mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ccm.c
00001 /* 00002 * NIST SP800-38C compliant CCM implementation 00003 * 00004 * Copyright (C) 2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 /* 00024 * Definition of CCM: 00025 * http://csrc.nist.gov/publications/nistpubs/800-38C/SP800-38C_updated-July20_2007.pdf 00026 * RFC 3610 "Counter with CBC-MAC (CCM)" 00027 * 00028 * Related: 00029 * RFC 5116 "An Interface and Algorithms for Authenticated Encryption" 00030 */ 00031 00032 #if !defined(POLARSSL_CONFIG_FILE) 00033 #include "polarssl/config.h" 00034 #else 00035 #include POLARSSL_CONFIG_FILE 00036 #endif 00037 00038 #if defined(POLARSSL_CCM_C) 00039 00040 #include "polarssl/ccm.h" 00041 00042 #include <string.h> 00043 00044 #if defined(POLARSSL_SELF_TEST) && defined(POLARSSL_AES_C) 00045 #if defined(POLARSSL_PLATFORM_C) 00046 #include "polarssl/platform.h" 00047 #else 00048 #include <stdio.h> 00049 #define polarssl_printf printf 00050 #endif /* POLARSSL_PLATFORM_C */ 00051 #endif /* POLARSSL_SELF_TEST && POLARSSL_AES_C */ 00052 00053 /* Implementation that should never be optimized out by the compiler */ 00054 static void polarssl_zeroize( void *v, size_t n ) { 00055 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00056 } 00057 00058 #define CCM_ENCRYPT 0 00059 #define CCM_DECRYPT 1 00060 00061 /* 00062 * Initialize context 00063 */ 00064 int ccm_init( ccm_context *ctx, cipher_id_t cipher, 00065 const unsigned char *key, unsigned int keysize ) 00066 { 00067 int ret; 00068 const cipher_info_t *cipher_info; 00069 00070 memset( ctx, 0, sizeof( ccm_context ) ); 00071 00072 cipher_init( &ctx->cipher_ctx ); 00073 00074 cipher_info = cipher_info_from_values( cipher, keysize, POLARSSL_MODE_ECB ); 00075 if( cipher_info == NULL ) 00076 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00077 00078 if( cipher_info->block_size != 16 ) 00079 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00080 00081 cipher_free( &ctx->cipher_ctx ); 00082 00083 if( ( ret = cipher_init_ctx( &ctx->cipher_ctx , cipher_info ) ) != 0 ) 00084 return( ret ); 00085 00086 if( ( ret = cipher_setkey( &ctx->cipher_ctx , key, keysize, 00087 POLARSSL_ENCRYPT ) ) != 0 ) 00088 { 00089 return( ret ); 00090 } 00091 00092 return( 0 ); 00093 } 00094 00095 /* 00096 * Free context 00097 */ 00098 void ccm_free( ccm_context *ctx ) 00099 { 00100 cipher_free( &ctx->cipher_ctx ); 00101 polarssl_zeroize( ctx, sizeof( ccm_context ) ); 00102 } 00103 00104 /* 00105 * Macros for common operations. 00106 * Results in smaller compiled code than static inline functions. 00107 */ 00108 00109 /* 00110 * Update the CBC-MAC state in y using a block in b 00111 * (Always using b as the source helps the compiler optimise a bit better.) 