mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
blowfish.h
00001 /** 00002 * \file blowfish.h 00003 * 00004 * \brief Blowfish block cipher 00005 * 00006 * Copyright (C) 2012-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_BLOWFISH_H 00025 #define POLARSSL_BLOWFISH_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 #define BLOWFISH_ENCRYPT 1 00043 #define BLOWFISH_DECRYPT 0 00044 #define BLOWFISH_MAX_KEY 448 00045 #define BLOWFISH_MIN_KEY 32 00046 #define BLOWFISH_ROUNDS 16 /**< Rounds to use. When increasing this value, make sure to extend the initialisation vectors */ 00047 #define BLOWFISH_BLOCKSIZE 8 /* Blowfish uses 64 bit blocks */ 00048 00049 #define POLARSSL_ERR_BLOWFISH_INVALID_KEY_LENGTH -0x0016 /**< Invalid key length. */ 00050 #define POLARSSL_ERR_BLOWFISH_INVALID_INPUT_LENGTH -0x0018 /**< Invalid data input length. */ 00051 00052 #if !defined(POLARSSL_BLOWFISH_ALT) 00053 // Regular implementation 00054 // 00055 00056 #ifdef __cplusplus 00057 extern "C" { 00058 #endif 00059 00060 /** 00061 * \brief Blowfish context structure 00062 */ 00063 typedef struct 00064 { 00065 uint32_t P[BLOWFISH_ROUNDS + 2]; /*!< Blowfish round keys */ 00066 uint32_t S[4][256]; /*!< key dependent S-boxes */ 00067 } 00068 blowfish_context; 00069 00070 /** 00071 * \brief Initialize Blowfish context 00072 * 00073 * \param ctx Blowfish context to be initialized 00074 */ 00075 void blowfish_init( blowfish_context *ctx ); 00076 00077 /** 00078 * \brief Clear Blowfish context 00079 * 00080 * \param ctx Blowfish context to be cleared 00081 */ 00082 void blowfish_free( blowfish_context *ctx ); 00083 00084 /** 00085 * \brief Blowfish key schedule 00086 * 00087 * \param ctx Blowfish context to be initialized 00088 * \param key encryption key 00089 * \param keysize must be between 32 and 448 bits 00090 * 00091 * \return 0 if successful, or POLARSSL_ERR_BLOWFISH_INVALID_KEY_LENGTH 00092 */ 00093 int blowfish_setkey( blowfish_context *ctx, const unsigned char *key, 00094 unsigned int keysize ); 00095 00096 /** 00097 * \brief Blowfish-ECB block encryption/decryption 00098 * 00099 * \param ctx Blowfish context 00100 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00101 * \param input 8-byte input block 00102 * \param output 8-byte output block 00103 * 00104 * \return 0 if successful 00105 */ 00106 int blowfish_crypt_ecb( blowfish_context *ctx, 00107 int mode, 00108 const unsigned char input[BLOWFISH_BLOCKSIZE], 00109 unsigned char output[BLOWFISH_BLOCKSIZE] ); 00110 00111 #if defined(POLARSSL_CIPHER_MODE_CBC) 00112 /** 00113 * \brief Blowfish-CBC buffer encryption/decryption 00114 * Length should be a multiple of the block 00115 * size (8 bytes) 00116 * 00117 * \note Upon exit, the content of the IV is updated so that you can 00118 * call the function same function again on the following 00119 * block(s) of data and get the same result as if it was 00120 * encrypted in one call. This allows a "streaming" usage. 00121 * If on the other hand you need to retain the contents of the 00122 * IV, you should either save it manually or use the cipher 00123 * module instead. 00124 * 00125 * \param ctx Blowfish context 00126 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00127 * \param length length of the input data 00128 * \param iv initialization vector (updated after use) 00129 * \param input buffer holding the input data 00130 * \param output buffer holding the output data 00131 * 00132 * \return 0 if successful, or 00133 * POLARSSL_ERR_BLOWFISH_INVALID_INPUT_LENGTH 00134 */ 00135 int blowfish_crypt_cbc( blowfish_context *ctx, 00136 int mode, 00137 size_t length, 00138 unsigned char iv[BLOWFISH_BLOCKSIZE], 00139 const unsigned char *input, 00140 unsigned char *output ); 00141 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00142 00143 #if defined(POLARSSL_CIPHER_MODE_CFB) 00144 /** 00145 * \brief Blowfish CFB buffer encryption/decryption. 00146 * 00147 * \note Upon exit, the content of the IV is updated so that you can 00148 * call the function same function again on the following 00149 * block(s) of data and get the same result as if it was 00150 * encrypted in one call. This allows a "streaming" usage. 00151 * If on the other hand you need to retain the contents of the 00152 * IV, you should either save it manually or use the cipher 00153 * module instead. 00154 * 00155 * \param ctx Blowfish context 00156 * \param mode BLOWFISH_ENCRYPT or BLOWFISH_DECRYPT 00157 * \param length length of the input data 00158 * \param iv_off offset in IV (updated after use) 00159 * \param iv initialization vector (updated after use) 00160 * \param input buffer holding the input data 00161 * \param output buffer holding the output data 00162 * 00163 * \return 0 if successful 00164 */ 00165 int blowfish_crypt_cfb64( blowfish_context *ctx, 00166 int mode, 00167 size_t length, 00168 size_t *iv_off, 00169 unsigned char iv[BLOWFISH_BLOCKSIZE], 00170 const unsigned char *input, 00171 unsigned char *output ); 00172 #endif /*POLARSSL_CIPHER_MODE_CFB */ 00173 00174 #if defined(POLARSSL_CIPHER_MODE_CTR) 00175 /** 00176 * \brief Blowfish-CTR buffer encryption/decryption 00177 * 00178 * Warning: You have to keep the maximum use of your counter in mind! 00179 * 00180 * \param ctx Blowfish context 00181 * \param length The length of the data 00182 * \param nc_off The offset in the current stream_block (for resuming 00183 * within current cipher stream). The offset pointer to 00184 * should be 0 at the start of a stream. 00185 * \param nonce_counter The 64-bit nonce and counter. 00186 * \param stream_block The saved stream-block for resuming. Is overwritten 00187 * by the function. 00188 * \param input The input data stream 00189 * \param output The output data stream 00190 * 00191 * \return 0 if successful 00192 */ 00193 int blowfish_crypt_ctr( blowfish_context *ctx, 00194 size_t length, 00195 size_t *nc_off, 00196 unsigned char nonce_counter[BLOWFISH_BLOCKSIZE], 00197 unsigned char stream_block[BLOWFISH_BLOCKSIZE], 00198 const unsigned char *input, 00199 unsigned char *output ); 00200 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00201 00202 #ifdef __cplusplus 00203 } 00204 #endif 00205 00206 #else /* POLARSSL_BLOWFISH_ALT */ 00207 #include "blowfish_alt.h" 00208 #endif /* POLARSSL_BLOWFISH_ALT */ 00209 00210 #endif /* blowfish.h */ 00211
Generated on Tue Jul 12 2022 13:50:36 by
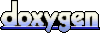