mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
entropy.h
00001 /** 00002 * \file entropy.h 00003 * 00004 * \brief Entropy accumulator implementation 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ENTROPY_H 00025 #define POLARSSL_ENTROPY_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(POLARSSL_SHA512_C) && !defined(POLARSSL_ENTROPY_FORCE_SHA256) 00036 #include "sha512.h" 00037 #define POLARSSL_ENTROPY_SHA512_ACCUMULATOR 00038 #else 00039 #if defined(POLARSSL_SHA256_C) 00040 #define POLARSSL_ENTROPY_SHA256_ACCUMULATOR 00041 #include "sha256.h" 00042 #endif 00043 #endif 00044 00045 #if defined(POLARSSL_THREADING_C) 00046 #include "threading.h" 00047 #endif 00048 00049 #if defined(POLARSSL_HAVEGE_C) 00050 #include "havege.h" 00051 #endif 00052 00053 #define POLARSSL_ERR_ENTROPY_SOURCE_FAILED -0x003C /**< Critical entropy source failure. */ 00054 #define POLARSSL_ERR_ENTROPY_MAX_SOURCES -0x003E /**< No more sources can be added. */ 00055 #define POLARSSL_ERR_ENTROPY_NO_SOURCES_DEFINED -0x0040 /**< No sources have been added to poll. */ 00056 #define POLARSSL_ERR_ENTROPY_FILE_IO_ERROR -0x0058 /**< Read/write error in file. */ 00057 00058 /** 00059 * \name SECTION: Module settings 00060 * 00061 * The configuration options you can set for this module are in this section. 00062 * Either change them in config.h or define them on the compiler command line. 00063 * \{ 00064 */ 00065 00066 #if !defined(ENTROPY_MAX_SOURCES) 00067 #define ENTROPY_MAX_SOURCES 20 /**< Maximum number of sources supported */ 00068 #endif 00069 00070 #if !defined(ENTROPY_MAX_GATHER) 00071 #define ENTROPY_MAX_GATHER 128 /**< Maximum amount requested from entropy sources */ 00072 #endif 00073 00074 /* \} name SECTION: Module settings */ 00075 00076 #if defined(POLARSSL_ENTROPY_SHA512_ACCUMULATOR) 00077 #define ENTROPY_BLOCK_SIZE 64 /**< Block size of entropy accumulator (SHA-512) */ 00078 #else 00079 #define ENTROPY_BLOCK_SIZE 32 /**< Block size of entropy accumulator (SHA-256) */ 00080 #endif 00081 00082 #define ENTROPY_MAX_SEED_SIZE 1024 /**< Maximum size of seed we read from seed file */ 00083 #define ENTROPY_SOURCE_MANUAL ENTROPY_MAX_SOURCES 00084 00085 #ifdef __cplusplus 00086 extern "C" { 00087 #endif 00088 00089 /** 00090 * \brief Entropy poll callback pointer 00091 * 00092 * \param data Callback-specific data pointer 00093 * \param output Data to fill 00094 * \param len Maximum size to provide 00095 * \param olen The actual amount of bytes put into the buffer (Can be 0) 00096 * 00097 * \return 0 if no critical failures occurred, 00098 * POLARSSL_ERR_ENTROPY_SOURCE_FAILED otherwise 00099 */ 00100 typedef int (*f_source_ptr)(void *data, unsigned char *output, size_t len, 00101 size_t *olen); 00102 00103 /** 00104 * \brief Entropy source state 00105 */ 00106 typedef struct 00107 { 00108 f_source_ptr f_source; /**< The entropy source callback */ 00109 void * p_source; /**< The callback data pointer */ 00110 size_t size; /**< Amount received */ 00111 size_t threshold; /**< Minimum level required before release */ 00112 } 00113 source_state; 00114 00115 /** 00116 * \brief Entropy context structure 00117 */ 00118 typedef struct 00119 { 00120 #if defined(POLARSSL_ENTROPY_SHA512_ACCUMULATOR) 00121 sha512_context accumulator; 00122 #else 00123 sha256_context accumulator; 00124 #endif 00125 int source_count; 00126 source_state source[ENTROPY_MAX_SOURCES]; 00127 #if defined(POLARSSL_HAVEGE_C) 00128 havege_state havege_data; 00129 #endif 00130 #if defined(POLARSSL_THREADING_C) 00131 threading_mutex_t mutex ; /*!