
test public
Dependencies: HttpServer_snapshot_mbed-os
ESP32 Class Reference
ESP32Interface class. More...
#include <ESP32.h>
Public Member Functions | |
bool | get_version_info (char *ver_info, int buf_size) |
Checks Version Information. | |
bool | set_mode (int mode) |
Sets the Wi-Fi Mode. | |
bool | dhcp (bool enabled, int mode) |
Enable/Disable DHCP. | |
bool | connect (const char *ap, const char *passPhrase) |
Connect ESP32 to AP. | |
bool | disconnect (void) |
Disconnect ESP32 from AP. | |
const char * | getIPAddress (void) |
Get the IP address of ESP32. | |
const char * | getMACAddress (void) |
Get the MAC address of ESP32. | |
const char * | getGateway () |
Get the local gateway. | |
const char * | getNetmask () |
Get the local network mask. | |
bool | isConnected (void) |
Check if ESP32 is conenected. | |
int | scan (WiFiAccessPoint *res, unsigned limit) |
Scan for available networks. | |
bool | open (const char *type, int id, const char *addr, int port, int opt=0) |
Open a socketed connection. | |
bool | send (int id, const void *data, uint32_t amount) |
Sends data to an open socket. | |
int32_t | recv (int id, void *data, uint32_t amount, uint32_t timeout=ESP32_RECV_TIMEOUT) |
Receives data from an open socket. | |
bool | close (int id, bool wait_close=false) |
Closes a socket. | |
void | setTimeout (uint32_t timeout_ms=ESP32_MISC_TIMEOUT) |
Allows timeout to be changed between commands. | |
bool | readable () |
Checks if data is available. | |
bool | writeable () |
Checks if data can be written. | |
void | attach_wifi_status (mbed::Callback< void(int8_t)> status_cb) |
Attach a function to call whenever network state has changed. | |
int8_t | get_wifi_status () const |
Get the connection status. | |
bool | ble_set_role (int role) |
Sets BLE Role. | |
bool | ble_get_role (int *role) |
Gets BLE Role. | |
bool | ble_set_device_name (const char *name) |
Sets BLE Device's Name. | |
bool | ble_get_device_name (char *name) |
Gets BLE Device's Name. | |
bool | ble_start_services () |
GATTS Creates and Starts Services. | |
bool | ble_set_scan_response (const uint8_t *data, int len) |
Sets BLE Scan Response. | |
bool | ble_start_advertising () |
Starts Advertising. | |
bool | ble_stop_advertising () |
Stops Advertising. | |
bool | ble_set_addr (int addr_type, const uint8_t *random_addr=NULL) |
Sets BLE Device's Address. | |
bool | ble_get_addr (uint8_t *public_addr) |
Gets BLE Device's Address. | |
bool | ble_set_advertising_param (const advertising_param_t *param) |
Sets Parameters of Advertising. | |
bool | ble_set_advertising_data (const uint8_t *data, int len) |
Sets Advertising Data. | |
bool | ble_set_service (const gatt_service_t *service_list, int num) |
GATT Sets Service. | |
bool | ble_set_characteristic (int srv_index, int char_index, const uint8_t *data, int len) |
GATTS Sets Characteristic. | |
bool | ble_notifies_characteristic (int srv_index, int char_index, const uint8_t *data, int len) |
GATTS Notifies of Characteristics. | |
bool | ble_set_scan_param (int scan_type, int own_addr_type, int filter_policy, int scan_interval, int scan_window) |
Sets Parameters of BLE Scanning. | |
bool | ble_start_scan (int interval=0) |
Enables BLE Scanning. | |
bool | ble_stop_scan () |
Disables BLE scan. | |
bool | ble_connect (int conn_index, const uint8_t *remote_addr) |
Establishes BLE connection. | |
bool | ble_disconnect (int conn_index) |
Ends BLE connection. | |
bool | ble_discovery_service (int conn_index, ble_primary_service_t *service, int *num) |
GATTC Discovers Primary Services. | |
bool | ble_discovery_characteristics (int conn_index, int srv_index, ble_discovers_char_t *discovers_char, int *char_num, ble_discovers_desc_t *discovers_desc, int *desc_num) |
GATTC Discovers Characteristics. | |
int32_t | ble_read_characteristic (int conn_index, int srv_index, int char_index, uint8_t *data, int amount) |
GATTC Reads a Characteristic. | |
int32_t | ble_read_descriptor (int conn_index, int srv_index, int char_index, int desc_index, uint8_t *data, int amount) |
GATTC Reads a Descriptor. | |
bool | ble_write_characteristic (int conn_index, int srv_index, int char_index, const uint8_t *data, int amount) |
GATTC Writes Characteristic. | |
bool | ble_write_descriptor (int conn_index, int srv_index, int char_index, int desc_index, const uint8_t *data, int amount) |
GATTC Writes Descriptor. | |
void | ble_process_oob (uint32_t timeout, bool all) |
For executing OOB processing on background. | |
void | ble_attach_sigio (mbed::Callback< void()> cb_func) |
Register a callback on state change. | |
void | ble_attach_conn (mbed::Callback< void(int, uint8_t *)> cb_func) |
Attach a function to call whenever the BLE connection establishes. | |
void | ble_attach_disconn (mbed::Callback< void(int)> cb_func) |
Attach a function to call whenever the BLE connection ends. | |
void | ble_attach_write (mbed::Callback< void(ble_packet_t *)> cb_func) |
Attach a function to call whenever characteristic data is written. | |
void | ble_attach_scan (mbed::Callback< void(ble_scan_t *)> cb_func) |
Attach a function to call whenever scan data is received. | |
Static Public Member Functions | |
static ESP32 * | getESP32Inst (PinName en, PinName io0, PinName tx, PinName rx, bool debug, PinName rts, PinName cts, int baudrate) |
Static method to create or retrieve the single ESP32 instance. |
Detailed Description
ESP32Interface class.
