mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
aes.c
00001 /* 00002 * FIPS-197 compliant AES implementation 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 /* 00023 * The AES block cipher was designed by Vincent Rijmen and Joan Daemen. 00024 * 00025 * http://csrc.nist.gov/encryption/aes/rijndael/Rijndael.pdf 00026 * http://csrc.nist.gov/publications/fips/fips197/fips-197.pdf 00027 */ 00028 00029 #if !defined(POLARSSL_CONFIG_FILE) 00030 #include "polarssl/config.h" 00031 #else 00032 #include POLARSSL_CONFIG_FILE 00033 #endif 00034 00035 #if defined(POLARSSL_AES_C) 00036 00037 #include <string.h> 00038 00039 #include "polarssl/aes.h" 00040 #if defined(POLARSSL_PADLOCK_C) 00041 #include "polarssl/padlock.h" 00042 #endif 00043 #if defined(POLARSSL_AESNI_C) 00044 #include "polarssl/aesni.h" 00045 #endif 00046 00047 #if defined(POLARSSL_SELF_TEST) 00048 #if defined(POLARSSL_PLATFORM_C) 00049 #include "polarssl/platform.h" 00050 #else 00051 #include <stdio.h> 00052 #define polarssl_printf printf 00053 #endif /* POLARSSL_PLATFORM_C */ 00054 #endif /* POLARSSL_SELF_TEST */ 00055 00056 #if !defined(POLARSSL_AES_ALT) 00057 00058 /* Implementation that should never be optimized out by the compiler */ 00059 static void polarssl_zeroize( void *v, size_t n ) { 00060 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00061 } 00062 00063 /* 00064 * 32-bit integer manipulation macros (little endian) 00065 */ 00066 #ifndef GET_UINT32_LE 00067 #define GET_UINT32_LE(n,b,i) \ 00068 { \ 00069 (n) = ( (uint32_t) (b)[(i) ] ) \ 00070 | ( (uint32_t) (b)[(i) + 1] << 8 ) \ 00071 | ( (uint32_t) (b)[(i) + 2] << 16 ) \ 00072 | ( (uint32_t) (b)[(i) + 3] << 24 ); \ 00073 } 00074 #endif 00075 00076 #ifndef PUT_UINT32_LE 00077 #define PUT_UINT32_LE(n,b,i) \ 00078 { \ 00079 (b)[(i) ] = (unsigned char) ( ( (n) ) & 0xFF ); \ 00080 (b)[(i) + 1] = (unsigned char) ( ( (n) >> 8 ) & 0xFF ); \ 00081 (b)[(i) + 2] = (unsigned char) ( ( (n) >> 16 ) & 0xFF ); \ 00082 (b)[(i) + 3] = (unsigned char) ( ( (n) >> 24 ) & 0xFF ); \ 00083 } 00084 #endif 00085 00086 #if defined(POLARSSL_PADLOCK_C) && \ 00087 ( defined(POLARSSL_HAVE_X86) || defined(PADLOCK_ALIGN16) ) 00088 static int aes_padlock_ace = -1; 00089 #endif 00090 00091 #if defined(POLARSSL_AES_ROM_TABLES) 00092 /* 00093 * Forward S-box 00094 */ 00095 static const unsigned char FSb[256] = 00096 { 00097 0x63, 0x7C, 0x77, 0x7B, 0xF2, 0x6B, 0x6F, 0xC5, 00098 0x30, 0x01, 0x67, 0x2B, 0xFE, 0xD7, 0xAB, 0x76, 00099 0xCA, 0x82, 0xC9, 0x7D, 0xFA, 0x59, 0x47, 0xF0, 00100 0xAD, 0xD4, 0xA2, 0xAF, 0x9C, 0xA4, 0x72, 0xC0, 00101 0xB7, 0xFD, 0x93, 0x26, 0x36, 0x3F, 0xF7, 0xCC, 00102 0x34, 0xA5, 0xE5, 0xF1, 0x71, 0xD8, 0x31, 0x15, 00103 0x04, 0xC7, 0x23, 0xC3, 0x18, 0x96, 0x05, 0x9A, 00104 0x07, 0x12, 0x80, 0xE2, 0xEB, 0x27, 0xB2, 0x75, 00105 0x09, 0x83, 0x2C, 0x1A, 0x1B, 0x6E, 0x5A, 0xA0, 00106 0x52, 0x3B, 0xD6, 0xB3, 0x29, 0xE3, 0x2F, 0x84, 00107 0x53, 0xD1, 0x00, 0xED, 0x20, 0xFC, 0xB1, 0x5B, 00108 0x6A, 0xCB, 0xBE, 0x39, 0x4A, 0x4C, 0x58, 0xCF, 00109 0xD0, 0xEF, 0xAA, 0xFB, 0x43, 0x4D, 0x33, 0x85, 00110 0x45, 0xF9, 0x02, 0x7F, 0x50, 0x3C, 0x9F, 0xA8, 00111 0x51, 0xA3, 0x40, 0x8F, 0x92, 0x9D, 0x38, 0xF5, 00112 0xBC, 0xB6, 0xDA, 0x21, 0x10, 0xFF, 0xF3, 0xD2, 00113 0xCD, 0x0C, 0x13, 0xEC, 0x5F, 0x97, 0x44, 0x17, 00114 0xC4, 0xA7, 0x7E, 0x3D, 0x64, 0x5D, 0x19, 0x73, 00115 0x60, 0x81, 0x4F, 0xDC, 0x22, 0x2A, 0x90, 0x88, 00116 0x46, 0xEE, 0xB8, 0x14, 0xDE, 0x5E, 0x0B, 0xDB, 00117 0xE0, 0x32, 0x3A, 0x0A, 0x49, 0x06, 0x24, 0x5C, 00118 0xC2, 0xD3, 0xAC, 0x62, 0x91, 0x95, 0xE4, 0x79, 00119 0xE7, 0xC8, 0x37, 0x6D, 0x8D, 0xD5, 0x4E, 0xA9, 00120 0x6C, 0x56, 0xF4, 0xEA, 0x65, 0x7A, 0xAE, 0x08, 00121 0xBA, 0x78, 0x25, 0x2E, 0x1C, 0xA6, 0xB4, 0xC6, 00122 0xE8, 0xDD, 0x74, 0x1F, 0x4B, 0xBD, 0x8B, 0x8A, 00123 0x70, 0x3E, 0xB5, 0x66, 0x48, 0x03, 0xF6, 0x0E, 00124 0x61, 0x35, 0x57, 0xB9, 0x86, 0xC1, 0x1D, 0x9E, 00125 0xE1, 0xF8, 0x98, 0x11, 0x69, 0xD9, 0x8E, 0x94, 00126 0x9B, 0x1E, 0x87, 0xE9, 0xCE, 0x55, 0x28, 0xDF, 00127 0x8C, 0xA1, 0x89, 0x0D, 0xBF, 0xE6, 0x42, 0x68, 00128 0x41, 0x99, 0x2D, 0x0F, 0xB0, 0x54, 0xBB, 0x16 00129 }; 00130 00131 /* 00132 * Forward tables 00133 */ 00134 #define FT \ 00135 \ 00136 V(A5,63,63,C6), V(84,7C,7C,F8), V(99,77,77,EE), V(8D,7B,7B,F6), \ 00137 V(0D,F2,F2,FF), V(BD,6B,6B,D6), V(B1,6F,6F,DE), V(54,C5,C5,91), \ 00138 V(50,30,30,60), V(03,01,01,02), V(A9,67,67,CE), V(7D,2B,2B,56), \ 00139 V(19,FE,FE,E7), V(62,D7,D7,B5), V(E6,AB,AB,4D), V(9A,76,76,EC), \ 00140 V(45,CA,CA,8F), V(9D,82,82,1F), V(40,C9,C9,89), V(87,7D,7D,FA), \ 00141 V(15,FA,FA,EF), V(EB,59,59,B2), V(C9,47,47,8E), V(0B,F0,F0,FB), \ 00142 V(EC,AD,AD,41), V(67,D4,D4,B3), V(FD,A2,A2,5F), V(EA,AF,AF,45), \ 00143 V(BF,9C,9C,23), V(F7,A4,A4,53), V(96,72,72,E4), V(5B,C0,C0,9B), \ 00144 V(C2,B7,B7,75), V(1C,FD,FD,E1), V(AE,93,93,3D), V(6A,26,26,4C), \ 00145 V(5A,36,36,6C), V(41,3F,3F,7E), V(02,F7,F7,F5), V(4F,CC,CC,83), \ 00146 V(5C,34,34,68), V(F4,A5,A5,51), V(34,E5,E5,D1), V(08,F1,F1,F9), \ 00147 V(93,71,71,E2), V(73,D8,D8,AB), V(53,31,31,62), V(3F,15,15,2A), \ 00148 V(0C,04,04,08), V(52,C7,C7,95), V(65,23,23,46), V(5E,C3,C3,9D), \ 00149 V(28,18,18,30), V(A1,96,96,37), V(0F,05,05,0A), V(B5,9A,9A,2F), \ 00150 V(09,07,07,0E), V(36,12,12,24), V(9B,80,80,1B), V(3D,E2,E2,DF), \ 00151 V(26,EB,EB,CD), V(69,27,27,4E), V(CD,B2,B2,7F), V(9F,75,75,EA), \ 00152 V(1B,09,09,12), V(9E,83,83,1D), V(74,2C,2C,58), V(2E,1A,1A,34), \ 00153 V(2D,1B,1B,36), V(B2,6E,6E,DC), V(EE,5A,5A,B4), V(FB,A0,A0,5B), \ 00154 V(F6,52,52,A4), V(4D,3B,3B,76), V(61,D6,D6,B7), V(CE,B3,B3,7D), \ 00155 V(7B,29,29,52), V(3E,E3,E3,DD), V(71,2F,2F,5E), V(97,84,84,13), \ 00156 V(F5,53,53,A6), V(68,D1,D1,B9), V(00,00,00,00), V(2C,ED,ED,C1), \ 00157 V(60,20,20,40), V(1F,FC,FC,E3), V(C8,B1,B1,79), V(ED,5B,5B,B6), \ 00158 V(BE,6A,6A,D4), V(46,CB,CB,8D), V(D9,BE,BE,67), V(4B,39,39,72), \ 00159 V(DE,4A,4A,94), V(D4,4C,4C,98), V(E8,58,58,B0), V(4A,CF,CF,85), \ 