mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
cipher_wrap.c
00001 /** 00002 * \file cipher_wrap.c 00003 * 00004 * \brief Generic cipher wrapper for mbed TLS 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "polarssl/config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #if defined(POLARSSL_CIPHER_C) 00034 00035 #include "polarssl/cipher_wrap.h" 00036 00037 #if defined(POLARSSL_AES_C) 00038 #include "polarssl/aes.h" 00039 #endif 00040 00041 #if defined(POLARSSL_ARC4_C) 00042 #include "polarssl/arc4.h" 00043 #endif 00044 00045 #if defined(POLARSSL_CAMELLIA_C) 00046 #include "polarssl/camellia.h" 00047 #endif 00048 00049 #if defined(POLARSSL_DES_C) 00050 #include "polarssl/des.h" 00051 #endif 00052 00053 #if defined(POLARSSL_BLOWFISH_C) 00054 #include "polarssl/blowfish.h" 00055 #endif 00056 00057 #if defined(POLARSSL_GCM_C) 00058 #include "polarssl/gcm.h" 00059 #endif 00060 00061 #if defined(POLARSSL_CCM_C) 00062 #include "polarssl/ccm.h" 00063 #endif 00064 00065 #if defined(POLARSSL_CIPHER_NULL_CIPHER) 00066 #include <string.h> 00067 #endif 00068 00069 #if defined(POLARSSL_PLATFORM_C) 00070 #include "polarssl/platform.h" 00071 #else 00072 #include <stdlib.h> 00073 #define polarssl_malloc malloc 00074 #define polarssl_free free 00075 #endif 00076 00077 #if defined(POLARSSL_GCM_C) 00078 /* shared by all GCM ciphers */ 00079 static void *gcm_ctx_alloc( void ) 00080 { 00081 return polarssl_malloc( sizeof( gcm_context ) ); 00082 } 00083 00084 static void gcm_ctx_free( void *ctx ) 00085 { 00086 gcm_free( ctx ); 00087 polarssl_free( ctx ); 00088 } 00089 #endif /* POLARSSL_GCM_C */ 00090 00091 #if defined(POLARSSL_CCM_C) 00092 /* shared by all CCM ciphers */ 00093 static void *ccm_ctx_alloc( void ) 00094 { 00095 return polarssl_malloc( sizeof( ccm_context ) ); 00096 } 00097 00098 static void ccm_ctx_free( void *ctx ) 00099 { 00100 ccm_free( ctx ); 00101 polarssl_free( ctx ); 00102 } 00103 #endif /* POLARSSL_CCM_C */ 00104 00105 #if defined(POLARSSL_AES_C) 00106 00107 static int aes_crypt_ecb_wrap( void *ctx, operation_t operation, 00108 const unsigned char *input, unsigned char *output ) 00109 { 00110 return aes_crypt_ecb( (aes_context *) ctx, operation, input, output ); 00111 } 00112 00113 #if defined(POLARSSL_CIPHER_MODE_CBC) 00114 static int aes_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00115 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00116 { 00117 return aes_crypt_cbc( (aes_context *) ctx, operation, length, iv, input, 00118 output ); 00119 } 00120 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00121 00122 #if defined(POLARSSL_CIPHER_MODE_CFB) 00123 static int aes_crypt_cfb128_wrap( void *ctx, operation_t operation, 00124 size_t length, size_t *iv_off, unsigned char *iv, 00125 const unsigned char *input, unsigned char *output ) 00126 { 00127 return aes_crypt_cfb128( (aes_context *) ctx, operation, length, iv_off, iv, 00128 input, output ); 00129 } 00130 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00131 00132 #if defined(POLARSSL_CIPHER_MODE_CTR) 00133 static int aes_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00134 unsigned char *nonce_counter, unsigned char *stream_block, 00135 const unsigned char *input, unsigned char *output ) 00136 { 00137 return aes_crypt_ctr( (aes_context *) ctx, length, nc_off, nonce_counter, 00138 stream_block, input, output ); 00139 } 00140 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00141 00142 static int aes_setkey_dec_wrap( void *ctx, const unsigned char *key, 00143 unsigned int key_length ) 00144 { 00145 return aes_setkey_dec( (aes_context *) ctx, key, key_length ); 00146 } 00147 00148 static int aes_setkey_enc_wrap( void *ctx, const unsigned char *key, 00149 unsigned int key_length ) 00150 { 00151 return aes_setkey_enc( (aes_context *) ctx, key, key_length ); 00152 } 00153 00154 static void * aes_ctx_alloc( void ) 00155 { 00156 aes_context *aes = polarssl_malloc( sizeof( aes_context ) ); 00157 00158 if( aes == NULL ) 00159 return( NULL ); 00160 00161 aes_init( aes ); 00162 00163 return( aes ); 00164 } 00165 00166 static void aes_ctx_free( void *ctx ) 00167 { 00168 aes_free( (aes_context *) ctx ); 00169 polarssl_free( ctx ); 00170 } 00171 00172 static const cipher_base_t aes_info = { 00173 POLARSSL_CIPHER_ID_AES, 00174 aes_crypt_ecb_wrap, 00175 #if defined(POLARSSL_CIPHER_MODE_CBC) 00176 aes_crypt_cbc_wrap, 00177 #endif 00178 #if defined(POLARSSL_CIPHER_MODE_CFB) 00179 aes_crypt_cfb128_wrap, 00180 #endif 00181 #if defined(POLARSSL_CIPHER_MODE_CTR) 00182 aes_crypt_ctr_wrap, 00183 #endif 00184 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00185 NULL, 00186 #endif 00187 aes_setkey_enc_wrap, 00188 aes_setkey_dec_wrap, 00189 aes_ctx_alloc, 00190 aes_ctx_free 00191 }; 00192 00193 static const cipher_info_t aes_128_ecb_info = { 00194 