This is an interface to a ESP32 radio.
Definition at line 53 of file ESP32.h.
Member Function Documentation
void attach_wifi_status | ( | mbed::Callback< void(int8_t)> | status_cb ) |
void ble_attach_conn | ( | mbed::Callback< void(int, uint8_t *)> | cb_func ) |
void ble_attach_disconn | ( | mbed::Callback< void(int)> | cb_func ) |
void ble_attach_scan | ( | mbed::Callback< void(ble_scan_t *)> | cb_func ) |
void ble_attach_sigio | ( | mbed::Callback< void()> | cb_func ) |
Register a callback on state change.
The specified callback will be called on state changes.
The callback may be called in an interrupt context and should not perform expensive operations.
Note! This is not intended as an attach-like asynchronous api, but rather as a building block for constructing such functionality.
The exact timing of when the registered function is called is not guaranteed and susceptible to change. It should be used as a cue to make ble_process_oobl calls to find the current state.
- Parameters:
-
cb_func function to call on state change
void ble_attach_write | ( | mbed::Callback< void(ble_packet_t *)> | cb_func ) |
bool ble_connect | ( | int | conn_index, |
const uint8_t * | remote_addr | ||
) |
Establishes BLE connection.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. remote_addr Remote BLE address
- Returns:
- true: success, false: failure
bool ble_disconnect | ( | int | conn_index ) |
bool ble_discovery_characteristics | ( | int | conn_index, |
int | srv_index, | ||
ble_discovers_char_t * | discovers_char, | ||
int * | char_num, | ||
ble_discovers_desc_t * | discovers_desc, | ||
int * | desc_num | ||
) |
GATTC Discovers Characteristics.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. srv_index Service's index. It can be fetched with "ble_discovery_service()" discovers_char Characteristic info char_num Number of characteristic info discovers_desc Descriptor info desc_num Number of descriptor info
- Returns:
- true: success, false: failure
bool ble_discovery_service | ( | int | conn_index, |
ble_primary_service_t * | service, | ||
int * | num | ||
) |
GATTC Discovers Primary Services.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. service Service info num Number of service info
- Returns:
- true: success, false: failure
bool ble_get_addr | ( | uint8_t * | public_addr ) |
bool ble_get_device_name | ( | char * | name ) |
bool ble_get_role | ( | int * | role ) |
bool ble_notifies_characteristic | ( | int | srv_index, |
int | char_index, | ||
const uint8_t * | data, | ||
int | len | ||
) |
void ble_process_oob | ( | uint32_t | timeout, |
bool | all | ||
) |
int32_t ble_read_characteristic | ( | int | conn_index, |
int | srv_index, | ||
int | char_index, | ||
uint8_t * | data, | ||
int | amount | ||
) |
GATTC Reads a Characteristic.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. srv_index Service's index. It can be fetched with "ble_discovery_service()" char_index Characteristic's index. It can be fetched with "ble_discovery_characteristics()" data Read data buffer amount Amount of bytes to be received
- Returns:
- Data size of received
int32_t ble_read_descriptor | ( | int | conn_index, |
int | srv_index, | ||
int | char_index, | ||
int | desc_index, | ||
uint8_t * | data, | ||
int | amount | ||
) |
GATTC Reads a Descriptor.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. srv_index Service's index. It can be fetched with "ble_discovery_service()" char_index Characteristic's index. It can be fetched with "ble_discovery_characteristics()" desc_index Descriptor's index. It can be fetched with "ble_discovery_characteristics()" data Read data buffer amount Amount of bytes to be received
- Returns:
- true: success, false: failure
bool ble_set_addr | ( | int | addr_type, |
const uint8_t * | random_addr = NULL |
||
) |
bool ble_set_advertising_data | ( | const uint8_t * | data, |
int | len | ||
) |
bool ble_set_advertising_param | ( | const advertising_param_t * | param ) |
bool ble_set_characteristic | ( | int | srv_index, |
int | char_index, | ||
const uint8_t * | data, | ||
int | len | ||
) |
bool ble_set_device_name | ( | const char * | name ) |
bool ble_set_role | ( | int | role ) |
bool ble_set_scan_param | ( | int | scan_type, |
int | own_addr_type, | ||
int | filter_policy, | ||
int | scan_interval, | ||
int | scan_window | ||
) |
Sets Parameters of BLE Scanning.