00160 V(6B,D0,D0,BB), V(2A,EF,EF,C5), V(E5,AA,AA,4F), V(16,FB,FB,ED), \ 00161 V(C5,43,43,86), V(D7,4D,4D,9A), V(55,33,33,66), V(94,85,85,11), \ 00162 V(CF,45,45,8A), V(10,F9,F9,E9), V(06,02,02,04), V(81,7F,7F,FE), \ 00163 V(F0,50,50,A0), V(44,3C,3C,78), V(BA,9F,9F,25), V(E3,A8,A8,4B), \ 00164 V(F3,51,51,A2), V(FE,A3,A3,5D), V(C0,40,40,80), V(8A,8F,8F,05), \ 00165 V(AD,92,92,3F), V(BC,9D,9D,21), V(48,38,38,70), V(04,F5,F5,F1), \ 00166 V(DF,BC,BC,63), V(C1,B6,B6,77), V(75,DA,DA,AF), V(63,21,21,42), \ 00167 V(30,10,10,20), V(1A,FF,FF,E5), V(0E,F3,F3,FD), V(6D,D2,D2,BF), \ 00168 V(4C,CD,CD,81), V(14,0C,0C,18), V(35,13,13,26), V(2F,EC,EC,C3), \ 00169 V(E1,5F,5F,BE), V(A2,97,97,35), V(CC,44,44,88), V(39,17,17,2E), \ 00170 V(57,C4,C4,93), V(F2,A7,A7,55), V(82,7E,7E,FC), V(47,3D,3D,7A), \ 00171 V(AC,64,64,C8), V(E7,5D,5D,BA), V(2B,19,19,32), V(95,73,73,E6), \ 00172 V(A0,60,60,C0), V(98,81,81,19), V(D1,4F,4F,9E), V(7F,DC,DC,A3), \ 00173 V(66,22,22,44), V(7E,2A,2A,54), V(AB,90,90,3B), V(83,88,88,0B), \ 00174 V(CA,46,46,8C), V(29,EE,EE,C7), V(D3,B8,B8,6B), V(3C,14,14,28), \ 00175 V(79,DE,DE,A7), V(E2,5E,5E,BC), V(1D,0B,0B,16), V(76,DB,DB,AD), \ 00176 V(3B,E0,E0,DB), V(56,32,32,64), V(4E,3A,3A,74), V(1E,0A,0A,14), \ 00177 V(DB,49,49,92), V(0A,06,06,0C), V(6C,24,24,48), V(E4,5C,5C,B8), \ 00178 V(5D,C2,C2,9F), V(6E,D3,D3,BD), V(EF,AC,AC,43), V(A6,62,62,C4), \ 00179 V(A8,91,91,39), V(A4,95,95,31), V(37,E4,E4,D3), V(8B,79,79,F2), \ 00180 V(32,E7,E7,D5), V(43,C8,C8,8B), V(59,37,37,6E), V(B7,6D,6D,DA), \ 00181 V(8C,8D,8D,01), V(64,D5,D5,B1), V(D2,4E,4E,9C), V(E0,A9,A9,49), \ 00182 V(B4,6C,6C,D8), V(FA,56,56,AC), V(07,F4,F4,F3), V(25,EA,EA,CF), \ 00183 V(AF,65,65,CA), V(8E,7A,7A,F4), V(E9,AE,AE,47), V(18,08,08,10), \ 00184 V(D5,BA,BA,6F), V(88,78,78,F0), V(6F,25,25,4A), V(72,2E,2E,5C), \ 00185 V(24,1C,1C,38), V(F1,A6,A6,57), V(C7,B4,B4,73), V(51,C6,C6,97), \ 00186 V(23,E8,E8,CB), V(7C,DD,DD,A1), V(9C,74,74,E8), V(21,1F,1F,3E), \ 00187 V(DD,4B,4B,96), V(DC,BD,BD,61), V(86,8B,8B,0D), V(85,8A,8A,0F), \ 00188 V(90,70,70,E0), V(42,3E,3E,7C), V(C4,B5,B5,71), V(AA,66,66,CC), \ 00189 V(D8,48,48,90), V(05,03,03,06), V(01,F6,F6,F7), V(12,0E,0E,1C), \ 00190 V(A3,61,61,C2), V(5F,35,35,6A), V(F9,57,57,AE), V(D0,B9,B9,69), \ 00191 V(91,86,86,17), V(58,C1,C1,99), V(27,1D,1D,3A), V(B9,9E,9E,27), \ 00192 V(38,E1,E1,D9), V(13,F8,F8,EB), V(B3,98,98,2B), V(33,11,11,22), \ 00193 V(BB,69,69,D2), V(70,D9,D9,A9), V(89,8E,8E,07), V(A7,94,94,33), \ 00194 V(B6,9B,9B,2D), V(22,1E,1E,3C), V(92,87,87,15), V(20,E9,E9,C9), \ 00195 V(49,CE,CE,87), V(FF,55,55,AA), V(78,28,28,50), V(7A,DF,DF,A5), \ 00196 V(8F,8C,8C,03), V(F8,A1,A1,59), V(80,89,89,09), V(17,0D,0D,1A), \ 00197 V(DA,BF,BF,65), V(31,E6,E6,D7), V(C6,42,42,84), V(B8,68,68,D0), \ 00198 V(C3,41,41,82), V(B0,99,99,29), V(77,2D,2D,5A), V(11,0F,0F,1E), \ 00199 V(CB,B0,B0,7B), V(FC,54,54,A8), V(D6,BB,BB,6D), V(3A,16,16,2C) 00200 00201 #define V(a,b,c,d) 0x##a##b##c##d 00202 static const uint32_t FT0[256] = { FT }; 00203 #undef V 00204 00205 #define V(a,b,c,d) 0x##b##c##d##a 00206 static const uint32_t FT1[256] = { FT }; 00207 #undef V 00208 00209 #define V(a,b,c,d) 0x##c##d##a##b 00210 static const uint32_t FT2[256] = { FT }; 00211 #undef V 00212 00213 #define V(a,b,c,d) 0x##d##a##b##c 00214 static const uint32_t FT3[256] = { FT }; 00215 #undef V 00216 00217 #undef FT 00218 00219 /* 00220 * Reverse S-box 00221 */ 00222 static const unsigned char RSb[256] = 00223 { 00224 0x52, 0x09, 0x6A, 0xD5, 0x30, 0x36, 0xA5, 0x38, 00225 0xBF, 0x40, 0xA3, 0x9E, 0x81, 0xF3, 0xD7, 0xFB, 00226 0x7C, 0xE3, 0x39, 0x82, 0x9B, 0x2F, 0xFF, 0x87, 00227 0x34, 0x8E, 0x43, 0x44, 0xC4, 0xDE, 0xE9, 0xCB, 00228 0x54, 0x7B, 0x94, 0x32, 0xA6, 0xC2, 0x23, 0x3D, 00229 0xEE, 0x4C, 0x95, 0x0B, 0x42, 0xFA, 0xC3, 0x4E, 00230 0x08, 0x2E, 0xA1, 0x66, 0x28, 0xD9, 0x24, 0xB2, 00231 0x76, 0x5B, 0xA2, 0x49, 0x6D, 0x8B, 0xD1, 0x25, 00232 0x72, 0xF8, 0xF6, 0x64, 0x86, 0x68, 0x98, 0x16, 00233 0xD4, 0xA4, 0x5C, 0xCC, 0x5D, 0x65, 0xB6, 0x92, 00234 0x6C, 0x70, 0x48, 0x50, 0xFD, 0xED, 0xB9, 0xDA, 00235 0x5E, 0x15, 0x46, 0x57, 0xA7, 0x8D, 0x9D, 0x84, 00236 0x90, 0xD8, 0xAB, 0x00, 0x8C, 0xBC, 0xD3, 0x0A, 00237 0xF7, 0xE4, 0x58, 0x05, 0xB8, 0xB3, 0x45, 0x06, 00238 0xD0, 0x2C, 0x1E, 0x8F, 0xCA, 0x3F, 0x0F, 0x02, 00239 0xC1, 0xAF, 0xBD, 0x03, 0x01, 0x13, 0x8A, 0x6B, 00240 0x3A, 0x91, 0x11, 0x41, 0x4F, 0x67, 0xDC, 0xEA, 00241 0x97, 0xF2, 0xCF, 0xCE, 0xF0, 0xB4, 0xE6, 0x73, 00242 0x96, 0xAC, 0x74, 0x22, 0xE7, 0xAD, 0x35, 0x85, 00243 0xE2, 0xF9, 0x37, 0xE8, 0x1C, 0x75, 0xDF, 0x6E, 00244 0x47, 0xF1, 0x1A, 0x71, 0x1D, 0x29, 0xC5, 0x89, 00245 0x6F, 0xB7, 0x62, 0x0E, 0xAA, 0x18, 0xBE, 0x1B, 00246 0xFC, 0x56, 0x3E, 0x4B, 0xC6, 0xD2, 0x79, 0x20, 00247 0x9A, 0xDB, 0xC0, 0xFE, 0x78, 0xCD, 0x5A, 0xF4, 00248 0x1F, 0xDD, 0xA8, 0x33, 0x88, 0x07, 0xC7, 0x31, 00249 0xB1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xEC, 0x5F, 00250 0x60, 0x51, 0x7F, 0xA9, 0x19, 0xB5, 0x4A, 0x0D, 00251 0x2D, 0xE5, 0x7A, 0x9F, 0x93, 0xC9, 0x9C, 0xEF, 00252 0xA0, 0xE0, 0x3B, 0x4D, 0xAE, 0x2A, 0xF5, 0xB0, 00253 0xC8, 0xEB, 0xBB, 0x3C, 0x83, 0x53, 0x99, 0x61, 00254 0x17, 0x2B, 0x04, 0x7E, 0xBA, 0x77, 0xD6, 0x26, 00255 0xE1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0C, 0x7D 00256 }; 00257 00258 /* 00259 * Reverse tables 00260 */ 00261 #define RT \ 00262 \ 00263 V(50,A7,F4,51), V(53,65,41,7E), V(C3,A4,17,1A), V(96,5E,27,3A), \ 00264 V(CB,6B,AB,3B), V(F1,45,9D,1F), V(AB,58,FA,AC), V(93,03,E3,4B), \ 00265 V(55,FA,30,20), V(F6,6D,76,AD), V(91,76,CC,88), V(25,4C,02,F5), \ 00266 V(FC,D7,E5,4F), V(D7,CB,2A,C5), V(80,44,35,26), V(8F,A3,62,B5), \ 00267 V(49,5A,B1,DE), V(67,1B,BA,25), V(98,0E,EA,45), V(E1,C0,FE,5D), \ 00268 V(02,75,2F,C3), V(12,F0,4C,81), V(A3,97,46,8D), V(C6,F9,D3,6B), \ 00269 V(E7,5F,8F,03), V(95,9C,92,15), V(EB,7A,6D,BF), V(DA,59,52,95), \ 00270 V(2D,83,BE,D4), V(D3,21,74,58), V(29,69,E0,49), V(44,C8,C9,8E), \ 