POLARSSL_CIPHER_AES_128_ECB, 00195 POLARSSL_MODE_ECB, 00196 128, 00197 "AES-128-ECB", 00198 16, 00199 0, 00200 16, 00201 &aes_info 00202 }; 00203 00204 static const cipher_info_t aes_192_ecb_info = { 00205 POLARSSL_CIPHER_AES_192_ECB, 00206 POLARSSL_MODE_ECB, 00207 192, 00208 "AES-192-ECB", 00209 16, 00210 0, 00211 16, 00212 &aes_info 00213 }; 00214 00215 static const cipher_info_t aes_256_ecb_info = { 00216 POLARSSL_CIPHER_AES_256_ECB, 00217 POLARSSL_MODE_ECB, 00218 256, 00219 "AES-256-ECB", 00220 16, 00221 0, 00222 16, 00223 &aes_info 00224 }; 00225 00226 #if defined(POLARSSL_CIPHER_MODE_CBC) 00227 static const cipher_info_t aes_128_cbc_info = { 00228 POLARSSL_CIPHER_AES_128_CBC, 00229 POLARSSL_MODE_CBC, 00230 128, 00231 "AES-128-CBC", 00232 16, 00233 0, 00234 16, 00235 &aes_info 00236 }; 00237 00238 static const cipher_info_t aes_192_cbc_info = { 00239 POLARSSL_CIPHER_AES_192_CBC, 00240 POLARSSL_MODE_CBC, 00241 192, 00242 "AES-192-CBC", 00243 16, 00244 0, 00245 16, 00246 &aes_info 00247 }; 00248 00249 static const cipher_info_t aes_256_cbc_info = { 00250 POLARSSL_CIPHER_AES_256_CBC, 00251 POLARSSL_MODE_CBC, 00252 256, 00253 "AES-256-CBC", 00254 16, 00255 0, 00256 16, 00257 &aes_info 00258 }; 00259 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00260 00261 #if defined(POLARSSL_CIPHER_MODE_CFB) 00262 static const cipher_info_t aes_128_cfb128_info = { 00263 POLARSSL_CIPHER_AES_128_CFB128, 00264 POLARSSL_MODE_CFB, 00265 128, 00266 "AES-128-CFB128", 00267 16, 00268 0, 00269 16, 00270 &aes_info 00271 }; 00272 00273 static const cipher_info_t aes_192_cfb128_info = { 00274 POLARSSL_CIPHER_AES_192_CFB128, 00275 POLARSSL_MODE_CFB, 00276 192, 00277 "AES-192-CFB128", 00278 16, 00279 0, 00280 16, 00281 &aes_info 00282 }; 00283 00284 static const cipher_info_t aes_256_cfb128_info = { 00285 POLARSSL_CIPHER_AES_256_CFB128, 00286 POLARSSL_MODE_CFB, 00287 256, 00288 "AES-256-CFB128", 00289 16, 00290 0, 00291 16, 00292 &aes_info 00293 }; 00294 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00295 00296 #if defined(POLARSSL_CIPHER_MODE_CTR) 00297 static const cipher_info_t aes_128_ctr_info = { 00298 POLARSSL_CIPHER_AES_128_CTR, 00299 POLARSSL_MODE_CTR, 00300 128, 00301 "AES-128-CTR", 00302 16, 00303 0, 00304 16, 00305 &aes_info 00306 }; 00307 00308 static const cipher_info_t aes_192_ctr_info = { 00309 POLARSSL_CIPHER_AES_192_CTR, 00310 POLARSSL_MODE_CTR, 00311 192, 00312 "AES-192-CTR", 00313 16, 00314 0, 00315 16, 00316 &aes_info 00317 }; 00318 00319 static const cipher_info_t aes_256_ctr_info = { 00320 POLARSSL_CIPHER_AES_256_CTR, 00321 POLARSSL_MODE_CTR, 00322 256, 00323 "AES-256-CTR", 00324 16, 00325 0, 00326 16, 00327 &aes_info 00328 }; 00329 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00330 00331 #if defined(POLARSSL_GCM_C) 00332 static int gcm_aes_setkey_wrap( void *ctx, const unsigned char *key, 00333 unsigned int key_length ) 00334 { 00335 return gcm_init( (gcm_context *) ctx, POLARSSL_CIPHER_ID_AES, 00336 key, key_length ); 00337 } 00338 00339 static const cipher_base_t gcm_aes_info = { 00340 POLARSSL_CIPHER_ID_AES, 00341 NULL, 00342 #if defined(POLARSSL_CIPHER_MODE_CBC) 00343 NULL, 00344 #endif 00345 #if defined(POLARSSL_CIPHER_MODE_CFB) 00346 NULL, 00347 #endif 00348 #if defined(POLARSSL_CIPHER_MODE_CTR) 00349 NULL, 00350 #endif 00351 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00352 NULL, 00353 #endif 00354 gcm_aes_setkey_wrap, 00355 gcm_aes_setkey_wrap, 00356 gcm_ctx_alloc, 00357 gcm_ctx_free, 00358 }; 00359 00360 static const cipher_info_t aes_128_gcm_info = { 00361 POLARSSL_CIPHER_AES_128_GCM, 00362 POLARSSL_MODE_GCM, 00363 128, 00364 "AES-128-GCM", 00365 12, 00366 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00367 16, 00368 &gcm_aes_info 00369 }; 00370 00371 static const cipher_info_t aes_192_gcm_info = { 00372 POLARSSL_CIPHER_AES_192_GCM, 00373 POLARSSL_MODE_GCM, 00374 192, 00375 "AES-192-GCM", 00376 12, 00377 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00378 16, 00379 &gcm_aes_info 00380 }; 00381 00382 static const cipher_info_t aes_256_gcm_info = { 00383 POLARSSL_CIPHER_AES_256_GCM, 00384 POLARSSL_MODE_GCM, 00385 256, 00386 "AES-256-GCM", 00387 12, 00388 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00389 16, 00390 &gcm_aes_info 00391 }; 00392 #endif /* POLARSSL_GCM_C */ 00393 00394 #if defined(POLARSSL_CCM_C) 00395 static int ccm_aes_setkey_wrap( void *ctx, const unsigned char *key, 00396 unsigned int key_length ) 00397 { 00398 return ccm_init( (ccm_context *) ctx, POLARSSL_CIPHER_ID_AES, 00399 key, key_length ); 00400 } 00401 00402 static const cipher_base_t ccm_aes_info = { 00403 POLARSSL_CIPHER_ID_AES, 00404 NULL, 00405 #if defined(POLARSSL_CIPHER_MODE_CBC) 00406 NULL, 00407 #endif 00408 #if defined(POLARSSL_CIPHER_MODE_CFB) 00409 NULL, 00410 #endif 00411 #if defined(POLARSSL_CIPHER_MODE_CTR) 