
Dreamforce 2015 BLE-based mDS HeartRate Monitor Endpoint
Dependencies: GroveEarbudSensor mbed mbedConnectorInterface mbedEndpointNetwork_BLE
LocationResource.h
00001 /** 00002 * @file LocationResource.h 00003 * @brief mbed CoAP Endpoint Location resource supporting CoAP GET 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __LOCATION_RESOURCE_H__ 00024 #define __LOCATION_RESOURCE_H__ 00025 00026 // Base class 00027 #include "DynamicResource.h" 00028 00029 // main.cpp can enable/disable the experimental location source 00030 #if ENABLE_BLE_LOCATION 00031 // our BLE Location source 00032 #include "BLELocation.h" 00033 extern RawSerial pc; // main.cpp 00034 BLELocation _ble_location(&pc); // BLE Location from the UART Proxy application 00035 #endif 00036 00037 // We have a static location by default - Moscone West - 37.783879,-122.4012538, altitude 30m msl 00038 #define DEF_LATITUDE 37.783879 00039 #define DEF_LONGITUDE -122.4012538 00040 #define DEF_ALTITUDE 30.0 00041 #define DEF_SPEED 0.0 00042 00043 // Maximum Location JSON Length : {"latitude":XXX.YYYYYY, "longitude":XXX.YYYYYY, "msl":XXXXXX, "speed":XXXXXX} 00044 #define LOCATION_JSON_LENGTH 256 00045 char __location_json[LOCATION_JSON_LENGTH+1]; 00046 00047 /** LocationResource class 00048 */ 00049 class LocationResource : public DynamicResource 00050 { 00051 public: 00052 /** 00053 Default constructor 00054 @param logger input logger instance for this resource 00055 @param name input the Location resource name 00056 @param observable input the resource is Observable (default: FALSE) 00057 */ 00058 LocationResource(const Logger *logger,const char *name,const bool observable = false) : DynamicResource(logger,name,"Location",SN_GRS_GET_ALLOWED,observable) { 00059 memset(__location_json,0,LOCATION_JSON_LENGTH+1); 00060 #if ENABLE_BLE_LOCATION 00061 _ble_location.setDefault(DEF_LATITUDE,DEF_LONGITUDE,DEF_ALTITUDE,DEF_SPEED); 00062 #endif 00063 } 00064 00065 /** 00066 Get the value of the Location sensor 00067 @returns string containing the location value 00068 */ 00069 virtual string get() { 00070 //_ble_location.updateLocation(); 00071 memset(__location_json,0,LOCATION_JSON_LENGTH); 00072 #if ENABLE_BLE_LOCATION 00073 sprintf(__location_json,"{\"latitude\":%s,\"longitude\":%s,\"msl\":%s,\"speed\":%s,\"src\":\"proxy\"}", 00074 _ble_location.getLatitude(), 00075 _ble_location.getLongitude(), 00076 _ble_location.getMSLAltitude(), // in meters 00077 _ble_location.getSpeed()); // in meters/second 00078 #else 00079 sprintf(__location_json,"{\"latitude\":%.6f,\"longitude\":%.6f,\"msl\":%.1f,\"speed\":%.1f,\"src\":\"static\"}", 00080 DEF_LATITUDE, 00081 DEF_LONGITUDE, 00082 DEF_ALTITUDE, // in meters 00083 DEF_SPEED); // in meters/second 00084 #endif 00085 return string(__location_json); 00086 } 00087 }; 00088 00089 #endif // __LOCATION_RESOURCE_H__
Generated on Thu Jul 21 2022 02:55:03 by
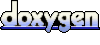