00271 V(6A,89,C2,75), V(78,79,8E,F4), V(6B,3E,58,99), V(DD,71,B9,27), \ 00272 V(B6,4F,E1,BE), V(17,AD,88,F0), V(66,AC,20,C9), V(B4,3A,CE,7D), \ 00273 V(18,4A,DF,63), V(82,31,1A,E5), V(60,33,51,97), V(45,7F,53,62), \ 00274 V(E0,77,64,B1), V(84,AE,6B,BB), V(1C,A0,81,FE), V(94,2B,08,F9), \ 00275 V(58,68,48,70), V(19,FD,45,8F), V(87,6C,DE,94), V(B7,F8,7B,52), \ 00276 V(23,D3,73,AB), V(E2,02,4B,72), V(57,8F,1F,E3), V(2A,AB,55,66), \ 00277 V(07,28,EB,B2), V(03,C2,B5,2F), V(9A,7B,C5,86), V(A5,08,37,D3), \ 00278 V(F2,87,28,30), V(B2,A5,BF,23), V(BA,6A,03,02), V(5C,82,16,ED), \ 00279 V(2B,1C,CF,8A), V(92,B4,79,A7), V(F0,F2,07,F3), V(A1,E2,69,4E), \ 00280 V(CD,F4,DA,65), V(D5,BE,05,06), V(1F,62,34,D1), V(8A,FE,A6,C4), \ 00281 V(9D,53,2E,34), V(A0,55,F3,A2), V(32,E1,8A,05), V(75,EB,F6,A4), \ 00282 V(39,EC,83,0B), V(AA,EF,60,40), V(06,9F,71,5E), V(51,10,6E,BD), \ 00283 V(F9,8A,21,3E), V(3D,06,DD,96), V(AE,05,3E,DD), V(46,BD,E6,4D), \ 00284 V(B5,8D,54,91), V(05,5D,C4,71), V(6F,D4,06,04), V(FF,15,50,60), \ 00285 V(24,FB,98,19), V(97,E9,BD,D6), V(CC,43,40,89), V(77,9E,D9,67), \ 00286 V(BD,42,E8,B0), V(88,8B,89,07), V(38,5B,19,E7), V(DB,EE,C8,79), \ 00287 V(47,0A,7C,A1), V(E9,0F,42,7C), V(C9,1E,84,F8), V(00,00,00,00), \ 00288 V(83,86,80,09), V(48,ED,2B,32), V(AC,70,11,1E), V(4E,72,5A,6C), \ 00289 V(FB,FF,0E,FD), V(56,38,85,0F), V(1E,D5,AE,3D), V(27,39,2D,36), \ 00290 V(64,D9,0F,0A), V(21,A6,5C,68), V(D1,54,5B,9B), V(3A,2E,36,24), \ 00291 V(B1,67,0A,0C), V(0F,E7,57,93), V(D2,96,EE,B4), V(9E,91,9B,1B), \ 00292 V(4F,C5,C0,80), V(A2,20,DC,61), V(69,4B,77,5A), V(16,1A,12,1C), \ 00293 V(0A,BA,93,E2), V(E5,2A,A0,C0), V(43,E0,22,3C), V(1D,17,1B,12), \ 00294 V(0B,0D,09,0E), V(AD,C7,8B,F2), V(B9,A8,B6,2D), V(C8,A9,1E,14), \ 00295 V(85,19,F1,57), V(4C,07,75,AF), V(BB,DD,99,EE), V(FD,60,7F,A3), \ 00296 V(9F,26,01,F7), V(BC,F5,72,5C), V(C5,3B,66,44), V(34,7E,FB,5B), \ 00297 V(76,29,43,8B), V(DC,C6,23,CB), V(68,FC,ED,B6), V(63,F1,E4,B8), \ 00298 V(CA,DC,31,D7), V(10,85,63,42), V(40,22,97,13), V(20,11,C6,84), \ 00299 V(7D,24,4A,85), V(F8,3D,BB,D2), V(11,32,F9,AE), V(6D,A1,29,C7), \ 00300 V(4B,2F,9E,1D), V(F3,30,B2,DC), V(EC,52,86,0D), V(D0,E3,C1,77), \ 00301 V(6C,16,B3,2B), V(99,B9,70,A9), V(FA,48,94,11), V(22,64,E9,47), \ 00302 V(C4,8C,FC,A8), V(1A,3F,F0,A0), V(D8,2C,7D,56), V(EF,90,33,22), \ 00303 V(C7,4E,49,87), V(C1,D1,38,D9), V(FE,A2,CA,8C), V(36,0B,D4,98), \ 00304 V(CF,81,F5,A6), V(28,DE,7A,A5), V(26,8E,B7,DA), V(A4,BF,AD,3F), \ 00305 V(E4,9D,3A,2C), V(0D,92,78,50), V(9B,CC,5F,6A), V(62,46,7E,54), \ 00306 V(C2,13,8D,F6), V(E8,B8,D8,90), V(5E,F7,39,2E), V(F5,AF,C3,82), \ 00307 V(BE,80,5D,9F), V(7C,93,D0,69), V(A9,2D,D5,6F), V(B3,12,25,CF), \ 00308 V(3B,99,AC,C8), V(A7,7D,18,10), V(6E,63,9C,E8), V(7B,BB,3B,DB), \ 00309 V(09,78,26,CD), V(F4,18,59,6E), V(01,B7,9A,EC), V(A8,9A,4F,83), \ 00310 V(65,6E,95,E6), V(7E,E6,FF,AA), V(08,CF,BC,21), V(E6,E8,15,EF), \ 00311 V(D9,9B,E7,BA), V(CE,36,6F,4A), V(D4,09,9F,EA), V(D6,7C,B0,29), \ 00312 V(AF,B2,A4,31), V(31,23,3F,2A), V(30,94,A5,C6), V(C0,66,A2,35), \ 00313 V(37,BC,4E,74), V(A6,CA,82,FC), V(B0,D0,90,E0), V(15,D8,A7,33), \ 00314 V(4A,98,04,F1), V(F7,DA,EC,41), V(0E,50,CD,7F), V(2F,F6,91,17), \ 00315 V(8D,D6,4D,76), V(4D,B0,EF,43), V(54,4D,AA,CC), V(DF,04,96,E4), \ 00316 V(E3,B5,D1,9E), V(1B,88,6A,4C), V(B8,1F,2C,C1), V(7F,51,65,46), \ 00317 V(04,EA,5E,9D), V(5D,35,8C,01), V(73,74,87,FA), V(2E,41,0B,FB), \ 00318 V(5A,1D,67,B3), V(52,D2,DB,92), V(33,56,10,E9), V(13,47,D6,6D), \ 00319 V(8C,61,D7,9A), V(7A,0C,A1,37), V(8E,14,F8,59), V(89,3C,13,EB), \ 00320 V(EE,27,A9,CE), V(35,C9,61,B7), V(ED,E5,1C,E1), V(3C,B1,47,7A), \ 00321 V(59,DF,D2,9C), V(3F,73,F2,55), V(79,CE,14,18), V(BF,37,C7,73), \ 00322 V(EA,CD,F7,53), V(5B,AA,FD,5F), V(14,6F,3D,DF), V(86,DB,44,78), \ 00323 V(81,F3,AF,CA), V(3E,C4,68,B9), V(2C,34,24,38), V(5F,40,A3,C2), \ 00324 V(72,C3,1D,16), V(0C,25,E2,BC), V(8B,49,3C,28), V(41,95,0D,FF), \ 00325 V(71,01,A8,39), V(DE,B3,0C,08), V(9C,E4,B4,D8), V(90,C1,56,64), \ 00326 V(61,84,CB,7B), V(70,B6,32,D5), V(74,5C,6C,48), V(42,57,B8,D0) 00327 00328 #define V(a,b,c,d) 0x##a##b##c##d 00329 static const uint32_t RT0[256] = { RT }; 00330 #undef V 00331 00332 #define V(a,b,c,d) 0x##b##c##d##a 00333 static const uint32_t RT1[256] = { RT }; 00334 #undef V 00335 00336 #define V(a,b,c,d) 0x##c##d##a##b 00337 static const uint32_t RT2[256] = { RT }; 00338 #undef V 00339 00340 #define V(a,b,c,d) 0x##d##a##b##c 00341 static const uint32_t RT3[256] = { RT }; 00342 #undef V 00343 00344 #undef RT 00345 00346 /* 00347 * Round constants 00348 */ 00349 static const uint32_t RCON[10] = 00350 { 00351 0x00000001, 0x00000002, 0x00000004, 0x00000008, 00352 0x00000010, 0x00000020, 0x00000040, 0x00000080, 00353 0x0000001B, 0x00000036 00354 }; 00355 00356 #else /* POLARSSL_AES_ROM_TABLES */ 00357 00358 /* 00359 * Forward S-box & tables 00360 */ 00361 static unsigned char FSb[256]; 00362 static uint32_t FT0[256]; 00363 static uint32_t FT1[256]; 00364 static uint32_t FT2[256]; 00365 static uint32_t FT3[256]; 00366 00367 /* 00368 * Reverse S-box & tables 00369 */ 00370 static unsigned char RSb[256]; 00371 static uint32_t RT0[256]; 00372 static uint32_t RT1[256]; 00373 static uint32_t RT2[256]; 00374 static uint32_t RT3[256]; 00375 00376 /* 00377 * Round constants 00378 */ 00379 static uint32_t RCON[10]; 00380 00381 /* 00382 * Tables generation code 00383 */ 00384 #define ROTL8(x) ( ( x << 8 ) & 0xFFFFFFFF ) | ( x >> 24 ) 00385 #define XTIME(x) ( ( x << 1 ) ^ ( ( x & 0x80 ) ? 