Library for inclusion of main.cpp
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* Copyright C2014 ARM, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files the "Software", to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "Definitions.h" 00020 #include "Logger.h" 00021 00022 // appboard LCD Panel 00023 #if _NXP_PLATFORM 00024 #include "C12832_lcd.h" 00025 C12832_LCD lcd; 00026 #endif 00027 #if _UBLOX_PLATFORM 00028 #include "C12832.h" 00029 C12832 lcd(D11, D13, D12, D7, D10); 00030 #endif 00031 00032 // our Serial port 00033 #include "BufferedSerial.h" 00034 BufferedSerial pc(USBTX, USBRX); 00035 00036 // Ethernet 00037 #include "EthernetInterface.h" 00038 EthernetInterface ethernet; 00039 00040 // HTTP 00041 #include "HTTPClient.h" 00042 HTTPClient http; 00043 00044 // HARD RESET 00045 extern "C" void HardFault_Handler() { NVIC_SystemReset(); } 00046 00047 #ifdef MAC_ADDRESS 00048 char mac[6] = {MAC_ADDRESS}; 00049 extern "C" void mbed_mac_address(char *s) { for(int i=0;i<6;++i) s[i] = mac[i]; } 00050 char fmt_mac[RESOURCE_VALUE_LEN+1]; 00051 #endif 00052 00053 // Main Task... 00054 void mainTask(void const *v) { 00055 // create our object instances 00056 #if _NXP_PLATFORM 00057 Logger logger(&pc,&lcd); 00058 #endif 00059 #if _UBLOX_PLATFORM 00060 Logger logger(&pc,&lcd); 00061 #endif 00062 #if _K64F_PLATFORM 00063 Logger logger(&pc,NULL); 00064 #endif 00065 00066 // announce 00067 logger.log("ARM/DreamForce 2014 Hello salesforce v%s",APP_VERSION); 00068 logger.turnLEDBlue(); 00069 00070 // initialize Ethernet 00071 logger.log("Initializing Ethernet..."); 00072 ethernet.init(); 00073 00074 // get a DHCP address and bring the network interface up 00075 logger.log("Getting IP Address..."); 00076 logger.turnLEDOrange(); 00077 if (ethernet.connect() == 0) { 00078 // log our IP address (DHCP) 00079 logger.log("IP Address: %s",ethernet.getIPAddress()); 00080 00081 // allocate something here... 00082 00083 // entering main loop 00084 logger.log("Entering Main Loop...\r\nStarting main loop..."); 00085 logger.turnLEDGreen(); 00086 00087 // Enter the main loop 00088 while(true) { 00089 // Do work with something here... 00090 Thread::wait(10*WAIT_TIME_MS);; 00091 } 00092 } 00093 else { 00094 logger.log("No Network... Exiting..."); 00095 logger.turnLEDRed(); 00096 exit(1); 00097 } 00098 00099 // disconnect 00100 logger.log("Disconnecting..."); 00101 logger.turnLEDOrange(); 00102 ethernet.disconnect(); 00103 00104 // Exit 00105 logger.log("Exiting..."); 00106 logger.turnLEDBlue(); 00107 exit(1); 00108 } 00109 00110 // main entry 00111 int main() { 00112 #if _K64F_PLATFORM 00113 Thread workerTask(mainTask, NULL, osPriorityNormal, STACK_SIZE); 00114 while (true) { 00115 Thread::wait(10*WAIT_TIME_MS); 00116 } 00117 #else 00118 mainTask(NULL); 00119 #endif 00120 }
Generated on Tue Jul 26 2022 08:30:54 by
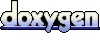