mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
error.h
00001 /** 00002 * \file error.h 00003 * 00004 * \brief Error to string translation 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ERROR_H 00025 #define POLARSSL_ERROR_H 00026 00027 #include <stddef.h> 00028 00029 /** 00030 * Error code layout. 00031 * 00032 * Currently we try to keep all error codes within the negative space of 16 00033 * bytes signed integers to support all platforms (-0x0000 - -0x8000). In 00034 * addition we'd like to give two layers of information on the error if 00035 * possible. 00036 * 00037 * For that purpose the error codes are segmented in the following manner: 00038 * 00039 * 16 bit error code bit-segmentation 00040 * 00041 * 1 bit - Sign bit 00042 * 3 bits - High level module ID 00043 * 5 bits - Module-dependent error code 00044 * 7 bits - Low level module errors 00045 * 00046 * For historical reasons, low-level error codes are divided in even and odd, 00047 * even codes were assigned first, and -1 is reserved for other errors. 00048 * 00049 * Low-level module errors (0x0002-0x007E, 0x0003-0x007F) 00050 * 00051 * Module Nr Codes assigned 00052 * MPI 7 0x0002-0x0010 00053 * GCM 2 0x0012-0x0014 00054 * BLOWFISH 2 0x0016-0x0018 00055 * THREADING 3 0x001A-0x001E 00056 * AES 2 0x0020-0x0022 00057 * CAMELLIA 2 0x0024-0x0026 00058 * XTEA 1 0x0028-0x0028 00059 * BASE64 2 0x002A-0x002C 00060 * OID 1 0x002E-0x002E 0x000B-0x000B 00061 * PADLOCK 1 0x0030-0x0030 00062 * DES 1 0x0032-0x0032 00063 * CTR_DBRG 4 0x0034-0x003A 00064 * ENTROPY 3 0x003C-0x0040 00065 * NET 11 0x0042-0x0056 00066 * ENTROPY 1 0x0058-0x0058 00067 * ASN1 7 0x0060-0x006C 00068 * MD2 1 0x0070-0x0070 00069 * MD4 1 0x0072-0x0072 00070 * MD5 1 0x0074-0x0074 00071 * SHA1 1 0x0076-0x0076 00072 * SHA256 1 0x0078-0x0078 00073 * SHA512 1 0x007A-0x007A 00074 * PBKDF2 1 0x007C-0x007C 00075 * RIPEMD160 1 0x007E-0x007E 00076 * HMAC_DRBG 4 0x0003-0x0009 00077 * CCM 2 0x000D-0x000F 00078 * 00079 * High-level module nr (3 bits - 0x0...-0x7...) 00080 * Name ID Nr of Errors 00081 * PEM 1 9 00082 * PKCS#12 1 4 (Started from top) 00083 * X509 2 18 00084 * PK 2 14 (Started from top, plus 0x2000) 00085 * DHM 3 9 00086 * PKCS5 3 4 (Started from top) 00087 * RSA 4 9 00088 * ECP 4 8 (Started from top) 00089 * MD 5 4 00090 * CIPHER 6 6 00091 * SSL 6 11 (Started from top) 00092 * SSL 7 31 00093 * 00094 * Module dependent error code (5 bits 0x.00.-0x.F8.) 00095 */ 00096 00097 #ifdef __cplusplus 00098 extern "C" { 00099 #endif 00100 00101 /** 00102 * \brief Translate a mbed TLS error code into a string representation, 00103 * Result is truncated if necessary and always includes a terminating 00104 * null byte. 00105 * 00106 * \param errnum error code 00107 * \param buffer buffer to place representation in 00108 * \param buflen length of the buffer 00109 */ 00110 void polarssl_strerror( int errnum, char *buffer, size_t buflen ); 00111 00112 #if defined(POLARSSL_ERROR_STRERROR_BC) 00113 void error_strerror( int errnum, char *buffer, size_t buflen ); 00114 #endif 00115 00116 #ifdef __cplusplus 00117 } 00118 #endif 00119 00120 #endif /* error.h */ 00121
Generated on Tue Jul 12 2022 13:50:37 by
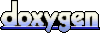