0x1B : 0x00 ) ) 00386 #define MUL(x,y) ( ( x && y ) ? pow[(log[x]+log[y]) % 255] : 0 ) 00387 00388 static int aes_init_done = 0; 00389 00390 static void aes_gen_tables( void ) 00391 { 00392 int i, x, y, z; 00393 int pow[256]; 00394 int log[256]; 00395 00396 /* 00397 * compute pow and log tables over GF(2^8) 00398 */ 00399 for( i = 0, x = 1; i < 256; i++ ) 00400 { 00401 pow[i] = x; 00402 log[x] = i; 00403 x = ( x ^ XTIME( x ) ) & 0xFF; 00404 } 00405 00406 /* 00407 * calculate the round constants 00408 */ 00409 for( i = 0, x = 1; i < 10; i++ ) 00410 { 00411 RCON[i] = (uint32_t) x; 00412 x = XTIME( x ) & 0xFF; 00413 } 00414 00415 /* 00416 * generate the forward and reverse S-boxes 00417 */ 00418 FSb[0x00] = 0x63; 00419 RSb[0x63] = 0x00; 00420 00421 for( i = 1; i < 256; i++ ) 00422 { 00423 x = pow[255 - log[i]]; 00424 00425 y = x; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00426 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00427 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00428 x ^= y; y = ( ( y << 1 ) | ( y >> 7 ) ) & 0xFF; 00429 x ^= y ^ 0x63; 00430 00431 FSb[i] = (unsigned char) x; 00432 RSb[x] = (unsigned char) i; 00433 } 00434 00435 /* 00436 * generate the forward and reverse tables 00437 */ 00438 for( i = 0; i < 256; i++ ) 00439 { 00440 x = FSb[i]; 00441 y = XTIME( x ) & 0xFF; 00442 z = ( y ^ x ) & 0xFF; 00443 00444 FT0[i] = ( (uint32_t) y ) ^ 00445 ( (uint32_t) x << 8 ) ^ 00446 ( (uint32_t) x << 16 ) ^ 00447 ( (uint32_t) z << 24 ); 00448 00449 FT1[i] = ROTL8( FT0[i] ); 00450 FT2[i] = ROTL8( FT1[i] ); 00451 FT3[i] = ROTL8( FT2[i] ); 00452 00453 x = RSb[i]; 00454 00455 RT0[i] = ( (uint32_t) MUL( 0x0E, x ) ) ^ 00456 ( (uint32_t) MUL( 0x09, x ) << 8 ) ^ 00457 ( (uint32_t) MUL( 0x0D, x ) << 16 ) ^ 00458 ( (uint32_t) MUL( 0x0B, x ) << 24 ); 00459 00460 RT1[i] = ROTL8( RT0[i] ); 00461 RT2[i] = ROTL8( RT1[i] ); 00462 RT3[i] = ROTL8( RT2[i] ); 00463 } 00464 } 00465 00466 #endif /* POLARSSL_AES_ROM_TABLES */ 00467 00468 void aes_init( aes_context *ctx ) 00469 { 00470 memset( ctx, 0, sizeof( aes_context ) ); 00471 } 00472 00473 void aes_free( aes_context *ctx ) 00474 { 00475 if( ctx == NULL ) 00476 return; 00477 00478 polarssl_zeroize( ctx, sizeof( aes_context ) ); 00479 } 00480 00481 /* 00482 * AES key schedule (encryption) 00483 */ 00484 int aes_setkey_enc( aes_context *ctx, const unsigned char *key, 00485 unsigned int keysize ) 00486 { 00487 unsigned int i; 00488 uint32_t *RK; 00489 00490 #if !defined(POLARSSL_AES_ROM_TABLES) 00491 if( aes_init_done == 0 ) 00492 { 00493 aes_gen_tables(); 00494 aes_init_done = 1; 00495 00496 } 00497 #endif 00498 00499 switch( keysize ) 00500 { 00501 case 128: ctx->nr = 10; break; 00502 case 192: ctx->nr = 12; break; 00503 case 256: ctx->nr = 14; break; 00504 default : return( POLARSSL_ERR_AES_INVALID_KEY_LENGTH ); 00505 } 00506 00507 #if defined(POLARSSL_PADLOCK_C) && defined(PADLOCK_ALIGN16) 00508 if( aes_padlock_ace == -1 ) 00509 aes_padlock_ace = padlock_supports( PADLOCK_ACE ); 00510 00511 if( aes_padlock_ace ) 00512 ctx->rk = RK = PADLOCK_ALIGN16( ctx->buf ); 00513 else 00514 #endif 00515 ctx->rk = RK = ctx->buf ; 00516 00517 #if defined(POLARSSL_AESNI_C) && defined(POLARSSL_HAVE_X86_64) 00518 if( aesni_supports( POLARSSL_AESNI_AES ) ) 00519 return( aesni_setkey_enc( (unsigned char *) ctx->rk , key, keysize ) ); 00520 #endif 00521 00522 for( i = 0; i < ( keysize >> 5 ); i++ ) 00523 { 00524 GET_UINT32_LE( RK[i], key, i << 2 ); 00525 } 00526 00527 switch( ctx->nr ) 00528 { 00529 case 10: 00530 00531 for( i = 0; i < 10; i++, RK += 4 ) 00532 { 00533 RK[4] = RK[0] ^ RCON[i] ^ 00534 ( (uint32_t) FSb[ ( RK[3] >> 8 ) & 0xFF ] ) ^ 00535 ( (uint32_t) FSb[ ( RK[3] >> 16 ) & 0xFF ] << 8 ) ^ 00536 ( (uint32_t) FSb[ ( RK[3] >> 24 ) & 0xFF ] << 16 ) ^ 00537 ( (uint32_t) FSb[ ( RK[3] ) & 0xFF ] << 24 ); 00538 00539 RK[5] = RK[1] ^ RK[4]; 00540 RK[6] = RK[2] ^ RK[5]; 00541 RK[7] = RK[3] ^ RK[6]; 00542 } 00543 break; 00544 00545 case 12: 00546 00547 for( i = 0; i < 8; i++, RK += 6 ) 00548 { 00549 RK[6] = RK[0] ^ RCON[i] ^ 00550 ( (uint32_t) FSb[ ( RK[5] >> 8 ) & 0xFF ] ) ^ 00551 ( (uint32_t) FSb[ ( RK[5] >> 16 ) & 0xFF ] << 8 ) ^ 00552 ( (uint32_t) FSb[ ( RK[5] >> 24 ) & 0xFF ] << 16 ) ^ 00553 ( (uint32_t) FSb[ ( RK[5] ) & 0xFF ] << 24 ); 00554 00555 RK[7] = RK[1] ^ RK[6]; 00556 RK[8] = RK[2] ^ RK[7]; 00557 RK[9] = RK[3] ^ RK[8]; 00558 RK[10] = RK[4] ^ RK[9]; 00559 RK[11] = RK[5] ^ RK[10]; 00560 } 00561 break; 00562 00563 case 14: 00564 00565 for( i = 0; i < 7; i++, RK += 8 ) 00566 { 00567 RK[8] = RK[0] ^ RCON[i] ^ 00568 ( (uint32_t) FSb[ ( RK[7] >> 8 ) & 0xFF ] ) ^ 00569 ( (uint32_t) FSb[ ( RK[7] >> 16 ) & 0xFF ] << 8 ) ^ 00570 ( (uint32_t) FSb[ ( RK[7] >> 24 ) & 0xFF ] << 16 ) ^ 00571 ( (uint32_t) FSb[ ( RK[7] ) & 0xFF ] << 24 ); 00572 00573 RK[9] = RK[1] ^ RK[8]; 00574 RK[10] = RK[2] ^ RK[9]; 00575 RK[11] = RK[3] ^ RK[10]; 00576 00577 RK[12] = RK[4] ^ 00578 ( (uint32_t) FSb[ ( RK[11] ) & 0xFF ] ) ^ 00579 ( (uint32_t) FSb[ ( RK[11] >> 8 ) & 0xFF ] << 8 ) ^ 00580 ( (uint32_t) FSb[ ( RK[11] >> 16 ) & 0xFF ] << 16 ) ^ 00581 ( (uint32_t) FSb[ ( RK[11] >> 24 ) & 0xFF ] << 24 ); 00582 00583 RK[13] = RK[5] ^ RK[12]; 00584 RK[14] = RK[6] ^ RK[13]; 00585 RK[15] = RK[7] ^ RK[14]; 00586 } 00587 break; 00588 } 00589 00590 return( 0 ); 00591 } 00592 00593 /* 00594 * AES key schedule (decryption) 00595 */ 00596 int aes_setkey_dec( aes_context *ctx, const unsigned char *key, 00597 unsigned int keysize ) 00598 { 00599 int i, j, ret; 00600 aes_context cty; 00601 uint32_t *RK; 00602 uint32_t *SK; 00603 00604 aes_init( &cty ); 00605 00606 #if defined(POLARSSL_PADLOCK_C) && defined(PADLOCK_ALIGN16) 00607 if( aes_padlock_ace == -1 ) 00608 aes_padlock_ace = padlock_supports( PADLOCK_ACE ); 00609 00610 if( aes_padlock_ace ) 00611 ctx->rk = RK = PADLOCK_ALIGN16( ctx->buf ); 00612 else 00613 #endif 00614 ctx->rk = RK = ctx->buf ; 00615 00616 /* Also checks keysize */ 00617 if( ( ret = aes_setkey_enc( &cty, key, keysize ) ) != 0 ) 00618 goto exit; 00619 00620 ctx->nr = cty.nr ; 00621 00622 #if defined(POLARSSL_AESNI_C) && defined(POLARSSL_HAVE_X86_64) 00623 if( aesni_supports( POLARSSL_AESNI_AES ) ) 00624 { 00625 aesni_inverse_key( (unsigned char *) ctx->rk , 00626 (const unsigned char *) cty.rk , ctx->nr ); 