00112 */ 00113 #define UPDATE_CBC_MAC \ 00114 for( i = 0; i < 16; i++ ) \ 00115 y[i] ^= b[i]; \ 00116 \ 00117 if( ( ret = cipher_update( &ctx->cipher_ctx, y, 16, y, &olen ) ) != 0 ) \ 00118 return( ret ); 00119 00120 /* 00121 * Encrypt or decrypt a partial block with CTR 00122 * Warning: using b for temporary storage! src and dst must not be b! 00123 * This avoids allocating one more 16 bytes buffer while allowing src == dst. 00124 */ 00125 #define CTR_CRYPT( dst, src, len ) \ 00126 if( ( ret = cipher_update( &ctx->cipher_ctx, ctr, 16, b, &olen ) ) != 0 ) \ 00127 return( ret ); \ 00128 \ 00129 for( i = 0; i < len; i++ ) \ 00130 dst[i] = src[i] ^ b[i]; 00131 00132 /* 00133 * Authenticated encryption or decryption 00134 */ 00135 static int ccm_auth_crypt( ccm_context *ctx, int mode, size_t length, 00136 const unsigned char *iv, size_t iv_len, 00137 const unsigned char *add, size_t add_len, 00138 const unsigned char *input, unsigned char *output, 00139 unsigned char *tag, size_t tag_len ) 00140 { 00141 int ret; 00142 unsigned char i; 00143 unsigned char q = 16 - 1 - iv_len; 00144 size_t len_left, olen; 00145 unsigned char b[16]; 00146 unsigned char y[16]; 00147 unsigned char ctr[16]; 00148 const unsigned char *src; 00149 unsigned char *dst; 00150 00151 /* 00152 * Check length requirements: SP800-38C A.1 00153 * Additional requirement: a < 2^16 - 2^8 to simplify the code. 00154 * 'length' checked later (when writing it to the first block) 00155 */ 00156 if( tag_len < 4 || tag_len > 16 || tag_len % 2 != 0 ) 00157 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00158 00159 /* Also implies q is within bounds */ 00160 if( iv_len < 7 || iv_len > 13 ) 00161 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00162 00163 if( add_len > 0xFF00 ) 00164 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00165 00166 /* 00167 * First block B_0: 00168 * 0 .. 0 flags 00169 * 1 .. iv_len nonce (aka iv) 00170 * iv_len+1 .. 15 length 00171 * 00172 * With flags as (bits): 00173 * 7 0 00174 * 6 add present? 00175 * 5 .. 3 (t - 2) / 2 00176 * 2 .. 0 q - 1 00177 */ 00178 b[0] = 0; 00179 b[0] |= ( add_len > 0 ) << 6; 00180 b[0] |= ( ( tag_len - 2 ) / 2 ) << 3; 00181 b[0] |= q - 1; 00182 00183 memcpy( b + 1, iv, iv_len ); 00184 00185 for( i = 0, len_left = length; i < q; i++, len_left >>= 8 ) 00186 b[15-i] = (unsigned char)( len_left & 0xFF ); 00187 00188 if( len_left > 0 ) 00189 return( POLARSSL_ERR_CCM_BAD_INPUT ); 00190 00191 00192 /* Start CBC-MAC with first block */ 00193 memset( y, 0, 16 ); 00194 UPDATE_CBC_MAC; 00195 00196 /* 00197 * If there is additional data, update CBC-MAC with 00198 * add_len, add, 0 (padding to a block boundary) 00199 */ 00200 if( add_len > 0 ) 00201 { 00202 size_t use_len; 00203 len_left = add_len; 00204 src = add; 00205 00206 memset( b, 0, 16 ); 00207 b[0] = (unsigned char)( ( add_len >> 8 ) & 0xFF ); 00208 b[1] = (unsigned char)( ( add_len ) & 0xFF ); 00209 00210 use_len = len_left < 16 - 2 ? len_left : 16 - 2; 00211 memcpy( b + 2, src, use_len ); 00212 len_left -= use_len; 00213 src += use_len; 00214 00215 UPDATE_CBC_MAC; 00216 00217 while( len_left > 0 ) 00218 { 00219 use_len = len_left > 16 ? 16 : len_left; 00220 00221 memset( b, 0, 16 ); 00222 memcpy( b, src, use_len ); 00223 UPDATE_CBC_MAC; 00224 00225 len_left -= use_len; 00226 src += use_len; 00227 } 00228 } 00229 00230 /* 00231 * Prepare counter block for encryption: 00232 * 0 .. 