mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
des.h
00001 /** 00002 * \file des.h 00003 * 00004 * \brief DES block cipher 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_DES_H 00025 #define POLARSSL_DES_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 #define DES_ENCRYPT 1 00043 #define DES_DECRYPT 0 00044 00045 #define POLARSSL_ERR_DES_INVALID_INPUT_LENGTH -0x0032 /**< The data input has an invalid length. */ 00046 00047 #define DES_KEY_SIZE 8 00048 00049 #if !defined(POLARSSL_DES_ALT) 00050 // Regular implementation 00051 // 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /** 00058 * \brief DES context structure 00059 */ 00060 typedef struct 00061 { 00062 int mode ; /*!< encrypt/decrypt */ 00063 uint32_t sk[32]; /*!< DES subkeys */ 00064 } 00065 des_context; 00066 00067 /** 00068 * \brief Triple-DES context structure 00069 */ 00070 typedef struct 00071 { 00072 int mode ; /*!< encrypt/decrypt */ 00073 uint32_t sk[96]; /*!< 3DES subkeys */ 00074 } 00075 des3_context; 00076 00077 /** 00078 * \brief Initialize DES context 00079 * 00080 * \param ctx DES context to be initialized 00081 */ 00082 void des_init( des_context *ctx ); 00083 00084 /** 00085 * \brief Clear DES context 00086 * 00087 * \param ctx DES context to be cleared 00088 */ 00089 void des_free( des_context *ctx ); 00090 00091 /** 00092 * \brief Initialize Triple-DES context 00093 * 00094 * \param ctx DES3 context to be initialized 00095 */ 00096 void des3_init( des3_context *ctx ); 00097 00098 /** 00099 * \brief Clear Triple-DES context 00100 * 00101 * \param ctx DES3 context to be cleared 00102 */ 00103 void des3_free( des3_context *ctx ); 00104 00105 /** 00106 * \brief Set key parity on the given key to odd. 00107 * 00108 * DES keys are 56 bits long, but each byte is padded with 00109 * a parity bit to allow verification. 00110 * 00111 * \param key 8-byte secret key 00112 */ 00113 void des_key_set_parity( unsigned char key[DES_KEY_SIZE] ); 00114 00115 /** 00116 * \brief Check that key parity on the given key is odd. 00117 * 00118 * DES keys are 56 bits long, but each byte is padded with 00119 * a parity bit to allow verification. 00120 * 00121 * \param key 8-byte secret key 00122 * 00123 * \return 0 is parity was ok, 1 if parity was not correct. 00124 */ 00125 int des_key_check_key_parity( const unsigned char key[DES_KEY_SIZE] ); 00126 00127 /** 00128 * \brief Check that key is not a weak or semi-weak DES key 00129 * 00130 * \param key 8-byte secret key 00131 * 00132 * \return 0 if no weak key was found, 1 if a weak key was identified. 00133 */ 00134 int des_key_check_weak( const unsigned char key[DES_KEY_SIZE] ); 00135 00136 /** 00137 * \brief DES key schedule (56-bit, encryption) 00138 * 00139 * \param ctx DES context to be initialized 00140 * \param key 8-byte secret key 00141 * 00142 * \return 0 00143 */ 00144 int des_setkey_enc( des_context *ctx, const unsigned char key[DES_KEY_SIZE] ); 00145 00146 /** 00147 * \brief DES key schedule (56-bit, decryption) 00148 * 00149 * \param ctx DES context to be initialized 00150 * \param key 8-byte secret key 00151 * 00152 * \return 0 00153 */ 00154 int des_setkey_dec( des_context *ctx, const unsigned char key[DES_KEY_SIZE] ); 00155 00156 /** 00157 * \brief Triple-DES key schedule (112-bit, encryption) 00158 * 00159 * \param ctx 3DES context to be initialized 00160 * \param key 16-byte secret key 00161 * 00162 * \return 0 00163 */ 00164 int des3_set2key_enc( des3_context *ctx, 00165 const unsigned char key[DES_KEY_SIZE * 2] ); 00166 00167 /** 00168 * \brief Triple-DES key schedule (112-bit, decryption) 00169 * 00170 * \param ctx 3DES context to be initialized 00171 * \param key 16-byte secret key 00172 * 00173 * \return 0 00174 */ 00175 int des3_set2key_dec( des3_context *ctx, 00176 const unsigned char key[DES_KEY_SIZE * 2] ); 00177 00178 /** 00179 * \brief Triple-DES key schedule (168-bit, encryption) 00180 * 00181 * \param ctx 3DES context to be initialized 00182 * \param key 24-byte secret key 00183 * 00184 * \return 0 00185 */ 00186 int des3_set3key_enc( des3_context *ctx, 00187 const unsigned char key[DES_KEY_SIZE * 3] ); 00188 00189 /** 00190 * \brief Triple-DES key schedule (168-bit, decryption) 00191 * 00192 * \param ctx 3DES context to be initialized 00193 * \param key 24-byte secret key 00194 * 00195 * \return 0 00196 */ 00197 int des3_set3key_dec( des3_context *ctx, 00198 const unsigned char key[DES_KEY_SIZE * 3] ); 00199 00200 /** 00201 * \brief DES-ECB block encryption/decryption 00202 * 00203 * \param ctx DES context 00204 * \param input 64-bit input block 00205 * \param output 64-bit output block 00206 * 00207 * \return 0 if successful 00208 */ 00209 int des_crypt_ecb( des_context *ctx, 00210 const unsigned char input[8], 00211 unsigned char output[8] ); 00212 00213 #if defined(POLARSSL_CIPHER_MODE_CBC) 00214 /** 00215 * \brief DES-CBC buffer encryption/decryption 00216 * 00217 * \note Upon exit, the content of the IV is updated so that you can 00218 * call the function same function again on the following 00219 * block(s) of data and get the same result as if it was 00220 * encrypted in one call. This allows a "streaming" usage. 00221 * If on the other hand you need to retain the contents of the 00222 * IV, you should either save it manually or use the cipher 00223 * module instead. 00224 * 00225 * \param ctx DES context 00226 * \param mode DES_ENCRYPT or DES_DECRYPT 00227 * \param length length of the input data 00228 * \param iv initialization vector (updated after use) 00229 * \param input buffer holding the input data 00230 * \param output buffer holding the output data 00231 */ 00232 int des_crypt_cbc( des_context *ctx, 00233 int mode, 00234 size_t length, 00235 unsigned char iv[8], 00236 const unsigned char *input, 00237 unsigned char *output ); 00238 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00239 00240 /** 00241 * \brief 3DES-ECB block encryption/decryption 00242 * 00243 * \param ctx 3DES context 00244 * \param input 64-bit input block 00245 * \param output 64-bit output block 00246 * 00247 * \return 0 if successful 00248 */ 00249 int des3_crypt_ecb( des3_context *ctx, 00250 const unsigned char input[8], 00251 unsigned char output[8] ); 00252 00253 #if defined(POLARSSL_CIPHER_MODE_CBC) 00254 /** 00255 * \brief 3DES-CBC buffer encryption/decryption 00256 * 00257 * \note Upon exit, the content of the IV is updated so that you can 00258 * call the function same function again on the following 00259 * block(s) of data and get the same result as if it was 00260 * encrypted in one call. This allows a "streaming" usage. 00261 * If on the other hand you need to retain the contents of the 00262 * IV, you should either save it manually or use the cipher 00263 * module instead. 00264 * 00265 * \param ctx 3DES context 00266 * \param mode DES_ENCRYPT or DES_DECRYPT 00267 * \param length length of the input data 00268 * \param iv initialization vector (updated after use) 00269 * \param input buffer holding the input data 00270 * \param output buffer holding the output data 00271 * 00272 * \return 0 if successful, or POLARSSL_ERR_DES_INVALID_INPUT_LENGTH 00273 */ 00274 int des3_crypt_cbc( des3_context *ctx, 00275 int mode, 00276 size_t length, 00277 unsigned char iv[8], 00278 const unsigned char *input, 00279 unsigned char *output ); 00280 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00281 00282 #ifdef __cplusplus 00283 } 00284 #endif 00285 00286 #else /* POLARSSL_DES_ALT */ 00287 #include "des_alt.h" 00288 #endif /* POLARSSL_DES_ALT */ 00289 00290 #ifdef __cplusplus 00291 extern "C" { 00292 #endif 00293 00294 /** 00295 * \brief Checkup routine 00296 * 00297 * \return 0 if successful, or 1 if the test failed 00298 */ 00299 int des_self_test( int verbose ); 00300 00301 #ifdef __cplusplus 00302 } 00303 #endif 00304 00305 #endif /* des.h */ 00306
Generated on Tue Jul 12 2022 13:50:37 by
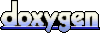