00627 goto exit; 00628 } 00629 #endif 00630 00631 SK = cty.rk + cty.nr * 4; 00632 00633 *RK++ = *SK++; 00634 *RK++ = *SK++; 00635 *RK++ = *SK++; 00636 *RK++ = *SK++; 00637 00638 for( i = ctx->nr - 1, SK -= 8; i > 0; i--, SK -= 8 ) 00639 { 00640 for( j = 0; j < 4; j++, SK++ ) 00641 { 00642 *RK++ = RT0[ FSb[ ( *SK ) & 0xFF ] ] ^ 00643 RT1[ FSb[ ( *SK >> 8 ) & 0xFF ] ] ^ 00644 RT2[ FSb[ ( *SK >> 16 ) & 0xFF ] ] ^ 00645 RT3[ FSb[ ( *SK >> 24 ) & 0xFF ] ]; 00646 } 00647 } 00648 00649 *RK++ = *SK++; 00650 *RK++ = *SK++; 00651 *RK++ = *SK++; 00652 *RK++ = *SK++; 00653 00654 exit: 00655 aes_free( &cty ); 00656 00657 return( ret ); 00658 } 00659 00660 #define AES_FROUND(X0,X1,X2,X3,Y0,Y1,Y2,Y3) \ 00661 { \ 00662 X0 = *RK++ ^ FT0[ ( Y0 ) & 0xFF ] ^ \ 00663 FT1[ ( Y1 >> 8 ) & 0xFF ] ^ \ 00664 FT2[ ( Y2 >> 16 ) & 0xFF ] ^ \ 00665 FT3[ ( Y3 >> 24 ) & 0xFF ]; \ 00666 \ 00667 X1 = *RK++ ^ FT0[ ( Y1 ) & 0xFF ] ^ \ 00668 FT1[ ( Y2 >> 8 ) & 0xFF ] ^ \ 00669 FT2[ ( Y3 >> 16 ) & 0xFF ] ^ \ 00670 FT3[ ( Y0 >> 24 ) & 0xFF ]; \ 00671 \ 00672 X2 = *RK++ ^ FT0[ ( Y2 ) & 0xFF ] ^ \ 00673 FT1[ ( Y3 >> 8 ) & 0xFF ] ^ \ 00674 FT2[ ( Y0 >> 16 ) & 0xFF ] ^ \ 00675 FT3[ ( Y1 >> 24 ) & 0xFF ]; \ 00676 \ 00677 X3 = *RK++ ^ FT0[ ( Y3 ) & 0xFF ] ^ \ 00678 FT1[ ( Y0 >> 8 ) & 0xFF ] ^ \ 00679 FT2[ ( Y1 >> 16 ) & 0xFF ] ^ \ 00680 FT3[ ( Y2 >> 24 ) & 0xFF ]; \ 00681 } 00682 00683 #define AES_RROUND(X0,X1,X2,X3,Y0,Y1,Y2,Y3) \ 00684 { \ 00685 X0 = *RK++ ^ RT0[ ( Y0 ) & 0xFF ] ^ \ 00686 RT1[ ( Y3 >> 8 ) & 0xFF ] ^ \ 00687 RT2[ ( Y2 >> 16 ) & 0xFF ] ^ \ 00688 RT3[ ( Y1 >> 24 ) & 0xFF ]; \ 00689 \ 00690 X1 = *RK++ ^ RT0[ ( Y1 ) & 0xFF ] ^ \ 00691 RT1[ ( Y0 >> 8 ) & 0xFF ] ^ \ 00692 RT2[ ( Y3 >> 16 ) & 0xFF ] ^ \ 00693 RT3[ ( Y2 >> 24 ) & 0xFF ]; \ 00694 \ 00695 X2 = *RK++ ^ RT0[ ( Y2 ) & 0xFF ] ^ \ 00696 RT1[ ( Y1 >> 8 ) & 0xFF ] ^ \ 00697 RT2[ ( Y0 >> 16 ) & 0xFF ] ^ \ 00698 RT3[ ( Y3 >> 24 ) & 0xFF ]; \ 00699 \ 00700 X3 = *RK++ ^ RT0[ ( Y3 ) & 0xFF ] ^ \ 00701 RT1[ ( Y2 >> 8 ) & 0xFF ] ^ \ 00702 RT2[ ( Y1 >> 16 ) & 0xFF ] ^ \ 00703 RT3[ ( Y0 >> 24 ) & 0xFF ]; \ 00704 } 00705 00706 /* 00707 * AES-ECB block encryption/decryption 00708 */ 00709 int aes_crypt_ecb( aes_context *ctx, 00710 int mode, 00711 const unsigned char input[16], 00712 unsigned char output[16] ) 00713 { 00714 int i; 00715 uint32_t *RK, X0, X1, X2, X3, Y0, Y1, Y2, Y3; 00716 00717 #if defined(POLARSSL_AESNI_C) && defined(POLARSSL_HAVE_X86_64) 00718 if( aesni_supports( POLARSSL_AESNI_AES ) ) 00719 return( aesni_crypt_ecb( ctx, mode, input, output ) ); 00720 #endif 00721 00722 #if defined(POLARSSL_PADLOCK_C) && defined(POLARSSL_HAVE_X86) 00723 if( aes_padlock_ace ) 00724 { 00725 if( padlock_xcryptecb( ctx, mode, input, output ) == 0 ) 00726 return( 0 ); 00727 00728 // If padlock data misaligned, we just fall back to 00729 // unaccelerated mode 00730 // 00731 } 00732 #endif 00733 00734 RK = ctx->rk ; 00735 00736 GET_UINT32_LE( X0, input, 0 ); X0 ^= *RK++; 00737 GET_UINT32_LE( X1, input, 4 ); X1 ^= *RK++; 00738 GET_UINT32_LE( X2, input, 8 ); X2 ^= *RK++; 00739 GET_UINT32_LE( X3, input, 12 ); X3 ^= *RK++; 00740 00741 if( mode == AES_DECRYPT ) 00742 { 00743 for( i = ( ctx->nr >> 1 ) - 1; i > 0; i-- ) 00744 { 00745 AES_RROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00746 AES_RROUND( X0, X1, X2, X3, Y0, Y1, Y2, Y3 ); 00747 } 00748 00749 AES_RROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00750 00751 X0 = *RK++ ^ \ 00752 ( (uint32_t) RSb[ ( Y0 ) & 0xFF ] ) ^ 00753 ( (uint32_t) RSb[ ( Y3 >> 8 ) & 0xFF ] << 8 ) ^ 00754 ( (uint32_t) RSb[ ( Y2 >> 16 ) & 0xFF ] << 16 ) ^ 00755 ( (uint32_t) RSb[ ( Y1 >> 24 ) & 0xFF ] << 24 ); 00756 00757 X1 = *RK++ ^ \ 00758 ( (uint32_t) RSb[ ( Y1 ) & 0xFF ] ) ^ 00759 ( (uint32_t) RSb[ ( Y0 >> 8 ) & 0xFF ] << 8 ) ^ 00760 ( (uint32_t) RSb[ ( Y3 >> 16 ) & 0xFF ] << 16 ) ^ 00761 ( (uint32_t) RSb[ ( Y2 >> 24 ) & 0xFF ] << 24 ); 00762 00763 X2 = *RK++ ^ \ 00764 ( (uint32_t) RSb[ ( Y2 ) & 0xFF ] ) ^ 00765 ( (uint32_t) RSb[ ( Y1 >> 8 ) & 0xFF ] << 8 ) ^ 00766 ( (uint32_t) RSb[ ( Y0 >> 16 ) & 0xFF ] << 16 ) ^ 00767 ( (uint32_t) RSb[ ( Y3 >> 24 ) & 0xFF ] << 24 ); 00768 00769 X3 = *RK++ ^ \ 00770 ( (uint32_t) RSb[ ( Y3 ) & 0xFF ] ) ^ 00771 ( (uint32_t) RSb[ ( Y2 >> 8 ) & 0xFF ] << 8 ) ^ 00772 ( (uint32_t) RSb[ ( Y1 >> 16 ) & 0xFF ] << 16 ) ^ 00773 ( (uint32_t) RSb[ ( Y0 >> 24 ) & 0xFF ] << 24 ); 00774 } 00775 else /* AES_ENCRYPT */ 00776 { 00777 for( i = ( ctx->nr >> 1 ) - 1; i > 0; i-- ) 00778 { 00779 AES_FROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00780 AES_FROUND( X0, X1, X2, X3, Y0, Y1, Y2, Y3 ); 00781 } 00782 00783 AES_FROUND( Y0, Y1, Y2, Y3, X0, X1, X2, X3 ); 00784 00785 X0 = *RK++ ^ \ 00786 ( (uint32_t) FSb[ ( Y0 ) & 0xFF ] ) ^ 00787 ( (uint32_t) FSb[ ( Y1 >> 8 ) & 0xFF ] << 8 ) ^ 00788 ( (uint32_t) FSb[ ( Y2 >> 16 ) & 0xFF ] << 16 ) ^ 00789 ( (uint32_t) FSb[ ( Y3 >> 24 ) & 0xFF ] << 24 ); 00790 00791 X1 = *RK++ ^ \ 00792 ( (uint32_t) FSb[ ( Y1 ) & 0xFF ] ) ^ 00793 ( (uint32_t) FSb[ ( Y2 >> 8 ) & 0xFF ] << 8 ) ^ 00794 ( (uint32_t) FSb[ ( Y3 >> 16 ) & 0xFF ] << 16 ) ^ 00795 ( (uint32_t) FSb[ ( Y0 >> 24 ) & 0xFF ] << 24 ); 00796 00797 X2 = *RK++ ^ \ 00798 ( (uint32_t) FSb[ ( Y2 ) & 0xFF ] ) ^ 00799 ( (uint32_t) FSb[ ( Y3 >> 8 ) & 0xFF ] << 8 ) ^ 00800 ( (uint32_t) FSb[ ( Y0 >> 16 ) & 0xFF ] << 16 ) ^ 00801 ( (uint32_t) FSb[ ( Y1 >> 24 ) & 0xFF ] << 24 ); 00802 00803 X3 = *RK++ ^ \ 00804 ( (uint32_t) FSb[ ( Y3 ) & 0xFF ] ) ^ 00805 ( (uint32_t) FSb[ ( Y0 >> 8 ) & 0xFF ] << 8 ) ^ 00806 ( (uint32_t) FSb[ ( Y1 >> 16 ) & 0xFF ] << 16 ) ^ 00807 ( (uint32_t) FSb[ ( Y2 >> 24 ) & 0xFF ] << 24 ); 00808 } 00809 00810 PUT_UINT32_LE( X0, output, 0 ); 00811 PUT_UINT32_LE( X1, output, 4 ); 00812 PUT_UINT32_LE( X2, output, 8 ); 00813 PUT_UINT32_LE( X3, output, 12 ); 00814 00815 return( 0 ); 00816 } 00817 00818 #if defined(POLARSSL_CIPHER_MODE_CBC) 00819 /* 00820 * AES-CBC buffer encryption/decryption 00821 */ 00822 int aes_crypt_cbc( aes_context *ctx, 00823 int mode, 00824 size_t length, 00825 unsigned char iv[16], 00826 const unsigned char *input, 00827 unsigned char *output ) 00828 { 00829 int i; 00830 unsigned char temp[16]; 00831 00832 if( length % 16 ) 00833 return( POLARSSL_ERR_AES_INVALID_INPUT_LENGTH ); 00834 00835 #if defined(POLARSSL_PADLOCK_C) && defined(POLARSSL_HAVE_X86) 00836 if( aes_padlock_ace ) 00837 { 00838 if( padlock_xcryptcbc( ctx, mode, length, iv, input, output ) == 0 ) 00839 return( 0 ); 00840 00841 // If padlock data misaligned, we just fall back to 00842 // unaccelerated mode 00843 // 00844 } 00845 #endif 00846 00847 if( mode == AES_DECRYPT ) 00848 { 00849 while( length > 0 ) 00850 { 00851 memcpy( temp, input, 16 ); 00852 aes_crypt_ecb( ctx, mode, input, output ); 00853 00854 for( i = 0; i < 16; i++ ) 00855 output[i] = (unsigned char)( output[i] ^ iv[i] ); 00856 00857 memcpy( iv, temp, 16 ); 00858 00859 input += 16; 00860 output += 16; 00861 length -= 16; 00862 } 00863 } 00864 else 00865 { 00866 while( length > 0 ) 00867 { 00868 for( i = 0; i < 16; i++ ) 00869 output[i] = (unsigned char)( input[i] ^ iv[i] ); 00870 00871 aes_crypt_ecb( ctx, mode, output, output ); 00872 memcpy( iv, output, 16 ); 00873 00874 input += 16; 00875 output += 16; 00876 length -= 16; 00877 } 00878 } 00879 00880 return( 0 ); 00881 } 00882 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00883 00884 #if defined(POLARSSL_CIPHER_MODE_CFB) 00885 /* 00886 * AES-CFB128 buffer encryption/decryption 00887 */ 00888 int aes_crypt_cfb128( aes_context *ctx, 00889 int mode, 00890 size_t length, 00891 size_t *iv_off, 00892 unsigned char iv[16], 00893 const unsigned char *input, 00894 unsigned char *output ) 00895 { 00896 int c; 00897 size_t n = *iv_off; 00898 00899 if( mode == AES_DECRYPT ) 00900 { 00901 while( length-- ) 00902 { 00903 if( n == 0 ) 00904 aes_crypt_ecb( ctx, AES_ENCRYPT, iv, iv ); 00905 00906 c = *input++; 00907 *output++ = (unsigned char)( c ^ iv[n] ); 00908 iv[n] = (unsigned char) c; 00909 00910 n = ( n + 1 ) & 0x0F; 00911 } 00912 } 00913 else 00914 { 00915 while( length-- ) 00916 { 00917 if( n == 0 ) 00918 aes_crypt_ecb( ctx, AES_ENCRYPT, iv, iv ); 00919 00920 iv[n] = *output++ = (unsigned char)( iv[n] ^ *input++ ); 00921 00922 n = ( n + 1 ) & 0x0F; 00923 } 00924 } 00925 00926 *iv_off = n; 00927 00928 return( 0 ); 00929 } 00930 00931 /* 00932 * AES-CFB8 buffer encryption/decryption 00933 */ 00934 int aes_crypt_cfb8( aes_context *ctx, 00935 int mode, 00936 size_t length, 00937 unsigned char iv[16], 00938 const unsigned char *input, 00939 unsigned char *output ) 00940 { 00941 unsigned char c; 00942 unsigned char ov[17]; 00943 00944 while( length-- ) 00945 { 00946 memcpy( ov, iv, 16 ); 00947 aes_crypt_ecb( ctx, AES_ENCRYPT, iv, iv ); 00948 00949 if( mode == AES_DECRYPT ) 00950 ov[16] = *input; 00951 00952 c = *output++ = (unsigned char)( iv[0] ^ *input++ ); 00953 00954 if( mode == AES_ENCRYPT ) 00955 ov[16] = c; 00956 00957 memcpy( iv, ov + 1, 16 ); 00958 } 00959 00960 return( 0 ); 00961 } 00962 #endif /*POLARSSL_CIPHER_MODE_CFB */ 00963 00964 #if defined(POLARSSL_CIPHER_MODE_CTR) 00965 /* 00966 * AES-CTR buffer encryption/decryption 00967 */ 00968 int aes_crypt_ctr( aes_context *ctx, 00969 size_t length, 00970 size_t *nc_off, 00971 unsigned char nonce_counter[16], 00972 unsigned char stream_block[16], 00973 const unsigned char *input, 00974 unsigned char *output ) 00975 { 00976 int c, i; 00977 size_t n = *nc_off; 00978 00979 while( length-- ) 00980 { 00981 if( n == 0 ) { 00982 aes_crypt_ecb( ctx, AES_ENCRYPT, nonce_counter, stream_block ); 00983 00984 for( i = 16; i > 0; i-- ) 00985 if( ++nonce_counter[i - 1] != 0 ) 00986 break; 00987 } 00988 c = *input++; 00989 *output++ = (unsigned char)( c ^ stream_block[n] ); 00990 00991 n = ( n + 1 ) & 0x0F; 00992 } 00993 00994 *nc_off = n; 00995 00996 return( 0 ); 00997 } 00998 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00999 01000 #endif /* !POLARSSL_AES_ALT */ 01001 01002 #if defined(POLARSSL_SELF_TEST) 01003 /* 01004 * AES test vectors from: 01005 * 01006 * http://csrc.nist.gov/archive/aes/rijndael/rijndael-vals.zip 01007 */ 01008 static const unsigned char aes_test_ecb_dec[3][16] = 01009 { 01010 { 0x44, 0x41, 0x6A, 0xC2, 0xD1, 0xF5, 0x3C, 0x58, 01011 0x33, 0x03, 0x91, 0x7E, 0x6B, 0xE9, 0xEB, 0xE0 }, 01012 { 0x48, 0xE3, 0x1E, 0x9E, 0x25, 0x67, 0x18, 0xF2, 01013 0x92, 0x29, 0x31, 0x9C, 0x19, 0xF1, 0x5B, 0xA4 }, 01014 { 0x05, 0x8C, 0xCF, 0xFD, 0xBB, 0xCB, 0x38, 0x2D, 01015 0x1F, 0x6F, 0x56, 0x58, 0x5D, 0x8A, 0x4A, 0xDE } 01016 }; 01017 01018 static const unsigned char aes_test_ecb_enc[3][16] = 01019 { 01020 { 0xC3, 0x4C, 0x05, 0x2C, 0xC0, 0xDA, 0x8D, 0x73, 01021 0x45, 0x1A, 0xFE, 0x5F, 0x03, 0xBE, 0x29, 0x7F }, 01022 { 0xF3, 0xF6, 0x75, 0x2A, 0xE8, 0xD7, 0x83, 0x11, 01023 0x38, 0xF0, 0x41, 0x56, 0x06, 0x31, 0xB1, 0x14 }, 01024 { 0x8B, 0x79, 0xEE, 0xCC, 0x93, 0xA0, 0xEE, 0x5D, 01025 0xFF, 0x30, 0xB4, 0xEA, 0x21, 0x63, 0x6D, 0xA4 } 01026 }; 01027 01028 #if defined(POLARSSL_CIPHER_MODE_CBC) 01029 static const unsigned char aes_test_cbc_dec[3][16] = 01030 { 01031 { 0xFA, 0xCA, 0x37, 0xE0, 0xB0, 0xC8, 0x53, 0x73, 01032 0xDF, 0x70, 0x6E, 0x73, 0xF7, 0xC9, 0xAF, 0x86 }, 01033 { 0x5D, 0xF6, 0x78, 0xDD, 0x17, 0xBA, 0x4E, 0x75, 01034 0xB6, 0x17, 0x68, 0xC6, 0xAD, 0xEF, 0x7C, 0x7B }, 01035 { 0x48, 0x04, 0xE1, 0x81, 0x8F, 0xE6, 0x29, 0x75, 01036 0x19, 0xA3, 0xE8, 0x8C, 0x57, 0x31, 0x04, 0x13 } 01037 }; 01038 01039 static const unsigned char aes_test_cbc_enc[3][16] = 01040 { 01041 { 0x8A, 0x05, 0xFC, 0x5E, 0x09, 0x5A, 0xF4, 0x84, 01042 0x8A, 0x08, 0xD3, 0x28, 0xD3, 0x68, 0x8E, 0x3D }, 01043 { 0x7B, 0xD9, 0x66, 0xD5, 0x3A, 0xD8, 0xC1, 0xBB, 01044 0x85, 0xD2, 0xAD, 0xFA, 0xE8, 0x7B, 0xB1, 0x04 }, 01045 { 0xFE, 0x3C, 0x53, 0x65, 0x3E, 0x2F, 0x45, 0xB5, 01046 0x6F, 0xCD, 0x88, 0xB2, 0xCC, 0x89, 0x8F, 0xF0 } 01047 }; 01048 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01049 01050 #if defined(POLARSSL_CIPHER_MODE_CFB) 01051 /* 01052 * AES-CFB128 test vectors from: 01053 * 01054 * http://csrc.nist.gov/publications/nistpubs/800-38a/sp800-38a.pdf 01055 */ 01056 static const unsigned char aes_test_cfb128_key[3][32] = 01057 { 01058 { 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6, 01059 0xAB, 0xF7, 0x15, 0x88, 0x09, 0xCF, 0x4F, 0x3C }, 01060 { 0x8E, 0x73, 0xB0, 0xF7, 0xDA, 0x0E, 0x64, 0x52, 01061 0xC8, 0x10, 0xF3, 0x2B, 0x80, 0x90, 0x79, 0xE5, 01062 0x62, 0xF8, 0xEA, 0xD2, 0x52, 0x2C, 0x6B, 0x7B }, 01063 { 0x60, 0x3D, 0xEB, 0x10, 0x15, 0xCA, 0x71, 0xBE, 01064 0x2B, 0x73, 0xAE, 0xF0, 0x85, 0x7D, 0x77, 0x81, 01065 0x1F, 0x35, 0x2C, 0x07, 0x3B, 0x61, 0x08, 0xD7, 01066 0x2D, 0x98, 0x10, 0xA3, 0x09, 0x14, 0xDF, 0xF4 } 01067 }; 01068 01069 static const unsigned char aes_test_cfb128_iv[16] = 01070 { 01071 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01072 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F 01073 }; 01074 01075 static const unsigned char aes_test_cfb128_pt[64] = 01076 { 01077 0x6B, 0xC1, 0xBE, 0xE2, 0x2E, 0x40, 0x9F, 0x96, 01078 0xE9, 0x3D, 0x7E, 0x11, 0x73, 0x93, 0x17, 0x2A, 01079 0xAE, 0x2D, 0x8A, 0x57, 0x1E, 0x03, 0xAC, 0x9C, 01080 0x9E, 0xB7, 0x6F, 0xAC, 0x45, 0xAF, 0x8E, 0x51, 01081 0x30, 0xC8, 0x1C, 0x46, 0xA3, 0x5C, 0xE4, 0x11, 01082 0xE5, 0xFB, 0xC1, 0x19, 0x1A, 0x0A, 0x52, 0xEF, 01083 0xF6, 0x9F, 0x24, 0x45, 0xDF, 0x4F, 0x9B, 0x17, 01084 0xAD, 0x2B, 0x41, 0x7B, 0xE6, 0x6C, 0x37, 0x10 01085 }; 01086 01087 static const unsigned char aes_test_cfb128_ct[3][64] = 01088 { 01089 { 0x3B, 0x3F, 0xD9, 0x2E, 0xB7, 0x2D, 0xAD, 0x20, 01090 0x33, 0x34, 0x49, 0xF8, 0xE8, 0x3C, 0xFB, 0x4A, 01091 0xC8, 0xA6, 0x45, 0x37, 0xA0, 0xB3, 0xA9, 0x3F, 01092 0xCD, 0xE3, 0xCD, 0xAD, 0x9F, 0x1C, 0xE5, 0x8B, 01093 0x26, 0x75, 0x1F, 0x67, 0xA3, 0xCB, 0xB1, 0x40, 01094 0xB1, 0x80, 0x8C, 0xF1, 0x87, 0xA4, 0xF4, 0xDF, 01095 0xC0, 0x4B, 0x05, 0x35, 0x7C, 0x5D, 0x1C, 0x0E, 01096 0xEA, 0xC4, 0xC6, 0x6F, 0x9F, 0xF7, 0xF2, 0xE6 }, 01097 { 0xCD, 0xC8, 0x0D, 0x6F, 0xDD, 0xF1, 0x8C, 0xAB, 01098 0x34, 0xC2, 0x59, 0x09, 0xC9, 0x9A, 0x41, 0x74, 01099 0x67, 0xCE, 0x7F, 0x7F, 0x81, 0x17, 0x36, 0x21, 01100 0x96, 0x1A, 0x2B, 0x70, 0x17, 0x1D, 0x3D, 0x7A, 01101 0x2E, 0x1E, 0x8A, 0x1D, 0xD5, 0x9B, 0x88, 0xB1, 01102 0xC8, 0xE6, 0x0F, 0xED, 0x1E, 0xFA, 0xC4, 0xC9, 01103 0xC0, 0x5F, 0x9F, 0x9C, 0xA9, 0x83, 0x4F, 0xA0, 01104 0x42, 0xAE, 0x8F, 0xBA, 0x58, 0x4B, 0x09, 0xFF }, 01105 { 0xDC, 0x7E, 0x84, 0xBF, 0xDA, 0x79, 0x16, 0x4B, 01106 0x7E, 0xCD, 0x84, 0x86, 0x98, 0x5D, 0x38, 0x60, 01107 0x39, 0xFF, 0xED, 0x14, 0x3B, 0x28, 0xB1, 0xC8, 01108 0x32, 0x11, 0x3C, 0x63, 0x31, 0xE5, 0x40, 0x7B, 01109 0xDF, 0x10, 0x13, 0x24, 0x15, 0xE5, 0x4B, 0x92, 01110 0xA1, 0x3E, 0xD0, 0xA8, 0x26, 0x7A, 0xE2, 0xF9, 01111 0x75, 0xA3, 0x85, 0x74, 0x1A, 0xB9, 0xCE, 0xF8, 01112 0x20, 0x31, 0x62, 0x3D, 0x55, 0xB1, 0xE4, 0x71 } 01113 }; 01114 #endif /* POLARSSL_CIPHER_MODE_CFB */ 01115 01116 #if defined(POLARSSL_CIPHER_MODE_CTR) 01117 /* 01118 * AES-CTR test vectors from: 01119 * 01120 * http://www.faqs.org/rfcs/rfc3686.html 01121 */ 01122 01123 static const unsigned char aes_test_ctr_key[3][16] = 01124 { 01125 { 0xAE, 0x68, 0x52, 0xF8, 0x12, 0x10, 0x67, 0xCC, 01126 0x4B, 0xF7, 0xA5, 0x76, 0x55, 0x77, 0xF3, 0x9E }, 01127 { 0x7E, 0x24, 0x06, 0x78, 0x17, 0xFA, 0xE0, 0xD7, 01128 0x43, 0xD6, 0xCE, 0x1F, 0x32, 0x53, 0x91, 0x63 }, 01129 { 0x76, 0x91, 0xBE, 0x03, 0x5E, 0x50, 0x20, 0xA8, 01130 0xAC, 0x6E, 0x61, 0x85, 0x29, 0xF9, 0xA0, 0xDC } 01131 }; 01132 01133 static const unsigned char aes_test_ctr_nonce_counter[3][16] = 01134 { 01135 { 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x00, 01136 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01 }, 01137 { 0x00, 0x6C, 0xB6, 0xDB, 0xC0, 0x54, 0x3B, 0x59, 01138 0xDA, 0x48, 0xD9, 0x0B, 0x00, 0x00, 0x00, 0x01 }, 01139 { 0x00, 0xE0, 0x01, 0x7B, 0x27, 0x77, 0x7F, 0x3F, 01140 0x4A, 0x17, 0x86, 0xF0, 0x00, 0x00, 0x00, 0x01 } 01141 }; 01142 01143 static const unsigned char aes_test_ctr_pt[3][48] = 01144 { 01145 { 0x53, 0x69, 0x6E, 0x67, 0x6C, 0x65, 0x20, 0x62, 01146 0x6C, 0x6F, 0x63, 0x6B, 0x20, 0x6D, 0x73, 0x67 }, 01147 01148 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01149 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 01150 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 01151 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F }, 01152 01153 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 01154 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 01155 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 01156 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F, 01157 0x20, 0x21, 0x22, 0x23 } 01158 }; 01159 01160 static const unsigned char aes_test_ctr_ct[3][48] = 01161 { 01162 { 0xE4, 0x09, 0x5D, 0x4F, 0xB7, 0xA7, 0xB3, 0x79, 01163 0x2D, 0x61, 0x75, 0xA3, 0x26, 0x13, 0x11, 0xB8 }, 01164 { 0x51, 0x04, 0xA1, 0x06, 0x16, 0x8A, 0x72, 0xD9, 01165 0x79, 0x0D, 0x41, 0xEE, 0x8E, 0xDA, 0xD3, 0x88, 01166 0xEB, 0x2E, 0x1E, 0xFC, 0x46, 0xDA, 0x57, 0xC8, 01167 0xFC, 0xE6, 0x30, 0xDF, 0x91, 0x41, 0xBE, 0x28 }, 01168 { 0xC1, 0xCF, 0x48, 0xA8, 0x9F, 0x2F, 0xFD, 0xD9, 01169 0xCF, 0x46, 0x52, 0xE9, 0xEF, 0xDB, 0x72, 0xD7, 01170 0x45, 0x40, 0xA4, 0x2B, 0xDE, 0x6D, 0x78, 0x36, 01171 0xD5, 0x9A, 0x5C, 0xEA, 0xAE, 0xF3, 0x10, 0x53, 01172 0x25, 0xB2, 0x07, 0x2F } 01173 }; 01174 01175 static const int aes_test_ctr_len[3] = 01176 { 16, 32, 36 }; 01177 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01178 01179 /* 01180 * Checkup routine 01181 */ 01182 int aes_self_test( int verbose ) 01183 { 01184 int ret = 0, i, j, u, v; 01185 unsigned char key[32]; 01186 unsigned char buf[64]; 01187 unsigned char iv[16]; 01188 #if defined(POLARSSL_CIPHER_MODE_CBC) 01189 unsigned char prv[16]; 01190 #endif 01191 #if defined(POLARSSL_CIPHER_MODE_CTR) || defined(POLARSSL_CIPHER_MODE_CFB) 