0 flags 00233 * 1 .. iv_len nonce (aka iv) 00234 * iv_len+1 .. 15 counter (initially 1) 00235 * 00236 * With flags as (bits): 00237 * 7 .. 3 0 00238 * 2 .. 0 q - 1 00239 */ 00240 ctr[0] = q - 1; 00241 memcpy( ctr + 1, iv, iv_len ); 00242 memset( ctr + 1 + iv_len, 0, q ); 00243 ctr[15] = 1; 00244 00245 /* 00246 * Authenticate and {en,de}crypt the message. 00247 * 00248 * The only difference between encryption and decryption is 00249 * the respective order of authentication and {en,de}cryption. 00250 */ 00251 len_left = length; 00252 src = input; 00253 dst = output; 00254 00255 while( len_left > 0 ) 00256 { 00257 unsigned char use_len = len_left > 16 ? 16 : len_left; 00258 00259 if( mode == CCM_ENCRYPT ) 00260 { 00261 memset( b, 0, 16 ); 00262 memcpy( b, src, use_len ); 00263 UPDATE_CBC_MAC; 00264 } 00265 00266 CTR_CRYPT( dst, src, use_len ); 00267 00268 if( mode == CCM_DECRYPT ) 00269 { 00270 memset( b, 0, 16 ); 00271 memcpy( b, dst, use_len ); 00272 UPDATE_CBC_MAC; 00273 } 00274 00275 dst += use_len; 00276 src += use_len; 00277 len_left -= use_len; 00278 00279 /* 00280 * Increment counter. 00281 * No need to check for overflow thanks to the length check above. 00282 */ 00283 for( i = 0; i < q; i++ ) 00284 if( ++ctr[15-i] != 0 ) 00285 break; 00286 } 00287 00288 /* 00289 * Authentication: reset counter and crypt/mask internal tag 00290 */ 00291 for( i = 0; i < q; i++ ) 00292 ctr[15-i] = 0; 00293 00294 CTR_CRYPT( y, y, 16 ); 00295 memcpy( tag, y, tag_len ); 00296 00297 return( 0 ); 00298 } 00299 00300 /* 00301 * Authenticated encryption 00302 */ 00303 int ccm_encrypt_and_tag( ccm_context *ctx, size_t length, 00304 const unsigned char *iv, size_t iv_len, 00305 const unsigned char *add, size_t add_len, 00306 const unsigned char *input, unsigned char *output, 00307 unsigned char *tag, size_t tag_len ) 00308 { 00309 return( ccm_auth_crypt( ctx, CCM_ENCRYPT, length, iv, iv_len, 00310 add, add_len, input, output, tag, tag_len ) ); 00311 } 00312 00313 /* 00314 * Authenticated decryption 00315 */ 00316 int ccm_auth_decrypt( ccm_context *ctx, size_t length, 00317 const unsigned char *iv, size_t iv_len, 00318 const unsigned char *add, size_t add_len, 00319 const unsigned char *input, unsigned char *output, 00320 const unsigned char *tag, size_t tag_len ) 00321 { 00322 int ret; 00323 unsigned char check_tag[16]; 00324 unsigned char i; 00325 int diff; 00326 00327 if( ( ret = ccm_auth_crypt( ctx, CCM_DECRYPT, length, 00328 iv, iv_len, add, add_len, 00329 input, output, check_tag, tag_len ) ) != 0 ) 00330 { 00331 return( ret ); 00332 } 00333 00334 /* Check tag in "constant-time" */ 00335 for( diff = 0, i = 0; i < tag_len; i++ ) 00336 diff |= tag[i] ^ check_tag[i]; 00337 00338 if( diff != 0 ) 00339 { 00340 polarssl_zeroize( output, length ); 00341 return( POLARSSL_ERR_CCM_AUTH_FAILED ); 00342 } 00343 00344 return( 0 ); 00345 } 00346 00347 00348 #if defined(POLARSSL_SELF_TEST) && defined(POLARSSL_AES_C) 00349 /* 00350 * Examples 1 to 3 from SP800-38C Appendix C 00351 */ 00352 00353 #define NB_TESTS 3 00354 00355 /* 00356 * The data is