mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
arc4.c
00001 /* 00002 * An implementation of the ARCFOUR algorithm 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 /* 00023 * The ARCFOUR algorithm was publicly disclosed on 94/09. 00024 * 00025 * http://groups.google.com/group/sci.crypt/msg/10a300c9d21afca0 00026 */ 00027 00028 #if !defined(POLARSSL_CONFIG_FILE) 00029 #include "polarssl/config.h" 00030 #else 00031 #include POLARSSL_CONFIG_FILE 00032 #endif 00033 00034 #if defined(POLARSSL_ARC4_C) 00035 00036 #include "polarssl/arc4.h" 00037 00038 #include <string.h> 00039 00040 #if defined(POLARSSL_SELF_TEST) 00041 #if defined(POLARSSL_PLATFORM_C) 00042 #include "polarssl/platform.h" 00043 #else 00044 #include <stdio.h> 00045 #define polarssl_printf printf 00046 #endif /* POLARSSL_PLATFORM_C */ 00047 #endif /* POLARSSL_SELF_TEST */ 00048 00049 #if !defined(POLARSSL_ARC4_ALT) 00050 00051 /* Implementation that should never be optimized out by the compiler */ 00052 static void polarssl_zeroize( void *v, size_t n ) { 00053 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00054 } 00055 00056 void arc4_init( arc4_context *ctx ) 00057 { 00058 memset( ctx, 0, sizeof( arc4_context ) ); 00059 } 00060 00061 void arc4_free( arc4_context *ctx ) 00062 { 00063 if( ctx == NULL ) 00064 return; 00065 00066 polarssl_zeroize( ctx, sizeof( arc4_context ) ); 00067 } 00068 00069 /* 00070 * ARC4 key schedule 00071 */ 00072 void arc4_setup( arc4_context *ctx, const unsigned char *key, 00073 unsigned int keylen ) 00074 { 00075 int i, j, a; 00076 unsigned int k; 00077 unsigned char *m; 00078 00079 ctx->x = 0; 00080 ctx->y = 0; 00081 m = ctx->m ; 00082 00083 for( i = 0; i < 256; i++ ) 00084 m[i] = (unsigned char) i; 00085 00086 j = k = 0; 00087 00088 for( i = 0; i < 256; i++, k++ ) 00089 { 00090 if( k >= keylen ) k = 0; 00091 00092 a = m[i]; 00093 j = ( j + a + key[k] ) & 0xFF; 00094 m[i] = m[j]; 00095 m[j] = (unsigned char) a; 00096 } 00097 } 00098 00099 /* 00100 * ARC4 cipher function 00101 */ 00102 int arc4_crypt( arc4_context *ctx, size_t length, const unsigned char *input, 00103 unsigned char *output ) 00104 { 00105 int x, y, a, b; 00106 size_t i; 00107 unsigned char *m; 00108 00109 x = ctx->x ; 00110 y = ctx->y ; 00111 m = ctx->m ; 00112 00113 for( i = 0; i < length; i++ ) 00114 { 00115 x = ( x + 1 ) & 0xFF; a = m[x]; 00116 y = ( y + a ) & 0xFF; b = m[y]; 00117 00118 m[x] = (unsigned char) b; 00119 m[y] = (unsigned char) a; 00120 00121 output[i] = (unsigned char) 00122 ( input[i] ^ m[(unsigned char)( a + b )] ); 00123 } 00124 00125 ctx->x = x; 00126 ctx->y = y; 00127 00128 return( 0 ); 00129 } 00130 00131 #endif /* !POLARSSL_ARC4_ALT */ 00132 00133 #if defined(POLARSSL_SELF_TEST) 00134 /* 00135 * ARC4 tests vectors as posted by Eric Rescorla in sep. 1994: 00136 * 00137 * http://groups.google.com/group/comp.security.misc/msg/10a300c9d21afca0 00138 */ 00139 static const unsigned char arc4_test_key[3][8] = 00140 { 00141 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF }, 00142 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF }, 00143 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00144 }; 00145 00146 static const unsigned char arc4_test_pt[3][8] = 00147 { 00148 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF }, 00149 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }, 00150 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00151 }; 00152 00153 static const unsigned char arc4_test_ct[3][8] = 00154 { 00155 { 0x75, 0xB7, 0x87, 0x80, 0x99, 0xE0, 0xC5, 0x96 }, 00156 { 0x74, 0x94, 0xC2, 0xE7, 0x10, 0x4B, 0x08, 0x79 }, 00157 { 0xDE, 0x18, 0x89, 0x41, 0xA3, 0x37, 0x5D, 0x3A } 00158 }; 00159 00160 /* 00161 * Checkup routine 00162 */ 00163 int arc4_self_test( int verbose ) 00164 { 00165 int i, ret = 0; 00166 unsigned char ibuf[8]; 00167 unsigned char obuf[8]; 00168 arc4_context ctx; 00169 00170 arc4_init( &ctx ); 00171 00172 for( i = 0; i < 3; i++ ) 00173 { 00174 if( verbose != 0 ) 00175 polarssl_printf( " ARC4 test #%d: ", i + 1 ); 00176 00177 memcpy( ibuf, arc4_test_pt[i], 8 ); 00178 00179 arc4_setup( &ctx, arc4_test_key[i], 8 ); 00180 arc4_crypt( &ctx, 8, ibuf, obuf ); 00181 00182 if( memcmp( obuf, arc4_test_ct[i], 8 ) != 0 ) 00183 { 00184 if( verbose != 0 ) 00185 polarssl_printf( "failed\n" ); 00186 00187 ret = 1; 00188 goto exit; 00189 } 00190 00191 if( verbose != 0 ) 00192 polarssl_printf( "passed\n" ); 00193 } 00194 00195 if( verbose != 0 ) 00196 polarssl_printf( "\n" ); 00197 00198 exit: 00199 arc4_free( &ctx ); 00200 00201 return( ret ); 00202 } 00203 00204 #endif /* POLARSSL_SELF_TEST */ 00205 00206 #endif /* POLARSSL_ARC4_C */ 00207
Generated on Tue Jul 12 2022 13:50:36 by
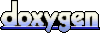