01192 size_t offset; 01193 #endif 01194 #if defined(POLARSSL_CIPHER_MODE_CTR) 01195 int len; 01196 unsigned char nonce_counter[16]; 01197 unsigned char stream_block[16]; 01198 #endif 01199 aes_context ctx; 01200 01201 memset( key, 0, 32 ); 01202 aes_init( &ctx ); 01203 01204 /* 01205 * ECB mode 01206 */ 01207 for( i = 0; i < 6; i++ ) 01208 { 01209 u = i >> 1; 01210 v = i & 1; 01211 01212 if( verbose != 0 ) 01213 polarssl_printf( " AES-ECB-%3d (%s): ", 128 + u * 64, 01214 ( v == AES_DECRYPT ) ? "dec" : "enc" ); 01215 01216 memset( buf, 0, 16 ); 01217 01218 if( v == AES_DECRYPT ) 01219 { 01220 aes_setkey_dec( &ctx, key, 128 + u * 64 ); 01221 01222 for( j = 0; j < 10000; j++ ) 01223 aes_crypt_ecb( &ctx, v, buf, buf ); 01224 01225 if( memcmp( buf, aes_test_ecb_dec[u], 16 ) != 0 ) 01226 { 01227 if( verbose != 0 ) 01228 polarssl_printf( "failed\n" ); 01229 01230 ret = 1; 01231 goto exit; 01232 } 01233 } 01234 else 01235 { 01236 aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01237 01238 for( j = 0; j < 10000; j++ ) 01239 aes_crypt_ecb( &ctx, v, buf, buf ); 01240 01241 if( memcmp( buf, aes_test_ecb_enc[u], 16 ) != 0 ) 01242 { 01243 if( verbose != 0 ) 01244 polarssl_printf( "failed\n" ); 01245 01246 ret = 1; 01247 goto exit; 01248 } 01249 } 01250 01251 if( verbose != 0 ) 01252 polarssl_printf( "passed\n" ); 01253 } 01254 01255 if( verbose != 0 ) 01256 polarssl_printf( "\n" ); 01257 01258 #if defined(POLARSSL_CIPHER_MODE_CBC) 01259 /* 01260 * CBC mode 01261 */ 01262 for( i = 0; i < 6; i++ ) 01263 { 01264 u = i >> 1; 01265 v = i & 1; 01266 01267 if( verbose != 0 ) 01268 polarssl_printf( " AES-CBC-%3d (%s): ", 128 + u * 64, 01269 ( v == AES_DECRYPT ) ? "dec" : "enc" ); 01270 01271 memset( iv , 0, 16 ); 01272 memset( prv, 0, 16 ); 01273 memset( buf, 0, 16 ); 01274 01275 if( v == AES_DECRYPT ) 01276 { 01277 aes_setkey_dec( &ctx, key, 128 + u * 64 ); 01278 01279 for( j = 0; j < 10000; j++ ) 01280 aes_crypt_cbc( &ctx, v, 16, iv, buf, buf ); 01281 01282 if( memcmp( buf, aes_test_cbc_dec[u], 16 ) != 0 ) 01283 { 01284 if( verbose != 0 ) 01285 polarssl_printf( "failed\n" ); 01286 01287 ret = 1; 01288 goto exit; 01289 } 01290 } 01291 else 01292 { 01293 aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01294 01295 for( j = 0; j < 10000; j++ ) 01296 { 01297 unsigned char tmp[16]; 01298 01299 aes_crypt_cbc( &ctx, v, 16, iv, buf, buf ); 01300 01301 memcpy( tmp, prv, 16 ); 01302 memcpy( prv, buf, 16 ); 01303 memcpy( buf, tmp, 16 ); 01304 } 01305 01306 if( memcmp( prv, aes_test_cbc_enc[u], 16 ) != 0 ) 01307 { 01308 if( verbose != 0 ) 01309 polarssl_printf( "failed\n" ); 01310 01311 ret = 1; 01312 goto exit; 01313 } 01314 } 01315 01316 if( verbose != 0 ) 01317 polarssl_printf( "passed\n" ); 01318 } 01319 01320 if( verbose != 0 ) 01321 polarssl_printf( "\n" ); 01322 #endif /* POLARSSL_CIPHER_MODE_CBC */ 01323 01324 #if defined(POLARSSL_CIPHER_MODE_CFB) 01325 /* 01326 * CFB128 mode 01327 */ 01328 for( i = 0; i < 6; i++ ) 01329 { 01330 u = i >> 1; 01331 v = i & 1; 01332 01333 if( verbose != 0 ) 01334 polarssl_printf( " AES-CFB128-%3d (%s): ", 128 + u * 64, 01335 ( v == AES_DECRYPT ) ? "dec" : "enc" ); 01336 01337 memcpy( iv, aes_test_cfb128_iv, 16 ); 01338 memcpy( key, aes_test_cfb128_key[u], 16 + u * 8 ); 01339 01340 offset = 0; 01341 aes_setkey_enc( &ctx, key, 128 + u * 64 ); 01342 01343 if( v == AES_DECRYPT ) 01344 { 01345 memcpy( buf, aes_test_cfb128_ct[u], 64 ); 01346 aes_crypt_cfb128( &ctx, v, 64, &offset, iv, buf, buf ); 01347 01348 if( memcmp( buf, aes_test_cfb128_pt, 64 ) != 0 ) 01349 { 01350 if( verbose != 0 ) 01351 polarssl_printf( "failed\n" ); 01352 01353 ret = 1; 01354 goto exit; 01355 } 01356 } 01357 else 01358 { 01359 memcpy( buf, aes_test_cfb128_pt, 64 ); 01360 aes_crypt_cfb128( &ctx, v, 64, &offset, iv, buf, buf ); 01361 01362 if( memcmp( buf, aes_test_cfb128_ct[u], 64 ) != 0 ) 01363 { 01364 if( verbose != 0 ) 01365 polarssl_printf( "failed\n" ); 01366 01367 ret = 1; 01368 goto exit; 01369 } 01370 } 01371 01372 if( verbose != 0 ) 01373 polarssl_printf( "passed\n" ); 01374 } 01375 01376 if( verbose != 0 ) 01377 polarssl_printf( "\n" ); 01378 #endif /* POLARSSL_CIPHER_MODE_CFB */ 01379 01380 #if defined(POLARSSL_CIPHER_MODE_CTR) 01381 /* 01382 * CTR mode 01383 */ 01384 for( i = 0; i < 6; i++ ) 01385 { 01386 u = i >> 1; 01387 v = i & 1; 01388 01389 if( verbose != 0 ) 01390 polarssl_printf( " AES-CTR-128 (%s): ", 01391 ( v == AES_DECRYPT ) ? "dec" : "enc" ); 01392 01393 memcpy( nonce_counter, aes_test_ctr_nonce_counter[u], 16 ); 01394 memcpy( key, aes_test_ctr_key[u], 16 ); 01395 01396 offset = 0; 01397 aes_setkey_enc( &ctx, key, 128 ); 01398 01399 if( v == AES_DECRYPT ) 01400 { 01401 len = aes_test_ctr_len[u]; 01402 memcpy( buf, aes_test_ctr_ct[u], len ); 01403 01404 aes_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01405 buf, buf ); 01406 01407 if( memcmp( buf, aes_test_ctr_pt[u], len ) != 0 ) 01408 { 01409 if( verbose != 0 ) 01410 polarssl_printf( "failed\n" ); 01411 01412 ret = 1; 01413 goto exit; 01414 } 01415 } 01416 else 01417 { 01418 len = aes_test_ctr_len[u]; 01419 memcpy( buf, aes_test_ctr_pt[u], len ); 01420 01421 aes_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01422 buf, buf ); 01423 01424 if( memcmp( buf, aes_test_ctr_ct[u], len ) != 0 ) 01425 { 01426 if( verbose != 0 ) 01427 polarssl_printf( "failed\n" ); 01428 01429 ret = 1; 01430 goto exit; 01431 } 01432 } 01433 01434 if( verbose != 0 ) 01435 polarssl_printf( "passed\n" ); 01436 } 01437 01438 if( verbose != 0 ) 01439 polarssl_printf( "\n" ); 01440 #endif /* POLARSSL_CIPHER_MODE_CTR */ 01441 01442 ret = 0; 01443 01444 exit: 01445 aes_free( &ctx ); 01446 01447 return( ret ); 01448 } 01449 01450 #endif /* POLARSSL_SELF_TEST */ 01451 01452 #endif /* POLARSSL_AES_C */ 01453
Generated on Tue Jul 12 2022 13:50:36 by
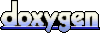