the same for all tests, only the used length changes 00357 */ 00358 static const unsigned char key[] = { 00359 0x40, 0x41, 0x42, 0x43, 0x44, 0x45, 0x46, 0x47, 00360 0x48, 0x49, 0x4a, 0x4b, 0x4c, 0x4d, 0x4e, 0x4f 00361 }; 00362 00363 static const unsigned char iv[] = { 00364 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 00365 0x18, 0x19, 0x1a, 0x1b 00366 }; 00367 00368 static const unsigned char ad[] = { 00369 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 00370 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f, 00371 0x10, 0x11, 0x12, 0x13 00372 }; 00373 00374 static const unsigned char msg[] = { 00375 0x20, 0x21, 0x22, 0x23, 0x24, 0x25, 0x26, 0x27, 00376 0x28, 0x29, 0x2a, 0x2b, 0x2c, 0x2d, 0x2e, 0x2f, 00377 0x30, 0x31, 0x32, 0x33, 0x34, 0x35, 0x36, 0x37, 00378 }; 00379 00380 static const size_t iv_len [NB_TESTS] = { 7, 8, 12 }; 00381 static const size_t add_len[NB_TESTS] = { 8, 16, 20 }; 00382 static const size_t msg_len[NB_TESTS] = { 4, 16, 24 }; 00383 static const size_t tag_len[NB_TESTS] = { 4, 6, 8 }; 00384 00385 static const unsigned char res[NB_TESTS][32] = { 00386 { 0x71, 0x62, 0x01, 0x5b, 0x4d, 0xac, 0x25, 0x5d }, 00387 { 0xd2, 0xa1, 0xf0, 0xe0, 0x51, 0xea, 0x5f, 0x62, 00388 0x08, 0x1a, 0x77, 0x92, 0x07, 0x3d, 0x59, 0x3d, 00389 0x1f, 0xc6, 0x4f, 0xbf, 0xac, 0xcd }, 00390 { 0xe3, 0xb2, 0x01, 0xa9, 0xf5, 0xb7, 0x1a, 0x7a, 00391 0x9b, 0x1c, 0xea, 0xec, 0xcd, 0x97, 0xe7, 0x0b, 00392 0x61, 0x76, 0xaa, 0xd9, 0xa4, 0x42, 0x8a, 0xa5, 00393 0x48, 0x43, 0x92, 0xfb, 0xc1, 0xb0, 0x99, 0x51 } 00394 }; 00395 00396 int ccm_self_test( int verbose ) 00397 { 00398 ccm_context ctx; 00399 unsigned char out[32]; 00400 size_t i; 00401 int ret; 00402 00403 if( ccm_init( &ctx, POLARSSL_CIPHER_ID_AES, key, 8 * sizeof key ) != 0 ) 00404 { 00405 if( verbose != 0 ) 00406 polarssl_printf( " CCM: setup failed" ); 00407 00408 return( 1 ); 00409 } 00410 00411 for( i = 0; i < NB_TESTS; i++ ) 00412 { 00413 if( verbose != 0 ) 00414 polarssl_printf( " CCM-AES #%u: ", (unsigned int) i + 1 ); 00415 00416 ret = ccm_encrypt_and_tag( &ctx, msg_len[i], 00417 iv, iv_len[i], ad, add_len[i], 00418 msg, out, 00419 out + msg_len[i], tag_len[i] ); 00420 00421 if( ret != 0 || 00422 memcmp( out, res[i], msg_len[i] + tag_len[i] ) != 0 ) 00423 { 00424 if( verbose != 0 ) 00425 polarssl_printf( "failed\n" ); 00426 00427 return( 1 ); 00428 } 00429 00430 ret = ccm_auth_decrypt( &ctx, msg_len[i], 00431 iv, iv_len[i], ad, add_len[i], 00432 res[i], out, 00433 res[i] + msg_len[i], tag_len[i] ); 00434 00435 if( ret != 0 || 00436 memcmp( out, msg, msg_len[i] ) != 0 ) 00437 { 00438 if( verbose != 0 ) 00439 polarssl_printf( "failed\n" ); 00440 00441 return( 1 ); 00442 } 00443 00444 if( verbose != 0 ) 00445 polarssl_printf( "passed\n" ); 00446 } 00447 00448 ccm_free( &ctx ); 00449 00450 if( verbose != 0 ) 00451 polarssl_printf( "\n" ); 00452 00453 return( 0 ); 00454 } 00455 00456 #endif /* POLARSSL_SELF_TEST && POLARSSL_AES_C */ 00457 00458 #endif /* POLARSSL_CCM_C */ 00459
Generated on Tue Jul 12 2022 13:50:36 by
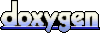