00412 NULL, 00413 #endif 00414 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00415 NULL, 00416 #endif 00417 ccm_aes_setkey_wrap, 00418 ccm_aes_setkey_wrap, 00419 ccm_ctx_alloc, 00420 ccm_ctx_free, 00421 }; 00422 00423 static const cipher_info_t aes_128_ccm_info = { 00424 POLARSSL_CIPHER_AES_128_CCM, 00425 POLARSSL_MODE_CCM, 00426 128, 00427 "AES-128-CCM", 00428 12, 00429 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00430 16, 00431 &ccm_aes_info 00432 }; 00433 00434 static const cipher_info_t aes_192_ccm_info = { 00435 POLARSSL_CIPHER_AES_192_CCM, 00436 POLARSSL_MODE_CCM, 00437 192, 00438 "AES-192-CCM", 00439 12, 00440 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00441 16, 00442 &ccm_aes_info 00443 }; 00444 00445 static const cipher_info_t aes_256_ccm_info = { 00446 POLARSSL_CIPHER_AES_256_CCM, 00447 POLARSSL_MODE_CCM, 00448 256, 00449 "AES-256-CCM", 00450 12, 00451 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00452 16, 00453 &ccm_aes_info 00454 }; 00455 #endif /* POLARSSL_CCM_C */ 00456 00457 #endif /* POLARSSL_AES_C */ 00458 00459 #if defined(POLARSSL_CAMELLIA_C) 00460 00461 static int camellia_crypt_ecb_wrap( void *ctx, operation_t operation, 00462 const unsigned char *input, unsigned char *output ) 00463 { 00464 return camellia_crypt_ecb( (camellia_context *) ctx, operation, input, 00465 output ); 00466 } 00467 00468 #if defined(POLARSSL_CIPHER_MODE_CBC) 00469 static int camellia_crypt_cbc_wrap( void *ctx, operation_t operation, 00470 size_t length, unsigned char *iv, 00471 const unsigned char *input, unsigned char *output ) 00472 { 00473 return camellia_crypt_cbc( (camellia_context *) ctx, operation, length, iv, 00474 input, output ); 00475 } 00476 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00477 00478 #if defined(POLARSSL_CIPHER_MODE_CFB) 00479 static int camellia_crypt_cfb128_wrap( void *ctx, operation_t operation, 00480 size_t length, size_t *iv_off, unsigned char *iv, 00481 const unsigned char *input, unsigned char *output ) 00482 { 00483 return camellia_crypt_cfb128( (camellia_context *) ctx, operation, length, 00484 iv_off, iv, input, output ); 00485 } 00486 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00487 00488 #if defined(POLARSSL_CIPHER_MODE_CTR) 00489 static int camellia_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00490 unsigned char *nonce_counter, unsigned char *stream_block, 00491 const unsigned char *input, unsigned char *output ) 00492 { 00493 return camellia_crypt_ctr( (camellia_context *) ctx, length, nc_off, 00494 nonce_counter, stream_block, input, output ); 00495 } 00496 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00497 00498 static int camellia_setkey_dec_wrap( void *ctx, const unsigned char *key, 00499 unsigned int key_length ) 00500 { 00501 return camellia_setkey_dec( (camellia_context *) ctx, key, key_length ); 00502 } 00503 00504 static int camellia_setkey_enc_wrap( void *ctx, const unsigned char *key, 00505 unsigned int key_length ) 00506 { 00507 return camellia_setkey_enc( (camellia_context *) ctx, key, key_length ); 00508 } 00509 00510 static void * camellia_ctx_alloc( void ) 00511 { 00512 camellia_context *ctx; 00513 ctx = polarssl_malloc( sizeof( camellia_context ) ); 00514 00515 if( ctx == NULL ) 00516 return( NULL ); 00517 00518 camellia_init( ctx ); 00519 00520 return( ctx ); 00521 } 00522 00523 static void camellia_ctx_free( void *ctx ) 00524 { 00525 camellia_free( (camellia_context *) ctx ); 00526 polarssl_free( ctx ); 00527 } 00528 00529 static const cipher_base_t camellia_info = { 00530 POLARSSL_CIPHER_ID_CAMELLIA, 00531 camellia_crypt_ecb_wrap, 00532 #if defined(POLARSSL_CIPHER_MODE_CBC) 00533 camellia_crypt_cbc_wrap, 00534 #endif 00535 #if defined(POLARSSL_CIPHER_MODE_CFB) 00536 camellia_crypt_cfb128_wrap, 00537 #endif 00538 #if defined(POLARSSL_CIPHER_MODE_CTR) 00539 camellia_crypt_ctr_wrap, 00540 #endif 00541 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00542 NULL, 00543 #endif 00544 camellia_setkey_enc_wrap, 00545 camellia_setkey_dec_wrap, 00546 camellia_ctx_alloc, 00547 camellia_ctx_free 00548 }; 00549 00550 static const cipher_info_t camellia_128_ecb_info = { 00551 POLARSSL_CIPHER_CAMELLIA_128_ECB, 00552 POLARSSL_MODE_ECB, 00553 128, 00554 "CAMELLIA-128-ECB", 00555 16, 00556 0, 00557 16, 00558 &camellia_info 00559 }; 00560 00561 static const cipher_info_t camellia_192_ecb_info = { 00562 POLARSSL_CIPHER_CAMELLIA_192_ECB, 00563 POLARSSL_MODE_ECB, 00564 192, 00565 "CAMELLIA-192-ECB", 00566 16, 00567 0, 00568 16, 00569 &camellia_info 00570 }; 00571 00572 static const cipher_info_t camellia_256_ecb_info = { 00573 POLARSSL_CIPHER_CAMELLIA_256_ECB, 00574 POLARSSL_MODE_ECB, 00575 256, 00576 "CAMELLIA-256-ECB", 00577 16, 00578 0, 00579 16, 00580 &camellia_info 00581 }; 00582 00583 #if defined(POLARSSL_CIPHER_MODE_CBC) 