mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
camellia.h
00001 /** 00002 * \file camellia.h 00003 * 00004 * \brief Camellia block cipher 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_CAMELLIA_H 00025 #define POLARSSL_CAMELLIA_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 #define CAMELLIA_ENCRYPT 1 00043 #define CAMELLIA_DECRYPT 0 00044 00045 #define POLARSSL_ERR_CAMELLIA_INVALID_KEY_LENGTH -0x0024 /**< Invalid key length. */ 00046 #define POLARSSL_ERR_CAMELLIA_INVALID_INPUT_LENGTH -0x0026 /**< Invalid data input length. */ 00047 00048 #if !defined(POLARSSL_CAMELLIA_ALT) 00049 // Regular implementation 00050 // 00051 00052 #ifdef __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 /** 00057 * \brief CAMELLIA context structure 00058 */ 00059 typedef struct 00060 { 00061 int nr ; /*!< number of rounds */ 00062 uint32_t rk[68]; /*!< CAMELLIA round keys */ 00063 } 00064 camellia_context; 00065 00066 /** 00067 * \brief Initialize CAMELLIA context 00068 * 00069 * \param ctx CAMELLIA context to be initialized 00070 */ 00071 void camellia_init( camellia_context *ctx ); 00072 00073 /** 00074 * \brief Clear CAMELLIA context 00075 * 00076 * \param ctx CAMELLIA context to be cleared 00077 */ 00078 void camellia_free( camellia_context *ctx ); 00079 00080 /** 00081 * \brief CAMELLIA key schedule (encryption) 00082 * 00083 * \param ctx CAMELLIA context to be initialized 00084 * \param key encryption key 00085 * \param keysize must be 128, 192 or 256 00086 * 00087 * \return 0 if successful, or POLARSSL_ERR_CAMELLIA_INVALID_KEY_LENGTH 00088 */ 00089 int camellia_setkey_enc( camellia_context *ctx, const unsigned char *key, 00090 unsigned int keysize ); 00091 00092 /** 00093 * \brief CAMELLIA key schedule (decryption) 00094 * 00095 * \param ctx CAMELLIA context to be initialized 00096 * \param key decryption key 00097 * \param keysize must be 128, 192 or 256 00098 * 00099 * \return 0 if successful, or POLARSSL_ERR_CAMELLIA_INVALID_KEY_LENGTH 00100 */ 00101 int camellia_setkey_dec( camellia_context *ctx, const unsigned char *key, 00102 unsigned int keysize ); 00103 00104 /** 00105 * \brief CAMELLIA-ECB block encryption/decryption 00106 * 00107 * \param ctx CAMELLIA context 00108 * \param mode CAMELLIA_ENCRYPT or CAMELLIA_DECRYPT 00109 * \param input 16-byte input block 00110 * \param output 16-byte output block 00111 * 00112 * \return 0 if successful 00113 */ 00114 int camellia_crypt_ecb( camellia_context *ctx, 00115 int mode, 00116 const unsigned char input[16], 00117 unsigned char output[16] ); 00118 00119 #if defined(POLARSSL_CIPHER_MODE_CBC) 00120 /** 00121 * \brief CAMELLIA-CBC buffer encryption/decryption 00122 * Length should be a multiple of the block 00123 * size (16 bytes) 00124 * 00125 * \note Upon exit, the content of the IV is updated so that you can 00126 * call the function same function again on the following 00127 * block(s) of data and get the same result as if it was 00128 * encrypted in one call. This allows a "streaming" usage. 00129 * If on the other hand you need to retain the contents of the 00130 * IV, you should either save it manually or use the cipher 00131 * module instead. 