mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
asn1write.h
00001 /** 00002 * \file asn1write.h 00003 * 00004 * \brief ASN.1 buffer writing functionality 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ASN1_WRITE_H 00025 #define POLARSSL_ASN1_WRITE_H 00026 00027 #include "asn1.h" 00028 00029 #define ASN1_CHK_ADD(g, f) do { if( ( ret = f ) < 0 ) return( ret ); else \ 00030 g += ret; } while( 0 ) 00031 00032 #ifdef __cplusplus 00033 extern "C" { 00034 #endif 00035 00036 /** 00037 * \brief Write a length field in ASN.1 format 00038 * Note: function works backwards in data buffer 00039 * 00040 * \param p reference to current position pointer 00041 * \param start start of the buffer (for bounds-checking) 00042 * \param len the length to write 00043 * 00044 * \return the length written or a negative error code 00045 */ 00046 int asn1_write_len( unsigned char **p, unsigned char *start, size_t len ); 00047 00048 /** 00049 * \brief Write a ASN.1 tag in ASN.1 format 00050 * Note: function works backwards in data buffer 00051 * 00052 * \param p reference to current position pointer 00053 * \param start start of the buffer (for bounds-checking) 00054 * \param tag the tag to write 00055 * 00056 * \return the length written or a negative error code 00057 */ 00058 int asn1_write_tag( unsigned char **p, unsigned char *start, 00059 unsigned char tag ); 00060 00061 /** 00062 * \brief Write raw buffer data 00063 * Note: function works backwards in data buffer 00064 * 00065 * \param p reference to current position pointer 00066 * \param start start of the buffer (for bounds-checking) 00067 * \param buf data buffer to write 00068 * \param size length of the data buffer 00069 * 00070 * \return the length written or a negative error code 00071 */ 00072 int asn1_write_raw_buffer( unsigned char **p, unsigned char *start, 00073 const unsigned char *buf, size_t size ); 00074 00075 #if defined(POLARSSL_BIGNUM_C) 00076 /** 00077 * \brief Write a big number (ASN1_INTEGER) in ASN.1 format 00078 * Note: function works backwards in data buffer 00079 * 00080 * \param p reference to current position pointer 00081 * \param start start of the buffer (for bounds-checking) 00082 * \param X the MPI to write 00083 * 00084 * \return the length written or a negative error code 00085 */ 00086 int asn1_write_mpi( unsigned char **p, unsigned char *start, mpi *X ); 00087 #endif /* POLARSSL_BIGNUM_C */ 00088 00089 /** 00090 * \brief Write a NULL tag (ASN1_NULL) with zero data in ASN.1 format 00091 * Note: function works backwards in data buffer 00092 * 00093 * \param p reference to current position pointer 00094 * \param start start of the buffer (for bounds-checking) 00095 * 00096 * \return the length written or a negative error code 00097 */ 00098 int asn1_write_null( unsigned char **p, unsigned char *start ); 00099 00100 /** 00101 * \brief Write an OID tag (ASN1_OID) and data in ASN.1 format 00102 * Note: function works backwards in data buffer 00103 * 00104 * \param p reference to current position pointer 00105 * \param start start of the buffer (for bounds-checking) 00106 * \param oid the OID to write 00107 * \param oid_len length of the OID 00108 * 00109 * \return the length written or a negative error code 00110 */ 00111 int asn1_write_oid( unsigned char **p, unsigned char *start, 00112 const char *oid, size_t oid_len ); 00113 00114 /** 00115 * \brief Write an AlgorithmIdentifier sequence in ASN.1 format 00116 * Note: function works backwards in data buffer 00117 * 00118 * \param p reference to current position pointer 00119 * \param start start of the buffer (for bounds-checking) 00120 * \param oid the OID of the algorithm 00121 * \param oid_len length of the OID 00122 * \param par_len length of parameters, which must be already written. 