00584 static const cipher_info_t camellia_128_cbc_info = { 00585 POLARSSL_CIPHER_CAMELLIA_128_CBC, 00586 POLARSSL_MODE_CBC, 00587 128, 00588 "CAMELLIA-128-CBC", 00589 16, 00590 0, 00591 16, 00592 &camellia_info 00593 }; 00594 00595 static const cipher_info_t camellia_192_cbc_info = { 00596 POLARSSL_CIPHER_CAMELLIA_192_CBC, 00597 POLARSSL_MODE_CBC, 00598 192, 00599 "CAMELLIA-192-CBC", 00600 16, 00601 0, 00602 16, 00603 &camellia_info 00604 }; 00605 00606 static const cipher_info_t camellia_256_cbc_info = { 00607 POLARSSL_CIPHER_CAMELLIA_256_CBC, 00608 POLARSSL_MODE_CBC, 00609 256, 00610 "CAMELLIA-256-CBC", 00611 16, 00612 0, 00613 16, 00614 &camellia_info 00615 }; 00616 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00617 00618 #if defined(POLARSSL_CIPHER_MODE_CFB) 00619 static const cipher_info_t camellia_128_cfb128_info = { 00620 POLARSSL_CIPHER_CAMELLIA_128_CFB128, 00621 POLARSSL_MODE_CFB, 00622 128, 00623 "CAMELLIA-128-CFB128", 00624 16, 00625 0, 00626 16, 00627 &camellia_info 00628 }; 00629 00630 static const cipher_info_t camellia_192_cfb128_info = { 00631 POLARSSL_CIPHER_CAMELLIA_192_CFB128, 00632 POLARSSL_MODE_CFB, 00633 192, 00634 "CAMELLIA-192-CFB128", 00635 16, 00636 0, 00637 16, 00638 &camellia_info 00639 }; 00640 00641 static const cipher_info_t camellia_256_cfb128_info = { 00642 POLARSSL_CIPHER_CAMELLIA_256_CFB128, 00643 POLARSSL_MODE_CFB, 00644 256, 00645 "CAMELLIA-256-CFB128", 00646 16, 00647 0, 00648 16, 00649 &camellia_info 00650 }; 00651 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00652 00653 #if defined(POLARSSL_CIPHER_MODE_CTR) 00654 static const cipher_info_t camellia_128_ctr_info = { 00655 POLARSSL_CIPHER_CAMELLIA_128_CTR, 00656 POLARSSL_MODE_CTR, 00657 128, 00658 "CAMELLIA-128-CTR", 00659 16, 00660 0, 00661 16, 00662 &camellia_info 00663 }; 00664 00665 static const cipher_info_t camellia_192_ctr_info = { 00666 POLARSSL_CIPHER_CAMELLIA_192_CTR, 00667 POLARSSL_MODE_CTR, 00668 192, 00669 "CAMELLIA-192-CTR", 00670 16, 00671 0, 00672 16, 00673 &camellia_info 00674 }; 00675 00676 static const cipher_info_t camellia_256_ctr_info = { 00677 POLARSSL_CIPHER_CAMELLIA_256_CTR, 00678 POLARSSL_MODE_CTR, 00679 256, 00680 "CAMELLIA-256-CTR", 00681 16, 00682 0, 00683 16, 00684 &camellia_info 00685 }; 00686 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00687 00688 #if defined(POLARSSL_GCM_C) 00689 static int gcm_camellia_setkey_wrap( void *ctx, const unsigned char *key, 00690 unsigned int key_length ) 00691 { 00692 return gcm_init( (gcm_context *) ctx, POLARSSL_CIPHER_ID_CAMELLIA, 00693 key, key_length ); 00694 } 00695 00696 static const cipher_base_t gcm_camellia_info = { 00697 POLARSSL_CIPHER_ID_CAMELLIA, 00698 NULL, 00699 #if defined(POLARSSL_CIPHER_MODE_CBC) 00700 NULL, 00701 #endif 00702 #if defined(POLARSSL_CIPHER_MODE_CFB) 00703 NULL, 00704 #endif 00705 #if defined(POLARSSL_CIPHER_MODE_CTR) 00706 NULL, 00707 #endif 00708 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00709 NULL, 00710 #endif 00711 gcm_camellia_setkey_wrap, 00712 gcm_camellia_setkey_wrap, 00713 gcm_ctx_alloc, 00714 gcm_ctx_free, 00715 }; 00716 00717 static const cipher_info_t camellia_128_gcm_info = { 00718 POLARSSL_CIPHER_CAMELLIA_128_GCM, 00719 POLARSSL_MODE_GCM, 00720 128, 00721 "CAMELLIA-128-GCM", 00722 12, 00723 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00724 16, 00725 &gcm_camellia_info 00726 }; 00727 00728 static const cipher_info_t camellia_192_gcm_info = { 00729 POLARSSL_CIPHER_CAMELLIA_192_GCM, 00730 POLARSSL_MODE_GCM, 00731 192, 00732 "CAMELLIA-192-GCM", 00733 12, 00734 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00735 16, 00736 &gcm_camellia_info 00737 }; 00738 00739 static const cipher_info_t camellia_256_gcm_info = { 00740 POLARSSL_CIPHER_CAMELLIA_256_GCM, 00741 POLARSSL_MODE_GCM, 00742 256, 00743 "CAMELLIA-256-GCM", 00744 12, 00745 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00746 16, 00747 &gcm_camellia_info 00748 }; 00749 #endif /* POLARSSL_GCM_C */ 00750 00751 #if defined(POLARSSL_CCM_C) 00752 static int ccm_camellia_setkey_wrap( void *ctx, const unsigned char *key, 00753 unsigned int key_length ) 00754 { 00755 return ccm_init( (ccm_context *) ctx, POLARSSL_CIPHER_ID_CAMELLIA, 00756 key, key_length ); 00757 } 00758 00759 static const cipher_base_t ccm_camellia_info = { 00760 POLARSSL_CIPHER_ID_CAMELLIA, 00761 NULL, 00762 #if defined(POLARSSL_CIPHER_MODE_CBC) 00763 NULL, 00764 #endif 00765 #if defined(POLARSSL_CIPHER_MODE_CFB) 00766 NULL, 00767 #endif 00768 #if defined(POLARSSL_CIPHER_MODE_CTR) 00769 NULL, 00770 #endif 00771 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00772 NULL, 00773 #endif 00774 ccm_camellia_setkey_wrap, 00775 ccm_camellia_setkey_wrap, 00776 ccm_ctx_alloc, 00777 ccm_ctx_free, 00778 }; 00779 00780 static const cipher_info_t camellia_128_ccm_info = { 00781 POLARSSL_CIPHER_CAMELLIA_128_CCM, 00782 POLARSSL_MODE_CCM, 00783 128, 00784 "CAMELLIA-128-CCM", 00785 12, 00786 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00787 16, 00788 &ccm_camellia_info 00789 }; 00790 00791 static const cipher_info_t camellia_192_ccm_info = { 00792 POLARSSL_CIPHER_CAMELLIA_192_CCM, 00793 POLARSSL_MODE_CCM, 00794 192, 00795 "CAMELLIA-192-CCM", 00796 12, 00797 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00798 16, 00799 &ccm_camellia_info 00800 }; 00801 00802 static const cipher_info_t camellia_256_ccm_info = { 00803 POLARSSL_CIPHER_CAMELLIA_256_CCM, 00804 POLARSSL_MODE_CCM, 00805 256, 00806 "CAMELLIA-256-CCM", 00807 12, 00808 POLARSSL_CIPHER_VARIABLE_IV_LEN, 00809 16, 00810 &ccm_camellia_info 00811 }; 00812 #endif /* POLARSSL_CCM_C */ 00813 00814 #endif /* POLARSSL_CAMELLIA_C */ 00815 00816 #if defined(POLARSSL_DES_C) 00817 00818 static int des_crypt_ecb_wrap( void *ctx, operation_t operation, 00819 const unsigned char *input, unsigned char *output ) 00820 { 00821 ((void) operation); 00822 return des_crypt_ecb( (des_context *) ctx, input, output ); 00823 } 00824 00825 static int des3_crypt_ecb_wrap( void *ctx, operation_t operation, 00826 const unsigned char *input, unsigned char *output ) 00827 { 00828 ((void) operation); 00829 return des3_crypt_ecb( (des3_context *) ctx, input, output ); 00830 } 00831 00832 #if defined(POLARSSL_CIPHER_MODE_CBC) 00833 static int des_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00834 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00835 { 00836 return des_crypt_cbc( (des_context *) ctx, operation, length, iv, input, 00837 output ); 00838 } 00839 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00840 00841 #if defined(POLARSSL_CIPHER_MODE_CBC) 00842 static int des3_crypt_cbc_wrap( void *ctx, operation_t operation, size_t length, 00843 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00844 { 00845 return des3_crypt_cbc( (des3_context *) ctx, operation, length, iv, input, 00846 output ); 00847 } 00848 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00849 00850 static int des_setkey_dec_wrap( void *ctx, const unsigned char *key, 00851 unsigned int key_length ) 00852 { 00853 ((void) key_length); 00854 00855 return des_setkey_dec( (des_context *) ctx, key ); 00856 } 00857 00858 static int des_setkey_enc_wrap( void *ctx, const unsigned char *key, 00859 unsigned int key_length ) 00860 { 00861 ((void) key_length); 00862 00863 return des_setkey_enc( (des_context *) ctx, key ); 00864 } 00865 00866 static int des3_set2key_dec_wrap( void *ctx, const unsigned char *key, 00867 unsigned int key_length ) 00868 { 00869 ((void) key_length); 00870 00871 return des3_set2key_dec( (des3_context *) ctx, key ); 00872 } 00873 00874 static int des3_set2key_enc_wrap( void *ctx, const unsigned char *key, 00875 unsigned int key_length ) 00876 { 00877 ((void) key_length); 00878 00879 return des3_set2key_enc( (des3_context *) ctx, key ); 00880 } 00881 00882 static int des3_set3key_dec_wrap( void *ctx, const unsigned char *key, 00883 unsigned int key_length ) 00884 { 00885 ((void) key_length); 00886 00887 return des3_set3key_dec( (des3_context *) ctx, key ); 00888 } 00889 00890 static int des3_set3key_enc_wrap( void *ctx, const unsigned char *key, 00891 unsigned int key_length ) 00892 { 00893 ((void) key_length); 00894 00895 return des3_set3key_enc( (des3_context *) ctx, key ); 00896 } 00897 00898 static void * des_ctx_alloc( void ) 00899 { 00900 des_context *des = polarssl_malloc( sizeof( des_context ) ); 00901 00902 if( des == NULL ) 00903 return( NULL ); 00904 00905 des_init( des ); 00906 00907 return( des ); 00908 } 00909 00910 static void des_ctx_free( void *ctx ) 00911 { 00912 des_free( (des_context *) ctx ); 00913 polarssl_free( ctx ); 00914 } 00915 00916 static void * des3_ctx_alloc( void ) 00917 { 00918 des3_context *des3; 00919 des3 = polarssl_malloc( sizeof( des3_context ) ); 00920 00921 if( des3 == NULL ) 00922 return( NULL ); 00923 00924 des3_init( des3 ); 00925 00926 return( des3 ); 00927 } 00928 00929 static void des3_ctx_free( void *ctx ) 00930 { 00931 des3_free( (des3_context *) ctx ); 00932 polarssl_free( ctx ); 00933 } 00934 00935 static const cipher_base_t des_info = { 00936 POLARSSL_CIPHER_ID_DES, 00937 des_crypt_ecb_wrap, 00938 #if defined(POLARSSL_CIPHER_MODE_CBC) 00939 des_crypt_cbc_wrap, 00940 #endif 00941 #if defined(POLARSSL_CIPHER_MODE_CFB) 00942 NULL, 00943 #endif 00944 #if defined(POLARSSL_CIPHER_MODE_CTR) 00945 NULL, 00946 #endif 00947 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00948 NULL, 00949 #endif 00950 des_setkey_enc_wrap, 00951 des_setkey_dec_wrap, 00952 des_ctx_alloc, 00953 des_ctx_free 00954 }; 00955 00956 static const cipher_info_t des_ecb_info = { 00957 POLARSSL_CIPHER_DES_ECB, 00958 POLARSSL_MODE_ECB, 