mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
aes.h
00001 /** 00002 * \file aes.h 00003 * 00004 * \brief AES block cipher 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_AES_H 00025 #define POLARSSL_AES_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include <stddef.h> 00034 00035 #if defined(_MSC_VER) && !defined(EFIX64) && !defined(EFI32) 00036 #include <basetsd.h> 00037 typedef UINT32 uint32_t; 00038 #else 00039 #include <inttypes.h> 00040 #endif 00041 00042 /* padlock.c and aesni.c rely on these values! */ 00043 #define AES_ENCRYPT 1 00044 #define AES_DECRYPT 0 00045 00046 #define POLARSSL_ERR_AES_INVALID_KEY_LENGTH -0x0020 /**< Invalid key length. */ 00047 #define POLARSSL_ERR_AES_INVALID_INPUT_LENGTH -0x0022 /**< Invalid data input length. */ 00048 00049 #if !defined(POLARSSL_AES_ALT) 00050 // Regular implementation 00051 // 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /** 00058 * \brief AES context structure 00059 * 00060 * \note buf is able to hold 32 extra bytes, which can be used: 00061 * - for alignment purposes if VIA padlock is used, and/or 00062 * - to simplify key expansion in the 256-bit case by 00063 * generating an extra round key 00064 */ 00065 typedef struct 00066 { 00067 int nr ; /*!< number of rounds */ 00068 uint32_t *rk ; /*!< AES round keys */ 00069 uint32_t buf[68]; /*!< unaligned data */ 00070 } 00071 aes_context; 00072 00073 /** 00074 * \brief Initialize AES context 00075 * 00076 * \param ctx AES context to be initialized 00077 */ 00078 void aes_init( aes_context *ctx ); 00079 00080 /** 00081 * \brief Clear AES context 00082 * 00083 * \param ctx AES context to be cleared 00084 */ 00085 void aes_free( aes_context *ctx ); 00086 00087 /** 00088 * \brief AES key schedule (encryption) 00089 * 00090 * \param ctx AES context to be initialized 00091 * \param key encryption key 00092 * \param keysize must be 128, 192 or 256 00093 * 00094 * \return 0 if successful, or POLARSSL_ERR_AES_INVALID_KEY_LENGTH 00095 */ 00096 int aes_setkey_enc( aes_context *ctx, const unsigned char *key, 00097 unsigned int keysize ); 00098 00099 /** 00100 * \brief AES key schedule (decryption) 00101 * 00102 * \param ctx AES context to be initialized 00103 * \param key decryption key 00104 * \param keysize must be 128, 192 or 256 00105 * 00106 * \return 0 if successful, or POLARSSL_ERR_AES_INVALID_KEY_LENGTH 00107 */ 00108 int aes_setkey_dec( aes_context *ctx, const unsigned char *key, 00109 unsigned int keysize ); 00110 00111 /** 00112 * \brief AES-ECB block encryption/decryption 00113 * 00114 * \param ctx AES context 00115 * \param mode AES_ENCRYPT or AES_DECRYPT 00116 * \param input 16-byte input block 00117 * \param output 16-byte output block 00118 * 00119 * \return 0 if successful 00120 */ 00121 int aes_crypt_ecb( aes_context *ctx, 00122 int mode, 00123 const unsigned char input[16], 00124 unsigned char output[16] ); 00125 00126 #if defined(POLARSSL_CIPHER_MODE_CBC) 00127 /** 00128 * \brief AES-CBC buffer encryption/decryption 00129 * Length should be a multiple of the block 00130 * size (16 bytes) 00131 * 00132 * \note Upon exit, the content of the IV is updated so that you can 00133 * call the function same function again on the following 00134 * block(s) of data and get the same result as if it was 00135 * encrypted in one call. This allows a "streaming" usage. 00136 * If on the other hand you need to retain the contents of the 00137 * IV, you should either save it manually or use the cipher 00138 * module instead. 00139 * 00140 * \param ctx AES context 00141 * \param mode AES_ENCRYPT or AES_DECRYPT 00142 * \param length length of the input data 00143 * \param iv initialization vector (updated after use) 00144 * \param input buffer holding the input data 00145 * \param output buffer holding the output data 00146 * 00147 * \return 0 if successful, or POLARSSL_ERR_AES_INVALID_INPUT_LENGTH 00148 */ 00149 int aes_crypt_cbc( aes_context *ctx, 00150 int mode, 00151 size_t length, 00152 unsigned char iv[16], 00153 const unsigned char *input, 00154 unsigned char *output ); 00155 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00156 00157 #if defined(POLARSSL_CIPHER_MODE_CFB) 00158 /** 00159 * \brief AES-CFB128 buffer encryption/decryption. 