mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
debug.c
00001 /* 00002 * Debugging routines 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 #if !defined(POLARSSL_CONFIG_FILE) 00024 #include "polarssl/config.h" 00025 #else 00026 #include POLARSSL_CONFIG_FILE 00027 #endif 00028 00029 #if defined(POLARSSL_DEBUG_C) 00030 00031 #include "polarssl/debug.h" 00032 00033 #include <stdarg.h> 00034 #include <stdio.h> 00035 #include <string.h> 00036 00037 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00038 #if !defined snprintf 00039 #define snprintf _snprintf 00040 #endif 00041 00042 #if !defined vsnprintf 00043 #define vsnprintf _vsnprintf 00044 #endif 00045 #endif /* _MSC_VER */ 00046 00047 #if defined(POLARSSL_PLATFORM_C) 00048 #include "polarssl/platform.h" 00049 #else 00050 #define polarssl_snprintf snprintf 00051 #endif 00052 00053 static int debug_log_mode = POLARSSL_DEBUG_DFL_MODE; 00054 static int debug_threshold = 0; 00055 00056 void debug_set_log_mode( int log_mode ) 00057 { 00058 debug_log_mode = log_mode; 00059 } 00060 00061 void debug_set_threshold( int threshold ) 00062 { 00063 debug_threshold = threshold; 00064 } 00065 00066 char *debug_fmt( const char *format, ... ) 00067 { 00068 va_list argp; 00069 static char str[512]; 00070 int maxlen = sizeof( str ) - 1; 00071 00072 va_start( argp, format ); 00073 vsnprintf( str, maxlen, format, argp ); 00074 va_end( argp ); 00075 00076 str[maxlen] = '\0'; 00077 return( str ); 00078 } 00079 00080 void debug_print_msg( const ssl_context *ssl, int level, 00081 const char *file, int line, const char *text ) 00082 { 00083 char str[512]; 00084 int maxlen = sizeof( str ) - 1; 00085 00086 if( ssl->f_dbg == NULL || level > debug_threshold ) 00087 return; 00088 00089 if( debug_log_mode == POLARSSL_DEBUG_LOG_RAW ) 00090 { 00091 ssl->f_dbg( ssl->p_dbg, level, text ); 00092 return; 00093 } 00094 00095 polarssl_snprintf( str, maxlen, "%s(%04d): %s\n", file, line, text ); 00096 str[maxlen] = '\0'; 00097 ssl->f_dbg( ssl->p_dbg, level, str ); 00098 } 00099 00100 void debug_print_ret( const ssl_context *ssl, int level, 00101 const char *file, int line, 00102 const char *text, int ret ) 00103 { 00104 char str[512]; 00105 int maxlen = sizeof( str ) - 1; 00106 size_t idx = 0; 00107 00108 if( ssl->f_dbg == NULL || level > debug_threshold ) 00109 return; 00110 00111 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00112 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00113 00114 polarssl_snprintf( str + idx, maxlen - idx, "%s() returned %d (-0x%04x)\n", 00115 text, ret, -ret ); 00116 00117 str[maxlen] = '\0'; 00118 ssl->f_dbg( ssl->p_dbg, level, str ); 00119 } 00120 00121 void debug_print_buf( const ssl_context *ssl, int level, 00122 const char *file, int line, const char *text, 00123 unsigned char *buf, size_t len ) 00124 { 00125 char str[512]; 00126 char txt[17]; 00127 size_t i, maxlen = sizeof( str ) - 1, idx = 0; 00128 00129 if( ssl->f_dbg == NULL || level > debug_threshold ) 00130 return; 00131 00132 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00133 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00134 00135 polarssl_snprintf( str + idx, maxlen - idx, "dumping '%s' (%u bytes)\n", 00136 text, (unsigned