00959 POLARSSL_KEY_LENGTH_DES, 00960 "DES-ECB", 00961 8, 00962 0, 00963 8, 00964 &des_info 00965 }; 00966 00967 #if defined(POLARSSL_CIPHER_MODE_CBC) 00968 static const cipher_info_t des_cbc_info = { 00969 POLARSSL_CIPHER_DES_CBC, 00970 POLARSSL_MODE_CBC, 00971 POLARSSL_KEY_LENGTH_DES, 00972 "DES-CBC", 00973 8, 00974 0, 00975 8, 00976 &des_info 00977 }; 00978 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00979 00980 static const cipher_base_t des_ede_info = { 00981 POLARSSL_CIPHER_ID_DES, 00982 des3_crypt_ecb_wrap, 00983 #if defined(POLARSSL_CIPHER_MODE_CBC) 00984 des3_crypt_cbc_wrap, 00985 #endif 00986 #if defined(POLARSSL_CIPHER_MODE_CFB) 00987 NULL, 00988 #endif 00989 #if defined(POLARSSL_CIPHER_MODE_CTR) 00990 NULL, 00991 #endif 00992 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00993 NULL, 00994 #endif 00995 des3_set2key_enc_wrap, 00996 des3_set2key_dec_wrap, 00997 des3_ctx_alloc, 00998 des3_ctx_free 00999 }; 01000 01001 static const cipher_info_t des_ede_ecb_info = { 01002 POLARSSL_CIPHER_DES_EDE_ECB, 01003 POLARSSL_MODE_ECB, 01004 POLARSSL_KEY_LENGTH_DES_EDE, 01005 "DES-EDE-ECB", 01006 8, 01007 0, 01008 8, 01009 &des_ede_info 01010 }; 01011 01012 #if defined(POLARSSL_CIPHER_MODE_CBC) 01013 static const cipher_info_t des_ede_cbc_info = { 01014 POLARSSL_CIPHER_DES_EDE_CBC, 01015 POLARSSL_MODE_CBC, 01016 POLARSSL_KEY_LENGTH_DES_EDE, 01017 "DES-EDE-CBC", 01018 8, 01019 0, 01020 8, 01021 &des_ede_info 01022 }; 01023 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01024 01025 static const cipher_base_t des_ede3_info = { 01026 POLARSSL_CIPHER_ID_DES, 01027 des3_crypt_ecb_wrap, 01028 #if defined(POLARSSL_CIPHER_MODE_CBC) 01029 des3_crypt_cbc_wrap, 01030 #endif 01031 #if defined(POLARSSL_CIPHER_MODE_CFB) 01032 NULL, 01033 #endif 01034 #if defined(POLARSSL_CIPHER_MODE_CTR) 01035 NULL, 01036 #endif 01037 #if defined(POLARSSL_CIPHER_MODE_STREAM) 01038 NULL, 01039 #endif 01040 des3_set3key_enc_wrap, 01041 des3_set3key_dec_wrap, 01042 des3_ctx_alloc, 01043 des3_ctx_free 01044 }; 01045 01046 static const cipher_info_t des_ede3_ecb_info = { 01047 POLARSSL_CIPHER_DES_EDE3_ECB, 01048 POLARSSL_MODE_ECB, 01049 POLARSSL_KEY_LENGTH_DES_EDE3, 01050 "DES-EDE3-ECB", 01051 8, 01052 0, 01053 8, 01054 &des_ede3_info 01055 }; 01056 #if defined(POLARSSL_CIPHER_MODE_CBC) 01057 static const cipher_info_t des_ede3_cbc_info = { 01058 POLARSSL_CIPHER_DES_EDE3_CBC, 01059 POLARSSL_MODE_CBC, 01060 POLARSSL_KEY_LENGTH_DES_EDE3, 01061 "DES-EDE3-CBC", 01062 8, 01063 0, 01064 8, 01065 &des_ede3_info 01066 }; 01067 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01068 #endif /* POLARSSL_DES_C */ 01069 01070 #if defined(POLARSSL_BLOWFISH_C) 01071 01072 static int blowfish_crypt_ecb_wrap( void *ctx, operation_t operation, 01073 const unsigned char *input, unsigned char *output ) 01074 { 01075 return blowfish_crypt_ecb( (blowfish_context *) ctx, operation, input, 01076 output ); 01077 } 01078 01079 #if defined(POLARSSL_CIPHER_MODE_CBC) 01080 static int blowfish_crypt_cbc_wrap( void *ctx, operation_t operation, 01081 size_t length, unsigned char *iv, const unsigned char *input, 01082 unsigned char *output ) 01083 { 01084 return blowfish_crypt_cbc( (blowfish_context *) ctx, operation, length, iv, 01085 input, output ); 01086 } 01087 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01088 01089 #if defined(POLARSSL_CIPHER_MODE_CFB) 01090 static int blowfish_crypt_cfb64_wrap( void *ctx, operation_t operation, 01091 size_t length, size_t *iv_off, unsigned char *iv, 01092 const unsigned char *input, unsigned char *output ) 01093 { 01094 return blowfish_crypt_cfb64( (blowfish_context *) ctx, operation, length, 01095 iv_off, iv, input, output ); 01096 } 01097 #endif /* POLARSSL_CIPHER_MODE_CFB */ 01098 01099 #if defined(POLARSSL_CIPHER_MODE_CTR) 01100 static int blowfish_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 01101 unsigned char *nonce_counter, unsigned char *stream_block, 01102 const unsigned char *input, unsigned char *output ) 01103 { 01104 return blowfish_crypt_ctr( (blowfish_context *) ctx, length, nc_off, 01105 nonce_counter, stream_block, input, output ); 01106 } 01107 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01108 01109 static int blowfish_setkey_wrap( void *ctx, const unsigned char *key, 01110 unsigned int key_length ) 01111 { 01112 return blowfish_setkey( (blowfish_context *) ctx, key, key_length ); 01113 } 01114 01115 static void * blowfish_ctx_alloc( void ) 01116 { 01117 blowfish_context *ctx; 01118 ctx = polarssl_malloc( sizeof( blowfish_context ) ); 01119 01120 if( ctx == NULL ) 01121 return( NULL ); 01122 01123 blowfish_init( ctx ); 01124 01125 return( ctx ); 01126 } 01127 01128 static void blowfish_ctx_free( void *ctx ) 01129 { 01130 blowfish_free( (blowfish_context *) ctx ); 01131 polarssl_free( ctx ); 