mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
base64.h
00001 /** 00002 * \file base64.h 00003 * 00004 * \brief RFC 1521 base64 encoding/decoding 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_BASE64_H 00025 #define POLARSSL_BASE64_H 00026 00027 #include <stddef.h> 00028 00029 #define POLARSSL_ERR_BASE64_BUFFER_TOO_SMALL -0x002A /**< Output buffer too small. */ 00030 #define POLARSSL_ERR_BASE64_INVALID_CHARACTER -0x002C /**< Invalid character in input. */ 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /** 00037 * \brief Encode a buffer into base64 format 00038 * 00039 * \param dst destination buffer 00040 * \param dlen size of the buffer 00041 * \param src source buffer 00042 * \param slen amount of data to be encoded 00043 * 00044 * \return 0 if successful, or POLARSSL_ERR_BASE64_BUFFER_TOO_SMALL. 00045 * *dlen is always updated to reflect the amount 00046 * of data that has (or would have) been written. 00047 * 00048 * \note Call this function with *dlen = 0 to obtain the 00049 * required buffer size in *dlen 00050 */ 00051 int base64_encode( unsigned char *dst, size_t *dlen, 00052 const unsigned char *src, size_t slen ); 00053 00054 /** 00055 * \brief Decode a base64-formatted buffer 00056 * 00057 * \param dst destination buffer (can be NULL for checking size) 00058 * \param dlen size of the buffer 00059 * \param src source buffer 00060 * \param slen amount of data to be decoded 00061 * 00062 * \return 0 if successful, POLARSSL_ERR_BASE64_BUFFER_TOO_SMALL, or 00063 * POLARSSL_ERR_BASE64_INVALID_CHARACTER if the input data is 00064 * not correct. *dlen is always updated to reflect the amount 00065 * of data that has (or would have) been written. 00066 * 00067 * \note Call this function with *dst = NULL or *dlen = 0 to obtain 00068 * the required buffer size in *dlen 00069 */ 00070 int base64_decode( unsigned char *dst, size_t *dlen, 00071 const unsigned char *src, size_t slen ); 00072 00073 /** 00074 * \brief Checkup routine 00075 * 00076 * \return 0 if successful, or 1 if the test failed 00077 */ 00078 int base64_self_test( int verbose ); 00079 00080 #ifdef __cplusplus 00081 } 00082 #endif 00083 00084 #endif /* base64.h */ 00085
Generated on Tue Jul 12 2022 13:50:36 by
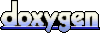