00132 * 00133 * \param ctx CAMELLIA context 00134 * \param mode CAMELLIA_ENCRYPT or CAMELLIA_DECRYPT 00135 * \param length length of the input data 00136 * \param iv initialization vector (updated after use) 00137 * \param input buffer holding the input data 00138 * \param output buffer holding the output data 00139 * 00140 * \return 0 if successful, or 00141 * POLARSSL_ERR_CAMELLIA_INVALID_INPUT_LENGTH 00142 */ 00143 int camellia_crypt_cbc( camellia_context *ctx, 00144 int mode, 00145 size_t length, 00146 unsigned char iv[16], 00147 const unsigned char *input, 00148 unsigned char *output ); 00149 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00150 00151 #if defined(POLARSSL_CIPHER_MODE_CFB) 00152 /** 00153 * \brief CAMELLIA-CFB128 buffer encryption/decryption 00154 * 00155 * Note: Due to the nature of CFB you should use the same key schedule for 00156 * both encryption and decryption. So a context initialized with 00157 * camellia_setkey_enc() for both CAMELLIA_ENCRYPT and CAMELLIE_DECRYPT. 00158 * 00159 * \note Upon exit, the content of the IV is updated so that you can 00160 * call the function same function again on the following 00161 * block(s) of data and get the same result as if it was 00162 * encrypted in one call. This allows a "streaming" usage. 00163 * If on the other hand you need to retain the contents of the 00164 * IV, you should either save it manually or use the cipher 00165 * module instead. 00166 * 00167 * \param ctx CAMELLIA context 00168 * \param mode CAMELLIA_ENCRYPT or CAMELLIA_DECRYPT 00169 * \param length length of the input data 00170 * \param iv_off offset in IV (updated after use) 00171 * \param iv initialization vector (updated after use) 00172 * \param input buffer holding the input data 00173 * \param output buffer holding the output data 00174 * 00175 * \return 0 if successful, or 00176 * POLARSSL_ERR_CAMELLIA_INVALID_INPUT_LENGTH 00177 */ 00178 int camellia_crypt_cfb128( camellia_context *ctx, 00179 int mode, 00180 size_t length, 00181 size_t *iv_off, 00182 unsigned char iv[16], 00183 const unsigned char *input, 00184 unsigned char *output ); 00185 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00186 00187 #if defined(POLARSSL_CIPHER_MODE_CTR) 00188 /** 00189 * \brief CAMELLIA-CTR buffer encryption/decryption 00190 * 00191 * Warning: You have to keep the maximum use of your counter in mind! 00192 * 00193 * Note: Due to the nature of CTR you should use the same key schedule for 00194 * both encryption and decryption. So a context initialized with 00195 * camellia_setkey_enc() for both CAMELLIA_ENCRYPT and CAMELLIA_DECRYPT. 00196 * 00197 * \param ctx CAMELLIA context 00198 * \param length The length of the data 00199 * \param nc_off The offset in the current stream_block (for resuming 00200 * within current cipher stream). The offset pointer to 00201 * should be 0 at the start of a stream. 00202 * \param nonce_counter The 128-bit nonce and counter. 00203 * \param stream_block The saved stream-block for resuming. Is overwritten 00204 * by the function. 00205 * \param input The input data stream 00206 * \param output The output data stream 00207 * 00208 * \return 0 if successful 00209 */ 00210 int camellia_crypt_ctr( camellia_context *ctx, 00211 size_t length, 00212 size_t *nc_off, 00213 unsigned char nonce_counter[16], 00214 unsigned char stream_block[16], 00215 const unsigned char *input, 00216 unsigned char *output ); 00217 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00218 00219 #ifdef __cplusplus 00220 } 00221 #endif 00222 00223 #else /* POLARSSL_CAMELLIA_ALT */ 00224 #include "camellia_alt.h" 00225 #endif /* POLARSSL_CAMELLIA_ALT */ 00226 00227 #ifdef __cplusplus 00228 extern "C" { 00229 #endif 00230 00231 /** 00232 * \brief Checkup routine 00233 * 00234 * \return 0 if successful, or 1 if the test failed 00235 */ 00236 int camellia_self_test( int verbose ); 00237 00238 #ifdef __cplusplus 00239 } 00240 #endif 00241 00242 #endif /* camellia.h */ 00243
Generated on Tue Jul 12 2022 13:50:36 by
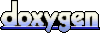