- Parameters:
-
scan_type 0: passive scan 1: active scan own_addr_type 0: public address 1: random address 2: RPA public address 3: RPA random address filter_policy 0: BLE_SCAN_FILTER_ALLOW_ALL 1: BLE_SCAN_FILTER_ALLOW_ONLY_WLST 2: BLE_SCAN_FILTER_ALLOW_UND_RPA_DIR 3: BLE_SCAN_FILTER_ALLOW_WLIST_PRA_DIR scan_interval scan interval scan_window scan window
- Returns:
- rue: success, false: failure
bool ble_set_scan_response | ( | const uint8_t * | data, |
int | len | ||
) |
bool ble_set_service | ( | const gatt_service_t * | service_list, |
int | num | ||
) |
bool ble_start_advertising | ( | ) |
bool ble_start_scan | ( | int | interval = 0 ) |
bool ble_start_services | ( | ) |
bool ble_stop_advertising | ( | ) |
bool ble_stop_scan | ( | ) |
bool ble_write_characteristic | ( | int | conn_index, |
int | srv_index, | ||
int | char_index, | ||
const uint8_t * | data, | ||
int | amount | ||
) |
GATTC Writes Characteristic.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. srv_index Service's index. It can be fetched with "ble_discovery_service()" char_index Characteristic's index. It can be fetched with "ble_discovery_characteristics()" data Write data buffer amount Amount of data to be written
- Returns:
- true: success, false: failure
bool ble_write_descriptor | ( | int | conn_index, |
int | srv_index, | ||
int | char_index, | ||
int | desc_index, | ||
const uint8_t * | data, | ||
int | amount | ||
) |
GATTC Writes Descriptor.
- Parameters:
-
conn_index Index of BLE connection; only 0 is supported for the single connection right now, but multiple BLE connections will be supported in the future. srv_index Service's index. It can be fetched with "ble_discovery_service()" char_index Characteristic's index. It can be fetched with "ble_discovery_characteristics()" desc_index Descriptor's index. It can be fetched with "ble_discovery_characteristics()" data Write data buffer amount Amount of data to be written
- Returns:
- true: success, false: failure
bool close | ( | int | id, |
bool | wait_close = false |
||
) |
bool connect | ( | const char * | ap, |
const char * | passPhrase | ||
) |
bool dhcp | ( | bool | enabled, |
int | mode | ||
) |
bool disconnect | ( | void | ) |
bool get_version_info | ( | char * | ver_info, |
int | buf_size | ||
) |
int8_t get_wifi_status | ( | ) | const |
ESP32 * getESP32Inst | ( | PinName | en, |
PinName | io0, | ||
PinName | tx, | ||
PinName | rx, | ||
bool | debug, | ||
PinName | rts, | ||
PinName | cts, | ||
int | baudrate | ||
) | [static] |
const char * getGateway | ( | ) |
const char * getIPAddress | ( | void | ) |
const char * getMACAddress | ( | void | ) |
const char * getNetmask | ( | ) |
bool isConnected | ( | void | ) |
bool open | ( | const char * | type, |
int | id, | ||
const char * | addr, | ||
int | port, | ||
int | opt = 0 |
||
) |
Open a socketed connection.
- Parameters:
-
type the type of socket to open "UDP" or "TCP" id id to give the new socket, valid 0-4 port port to open connection with addr the IP address of the destination addr the IP address of the destination opt type=" UDP" : UDP socket's local port, zero means any type=" TCP" : TCP connection's keep alive time, zero means disabled
- Returns:
- true only if socket opened successfully
int32_t recv | ( | int | id, |
void * | data, | ||
uint32_t | amount, | ||
uint32_t | timeout = ESP32_RECV_TIMEOUT |
||
) |
int scan | ( | WiFiAccessPoint * | res, |
unsigned | limit | ||
) |
Scan for available networks.
- Parameters:
-
ap Pointer to allocated array to store discovered AP limit Size of allocated res array, or 0 to only count available AP
- Returns:
- Number of entries in res, or if count was 0 number of available networks, negative on error see nsapi_error
bool send | ( | int | id, |
const void * | data, | ||
uint32_t | amount | ||
) |
bool set_mode | ( | int | mode ) |
void setTimeout | ( | uint32_t | timeout_ms = ESP32_MISC_TIMEOUT ) |
Generated on Wed Jul 13 2022 05:33:38 by
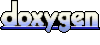