int) len ); 00137 00138 str[maxlen] = '\0'; 00139 ssl->f_dbg( ssl->p_dbg, level, str ); 00140 00141 idx = 0; 00142 memset( txt, 0, sizeof( txt ) ); 00143 for( i = 0; i < len; i++ ) 00144 { 00145 if( i >= 4096 ) 00146 break; 00147 00148 if( i % 16 == 0 ) 00149 { 00150 if( i > 0 ) 00151 { 00152 polarssl_snprintf( str + idx, maxlen - idx, " %s\n", txt ); 00153 ssl->f_dbg( ssl->p_dbg, level, str ); 00154 00155 idx = 0; 00156 memset( txt, 0, sizeof( txt ) ); 00157 } 00158 00159 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00160 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00161 00162 idx += polarssl_snprintf( str + idx, maxlen - idx, "%04x: ", 00163 (unsigned int) i ); 00164 00165 } 00166 00167 idx += polarssl_snprintf( str + idx, maxlen - idx, " %02x", 00168 (unsigned int) buf[i] ); 00169 txt[i % 16] = ( buf[i] > 31 && buf[i] < 127 ) ? buf[i] : '.' ; 00170 } 00171 00172 if( len > 0 ) 00173 { 00174 for( /* i = i */; i % 16 != 0; i++ ) 00175 idx += polarssl_snprintf( str + idx, maxlen - idx, " " ); 00176 00177 polarssl_snprintf( str + idx, maxlen - idx, " %s\n", txt ); 00178 ssl->f_dbg( ssl->p_dbg, level, str ); 00179 } 00180 } 00181 00182 #if defined(POLARSSL_ECP_C) 00183 void debug_print_ecp( const ssl_context *ssl, int level, 00184 const char *file, int line, 00185 const char *text, const ecp_point *X ) 00186 { 00187 char str[512]; 00188 int maxlen = sizeof( str ) - 1; 00189 00190 if( ssl->f_dbg == NULL || level > debug_threshold ) 00191 return; 00192 00193 polarssl_snprintf( str, maxlen, "%s(X)", text ); 00194 str[maxlen] = '\0'; 00195 debug_print_mpi( ssl, level, file, line, str, &X->X ); 00196 00197 polarssl_snprintf( str, maxlen, "%s(Y)", text ); 00198 str[maxlen] = '\0'; 00199 debug_print_mpi( ssl, level, file, line, str, &X->Y ); 00200 } 00201 #endif /* POLARSSL_ECP_C */ 00202 00203 #if defined(POLARSSL_BIGNUM_C) 00204 void debug_print_mpi( const ssl_context *ssl, int level, 00205 const char *file, int line, 00206 const char *text, const mpi *X ) 00207 { 00208 char str[512]; 00209 int j, k, maxlen = sizeof( str ) - 1, zeros = 1; 00210 size_t i, n, idx = 0; 00211 00212 if( ssl->f_dbg == NULL || X == NULL || level > debug_threshold ) 00213 return; 00214 00215 for( n = X->n - 1; n > 0; n-- ) 00216 if( X->p [n] != 0 ) 00217 break; 00218 00219 for( j = ( sizeof(t_uint) << 3 ) - 1; j >= 0; j-- ) 00220 if( ( ( X->p [n] >> j ) & 1 ) != 0 ) 00221 break; 00222 00223 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00224 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00225 00226 polarssl_snprintf( str + idx, maxlen - idx, "value of '%s' (%d bits) is:\n", 00227 text, (int) ( ( n * ( sizeof(t_uint) << 3 ) ) + j + 1 ) ); 00228 00229 str[maxlen] = '\0'; 00230 ssl->f_dbg( ssl->p_dbg, level, str ); 00231 00232 idx = 0; 00233 for( i = n + 1, j = 0; i > 0; i-- ) 00234 { 00235 if( zeros && X->p [i - 1] == 0 ) 00236 continue; 00237 00238 for( k = sizeof( t_uint ) - 1; k >= 0; k-- ) 00239 { 00240 if( zeros && ( ( X->p [i - 1] >> ( k << 3 ) ) & 0xFF ) == 0 ) 00241 continue; 00242 else 00243 zeros = 0; 00244 00245 if( j % 16 == 0 ) 00246 { 00247 if( j > 0 ) 00248 { 00249 polarssl_snprintf( str + idx, maxlen - idx, "\n" ); 00250 ssl->f_dbg( ssl->p_dbg, level, str ); 00251 idx = 0; 00252 } 00253 00254 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00255 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00256 } 00257 00258 idx += polarssl_snprintf( str + idx, maxlen - idx, " %02x", (unsigned int) 00259 ( X->p [i - 1] >> ( k << 3 ) ) & 0xFF ); 00260 00261 j++; 00262 } 00263 00264 } 00265 00266 if( zeros == 1 ) 00267 { 00268 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00269 { 00270 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00271 00272 } 00273 idx += polarssl_snprintf( str + idx, maxlen - idx, " 00" ); 00274 } 00275 00276 polarssl_snprintf( str + idx, maxlen - idx, "\n" ); 00277 ssl->f_dbg( ssl->p_dbg, level, str ); 00278 } 00279 #endif /* POLARSSL_BIGNUM_C */ 00280 00281 #if defined(POLARSSL_X509_CRT_PARSE_C) 00282 static void debug_print_pk( const ssl_context *ssl, int level, 00283 const char *file, int line, 00284 const char *text, const pk_context *pk ) 00285 { 00286 size_t i; 00287 pk_debug_item items[POLARSSL_PK_DEBUG_MAX_ITEMS]; 00288 char name[16]; 00289 00290 memset( items, 0, sizeof( items ) ); 00291 00292 if( pk_debug( pk, items ) != 0 ) 00293 { 00294 debug_print_msg( ssl, level, file, line, "invalid PK context" ); 00295 return; 00296 } 00297 00298 for( i = 0; i < POLARSSL_PK_DEBUG_MAX_ITEMS; i++ ) 00299 { 00300 if( items[i].type == POLARSSL_PK_DEBUG_NONE ) 00301 return; 00302 00303 polarssl_snprintf( name, sizeof( name ), "%s%s", text, items[i].name ); 00304 name[sizeof( name ) - 1] = '\0'; 00305 00306 if( items[i].type == POLARSSL_PK_DEBUG_MPI ) 00307 debug_print_mpi( ssl, level, file, line, name, items[i].value ); 00308 else 00309 #if defined(POLARSSL_ECP_C) 00310 if( items[i].type == POLARSSL_PK_DEBUG_ECP ) 00311 debug_print_ecp( ssl, level, file, line, name, items[i].value ); 00312 else 00313 #endif 00314 debug_print_msg( ssl, level, file, line, "should not happen" ); 00315 } 00316 } 00317 00318 void debug_print_crt( const ssl_context *ssl, int level, 00319 const char *file, int line, 00320 const char *text, const x509_crt *crt ) 00321 { 00322 char str[1024], prefix[64]; 00323 int i = 0, maxlen = sizeof( prefix ) - 1, idx = 0; 00324 00325 if( ssl->f_dbg == NULL || crt == NULL || level > debug_threshold ) 00326 return; 00327 00328 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00329 { 00330 polarssl_snprintf( prefix, maxlen, "%s(%04d): ", file, line ); 00331 prefix[maxlen] = '\0'; 00332 } 00333 else 00334 prefix[0] = '\0'; 00335 00336 maxlen = sizeof( str ) - 1; 00337 00338 while( crt != NULL ) 00339 { 00340 char buf[1024]; 00341 x509_crt_info( buf, sizeof( buf ) - 1, prefix, crt ); 00342 00343 if( debug_log_mode == POLARSSL_DEBUG_LOG_FULL ) 00344 idx = polarssl_snprintf( str, maxlen, "%s(%04d): ", file, line ); 00345 00346 polarssl_snprintf( str + idx, maxlen - idx, "%s #%d:\n%s", 00347 text, ++i, buf ); 00348 00349 str[maxlen] = '\0'; 00350 ssl->f_dbg( ssl->p_dbg, level, str ); 00351 00352 debug_print_pk( ssl, level, file, line, "crt->", &crt->pk ); 00353 00354 crt = crt->next; 00355 } 00356 } 00357 #endif /* POLARSSL_X509_CRT_PARSE_C */ 00358 00359 #endif /* POLARSSL_DEBUG_C */ 00360
Generated on Tue Jul 12 2022 13:50:37 by
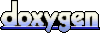