01132 } 01133 01134 static const cipher_base_t blowfish_info = { 01135 POLARSSL_CIPHER_ID_BLOWFISH, 01136 blowfish_crypt_ecb_wrap, 01137 #if defined(POLARSSL_CIPHER_MODE_CBC) 01138 blowfish_crypt_cbc_wrap, 01139 #endif 01140 #if defined(POLARSSL_CIPHER_MODE_CFB) 01141 blowfish_crypt_cfb64_wrap, 01142 #endif 01143 #if defined(POLARSSL_CIPHER_MODE_CTR) 01144 blowfish_crypt_ctr_wrap, 01145 #endif 01146 #if defined(POLARSSL_CIPHER_MODE_STREAM) 01147 NULL, 01148 #endif 01149 blowfish_setkey_wrap, 01150 blowfish_setkey_wrap, 01151 blowfish_ctx_alloc, 01152 blowfish_ctx_free 01153 }; 01154 01155 static const cipher_info_t blowfish_ecb_info = { 01156 POLARSSL_CIPHER_BLOWFISH_ECB, 01157 POLARSSL_MODE_ECB, 01158 128, 01159 "BLOWFISH-ECB", 01160 8, 01161 POLARSSL_CIPHER_VARIABLE_KEY_LEN, 01162 8, 01163 &blowfish_info 01164 }; 01165 01166 #if defined(POLARSSL_CIPHER_MODE_CBC) 01167 static const cipher_info_t blowfish_cbc_info = { 01168 POLARSSL_CIPHER_BLOWFISH_CBC, 01169 POLARSSL_MODE_CBC, 01170 128, 01171 "BLOWFISH-CBC", 01172 8, 01173 POLARSSL_CIPHER_VARIABLE_KEY_LEN, 01174 8, 01175 &blowfish_info 01176 }; 01177 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01178 01179 #if defined(POLARSSL_CIPHER_MODE_CFB) 01180 static const cipher_info_t blowfish_cfb64_info = { 01181 POLARSSL_CIPHER_BLOWFISH_CFB64, 01182 POLARSSL_MODE_CFB, 01183 128, 01184 "BLOWFISH-CFB64", 01185 8, 01186 POLARSSL_CIPHER_VARIABLE_KEY_LEN, 01187 8, 01188 &blowfish_info 01189 }; 01190 #endif /* POLARSSL_CIPHER_MODE_CFB */ 01191 01192 #if defined(POLARSSL_CIPHER_MODE_CTR) 01193 static const cipher_info_t blowfish_ctr_info = { 01194 POLARSSL_CIPHER_BLOWFISH_CTR, 01195 POLARSSL_MODE_CTR, 01196 128, 01197 "BLOWFISH-CTR", 01198 8, 01199 POLARSSL_CIPHER_VARIABLE_KEY_LEN, 01200 8, 01201 &blowfish_info 01202 }; 01203 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01204 #endif /* POLARSSL_BLOWFISH_C */ 01205 01206 #if defined(POLARSSL_ARC4_C) 01207 static int arc4_crypt_stream_wrap( void *ctx, size_t length, 01208 const unsigned char *input, 01209 unsigned char *output ) 01210 { 01211 return( arc4_crypt( (arc4_context *) ctx, length, input, output ) ); 01212 } 01213 01214 static int arc4_setkey_wrap( void *ctx, const unsigned char *key, 01215 unsigned int key_length ) 01216 { 01217 /* we get key_length in bits, arc4 expects it in bytes */ 01218 if( key_length % 8 != 0 ) 01219 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 01220 01221 arc4_setup( (arc4_context *) ctx, key, key_length / 8 ); 01222 return( 0 ); 01223 } 01224 01225 static void * arc4_ctx_alloc( void ) 01226 { 01227 arc4_context *ctx; 01228 ctx = polarssl_malloc( sizeof( arc4_context ) ); 01229 01230 if( ctx == NULL ) 01231 return( NULL ); 01232 01233 arc4_init( ctx ); 01234 01235 return( ctx ); 01236 } 01237 01238 static void arc4_ctx_free( void *ctx ) 01239 { 01240 arc4_free( (arc4_context *) ctx ); 01241 polarssl_free( ctx ); 01242 } 01243 01244 static const cipher_base_t arc4_base_info = { 01245 POLARSSL_CIPHER_ID_ARC4, 01246 NULL, 01247 #if defined(POLARSSL_CIPHER_MODE_CBC) 01248 NULL, 01249 #endif 01250 #if defined(POLARSSL_CIPHER_MODE_CFB) 01251 NULL, 01252 #endif 01253 #if defined(POLARSSL_CIPHER_MODE_CTR) 01254 NULL, 01255 #endif 01256 #if defined(POLARSSL_CIPHER_MODE_STREAM) 01257 arc4_crypt_stream_wrap, 01258 #endif 01259 arc4_setkey_wrap, 01260 arc4_setkey_wrap, 01261 arc4_ctx_alloc, 01262 arc4_ctx_free 01263 }; 01264 01265 static const cipher_info_t arc4_128_info = { 01266 POLARSSL_CIPHER_ARC4_128, 01267 POLARSSL_MODE_STREAM, 01268 128, 01269 "ARC4-128", 01270 0, 01271 0, 01272 1, 01273 &arc4_base_info 01274 }; 01275 #endif /* POLARSSL_ARC4_C */ 01276 01277 #if defined(POLARSSL_CIPHER_NULL_CIPHER) 01278 static int null_crypt_stream( void *ctx, size_t length, 01279 const unsigned char *input, 01280 unsigned char *output ) 01281 { 01282 ((void) ctx); 01283 memmove( output, input, length ); 01284 return( 0 ); 01285 } 01286 01287 static int null_setkey( void *ctx, const unsigned char *key, 01288 unsigned int key_length ) 01289 { 01290 ((void) ctx); 01291 ((void) key); 01292 ((void) key_length); 01293 01294 return( 0 ); 01295 } 01296 01297 static void * null_ctx_alloc( void ) 01298 { 01299 return( (void *) 1 ); 01300 } 01301 01302 static void null_ctx_free( void *ctx ) 01303 { 01304 ((void) ctx); 01305 } 01306 01307 static const cipher_base_t null_base_info = { 01308 POLARSSL_CIPHER_ID_NULL, 01309 NULL, 01310 #if defined(POLARSSL_CIPHER_MODE_CBC) 01311 NULL, 01312 #endif 01313 #if defined(POLARSSL_CIPHER_MODE_CFB) 01314 NULL, 01315 #endif 01316 #if defined(POLARSSL_CIPHER_MODE_CTR) 01317 NULL, 01318 #endif 01319 #if defined(POLARSSL_CIPHER_MODE_STREAM) 01320 null_crypt_stream, 01321 #endif 01322 null_setkey, 01323 null_setkey, 01324 null_ctx_alloc, 01325 null_ctx_free 01326 }; 01327 01328 static