< mutex */ 00132 #endif 00133 } 00134 entropy_context; 00135 00136 /** 00137 * \brief Initialize the context 00138 * 00139 * \param ctx Entropy context to initialize 00140 */ 00141 void entropy_init( entropy_context *ctx ); 00142 00143 /** 00144 * \brief Free the data in the context 00145 * 00146 * \param ctx Entropy context to free 00147 */ 00148 void entropy_free( entropy_context *ctx ); 00149 00150 /** 00151 * \brief Adds an entropy source to poll 00152 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00153 * 00154 * \param ctx Entropy context 00155 * \param f_source Entropy function 00156 * \param p_source Function data 00157 * \param threshold Minimum required from source before entropy is released 00158 * ( with entropy_func() ) 00159 * 00160 * \return 0 if successful or POLARSSL_ERR_ENTROPY_MAX_SOURCES 00161 */ 00162 int entropy_add_source( entropy_context *ctx, 00163 f_source_ptr f_source, void *p_source, 00164 size_t threshold ); 00165 00166 /** 00167 * \brief Trigger an extra gather poll for the accumulator 00168 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00169 * 00170 * \param ctx Entropy context 00171 * 00172 * \return 0 if successful, or POLARSSL_ERR_ENTROPY_SOURCE_FAILED 00173 */ 00174 int entropy_gather( entropy_context *ctx ); 00175 00176 /** 00177 * \brief Retrieve entropy from the accumulator 00178 * (Maximum length: ENTROPY_BLOCK_SIZE) 00179 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00180 * 00181 * \param data Entropy context 00182 * \param output Buffer to fill 00183 * \param len Number of bytes desired, must be at most ENTROPY_BLOCK_SIZE 00184 * 00185 * \return 0 if successful, or POLARSSL_ERR_ENTROPY_SOURCE_FAILED 00186 */ 00187 int entropy_func( void *data, unsigned char *output, size_t len ); 00188 00189 /** 00190 * \brief Add data to the accumulator manually 00191 * (Thread-safe if POLARSSL_THREADING_C is enabled) 00192 * 00193 * \param ctx Entropy context 00194 * \param data Data to add 00195 * \param len Length of data 00196 * 00197 * \return 0 if successful 00198 */ 00199 int entropy_update_manual( entropy_context *ctx, 00200 const unsigned char *data, size_t len ); 00201 00202 #if defined(POLARSSL_FS_IO) 00203 /** 00204 * \brief Write a seed file 00205 * 00206 * \param ctx Entropy context 00207 * \param path Name of the file 00208 * 00209 * \return 0 if successful, 00210 * POLARSSL_ERR_ENTROPY_FILE_IO_ERROR on file error, or 00211 * POLARSSL_ERR_ENTROPY_SOURCE_FAILED 00212 */ 00213 int entropy_write_seed_file( entropy_context *ctx, const char *path ); 00214 00215 /** 00216 * \brief Read and update a seed file. Seed is added to this 00217 * instance. No more than ENTROPY_MAX_SEED_SIZE bytes are 00218 * read from the seed file. The rest is ignored. 00219 * 00220 * \param ctx Entropy context 00221 * \param path Name of the file 00222 * 00223 * \return 0 if successful, 00224 * POLARSSL_ERR_ENTROPY_FILE_IO_ERROR on file error, 00225 * POLARSSL_ERR_ENTROPY_SOURCE_FAILED 00226 */ 00227 int entropy_update_seed_file( entropy_context *ctx, const char *path ); 00228 #endif /* POLARSSL_FS_IO */ 00229 00230 #if defined(POLARSSL_SELF_TEST) 00231 /** 00232 * \brief Checkup routine 00233 * 00234 * \return 0 if successful, or 1 if a test failed 00235 */ 00236 int entropy_self_test( int verbose ); 00237 #endif /* POLARSSL_SELF_TEST */ 00238 00239 #ifdef __cplusplus 00240 } 00241 #endif 00242 00243 #endif /* entropy.h */ 00244
Generated on Tue Jul 12 2022 13:50:37 by
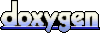