00123 * If 0, NULL parameters are added 00124 * 00125 * \return the length written or a negative error code 00126 */ 00127 int asn1_write_algorithm_identifier( unsigned char **p, unsigned char *start, 00128 const char *oid, size_t oid_len, 00129 size_t par_len ); 00130 00131 /** 00132 * \brief Write a boolean tag (ASN1_BOOLEAN) and value in ASN.1 format 00133 * Note: function works backwards in data buffer 00134 * 00135 * \param p reference to current position pointer 00136 * \param start start of the buffer (for bounds-checking) 00137 * \param boolean 0 or 1 00138 * 00139 * \return the length written or a negative error code 00140 */ 00141 int asn1_write_bool( unsigned char **p, unsigned char *start, int boolean ); 00142 00143 /** 00144 * \brief Write an int tag (ASN1_INTEGER) and value in ASN.1 format 00145 * Note: function works backwards in data buffer 00146 * 00147 * \param p reference to current position pointer 00148 * \param start start of the buffer (for bounds-checking) 00149 * \param val the integer value 00150 * 00151 * \return the length written or a negative error code 00152 */ 00153 int asn1_write_int( unsigned char **p, unsigned char *start, int val ); 00154 00155 /** 00156 * \brief Write a printable string tag (ASN1_PRINTABLE_STRING) and 00157 * value in ASN.1 format 00158 * Note: function works backwards in data buffer 00159 * 00160 * \param p reference to current position pointer 00161 * \param start start of the buffer (for bounds-checking) 00162 * \param text the text to write 00163 * \param text_len length of the text 00164 * 00165 * \return the length written or a negative error code 00166 */ 00167 int asn1_write_printable_string( unsigned char **p, unsigned char *start, 00168 const char *text, size_t text_len ); 00169 00170 /** 00171 * \brief Write an IA5 string tag (ASN1_IA5_STRING) and 00172 * value in ASN.1 format 00173 * Note: function works backwards in data buffer 00174 * 00175 * \param p reference to current position pointer 00176 * \param start start of the buffer (for bounds-checking) 00177 * \param text the text to write 00178 * \param text_len length of the text 00179 * 00180 * \return the length written or a negative error code 00181 */ 00182 int asn1_write_ia5_string( unsigned char **p, unsigned char *start, 00183 const char *text, size_t text_len ); 00184 00185 /** 00186 * \brief Write a bitstring tag (ASN1_BIT_STRING) and 00187 * value in ASN.1 format 00188 * Note: function works backwards in data buffer 00189 * 00190 * \param p reference to current position pointer 00191 * \param start start of the buffer (for bounds-checking) 00192 * \param buf the bitstring 00193 * \param bits the total number of bits in the bitstring 00194 * 00195 * \return the length written or a negative error code 00196 */ 00197 int asn1_write_bitstring( unsigned char **p, unsigned char *start, 00198 const unsigned char *buf, size_t bits ); 00199 00200 /** 00201 * \brief Write an octet string tag (ASN1_OCTET_STRING) and 00202 * value in ASN.1 format 00203 * Note: function works backwards in data buffer 00204 * 00205 * \param p reference to current position pointer 00206 * \param start start of the buffer (for bounds-checking) 00207 * \param buf data buffer to write 00208 * \param size length of the data buffer 00209 * 00210 * \return the length written or a negative error code 00211 */ 00212 int asn1_write_octet_string( unsigned char **p, unsigned char *start, 00213 const unsigned char *buf, size_t size ); 00214 00215 /** 00216 * \brief Create or find a specific named_data entry for writing in a 00217 * sequence or list based on the OID. If not already in there, 00218 * a new entry is added to the head of the list. 00219 * Warning: Destructive behaviour for the val data! 00220 * 00221 * \param list Pointer to the location of the head of the list to seek 00222 * through (will be updated in case of a new entry) 00223 * \param oid The OID to look for 00224 * \param oid_len Size of the OID 00225 * \param val Data to store (can be NULL if you want to fill it by hand) 00226 * \param val_len Minimum length of the data buffer needed 00227 * 00228 * \return NULL if if there was a memory allocation error, or a pointer 00229 * to the new / existing entry. 00230 */ 00231 asn1_named_data *asn1_store_named_data( asn1_named_data **list, 00232 const char *oid, size_t oid_len, 00233 const unsigned char *val, 00234 size_t val_len ); 00235 00236 #ifdef __cplusplus 00237 } 00238 #endif 00239 00240 #endif /* POLARSSL_ASN1_WRITE_H */ 00241
Generated on Tue Jul 12 2022 13:50:36 by
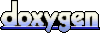