const cipher_info_t null_cipher_info = { 01329 POLARSSL_CIPHER_NULL, 01330 POLARSSL_MODE_STREAM, 01331 0, 01332 "NULL", 01333 0, 01334 0, 01335 1, 01336 &null_base_info 01337 }; 01338 #endif /* defined(POLARSSL_CIPHER_NULL_CIPHER) */ 01339 01340 const cipher_definition_t cipher_definitions[] = 01341 { 01342 #if defined(POLARSSL_AES_C) 01343 { POLARSSL_CIPHER_AES_128_ECB, &aes_128_ecb_info }, 01344 { POLARSSL_CIPHER_AES_192_ECB, &aes_192_ecb_info }, 01345 { POLARSSL_CIPHER_AES_256_ECB, &aes_256_ecb_info }, 01346 #if defined(POLARSSL_CIPHER_MODE_CBC) 01347 { POLARSSL_CIPHER_AES_128_CBC, &aes_128_cbc_info }, 01348 { POLARSSL_CIPHER_AES_192_CBC, &aes_192_cbc_info }, 01349 { POLARSSL_CIPHER_AES_256_CBC, &aes_256_cbc_info }, 01350 #endif 01351 #if defined(POLARSSL_CIPHER_MODE_CFB) 01352 { POLARSSL_CIPHER_AES_128_CFB128, &aes_128_cfb128_info }, 01353 { POLARSSL_CIPHER_AES_192_CFB128, &aes_192_cfb128_info }, 01354 { POLARSSL_CIPHER_AES_256_CFB128, &aes_256_cfb128_info }, 01355 #endif 01356 #if defined(POLARSSL_CIPHER_MODE_CTR) 01357 { POLARSSL_CIPHER_AES_128_CTR, &aes_128_ctr_info }, 01358 { POLARSSL_CIPHER_AES_192_CTR, &aes_192_ctr_info }, 01359 { POLARSSL_CIPHER_AES_256_CTR, &aes_256_ctr_info }, 01360 #endif 01361 #if defined(POLARSSL_GCM_C) 01362 { POLARSSL_CIPHER_AES_128_GCM, &aes_128_gcm_info }, 01363 { POLARSSL_CIPHER_AES_192_GCM, &aes_192_gcm_info }, 01364 { POLARSSL_CIPHER_AES_256_GCM, &aes_256_gcm_info }, 01365 #endif 01366 #if defined(POLARSSL_CCM_C) 01367 { POLARSSL_CIPHER_AES_128_CCM, &aes_128_ccm_info }, 01368 { POLARSSL_CIPHER_AES_192_CCM, &aes_192_ccm_info }, 01369 { POLARSSL_CIPHER_AES_256_CCM, &aes_256_ccm_info }, 01370 #endif 01371 #endif /* POLARSSL_AES_C */ 01372 01373 #if defined(POLARSSL_ARC4_C) 01374 { POLARSSL_CIPHER_ARC4_128, &arc4_128_info }, 01375 #endif 01376 01377 #if defined(POLARSSL_BLOWFISH_C) 01378 { POLARSSL_CIPHER_BLOWFISH_ECB, &blowfish_ecb_info }, 01379 #if defined(POLARSSL_CIPHER_MODE_CBC) 01380 { POLARSSL_CIPHER_BLOWFISH_CBC, &blowfish_cbc_info }, 01381 #endif 01382 #if defined(POLARSSL_CIPHER_MODE_CFB) 01383 { POLARSSL_CIPHER_BLOWFISH_CFB64, &blowfish_cfb64_info }, 01384 #endif 01385 #if defined(POLARSSL_CIPHER_MODE_CTR) 01386 { POLARSSL_CIPHER_BLOWFISH_CTR, &blowfish_ctr_info }, 01387 #endif 01388 #endif /* POLARSSL_BLOWFISH_C */ 01389 01390 #if defined(POLARSSL_CAMELLIA_C) 01391 { POLARSSL_CIPHER_CAMELLIA_128_ECB, &camellia_128_ecb_info }, 01392 { POLARSSL_CIPHER_CAMELLIA_192_ECB, &camellia_192_ecb_info }, 01393 { POLARSSL_CIPHER_CAMELLIA_256_ECB, &camellia_256_ecb_info }, 01394 #if defined(POLARSSL_CIPHER_MODE_CBC) 01395 { POLARSSL_CIPHER_CAMELLIA_128_CBC, &camellia_128_cbc_info }, 01396 { POLARSSL_CIPHER_CAMELLIA_192_CBC, &camellia_192_cbc_info }, 01397 { POLARSSL_CIPHER_CAMELLIA_256_CBC, &camellia_256_cbc_info }, 01398 #endif 01399 #if defined(POLARSSL_CIPHER_MODE_CFB) 01400 { POLARSSL_CIPHER_CAMELLIA_128_CFB128, &camellia_128_cfb128_info }, 01401 { POLARSSL_CIPHER_CAMELLIA_192_CFB128, &camellia_192_cfb128_info }, 01402 { POLARSSL_CIPHER_CAMELLIA_256_CFB128, &camellia_256_cfb128_info }, 01403 #endif 01404 #if defined(POLARSSL_CIPHER_MODE_CTR) 01405 { POLARSSL_CIPHER_CAMELLIA_128_CTR, &camellia_128_ctr_info }, 01406 { POLARSSL_CIPHER_CAMELLIA_192_CTR, &camellia_192_ctr_info }, 01407 { POLARSSL_CIPHER_CAMELLIA_256_CTR, &camellia_256_ctr_info }, 01408 #endif 01409 #if defined(POLARSSL_GCM_C) 01410 { POLARSSL_CIPHER_CAMELLIA_128_GCM, &camellia_128_gcm_info }, 01411 { POLARSSL_CIPHER_CAMELLIA_192_GCM, &camellia_192_gcm_info }, 01412 { POLARSSL_CIPHER_CAMELLIA_256_GCM, &camellia_256_gcm_info }, 01413 #endif 01414 #if defined(POLARSSL_CCM_C) 01415 { POLARSSL_CIPHER_CAMELLIA_128_CCM, &camellia_128_ccm_info }, 01416 { POLARSSL_CIPHER_CAMELLIA_192_CCM, &camellia_192_ccm_info }, 01417 { POLARSSL_CIPHER_CAMELLIA_256_CCM, &camellia_256_ccm_info }, 01418 #endif 01419 #endif /* POLARSSL_CAMELLIA_C */ 01420 01421 #if defined(POLARSSL_DES_C) 01422 { POLARSSL_CIPHER_DES_ECB, &des_ecb_info }, 01423 { POLARSSL_CIPHER_DES_EDE_ECB, &des_ede_ecb_info }, 01424 { POLARSSL_CIPHER_DES_EDE3_ECB, &des_ede3_ecb_info }, 01425 #if defined(POLARSSL_CIPHER_MODE_CBC) 01426 { POLARSSL_CIPHER_DES_CBC, &des_cbc_info }, 01427 { POLARSSL_CIPHER_DES_EDE_CBC, &des_ede_cbc_info }, 01428 { POLARSSL_CIPHER_DES_EDE3_CBC, &des_ede3_cbc_info }, 01429 #endif 01430 #endif /* POLARSSL_DES_C */ 01431 01432 #if defined(POLARSSL_CIPHER_NULL_CIPHER) 01433 { POLARSSL_CIPHER_NULL, &null_cipher_info }, 01434 #endif /* POLARSSL_CIPHER_NULL_CIPHER */ 01435 01436 { POLARSSL_CIPHER_NONE, NULL } 01437 }; 01438 01439 #define NUM_CIPHERS sizeof cipher_definitions / sizeof cipher_definitions[0] 01440 int supported_ciphers[NUM_CIPHERS]; 01441 01442 #endif /* POLARSSL_CIPHER_C */ 01443
Generated on Tue Jul 12 2022 13:50:36 by
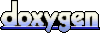