00160 * 00161 * Note: Due to the nature of CFB you should use the same key schedule for 00162 * both encryption and decryption. So a context initialized with 00163 * aes_setkey_enc() for both AES_ENCRYPT and AES_DECRYPT. 00164 * 00165 * \note Upon exit, the content of the IV is updated so that you can 00166 * call the function same function again on the following 00167 * block(s) of data and get the same result as if it was 00168 * encrypted in one call. This allows a "streaming" usage. 00169 * If on the other hand you need to retain the contents of the 00170 * IV, you should either save it manually or use the cipher 00171 * module instead. 00172 * 00173 * \param ctx AES context 00174 * \param mode AES_ENCRYPT or AES_DECRYPT 00175 * \param length length of the input data 00176 * \param iv_off offset in IV (updated after use) 00177 * \param iv initialization vector (updated after use) 00178 * \param input buffer holding the input data 00179 * \param output buffer holding the output data 00180 * 00181 * \return 0 if successful 00182 */ 00183 int aes_crypt_cfb128( aes_context *ctx, 00184 int mode, 00185 size_t length, 00186 size_t *iv_off, 00187 unsigned char iv[16], 00188 const unsigned char *input, 00189 unsigned char *output ); 00190 00191 /** 00192 * \brief AES-CFB8 buffer encryption/decryption. 00193 * 00194 * Note: Due to the nature of CFB you should use the same key schedule for 00195 * both encryption and decryption. So a context initialized with 00196 * aes_setkey_enc() for both AES_ENCRYPT and AES_DECRYPT. 00197 * 00198 * \note Upon exit, the content of the IV is updated so that you can 00199 * call the function same function again on the following 00200 * block(s) of data and get the same result as if it was 00201 * encrypted in one call. This allows a "streaming" usage. 00202 * If on the other hand you need to retain the contents of the 00203 * IV, you should either save it manually or use the cipher 00204 * module instead. 00205 * 00206 * \param ctx AES context 00207 * \param mode AES_ENCRYPT or AES_DECRYPT 00208 * \param length length of the input data 00209 * \param iv initialization vector (updated after use) 00210 * \param input buffer holding the input data 00211 * \param output buffer holding the output data 00212 * 00213 * \return 0 if successful 00214 */ 00215 int aes_crypt_cfb8( aes_context *ctx, 00216 int mode, 00217 size_t length, 00218 unsigned char iv[16], 00219 const unsigned char *input, 00220 unsigned char *output ); 00221 #endif /*POLARSSL_CIPHER_MODE_CFB */ 00222 00223 #if defined(POLARSSL_CIPHER_MODE_CTR) 00224 /** 00225 * \brief AES-CTR buffer encryption/decryption 00226 * 00227 * Warning: You have to keep the maximum use of your counter in mind! 00228 * 00229 * Note: Due to the nature of CTR you should use the same key schedule for 00230 * both encryption and decryption. So a context initialized with 00231 * aes_setkey_enc() for both AES_ENCRYPT and AES_DECRYPT. 00232 * 00233 * \param ctx AES context 00234 * \param length The length of the data 00235 * \param nc_off The offset in the current stream_block (for resuming 00236 * within current cipher stream). The offset pointer to 00237 * should be 0 at the start of a stream. 00238 * \param nonce_counter The 128-bit nonce and counter. 00239 * \param stream_block The saved stream-block for resuming. Is overwritten 00240 * by the function. 00241 * \param input The input data stream 00242 * \param output The output data stream 00243 * 00244 * \return 0 if successful 00245 */ 00246 int aes_crypt_ctr( aes_context *ctx, 00247 size_t length, 00248 size_t *nc_off, 00249 unsigned char nonce_counter[16], 00250 unsigned char stream_block[16], 00251 const unsigned char *input, 00252 unsigned char *output ); 00253 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00254 00255 #ifdef __cplusplus 00256 } 00257 #endif 00258 00259 #else /* POLARSSL_AES_ALT */ 00260 #include "aes_alt.h" 00261 #endif /* POLARSSL_AES_ALT */ 00262 00263 #ifdef __cplusplus 00264 extern "C" { 00265 #endif 00266 00267 /** 00268 * \brief Checkup routine 00269 * 00270 * \return 0 if successful, or 1 if the test failed 00271 */ 00272 int aes_self_test( int verbose ); 00273 00274 #ifdef __cplusplus 00275 } 00276 #endif 00277 00278 #endif /* aes.h */ 00279
Generated on Tue Jul 12 2022 13:50:36 by
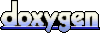