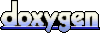