mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
arc4.h
00001 /** 00002 * \file arc4.h 00003 * 00004 * \brief The ARCFOUR stream cipher 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_ARC4_H 00025 #define POLARSSL_ARC4_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if !defined(POLARSSL_ARC4_ALT) 00036 // Regular implementation 00037 // 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /** 00044 * \brief ARC4 context structure 00045 */ 00046 typedef struct 00047 { 00048 int x ; /*!< permutation index */ 00049 int y ; /*!< permutation index */ 00050 unsigned char m[256]; /*!< permutation table */ 00051 } 00052 arc4_context; 00053 00054 /** 00055 * \brief Initialize ARC4 context 00056 * 00057 * \param ctx ARC4 context to be initialized 00058 */ 00059 void arc4_init( arc4_context *ctx ); 00060 00061 /** 00062 * \brief Clear ARC4 context 00063 * 00064 * \param ctx ARC4 context to be cleared 00065 */ 00066 void arc4_free( arc4_context *ctx ); 00067 00068 /** 00069 * \brief ARC4 key schedule 00070 * 00071 * \param ctx ARC4 context to be setup 00072 * \param key the secret key 00073 * \param keylen length of the key, in bytes 00074 */ 00075 void arc4_setup( arc4_context *ctx, const unsigned char *key, 00076 unsigned int keylen ); 00077 00078 /** 00079 * \brief ARC4 cipher function 00080 * 00081 * \param ctx ARC4 context 00082 * \param length length of the input data 00083 * \param input buffer holding the input data 00084 * \param output buffer for the output data 00085 * 00086 * \return 0 if successful 00087 */ 00088 int arc4_crypt( arc4_context *ctx, size_t length, const unsigned char *input, 00089 unsigned char *output ); 00090 00091 #ifdef __cplusplus 00092 } 00093 #endif 00094 00095 #else /* POLARSSL_ARC4_ALT */ 00096 #include "arc4_alt.h" 00097 #endif /* POLARSSL_ARC4_ALT */ 00098 00099 #ifdef __cplusplus 00100 extern "C" { 00101 #endif 00102 00103 /** 00104 * \brief Checkup routine 00105 * 00106 * \return 0 if successful, or 1 if the test failed 00107 */ 00108 int arc4_self_test( int verbose ); 00109 00110 #ifdef __cplusplus 00111 } 00112 #endif 00113 00114 #endif /* arc4.h */ 00115
Generated on Tue Jul 12 2022 13:50:36 by
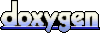