
Dreamforce 2015 BLE-based mDS HeartRate Monitor Endpoint
Dependencies: GroveEarbudSensor mbed mbedConnectorInterface mbedEndpointNetwork_BLE
HeartrateResource.h
00001 /** 00002 * @file HeartrateResource.h 00003 * @brief mbed CoAP Endpoint Heartrate resource (Grove Earbud) supporting CoAP GET and PUT 00004 * @author Doug Anson 00005 * @version 1.0 00006 * @see 00007 * 00008 * Copyright (c) 2014 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef __HEARTRATE_RESOURCE_H__ 00024 #define __HEARTRATE_RESOURCE_H__ 00025 00026 // Base class 00027 #include "DynamicResource.h" 00028 00029 // which Nordic board? 00030 #ifdef TARGET_NRF51_DK 00031 #define ENDPOINT_INTERRUPT_PIN P0_14 00032 #endif 00033 #ifdef TARGET_NRF51_DONGLE 00034 #define ENDPOINT_INTERRUPT_PIN P0_15 00035 #endif 00036 #ifdef TARGET_NRF51_MKIT 00037 #define ENDPOINT_INTERRUPT_PIN P0 00038 #endif 00039 #ifdef TARGET_K64F 00040 #define ENDPOINT_INTERRUPT_PIN D2 00041 #endif 00042 00043 // Grove Earbud Sensor 00044 #include "GroveEarbudSensor.h" 00045 //extern RawSerial pc; // declared in main.cpp for logger... just reference here... 00046 GroveEarbudSensor __earbud(ENDPOINT_INTERRUPT_PIN,&pc); 00047 00048 // HRM sensor value 00049 static char __hrm[10]; 00050 00051 // forward declaration 00052 extern void __hrm_callback(float value,void *instance); 00053 00054 /** HeartrateResource class 00055 */ 00056 class HeartrateResource : public DynamicResource 00057 { 00058 00059 public: 00060 /** 00061 Default constructor 00062 @param logger input logger instance for this resource 00063 @param name input the Light resource name 00064 @param observable input the resource is Observable (default: FALSE) 00065 */ 00066 HeartrateResource(const Logger *logger,const char *name,const bool observable = false) : DynamicResource(logger,name,"Heartrate",SN_GRS_GET_ALLOWED,observable) { 00067 // init... 00068 memset(__hrm,0,10); 00069 sprintf(__hrm,"0.0"); 00070 00071 // finish setup of the earbud sensor 00072 __earbud.registerCallback(&__hrm_callback,this); 00073 00074 // this resource implements its own observation handler... 00075 this->m_implements_observation = true; 00076 } 00077 00078 /** 00079 Get the value of the Heartrate sensor 00080 @returns string containing the heartrate in bpm 00081 */ 00082 virtual string get() { 00083 return string(__hrm); 00084 } 00085 }; 00086 00087 // Heartrate callback (Earbud) 00088 void __hrm_callback(float heartrate,void *data) { 00089 memset(__hrm,0,10); 00090 sprintf(__hrm,"%.1f",heartrate); 00091 00092 // observation handler implementation 00093 if (data != NULL) { 00094 HeartrateResource *res = (HeartrateResource *)data; 00095 if (res->isObservable()) { 00096 res->observe(); 00097 } 00098 } 00099 } 00100 00101 #endif // __HEARTRATE_RESOURCE_H__
Generated on Thu Jul 21 2022 02:55:03 by
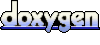